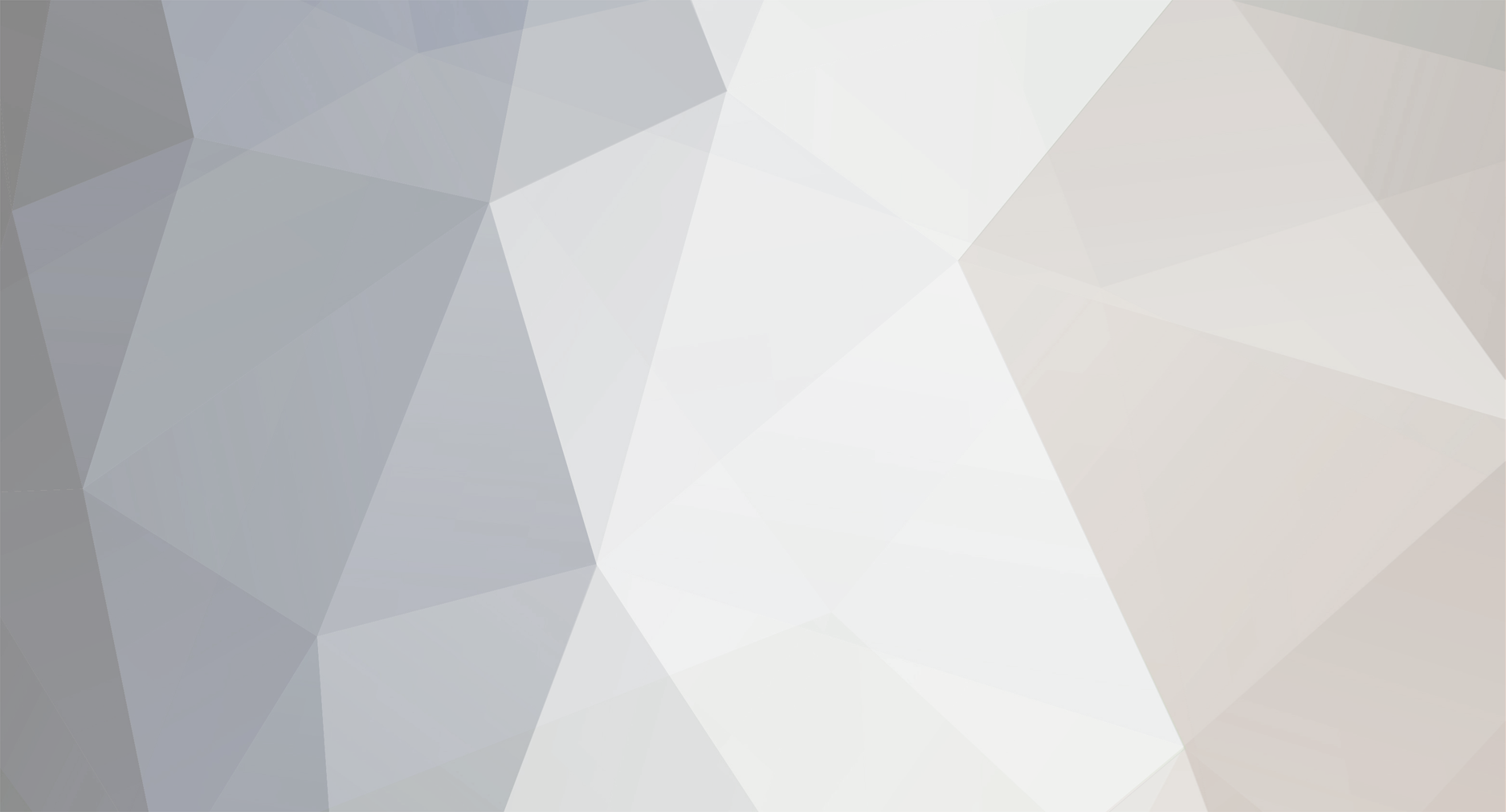
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
To call header.php in areas/area1/index.php you'd use the following path require '../../header.php'; In header.php you'd use the following path to call functions.php require 'functions/functions.php'; To call header.php in the root index.php file you'd use the following file path. require 'header.php';
-
Can you post your sites file structure.. You can go higher up in your directory structure using ../
-
Ok, omit that variable and do require 'testfunc.php';
-
You cannot include a file using a URL. You need to use a local file path! Eg require $_SERVER['DOCUMENT_ROOT'] . '/testfunc.php';
-
echo $content or insert $content into your database.
-
Your query is wrong. $sql = "SELECT * FROM `$mysql_db`.`short`"; You should only select the field named short. Not all fields otherwise the while loop wont work. $sql = "SELECT `short` FROM `$mysql_db`.`short`"; With the above fix, you should test it to see if it works.
-
randNumber($randNumbers); should be $number = randNumber($randomNumbers);
-
Trouble selecting entries from MySQL database - PHP
wildteen88 replied to theanteater's topic in PHP Coding Help
You're not quiet imploding the $genderArray correctly $check_gender = implode(',',$GenderArray); The above will implode the array to "male,female" You'll want to implode the array so it produces the string "'male','female'". The MySQL IN() clause requires each value to be wrapped in quotes. Otherwise it'll be trying to match the gender 'male,female'. Not the gender being either 'male' or 'female'. So change the above line to $check_gender = sprintf('\'%s\'', implode('\',\'',$GenderArray)); Now change your query to $qry = "SELECT * FROM register WHERE gender IN ($check_gender) ORDER BY age"; -
I'd first read the text file using file. Next use in_array to see if the email already exists. For example $leadsFile = "leads/emails.txt"; $emails = file($leadsFile, FILE_IGNORE_NEW_LINES); if(!in_array($conEM, $emails)) { // write email to text file $fh = fopen($leadsFile, 'a') or die ("can't open leads file!"); $writeData = "$conEM\r\n"; fwrite($fh, $writeData); fclose($fh); }
-
I wouldn't query mysql for each unique code you generate as this could cause a lot of server load. As you never know if the function will be called once, five times or even a hundred times (depending on how may codes you have). This is why I suggested in the other post to grab all the current codes within the database and add them to an array. I'd then use this array for checking to see if the current code exists. Like so // a function for getting all codes function getCodes() { $query = 'SELECT short FROM short'; $result = mysql_query($query); $codes = array(); while(list($code) = mysql_fetch_row($result)) $codes[] = $code; return $codes; } // grab the codes array $codes = getCodes(); // this generates the unique code. function getUniqueHash(&$codes) { $code = substr(md5(uniqid(rand(), true)), 0, 4); return ((in_array($code, $codes) ? getUniqueHash($codes) : $code ); } // grab the unique code $unquie_code = getUniqueHash($codes);
-
Oops, sorry. mysq_fetch_row($result) should read mysql_fetch_row($result)
-
Post your code in tags for us to review.
-
Take a look at this thread http://www.phpfreaks.com/forums/index.php/topic,307316.0.html It is a similar question to yours. You should be able to modify the function I posted to your needs.
-
Array has contents, but won't echo...
wildteen88 replied to techiefreak05's topic in PHP Coding Help
How is the array $xconfig being passed to the PageTop function? Functions have their own variable scope, meaning variables that are defined outside of the function will not be accessible to them (and vise versa). When using functions with variables you should pass them as arguments when you call that function, eg function PageTop(&$xconfig){ echo $xconfig['title']; } // index.php: include"config.php"; include "func.inc.php"; PageTop($xconfig); Documentation on variable scope http://php.net/manual/en/language.variables.scope.php Documentation on functions http://www.php.net/manual/en/language.functions.php -
It'll be be better/easier if you displayed a checkbox next to each actor and one button for deleting the actors (at the end of the form) <?php .... // Request query while($REQUESTDR = mysql_fetch_array ($QueryDramActReturn)) { ?> <div style="display: block; float: left; margin-left: 5px; margin-bottom: 5px;"> <?php echo $REQUESTDR['n_actor_fname'] . ' ' . $REQUESTDR['n_actor_name'];?> <input type="checbox" name="DeleteActor[]" value="<?php echo $REQUESTDR['n_dramaact_id'];?>" class="SubmitDelActor" /> </div> <?php }?> .... <input type="submit" name="DelectActorSubmit" value="Delete Selected" /> </form> Now with the checkboxes in place, you can process all the selected checkboxs in one go using if(isset($_POST['DeleteActorSubmit'])) { $actor_ids = implode(',', $_POST['DeleteActor']); $query = 'DELETE FROM `n_dramaact` WHERE `n_dramaact_id` IN('. $actor_ids .')'; mysql_query($query); }
-
You'll have to perform some validation within the foreach loop, eg if(isset($_POST['submit'])) { $values = array(); foreach($_POST['person'] as $person) { // performing some basic validation // checking to see if name and surname is not empty // and that age is a number if(!empty($_POST['name']) && !empty($_POST['surname']) && is_numeric($_POST['age'])) { $name = mysql_real_escape_string($person['name']); $surname = mysql_real_escape_string($person['surname']); $age = mysql_real_escape_string($person['age']); $values[] = "('$name', '$surname', '$age')"; } } if(count($values) > 0) { $query = "INSERT INTO plus_signup (name,surname,age) VALUES " . implode(', ', $values); $result = mysql_query($query) or die("Error!<br />Query: $query<br />Error: " . mysql_error()); } }
-
You will be better off grabbing all current random numbers first and putting them into an array. $sql = 'SELECT randomnumber FROM table'; $result = mysql_query($sql); $randomNumbers = array(); while(list($number) = mysq_fetch_row($result)) $randomNumbers[] = $number; Now we'll create a (recursive) function for generating the next random number, we'll pass the $randomNumbers array to this function. Within this function we'll generate a new random number and see if it is already in the $randomNumber array. If the number has not been picked we'll return the new number, otherwise we'll call the function again until a unique random number has been generated. function randNumber(&$randomNumbers) { // generate the new number $newNumber = rand(10000000, 99999999); // check to see if this number has been picked before if(in_array($newNumber, $randomNumbers)) { // this number has already been picked, call the function again return randNumber($randomNumbers); } // number is unique, return this number else { return $newNumber; } } // call the function $number = randNumber($randNumbers);
-
Post your code here. I think the issue maybe with you using echo within the function. You should instead save all output within the function to a temporary variable and the use return.
-
Wrap ${$test}[$x] in curly braces again, {${$test}[$x]}
-
Yea, you're sort of right there. You'll want to use the less than or equal to operator (<=) rather than equal to (==) on this line. for($i=1; $i == $needed; $i++) { EDIT: Yup you fixed it
-
Post your existing code. You should only need to make a few modifications.
-
I have a mistake in my code. I left off the ' after $old_password on this line $sql = "SELECT username, password FROM Member WHERE username='$username' AND password='$old_password"; Change "; to '";
-
I've not included the code for connecting to mysql/database. You'll need to add that. You may want to add mysqli_error when your query fails
-
You've not closed the string correctly here $message = " Contact Client Email = $Email Add "; after $Email
-
Wrap $test within curly braces echo(${$test}[$x]);