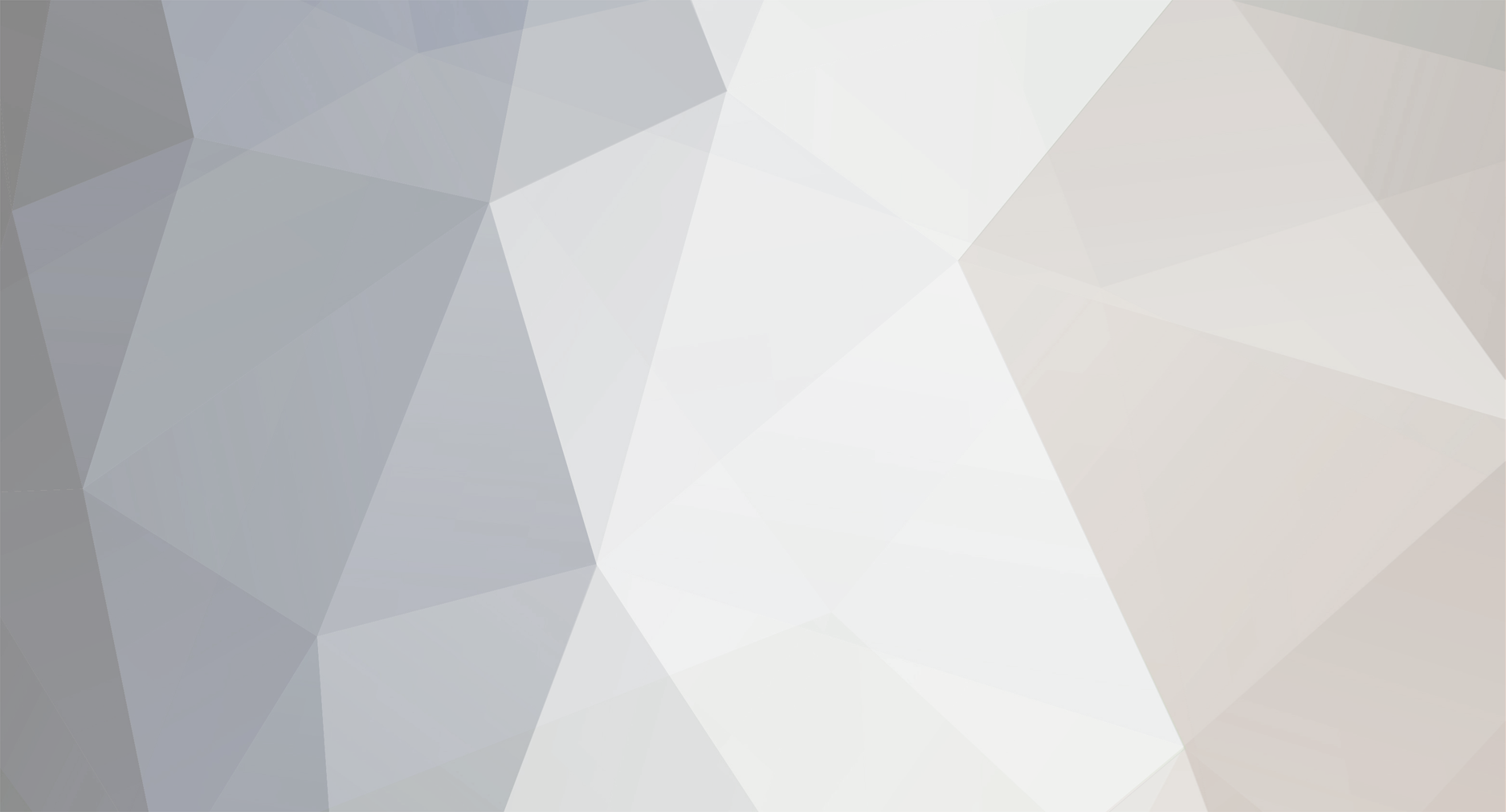
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
You're not processing the results of your queries properly. mysqli_query() only returns a result resource. It doesn't return a multi-dimensional array of results. To grab the results from your query you'll want to use one of the MySQLi Result functions, such as mysqli_fetch_assoc(). You'd then use a while loop to loop through the results. As per the (procedural style) example for mysqli_fetch_assoc here So your code should be <?php $area_result = mysqli_query($cxn, "SELECT areaID, area FROM area ORDER BY areaID"); echo("<ul id='MenuBar1' class='MenuBarHorizontal'>"); echo("<li><a href='index.php'>HOME</a></li>"); echo("<li><a href='#' class='MenuBarItemSubmenu'>PRODUCTS</a><ul>"); while( $h1 = mysqli_fetch_assoc($area_result) ) { echo("<li><a href='#' class='MenuBarItemSubmenu'>".$h1['area']."</a><ul>"); $category_result = mysqli_query($cxn, "SELECT catID, category, areaID, link FROM category WHERE areaID = '".$h1['areaID']."' ORDER BY catID"); while($h2 = mysqli_fetch_assoc($category_result)) { echo("<li><a href='".$h2['link']."'>".$h2['category']."</a></li>"); } echo("</ul></li>"); } echo("</ul></li>"); echo("<li><a href='services.php'>SERVICES</a></li>"); echo("<li><a href='aboutus.php'>ABOUT US</a></li>"); echo("<li><a href='contact.php'>CONTACT</a></li></ul>"); ?>
-
Sure, Look at Multi-column Results FAQ
-
Anyway of Decrypting a md5 Password? Forgot PW Form - HELP
wildteen88 replied to oliverj777's topic in PHP Coding Help
No, md5 cannot be decrypted. What you should do instead is reset their current password with a new one, which will contain a bunch of random letters/numbers etc. They then use this new password to login to their account. -
How to Display an Array Horizontally in A Set Number of Rows?
wildteen88 replied to Modernvox's topic in PHP Coding Help
Multi-column results -
Use nl2br?
-
What are you trying to do? Have a constant header/footer for your site?
-
You will also want to add these lines to your .htaccess file before your rewrite rules RewriteCond %{REQUEST_FILENAME} !-f RewriteCond %{REQUEST_FILENAME} !-d That should make mod rewrite ignore existing files/folders
-
It is a lot easier to use file $lines = file('somefile.txt'); foreach($lines as $line) { echo "$line<br />"; }
-
Add a / in front of the styles sheets file name, eg <link rel="stylesheet" type="text/css" href="/stylesheet.css" /> Now it will always load from the root of your url, eg http://www.somethig.com/stylesheet.css. Otherwise the browser is thinking you're within a directory and is loading the css file in http://www.somethig.com/users/ or http://www.somethig.com/users/pakistan/
-
I'm guessing the new value is in $_POST['amtpaid'] variable. Your query will be like this $sql = "UPDATE testtable SET amtpaid = '" . mysql_real_escape_sting($_POST['amptpaid']) . "' WHERE apt='" . mysql_real_escape_string($_POST['apt'] . "'"; mysql_query($sql) or die("Update query failed."); echo "Record for appartment ".$_POST["apt"]." has been updated ...";
-
The rewrite rule for http://MyWebSite.net/Track/AA.BB.CC.DD Would be RewriteRule Track/([a-z0-9.]+) track.php?ip=$1 [NC,L] To get AA.BB.CC.DD from the url you'd use $_GET['ip']
-
I think what you're after is Multi-column results.
-
You're not updating anything within your SQL query $sql = "UPDATE testtable SET dep, name, amtpaid, prevbal, misc, tentpay, hudpay, datepaid WHERE apt='apt'"; mysql_query($sql) or die("Update query failed."); echo "Record for appartment ".$_POST["apt"]." has been updated ..."; You're listing the fields to update but not providing anyvalues. An update query is formatted like so UPDATE table SET some_field='new value', another_field='some other value' WHERE whatever='something'
-
Open file from web browser (local server) directly into Photoshop
wildteen88 replied to sumonmg's topic in PHP Coding Help
You will not be able to launch an installed application on the users computer with HTML/Javascript/PHP etc. The only way you can do the above is maybe using Java to create a small Java Applet which will record the time the file is opened/saved. It'll then send these times to your PHP application for working out how long the file was worked on for etc. However this will require the user to install this applet onto their PC/Mac. -
Change this line if ($file != "." && $file != "..") { to if (!in_array($file, $filesToIgnore)) { Now before the while() loop define an array of files you want your script to ignore $filesToIgnore = array('.', '..', '.htaccess', 'index.html');
-
file_exists will only function correctly if the full (path and) filename are present, It doesn't support wildcards. You may want to look into the glob function.
-
This topic has been moved to mod_rewrite. http://www.phpfreaks.com/forums/index.php?topic=307587.0
-
A checkbox will only send its value if its is checked. The code you have posted is the correct way for retrieving the value from checkboxes. You will not be able to get the value from an unchecked checkbox Add the following to your code echo "<pre>" . print_r($_POST, true) . "</pre>"; Submit your form with the checkboxes checked and post the output here.
-
If each block, eg "STEAM_0:0:18130940" { "admin" "(Console)" "unban" "1274151724" "time" "1273546924" "name" "-=SAS=- Death Master511" "reason" "Gcombat on spawn/Server Crash Attempts 1 week appeal at halania.com" } is always going to occupy 8 lines. Then you could do something like this <?php function parseBans(&$dataArray) { $bans = array(); // split the array into chunks of 8 // this is because each block occupies 8 lines // Example block /* "STEAM_0:0:18130940" { "admin" "(Console)" "unban" "1274151724" "time" "1273546924" "name" "-=SAS=- Death Master511" "reason" "Gcombat on spawn/Server Crash Attempts 1 week appeal at halania.com" } */ $blocks = array_chunk($dataArray, ; // loop throught each ban block foreach($blocks as $block) { // get the steam id // matches "18130940" from "STEAM_0:0:18130940" preg_match('~[0-9]+)"~', $block[0], $m); $ban['steam_id'] = $m[1]; // remove the "STEAM_0:0:xxxxxxx"", "{" and "}" lines unset($block[0], $block[1], $block[7]); // implode the $block array back into a string $bits = implode($block); //This now leaves us with the following data /* "admin" "(Console)" "unban" "1274151724" "time" "1273546924" "name" "-=SAS=- Death Master511" "reason" "Gcombat on spawn/Server Crash Attempts 1 week appeal at halania.com" */ // parse the above into "key" "value" pairs preg_match_all('~"([a-z]+)"\s+"(.*?)"~', $bits, $pieces, PREG_SET_ORDER); foreach($pieces as $piece) { list(,$key, $value) = $piece; $ban[$key] = $value; } // add the $ban array into the $bans array $bans[] = $ban; } // return the $bans array return $bans; } // read the bans.txt file into an array. $arrayBans = file('bans.txt'); // run the function to parse the text file $bans = parseBans($arrayBans); ?> You'd parse the $bans array into a simple table using echo '<table border="1" cellpadding="10">'; echo '<tr><th>' . implode('</h><th>', array_map('ucwords', array_keys($bans[0]))) . '</th></tr>'; foreach($bans as $ban): echo '<tr><td>' . implode('</td><td>', $ban) . '</td></tr>'; endforeach; echo '</table>';
-
The snippets you have posted are fine. PHP will only be able to retrieve the values from the form once it is submitted, you do have a submit button? Maybe you need to show more code.
-
Removing extra white space in Text area boxes
wildteen88 replied to kevinkhan's topic in PHP Coding Help
All you need to do is replace all newlines with a space, $descr = preg_replace("~(\r?\n)+~", ' ', $descr); -
No need for those two queries. You only need to use one query, a basic join $query = "SELECT p.plt_crp_id, c.crp_name FROM kb3_pilots p, kb3_corps c WHERE p.plt_name='$search' AND p.plt_crp_id = c.crp_id"; $result = mysql_query($query); list($pilotcorpid, $pilotcorpname) = mysql_fetch_row($result); echo "TEST: Pilot Id: $pilotcorpid and corp name: $pilotcorpname";
-
Left off a quote, on line 34 trigger_error('There is a problem with: ' . mysql_error(), E_USER_ERROR); Also the query is wrong $query = "SELECT session_user FROM product_table WHERE session_user = '{$_SESSION['_login_id']}"; It should be $query = "SELECT session_user FROM product_table WHERE session_user = '{$_SESSION['_login_id']}'";
-
Your PHP configuration most probably has a setting called magic_quotes enabled. Which automatically escapes quotes in variables such as _GET, _POST, _COOKIE etc. Reading this will help you http://php.net/manual/en/security.magicquotes.php
-
Make sure you don't have anything on the same as HTML;, this includes whitespace characters such as a space or tabs etc.