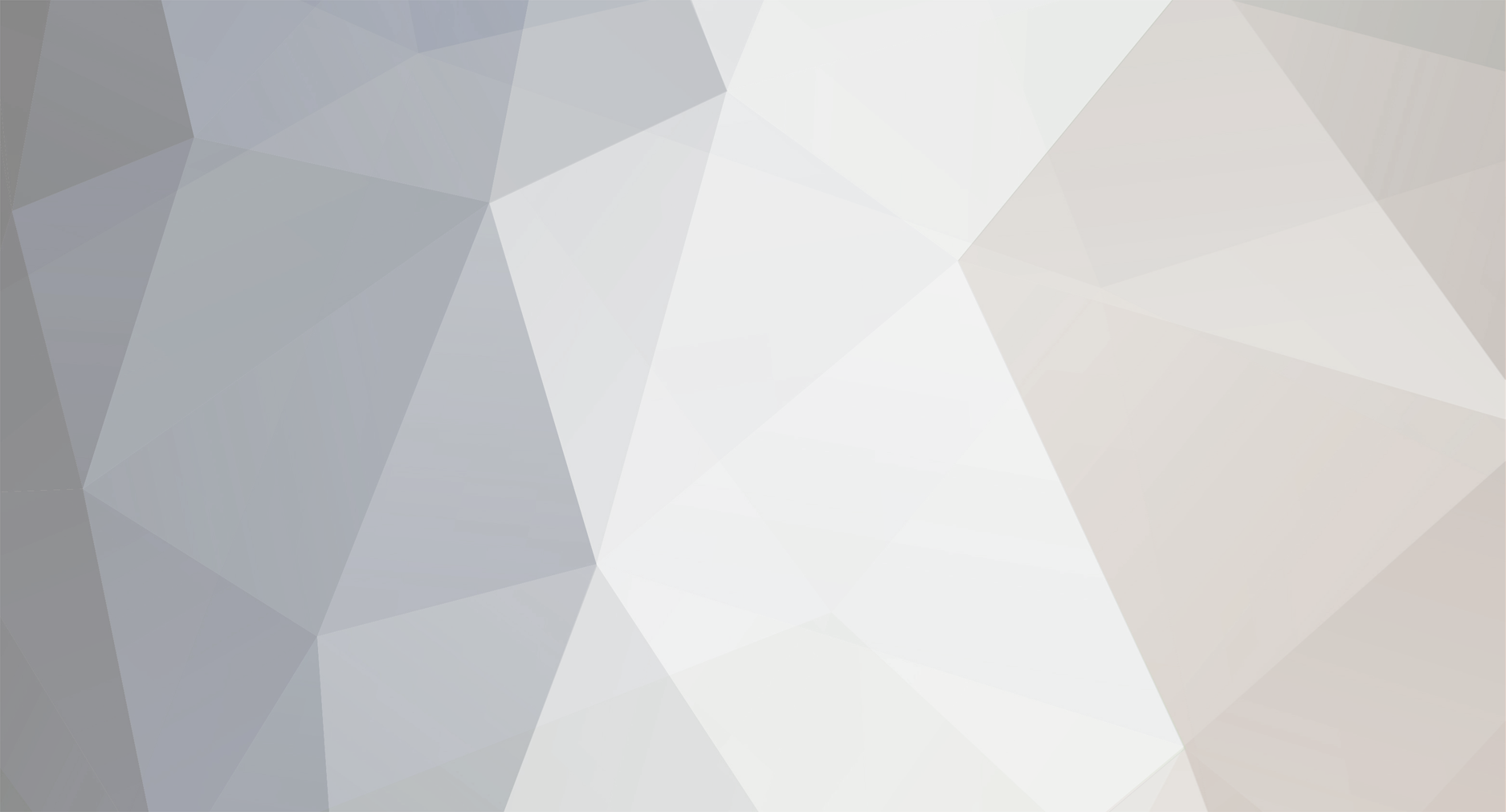
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
You should compare the username/password within the query, if the old password and username matches a record then change the password to the new one. <?php // check that form has been submitted if(isset($_POST['changepass'])) { // grab username and old password $username = $_SESSION['username']; // md5 the old password $old_password = md5($_POST['password']); // make sure the old password matches the current password within the database $sql = "SELECT username, password FROM Member WHERE username='$username' AND password='$old_password"; $result = mysqli_query($cxn, $sql) or die("Query died: password"); // check that there has been a match. if(mysqli_num_rows($result) === 1) { // md5 the new password and update the database $new_password = md5($_POST['newpass']); $sql = "UPDATE Member SET password = '$new_password' WHERE username = '$username'"; mysqli_query($cxn, $sql) or die("Query died: update");; } } ?>
-
For got echo $path in the loop $path = "$base_folder/{$hosting[$i]}"; echo "Crteating the following path: {$path}"; mkdir($path); Post the output here
-
There is no need for that query within your second while loop. You are already fetching the id and locationname from your original query (outside the loop) $donor=@mysql_query('SELECT id, locationname FROM donors'); You can recode the while loop to just <?php while (list($id, $name) = mysql_fetch_row($donor)) { echo "<option value=\"$id\">$name</option>"; } ?>
-
You're passing mkdir an invalid path. To see what the path is change your code to $base_folder = "{$base}uploads/movies/{$year}/{$month}/{$title}/" for$(i = 0; $i < 5; $i++) { if(is_dir($base_folder) { $path = "$base_folder/{$hosting[$i]}"; mkdir($path); } else { echo "{$base_folder} is not a directory"; } }
-
mysql_query only returns a result resource. In order to retrieve the results from the query, you'll need to use one the mysql_fetch_*() functions, for example mysql_fetch_assoc. Example php code $query = 'SELECT thumb FROM escorts WHERE base = 'manchester' ORDER BY RAND()'; $result = mysql_query($query); while($row = mysql_fetch_assoc($result)) { echo $row['thumb'] . '<br />'; }
-
This topic has been moved to HTML Help. http://www.phpfreaks.com/forums/index.php?topic=307147.0
-
Umm, not really. Why what is wrong? Post what you're trying to do and any errors you're getting.
-
You'll want to use regex, an example function gallery($id) { return "[sHOW GALLERY ID: $id]"; } $str = '...Lorem ipsom {gallery:1} sit imet...'; $str = preg_replace('~\{gallery:([0-9]+)\}~ies', 'gallery($1)', $str); echo $str;
-
Form Data Not Appearing in MySQL Database
wildteen88 replied to Skelly123's topic in PHP Coding Help
Just noticed your form <form action="form-data.php" method=”post”> The ” is invalid HTML, it should be a ". Change the quotes and your code should run as expected. -
How to access database fields as an array variables ?
wildteen88 replied to linux1880's topic in PHP Coding Help
Then you'll be defining this array within your controller. What is the name of the variable that contains the array you posted above? -
Form Data Not Appearing in MySQL Database
wildteen88 replied to Skelly123's topic in PHP Coding Help
Post your table structure here. Your code is fine, however placing raw $_POST values into a query is not recommended for security reasons. You should be sanitizing any user input, as suggested by jonsjava earlier. -
When the form has been submitted you'll need to capture the selected value first and then pass this value as a hidden field to your next form.
-
How do I get a form to keep its values after submit?
wildteen88 replied to DeX's topic in PHP Coding Help
If you're submitting the form to itself, then you can do something like this echo '<input type="text" name="field_name" value="' . (isset($_POST['field_name']) ? $_POST['field_name'] : '') . '" />'; -
Installing PHP 5.3 on Apache 2.2 + PHPMyAdmin
wildteen88 replied to Sleipnirx86's topic in PHP Installation and Configuration
phpMyAdmin doesn't require any configuration in its default state, just download phpMyAdmin (zip) from phpmyadmin.net. Create a new folder called phpmyadmin within Apaches htdocs folder (I think the default path is C:/Program Files/Apache Group/Apache2/htdocs). Now extract the zip to the folder you just created. Next rename the file config.sample.inc.php. to config.inc.php. Now open http://localhost/phpmyadmin and away you go. PHPMyAdmins configuration file is the config.inc.php script just edit it as necessary. Documentation is available at phpmyadmin.net. -
fun and frolic with multi-dimensional arrays
wildteen88 replied to ThunderVike's topic in PHP Coding Help
If I'm understanding that loop correctly. You could check to see if $meta_value is an array when checking to see if $meta_key starts with cp_checkbox. If $meta_key does start with cp_checkbox and is an array then you can implode the values into a comma delimited list. So you'd change this if(cp_str_starts_with($meta_key, 'cp_checkbox')) { unserialize($meta_value); add_post_meta($post_id, $meta_key, $meta_value, true);} To if(cp_str_starts_with($meta_key, 'cp_checkbox') && is_array($meta_value)) { // implode the values into a comma delimited list $meta_value = implode(', ', $meta_value); add_post_meta($post_id, $meta_key, $meta_value, true);} -
Store the uploaded files file name within the database, rather than just the id of the photo. That way when you want to display the image you'll use <a href="<?php echo safe_output($photo['web_url']); ?>" target="_blank" class="bannerImg"><img src="banners/<?php echo $photo['filename']; ?>"/></a>
-
Sure, Change the states while loop to while($row = mysql_fetch_array($sql)) { $state = $row['states']; $selected = (isset($_POST['search']) && $_POST['search'] == $state) ? ' selected="selected"' : ''; echo "<option value=\"$state\"$selected>$state</option>"; }//End While
-
There is no right or wrong way. Its down to personal preference really. I'm just used to wrapping HTML attribute values within double quotes.
-
This topic has been moved to MySQL Help. http://www.phpfreaks.com/forums/index.php?topic=306966.0
-
I used the backslash for escaping the (double) quote echo "<option value=\"$state\">$state</option>"; If you remove the slash PHP will report a syntax error, due the string being defined prematurely.
-
fun and frolic with multi-dimensional arrays
wildteen88 replied to ThunderVike's topic in PHP Coding Help
Try the following function // loops through the custom fields and builds the step2 review page function cp_formbuilder_review($results) { global $wpdb; ?> <li><label><strong><?php _e('Category','cp');?>:</strong></label> <div id="review"><?php echo $_POST['catname']; ?></div> <div class="clr"></div> </li> <?php foreach ($results as $result) { $form_field_value = $_POST[$result->field_name]; if(is_array($form_field_value) { $field_review_value = '<ul><li> ' . implode('</li><li>', $form_field_value) . ' </li></ul>'; } else { $field_review_value = stripslashes(nl2br($form_field_value)); } ?> <li> <label><strong><?php echo $result->field_label; ?>:</strong></label> <div id="review"><?php echo $field_review_value; ?></div> <div class="clr"></div> </li> <?php } } -
When using drop down lists you only give the <select> tag a name. But for each <option> you'll assign their value. Example dropdown list <form action="" method="post"> <select name="list_name"> <option value="option1">Option One</option> <option value="option2">Option Two</option> <option value="option3">Option Three</option> </select> <input type="submit" name="submit" value="Submit" /> </form> Notice how each option has a unique value assigned. Now when the form is submitted $_POST['list_name'] will contain the selected value. So to correct your code, change while($row = mysql_fetch_array($sql)){ $state = $row['states']; echo "<option name='statebox'>$state</option>"; }//End While To while($row = mysql_fetch_array($sql)){ $state = $row['states']; echo "<option value=\"$state\">$state</option>"; }//End While Next change $statesearch = $_POST['statebox']; to $statesearch = $_POST['search']; The next two lines can be remove, they are not necessary. $selected = $_POST['statebox']; $select = "<option name='statebox'>$selected</option>"; On your second form you'll want to pass the selected state as a hidden value echo "</select>"; echo "<input type='hidden' name='statesearch' value='$statesearch'> "; echo "<input align='left' type='submit' name='button' value='Search'> ";
-
Post the code that outputs the message. It sounds like you'll need to use htmlentities with the ENT_QUOTES flag when displaying the messages
-
fun and frolic with multi-dimensional arrays
wildteen88 replied to ThunderVike's topic in PHP Coding Help
Umm, Where's the code for the cp_formbuilder_review() function? -
php is not working with MySQL - can't add AND
wildteen88 replied to jeger003's topic in PHP Coding Help
EDIT: Beaten to it $this->sql_query = sprintf("SELECT * FROM %s WHERE category = '%s' AND live=1 AND location = '%s'", $this->classifieds_table , $this->in_statement , mysql_real_escape_string($_COOKIE["State"]) ); If your query is still failing you can get the error message using mysql_error function.