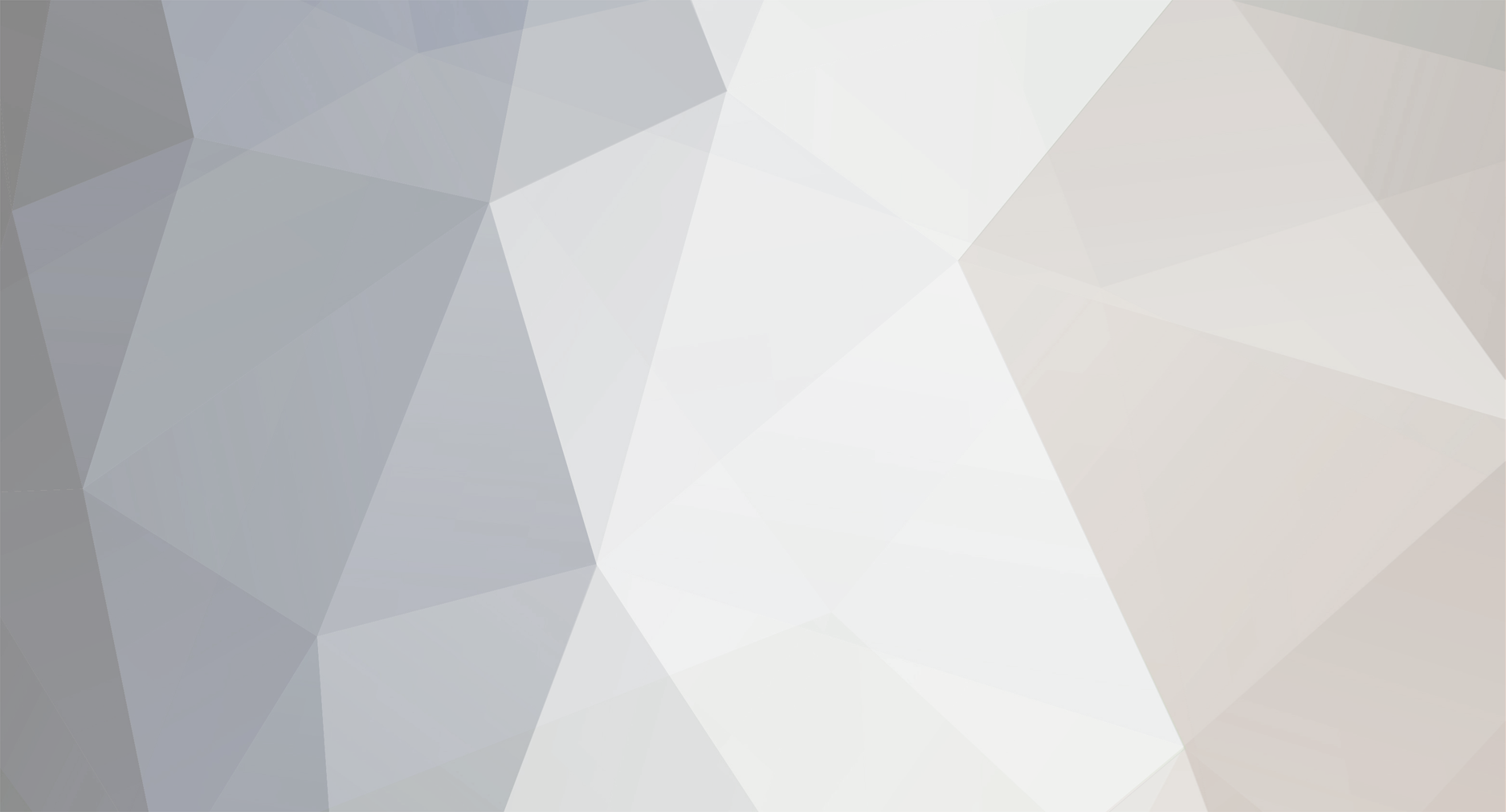
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
Add session_start() before the require.
-
or just run this code: [code=php:0]<?php echo 'Current PHP version: ' . phpversion(); ?>[/code] to get the version of PHP your server is running. However I can confirm that you probably are not running PHP5 if PHP is reporting an error on this line: [code=php:0]private $id;[/code] Your version is most probably PHP4. PHP4 has very low OOP support. PHP5 is the version you will want for creating OOP scripts.
-
Add ob_end_flush(); at the end of your script too (before the closing PHP tag (?>) ob_start starts output buffering. Meaning PHP will not out anything until the script has been parsed. You need to call ob_end_flush which turns off output buffering which will release the buffered contents.
-
You cant put PHP code in a string. I guess what needs to be done is change this: [code]print "<tep_href=\"$partial_path"."_". $all_page . ".php\"><img src=\"images/prev.gif\" alt=\"$all_page\" border=\"0\"></a>"; [/code] to this: [code]print tep_href_link('engtech_1.php');[/code]
-
PHP doesn't understand me (Im telling it to do the wrong thing)
wildteen88 replied to mattd8752's topic in PHP Coding Help
You are setting id and carid's datatype to a string (VARCHAR) These columns should have their data types set to INT not VARCHAR. If your column is VARCHAR then any data in that column is treated as a string - even if its a number. When its INT the data will be treated as an interger not a string. So when you are doing this: [code]$usrid = $usrid + "1"; // OR $carid = $carid + "1";[/code] What you are telling PHP is to add the [b]string[/b] 1 to the end of the [b]string[/b] in $carid so you will be getting 11 all the time. PHP wont add the numbers! In order for PHP to treat it as a number you need change the id and carid data types to INT and remove the quotes from 1. So this will be your query when you create your userdata table: [code]CREATE TABLE `userdata` ( `id` INT(11) NOT NULL default '', `owner` varchar(255) NOT NULL default '', `cash` varchar(255) NOT NULL default '', `carid` INT(11) NOT NULL default '' ) ENGINE=MyISAM DEFAULT CHARSET=latin1;[/code] to change you code so the id column autoincrements you need to change your SQL query for the id column to this: [code]`id` INT( 11 ) NOT NULL AUTO_INCREMENT PRIMARY KEY[/code] MySQL will now increase the id by one automatically when ever you add an entry to the usertable. -
Problem with installing PHP. HELP NEEDED!
wildteen88 replied to mr02077's topic in PHP Installation and Configuration
The mysql or mysqli extension requires an extra file. This file is called libmysql.dll - should be located in C:/php Copy this file to your WINDOWS directory (C:/WINDOWS ( or C:/WINNT if you don't have a WINDOWS folder)). Restart Apache server. Is the library now loaded? -
If you are getting that error then it more like to be an error with your query. Change this line: [code]$result = mysql_query($query, $db->conn); [/code] to: [code]$result = mysql_query($query, $db->conn) or die("Error with query:<br /><pre>{$query}</pre><br /><br >" . mysql_error());[/code] Post the error message you get in full here. [b]EDIT:[/b] Oh poop! I Forgot to set up the conn variable in the db class, Add the following code: [code]var $conn = '';[/code] after [tt]var $dbase = '';[/tt] in the DB class.
-
Best of using herdoc syntax: [code=php:0]echo <<<HTMLBLOCK <!-- Begin: AdBrite --> <br /><div align="center"> <script type="text/javascript"> var AdBrite_Title_Color = '000000'; var AdBrite_Text_Color = '000000'; var AdBrite_Background_Color = 'FFFFFF'; var AdBrite_Border_Color = 'FFFFFF'; </script> <span style="white-space:nowrap;"><script src="http://ads.adbrite.com/mb/text_group.php?sid=197042&zs=3436385f3630" type="text/javascript"></script><!-- --><br /></span> <!-- End: AdBrite --> HTMLBLOCK; // DO NOT PLACE ANYTHINK ON/CHANGE THE LINE ABOVE![/code] It is best to use HEREDOC syntax when echo'ing large amounts of HTML. Note if you use variables within heredoc you may need to wrap it in curly braces (example: [code=php:0]{$varname}[/code])
-
Yes that is correct. However do not use it to get rid of the errors! Always try to fix the errors first!
-
PHP (and other Webprogramlanguages) v.s. WYSIWYG
wildteen88 replied to meanrat's topic in PHP Coding Help
Programmers that use WYSIWYG programs are lazy programmers in my books and depending on... lets say the 'intelligence' (dont know what other word to describe it) behind the WYSIWYG editors as they tend to churn out tones of unneeded code and the code is all messy. Take DW for example use the built in PHP tools and it puts in a lot of unneeded code, especially when it comes to databases! And I agree with thopre's statement if you cant be bothered to learn then don't even bother! WYSWYG editors are OK for doing basic level (web) programming like HTML (and CSS). But not for advanced languages like PHP programming.The only time I use a WYSIWYG editor is when getting the real basics of a layout together quickly. -
Php want me to download it?
wildteen88 replied to wamasterhunter's topic in PHP Installation and Configuration
[quote author=ball420 link=topic=123087.msg512805#msg512805 date=1169683406] i'm having the same problem!! but with my instance i have set up IIS and am using dreamweaver to write my code. when i go to check if my code works or not it ask's me to open save cancel. anything i do it opens in dreamweaver<exept for cancel obviously> even if i right click and choose open with... what is the problem??? i'm running on xp pro. [/quote] You need to do either of the following: - setup a project in Dreamweaver and tell it to use your local server when previewing files (read DW documentation on setting up projects) - or you need to setup IIS to parse PHP files with the PHP interpreter. @[b]wamasterhunter[/b] [quote author=wamasterhunter link=topic=123087.msg508672#msg508672 date=1169225346] What do you mean by that? I have Apache and IIS, Apache is disabled though. [/quote] So which server is setup to parse PHP files with the PHP interpreter? IIS or Apache? -
PHP On My Little Home Computer
wildteen88 replied to tarun's topic in PHP Installation and Configuration
You cannot run .php files by "double clicking" them, like you can with .html or .exe files for example. You have to move it to you servers document root and then go to http://localhost/ to run the php file. PHP is a server side programming language and thus requires to be run in a server setup. Or you can run then via the command line. You can install Apache (the server) and PHP on your PC locally. Read [url=http://www.phpfreaks.com/forums/index.php/topic,120152.msg492660.html#msg492660]this[/url] post of mine to do so. Or you can go the lazy root and install one of those horrid all-in-one installations over at wampserver.com or xampp.com -
Wrap the html in single quotes: [code=php:0]echo '<img src="http://www.mysite.com/path/to/ad/image">';[/code] Note if you do this: [code=php:0]echo "<img src="http://www.mysite.com/path/to/ad/image">";[/code] You will have to escape the double quotes in the string too. So it will be like this: [code=php:0]echo "<img src=\"http://www.mysite.com/path/to/ad/image\">";[/code] If you dont you will get errors. As PHP will think you are ending the print/echo statement and then throw's an unexpecting string in blah bla blah.
-
Have you tried restarting WAMP? Click WAMP's tray icon (small white icon that looks like a speedometer). Right click and and select [b]Restart All Services[/b] You must restart WAMP when you change any of WAMP's configuration files (php.ini, httpd.conf or my.conf). Also I got myself mixed up in my earlier post with WAMP being XAMPP so ignore what I said. ;)
-
[quote author=andrewholway link=topic=121968.msg513157#msg513157 date=1169731695] I dont think apache 2.2 is that stable yet. Especially the windows version.[/quote] WHat you talking about! It is stable. It just you need to use the correct module for the version of PHP you are using! A lot of people have got confused over this when upgrading to Apache2.2.x This is what the different modules are for: php5apache2_2.dll - for use with PHP5.2 (or above) and Apache2.2.x php5apache2.dll - for use with PHP5.x and Apache2.0.x (NOT for Apache2.2.x!) php5apache.dll - for use with PHP5 and Apache1.3.x
-
Here: [code]include 'classes/cls_db.php'; $this->dblink = new DB();[/code] is wrong. The DB Class does not return a MySQL resource link, however it does hold the resource link in a variable called conn within the DB class. What you'll want to do use this when connecting to the database: [code]nclude 'classes/cls_db.php'; $db = new DB();[/code] Then to get the resource link use $db->conn intead of $this->dblink
-
Just include the file that has your database details in your constructor or function that creates the connection to the database Here is a simple database class: [code]class DB { var $host = ''; var $user = ''; var $pass = ''; var $dbase = ''; // this gets called when we initiate the class // this is called a constructor // constructors have the same name as the class function DB() { // change this to location of you php file with you details include 'path/to/your/dbdetails.php'; // setting up the class variables $this->host = $host; $this->user = $user; $this->pass = $pass; $this->dbase = $dbase; // connect to the database automatically $this->connect(); } function connect() { $this->conn = mysql_connect($this->host, $this->user, $this->pass); mysql_select_db($this->dbase, $this->conn); } }[/code] When you go to connect to the database initiate the class: [code]// connect to database. $db = new DB;[/code]
-
If you installed WAMP, the GD should already be enabled as if I rememeber correctly wamp enables other extensions during setup by default which includes GD. Have you got an extra php_gd2.dll from somewhere else?
-
PHP on WinXP local server. I am stuck!
wildteen88 replied to Lengo's topic in PHP Installation and Configuration
You are not supposed to run php-win.exe What are supposed to do is configure PHP with an http server. Apache is an http server and is best to use (IMO anyway). YOu have to editing Apache's configuration in order for PHP to be loaded. I provided the following steps to do this: 1. Find and apaches configuration file (httpd.conf - C:/program files/Apache Group/Apache2/conf). Open in notepad or another text editor add the following at the end of the file: [code]LoadModule php5_module "C:/PHP/php5apache2_2.dll" PHPIniDir "C:/WINDOWS" AddType application/x-httpd-php .php[/code] Save the httpd.conf, Next go to C:\PHP and renamed php.ini-recommended to just php.ini move this file to the WINDOWS folder (C:\WINDOWS). Now open it in notepad and file the following line: [code]extension_dir = "./"[/code] change ./ to C:/PHP/ext (if isn't set to it already). Scroll down further and until you come to the following: [b];extension=php_mbstring.dll[/b] Remove the semi-colon from the start of the line. Now save the php.ini and restart the Apache server. PHP should now be loaded as an Apache module. NOTE: It is best to add the PHP folder to the Windows PATH Variable. I will show you how to if you do not know. Add PHP to the Windows PATH keeps all PHP's files in one location and should keep any problems that may raise with PHPs configuration to a minimum. -
[quote]If I don't tell the form to enter something into the two fields above I get a "column must not be null" message.[/quote] Looks like you will want to set you columns to [tt]NULL[/tt] instead of [tt]NOT NULL[/tt]. If you have PMA (phpMyAdmin) it is easy to do. Just login to PMA got to the table that has the quote and ONT columns, select the checkbox next to the two columns and click the pencil icon under the table. Now change the [b]Null[/b] column to value from NOT NULL to NULL click Save button to apply the changes.
-
remove the Action line: Action Application/x-httpd-php "/PHP5/php.exe" not needed. I believe that's only needed if you load PHP as cgi. But you are loading PHP as a module - recommended. Also try not to install Apache or PHP in the the program files folder. I prefer to install Apache, PHP, MySQL and anything else relating to them in a folder called server in the root of my hard drive.
-
mysqli_real_escape_string shouldn't effect it, as \r and \n are invisible characters. Somewhere in your script it is causing this. What happens when you run nl2br on the string does it convert the \r\n in to a HTML line break ([nobbc]<br />[/nobbc])?
-
You have to provide an absolute url for the new url, the old url has to begin with a forward slash(/) eg: [code] redirect 301 /oldURL http://mysite.com/newURL[/code] So the following should now work: [code]redirect 301 /old_page.html http://mysite.com/new_page.php[/code] Read the [url=http://httpd.apache.org/docs/2.0/mod/mod_alias.html#redirect]Apache documentation[/url] on the redirect directive.
-
I believe you've got to list your column names for the data to go into for the results table. So try this: [code]INSERT INTO results (round1, name, rank, score) SELECT p.round1, p.name, p.rank, p.score FROM players AS p WHERE p.id > 1 ORDER BY p.level DESC LIMIT 10[/code]
-
When dealing with bbcode it is best to you use regex (preg_replace) rather than str_replace. str_replace is only for doing basic string replacements. If you look in the regex board you will see plenty of examples. Here is a very basic example: [code]<?php function bbcode($txt) { // bbcodes $bbcodes = array( "|\[b\](.+?)\[/b\]|is", "|\[u\](.+?)\[/u\]|is", "|\[i\](.+?)\[/i\]|is" ); // html $replace = array( "<strong>$1</strong>", "<u>$1</u>", "<em>$1</em>" ); $txt = preg_replace($bbcodes, $replace, $txt); return nl2br($txt); } $str = "[b]hey[/b] a [u][i]BBCode parser[/i][/u]!"; $str = bbcode($str); echo $str; ?>[/code]