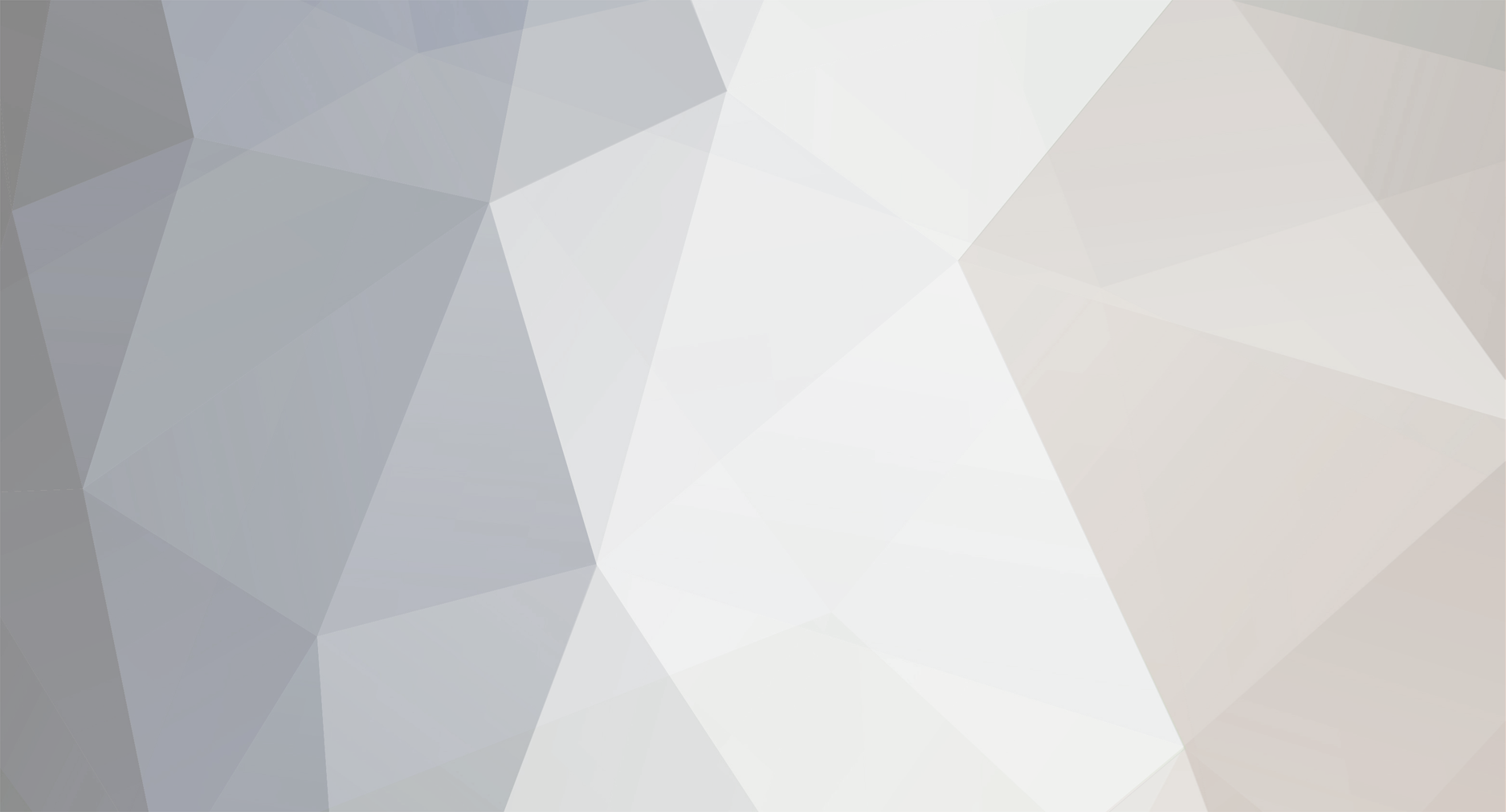
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
Need help checking if $_POST filed is empty
wildteen88 replied to Beauford's topic in PHP Coding Help
You should do it like this if(isset($_POST['field_name_here']) && !empty($_POST['field_name_here'])) { // field has a value } -
Yes. However if nothing is being displayed it means nothing has been returned from your query. In which case you'll need to debug it. Change $username = $_SESSION['username']; $result = mysql_query("SELECT orderID, username, QtyHam, QtyCheese, QtyBLT FROM sandwich WHERE username='$username'"); to $username = $_SESSION['username']; $sql = "SELECT orderID, username, QtyHam, QtyCheese, QtyBLT FROM sandwich WHERE username='$username'"; $result = mysql_query($sql) or die('MySQL Error!<br />Query: '.htmlentities($sql).'<br />'.mysql_error());
-
The correct syntax for a drop down menu is <select name="aid"> <option value="value1">Value 1</option> <option value="value2">Value 2</option> <option value="value3">Value 3</option> </select> In PHP it'll be // start of form/menu echo '<form> <select name="aid">'; // produce the <option>'s while($row_ID = mysql_fetch_array($result)) { echo '<option value="'.$row_ID['AID'].'">'.$row_ID['AID'].'</option>'; } // end the menu/form echo '</select> </form>';
-
Yea you'll need to connect to mysql in order for your queries to work (I didnt check that earlier) That because mysql_query() returns nothing else but a result resource. To grab the results from your query you'd use on of the mysql_fetch_(row, array, assoc or object) functions. Before using either of the above functions you should make sure your query returned any results. You can do this using mysql_num_rows
-
Your code should work fine as it is then. Are you getting any errors? Whats happening now/supposed to do
-
Before using $_SESSION vars make sure you call session_start. Is it in header.php?
-
No files that are included/required can have any file extension. Only the parent file needs to be have a .php extension (or in your case .htm) Example test.php <?php include 'non-php-file.txt'; ?> non-php-file.txt <?php echo 'Hello, Word'; ?> When you run test.php the message "Hello, World" will be displayed
-
You're not selecting a database after your have connected to the server. This how your code should be:
-
In order for that line to work your actuall files will need to be named as filename.htm not as filename.php. As that line now parse's .htm files as .php files.
-
EDIT: Beaten to itc By ACP I presume you mean Admin Control Panel? For the ACP you're be basically doing the exacpt same thing as you'e doing in article.php. Except this time you're allowing for the article to be editted. All you need to do is come up with a simple form with two fields and a submit button. The first field will be for the article name. The secoud field will be a <textarea></textarea> where the actuall article can be editted (FCKEDitor replaces this with a graphical WYSIWYG editor) The last will be submit button. When the form is submitted you'd write the new contents to the .txt file. Baisc example if(isset($_POST['submit'])) { $sArticleDirectory = './articles/'; $sArticleName = $sArticleDirectory . str_replace(' ', '_', $_POST['article_name']) . '.txt'; $sArticleContent = $_POST['article_content']; file_put_contents($sArticleName, $sArticleContent); }
-
Upgraded, now phpinfo shows wrong version number
wildteen88 replied to cajun67's topic in PHP Installation and Configuration
Try doing Ctrl + F5, its most probably your browser caching the page. -
You should be using " and not ” on the last few lines of your script Also nothing will be written to menu.txt as you're using echo within your function which will cause it to not return anything. Instead of doing echo 'whatever'; you should do $output .= 'whatever'; Then at the last line of your function place the following line return $output; Now your function will return something which will get written to your file.
-
I see. yea that should do it. However you may want to allow for periods in case a filename gets passed maybe
-
If sending everything to index.php then use (.*) as the pattern. But yea you're on the right lines
-
Its most probably some form of character encoding issue. Where on "page 2" is this code used? Need more info than just your query
-
[SOLVED] include .php-file with includes (array not working)
wildteen88 replied to willdk's topic in PHP Coding Help
Why is your PHP code within an array in the first place? -
[SOLVED] include .php-file with includes (array not working)
wildteen88 replied to willdk's topic in PHP Coding Help
Your code makes no sense. Why you putting PHP code within an array? PHP is not executed from within strings. You need to use eval in order for code within strings to execute. Also as $arrPHP is an array, just echo'ing it on its own will result in Array being displayed, -
[SOLVED] loading images in back order (from Z to A)
wildteen88 replied to zolder's topic in PHP Coding Help
Yes that'll work. However glob can still be used define('IMAGE_DIR', 'test'); define('THUMB_DIR', 'test2'); $images = glob(IMAGE_DIR."/*.{png,jpg,bmp}", GLOB_BRACE); rsort($images); foreach($images as $image) { $thumb = str_replace(IMAGE_DIR, THUMB_DIR, $image); echo '<img src="'.$image.'" /><br />'; echo '<img src="'.$thumb.'" />'; } -
[SOLVED] loading images in back order (from Z to A)
wildteen88 replied to zolder's topic in PHP Coding Help
Cleaner/easier to use glob $images = glob("test/*.jpg"); rsort($images); foreach($images as $image) { echo '<img src="'.$image.'" />'; } -
Yes it should do. However why didnt you use C++ for this, if thats all you're doing.
-
yes the script has a flaw in it. its because this line: $last_num = substr($contents, -1); Only grabs the last character from the file. So when 10 gets written to the file, The next time you run the script, 0 will be the last character and this is why the counter restarts again. A better way would be <?php $filename = 'test.txt'; $num = file_get_contents($filename); $num += 1; if (is_writable($filename)) { // finally write new contents to test.txt file_put_contents($filename, $num); echo "Success, wrote ($num) to file ($filename)"; } else { echo "The file $filename is not writable"; } ?>
-
Yea, I know that. But what do you want the install script to do. If you cant tell us what you are wanting to achieve we wont be able to help
-
variables do not hold their value once a script has finished being parsed. So everytime your script is run all this line is doing $somecontent +=1; is setting $somecontent to the value of 1. After that you're appending this value to the contents of test.txt. This is why you get a series of 1's not 123456 etc. What you'll want to do is this: <?php $filename = 'test.txt'; // Let's make sure the file exists and is writable first. if (is_writable($filename)) { // In our example we're opening $filename in append mode. // The file pointer is at the bottom of the file hence // that's where $somecontent will go when we fwrite() it. if (!$handle = fopen($filename, 'a+')) { echo "Cannot open file ($filename)"; exit; } // read contents of the file $contents = fread($handle, filesize($filename)+1); // get the last number $last_num = substr($contents, -1); // increment last number by 1 $last_num += 1; // Write $last_num to our opened file. if (fwrite($handle, $last_num) === FALSE) { echo "Cannot write to file ($filename)"; exit; } echo "Success, wrote ($last_num) to file ($filename)"; fclose($handle); } else { echo "The file $filename is not writable"; } ?>
-
What do you mean by If you're getting errors post them here in full
-
Add the following at the top of you script: echo 'POST Data: <pre>' . print_r($_POST, true) . '</pre>'; exit; Whats the output