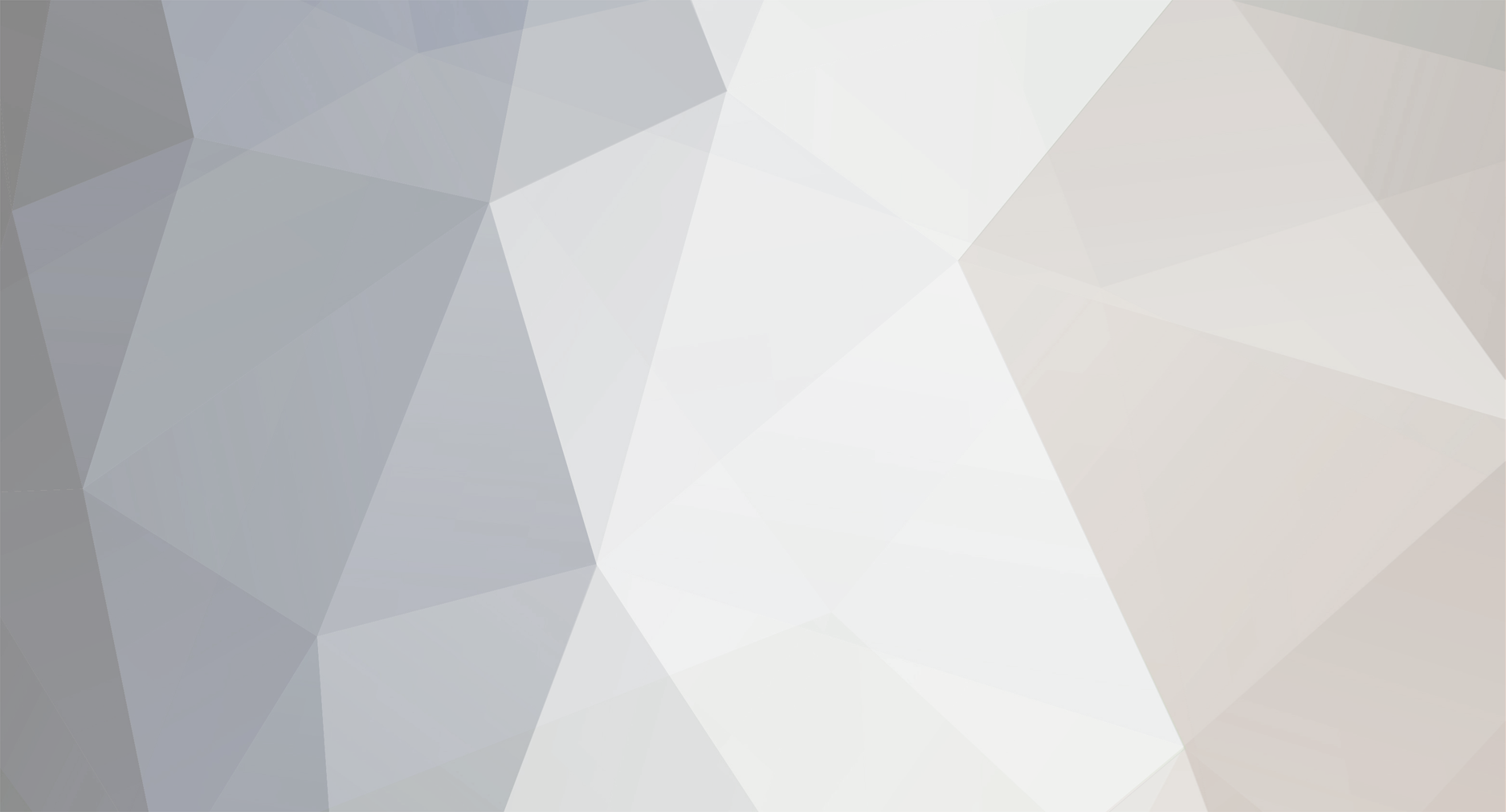
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
Yes when you use variables that is set out side of the function you need to declare them as global, otherwise the variables you use in the functioin will be tied to that function only.
-
If you use sessions it'll be much better as the referer variabled can be spoofed and sometimes it is not set by the clients web browser. So if you have sessions you'll have this block of code: [code]<?php session_start(); if(!isset($_SESSION['loggedIn'] || !$_SESSION['loggedIn'] == 1) { die("Please login you are not authorised to access this page"); } // rest of code here[/code] WHen they login use this: [code]session_start(); $_SESSION['loggedIn'] = 1;[/code] Thats is a far better way of doing what you want to do.
-
HA! No problem. If you have any problems post them here I'll try to help. The script should catch a few errors that may arise.
-
Have a read of the comments here: [code]<?php // connect to your database here // replace prices.txt with your file that stores the info $file = "prices.txt"; // open the file $handle = fopen ($file, 'r'); // get the contents of the file $contents = fread ($handle, 9999); // close file fclose ($handle); // extract each row from the file: $row = explode ("\n", $contents); // get total number of rows $rows = count ($row); // now loop through each row and put each column into the database for($i = 0; $i <= $rows-1; $i++) { // this gets each column from the row! list ($id, $price, $qty) = explode (" ", $row[$i]); // now insert each column into the database! $query = "INSERT INTO table_name (id, price, qty) VALUES ('$id', '$price', '$qty')"; $result = @mysql_query ($query) or ($error[] = "row{$i} failed to insert<br /><code>{$query}</code><br />" . mysql_error() . "<br /><br />"); } if(isset($error)) { foreach ($error as $k => $v) { echo $error[$k]; } } else { echo "Data has been inserted successfully!"; } ?>[/code] Also change where it says [i]table_name (id, price, qty)[/i] in the code above to the table that stores the prices in the database and id, price and qty to the correct field names
-
[!--quoteo(post=387190:date=Jun 23 2006, 04:09 PM:name=wizzkid)--][div class=\'quotetop\']QUOTE(wizzkid @ Jun 23 2006, 04:09 PM) [snapback]387190[/snapback][/div][div class=\'quotemain\'][!--quotec--] Thanks so much wildteen88... Yes, thats right the line break. it was working :) but can I put this on the script? so that the user (the one will enter the article) will not have to type <br/> . is that possible? :) if so, how? :) Then how can I put something like[b] BOLD button [/b][i]ITALICS button[/i] and [u]Underline Button[/u], so the user will just highlight the text and click B, I or U? :) Thank for your help :) [/quote] You use the nl2br function on the variable that stores the news article, which I believe is $row['vc_article'] in view_news.txt. So you'll do this: [code]nl2br($row['vc_article']);[/code]
-
To do what you want to do place the following bit of code: [code]// check whether the 'more' link has not been clicked. // if it hasn't we'll only show the five most recent news articles if(!isset($_GET['more']) || $_GET['more'] == 0) { // this tells MySQL to select only 5 results $query .= " LIMIT 5"; }[/code] after this line: [code]$query = 'SELECT * FROM news ORDER BY date DESC';[/code] Now to add the link you'll need this code: [code]<a href="?more=1">View all</a>[/code] This will need to be placed after you news article is displayed, proabably after the while loop if I'm correct. So now what will happen is PHP will check 'more' has been set in the url and that it is set to '1'. If more is not set in the url it'll append 'LIMIT 5' to end of the query. This will then only show 5 news articles. But if more is set in the url and is set 1 it'll show all the articles. Now to linke the title you'll need to do this: [code]<h3><a href=\"news.php?id={$row['vc_title']}\">{$row['vc_title']}</a></h3>[/code] Change news.php to the correct file you want the user to go to
-
Password Prompt - something is not working
wildteen88 replied to Conjurer's topic in PHP Coding Help
The reason why you get [i]Your Username and Password are not valid![/i] straight away is because you are not checking whether the form has been submitted yet, so PHP is going to parse your PHP whether the form has been submitted or not! To check whether the form has been submitted use this code: [code]<?php // check whether form has been submitted: if(isset($_POST['Submit'])) { // check whether username and password match here } else // form hasnt been submitted so ask them to login { echo "Please login<br />"; } ?> // form code here[/code] -
Yeah session_destory kills the whole session. You'll want to use unset to kill seperate session vars within a session.
-
If you want to do it automatically then it'll require you to setup a cron job which will execute your database backup script. Theres are tutorials [a href=\"http://www.php-mysql-tutorial.com/perform-mysql-backup-php.php\" target=\"_blank\"]here[/a] and [a href=\"http://www.developertutorials.com/tutorials/php/backup-mysql-database-php-050409/page1.html\" target=\"_blank\"]here[/a] on backing up a mysql database with PHP /* Off topic */ Your avator is really distracting! I cant stop looking at it.
-
What do you mean text formatting? Do you mean your text is being displayed in one line, rather than keeping its line breaks that you entered in the textarea? If thats what you mean you'll want to use a function called nl2br which converts \r\n, \n and \r characters into html line breaks (< br />)
-
Your code will not work as PHP is unsetting a session var called 'test$i' it is treating your variable as-is meaning it is not swaping $i with its value. So change your unset code to this: [code]unset($_SESSION['test' . $i]);[/code] Now PHP will parse the variable $i rather than treating it as text. Also if you want to destroy a session you should use session_destroy();
-
If you have phpMyAdmin you can do a full database thgrough that. To do so login to phpMyAdmin and click the Export link on the main page of phpMyAdmin. This now brings up the database backup page. Select the database(s) from the list on the left. To select multiple databases in the list Crtl + Click on the databases you want to backup. Now read through all various options on the right hand side, the defualt settings should do just fine. Now scroll to the bottom of the page and tick the save as file option, then chose a name and the compression of the file. Once finished click Go. phpMyAdmin will now backup the databases you selected and will prompt you to download the backup file. Thats how you do a database backup through phpMyAdmin
-
fopen can only create files it cannot create folders. However mkdir attempts to create a directory.
-
A few here: phpBB myBB Simple Machines
-
One problem I see is that you have deleted the space ( ) in the empty divs. Those spaces will need to be in there, otherwise the cell will collapse in some browers. Also another problem is that you havn't positioned the rows thats why they are stuck to the left.
-
Do you mean the rows? If you do then you haven't positioned them. I'm a little confused. Helps if you could post us an image/ live view of the problem.
-
Installing PHP/MySQL on a PC
wildteen88 replied to ablaye's topic in PHP Installation and Configuration
Try adding your PHP folder to the Windows PATH variable by doing the following: Start -> Control Panel -> double click System icon (classic view). Now click the Advanced tab and click the Environment variables button. Now goto the Systems box and select the PATH variable from the list. Now click the Edit button. Now click the END key on your keyboard. This will make the cursor go to the end of the list of variables. Type in the following: [code];C:\php;[/code] Now click Ok, OK, OK Once you have returned to the desktop you'll need to restart your computer in order for the new Path variable become available. -
Okay no problem! I have missed out a little detail in my last post. You will need to use explode again in your whle loop in order for you to put the the id, price and quanty into their own sepreat columns in the database.
-
You'll want to use a function called nl2br to convert you line breaks into HTML line breaks. The problem is with the browser and not the database. The browser ignores whitespace chars so you need to use nl2br to convert your newline chars into html line breaks
-
You sure its this code? As no errors pop up when I run it through PHP. Also your syntax is a little wrong here: [code]if (isset($_POST['stage']) && ('process' == $_POST['stage'])) {[/code] it should be this: [code]if (isset($_POST['stage']) && ($_POST['stage'] == 'process')) {[/code]
-
Okay to do this you'll need to use the following functions: fopen, fread and fclose to read the contents of the file Next you'll need to use a function called explode, to get each row out of the text file. And finally you'll want to use a while loop to insert each row into the database. Hope that helps. If you need help with coding it thnen first consult the fine manual over at [a href=\"http://www.php.net\" target=\"_blank\"]http://www.php.net[/a] and sue the search box to search for each function I have mentioned in order to understand how to use them. If you're still stuck I'll provide some code.
-
Installing PHP/MySQL on a PC
wildteen88 replied to ablaye's topic in PHP Installation and Configuration
In order for your PHP pages to be parsed you need to configure Apace to send these files to PHP intepreter like so: Find and opne you httpd.conf file for editing. Now find the following line: [code]#LoadModule ssl_module modules/mod_ssl.so[/code] Add the following after it: [code]LoadModule php4_module "C:/php/sapi/php4apache2.dll" #PHPIniDir PHPIniDir "C:/WINDOWS/[/code] [b]Note:[/b] I am assuming you have PHP located in a folder called PHP in the root of the hard drive. Change C:/php to the correct path of you PHP folder. Now scroll down and find this line: [code]DirectoryIndex index.html index.html.var[/code] Now append index.php to the end of that line, like so: [code]DirectoryIndex index.html index.html.var index.php[/code] Now scroll a litttle further and find this line: [code]AddType application/x-gzip .gz .tgz[/code] And add the following after it: [code]AddType application/x-httpd-php .php[/code] Save your httpd.conf and restart Apache. Next open up notepad ands put the following in it [code]<?php phpinfo (); ?>[/code] Save it as phpinfo.php (making sure that the file type pull down menu has [b]all files[/b] selected) and save it to the folder where apache accesses your files, by defualt it is something like [i]C :/program files/apache group/apache2/htdocs[/i] Now open up your web browser and type this in: [a href=\"http://localhost/phpinfo.php\" target=\"_blank\"]http://localhost/phpinfo.php[/a] You should now get a page full of current PHP and server settings. -
Because prior to PHP 4.3.x a setting called register_globals was turned off by defualt, however the tutorials/books you are reading either old or haven't been updated yet. You can tell when a book has been updated as it'll have something like: [i] edition x[/i] some where on the cover. x being the number of times books have been rewritten. But most tutorials was done before PHP 4.3.x was released, however still people tunr this setting register_globals on despite this setting can cause secuirity holes within a script.
-
They are both the same! 4.3 just has bug fixes and secuirty updates that it. Obviously the advantage between the two is go for the latest one as it has the bug fixes and the security holes fixes.
-
if you have no output then it loos like there is an error in your script check your servers error logs to see what the error might be.