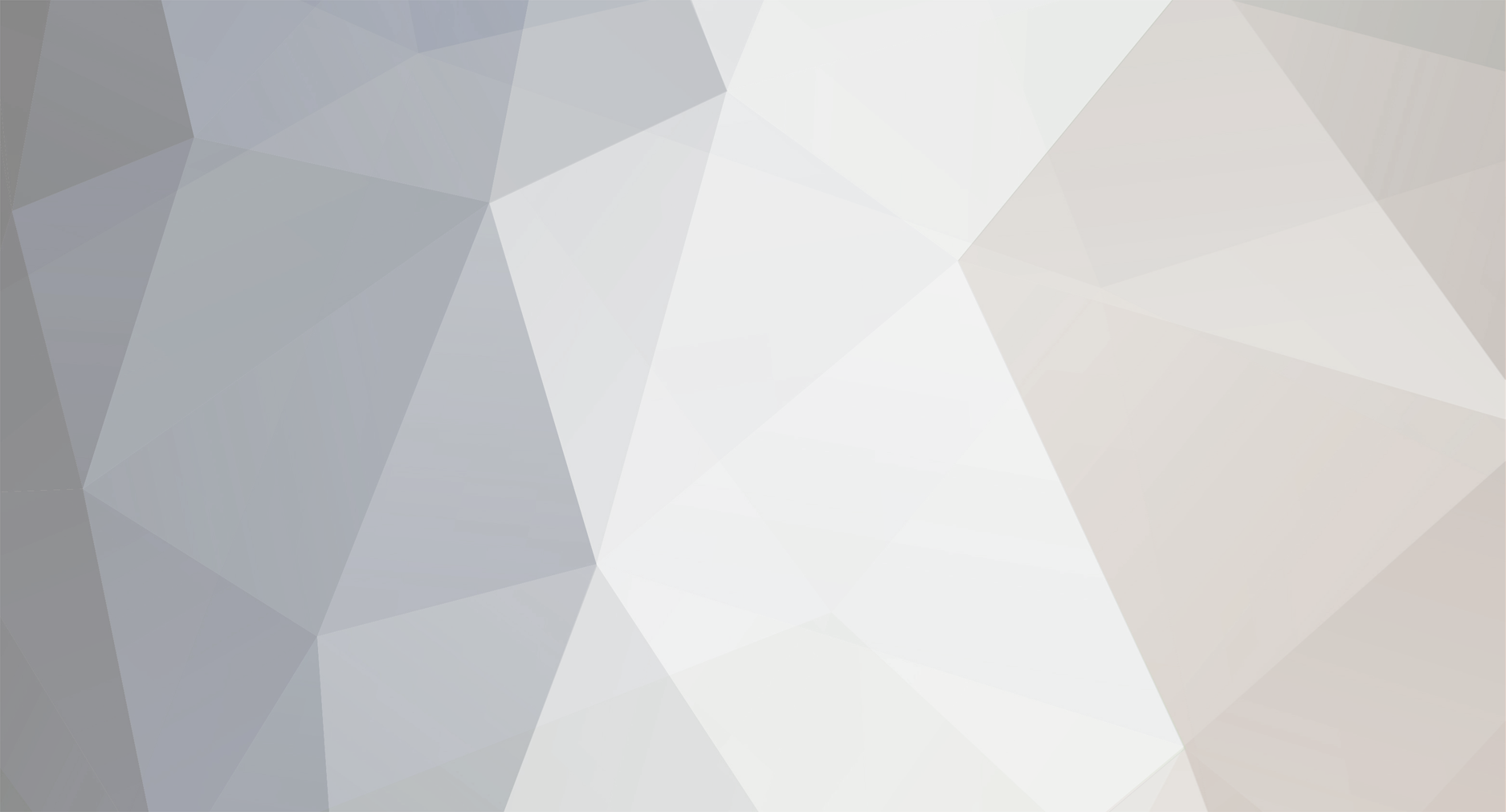
phporcaffeine
Members-
Posts
361 -
Joined
-
Last visited
Never
Everything posted by phporcaffeine
-
I think you may be making it more complicated than it need be ... <?php $input = $_POST['input']; $split = explode("\n", $input); foreach ($split as $key => $value) { //DO ACTION ON THE INDIVIDUAL SPLIT INDEX echo $value . "<br />"; } ?>
-
Well in javascript you have the window.open and window/parent.location methods that may be of some help. window.open however will generally run you into a pop-up blocker issue.
-
Are you serious?
-
Here is a function that you can call to make POST requests against resource locators (should be all you need). function makePost($url, $referer, $_data) { $data = array(); while(list($n,$v) = each($_data)){ $data[] = "$n=$v"; } $data = implode('&', $data); $url = parse_url($url); if ($url['scheme'] != 'http') { die('Only HTTP request are supported !'); } $host = $url['host']; $path = $url['path']; //CREATE A SOCKET $fp = fsockopen($host, 80); //HEADERS fputs($fp, "POST $path HTTP/1.1\r\n"); fputs($fp, "Host: $host\r\n"); fputs($fp, "Referer: $referer\r\n"); fputs($fp, "Content-type: application/x-www-form-urlencoded\r\n"); fputs($fp, "Content-length: ". strlen($data) ."\r\n"); fputs($fp, "Connection: close\r\n\r\n"); fputs($fp, $data); $result = ''; while(!feof($fp)) { $result .= fgets($fp, 128); } fclose($fp); $result = explode("\r\n\r\n", $result, 2); $header = isset($result[0]) ? $result[0] : ''; $content = isset($result[1]) ? $result[1] : ''; return array($header, $content); } $data = array( 'field1' => 'value1', 'field2' => 'value2', 'field3' => 'value3' ); list($header, $content) = makePost("http://www.example.com/", "http://www.referersite.com/", $data); print $content;
-
I don't think cURL is where you need to be looking. You need to make a POST request to the website. Example: function makePost($url, $referer, $_data) { $data = array(); while(list($n,$v) = each($_data)){ $data[] = "$n=$v"; } $data = implode('&', $data); $url = parse_url($url); if ($url['scheme'] != 'http') { die('Only HTTP request are supported !'); } $host = $url['host']; $path = $url['path']; //CREATE A SOCKET $fp = fsockopen($host, 80); //HEADERS fputs($fp, "POST $path HTTP/1.1\r\n"); fputs($fp, "Host: $host\r\n"); fputs($fp, "Referer: $referer\r\n"); fputs($fp, "Content-type: application/x-www-form-urlencoded\r\n"); fputs($fp, "Content-length: ". strlen($data) ."\r\n"); fputs($fp, "Connection: close\r\n\r\n"); fputs($fp, $data); $result = ''; while(!feof($fp)) { $result .= fgets($fp, 128); } fclose($fp); $result = explode("\r\n\r\n", $result, 2); $header = isset($result[0]) ? $result[0] : ''; $content = isset($result[1]) ? $result[1] : ''; return array($header, $content); } $data = array( 'field1' => 'value1', 'field2' => 'value2', 'field3' => 'value3' ); list($header, $content) = makePost("http://www.example.com/", "http://www.referersite.com/", $data); print $content;
-
Try this: foreach ($array as $key => $value) { if ($key == 'name') { echo "$key: $value<br />"; } } It may be different depending on the scope of your array however. If the above doesn't do it then add var_dump($key); and var_dump($value); inside the foreach statement to find out how your array is structured.
-
That is true. Often times 3rd party hosts will whack the /tmp folder randomly for security purposes. That seems likely since you can't see a reliable pattern. Other than that, I would need to see more of your code.
-
I think one of those 'all-in-one' projects might work for you like a one of those WAMP installers ... http://www.wampserver.com/en/ It's Apache, PHP and MySQL all in one and you can have it up and running in minutes. They are great if your not sure how to configure everything to work together but I do not recommend using an all-in-one installer for production environments.
-
Okay. Yes there have been projects that have taken the interpreted language we know as PHP and ported the syntax to a program that can generate php code. However, if you visit http://php.net (the official site for php), no where will you find a 'compiler'. So since the official maintainers of the language do not offer it as a 'compiled' language then in my opinion it is not a 'compiled' language. Lets also take note the differences between a 'compiled language' and a 'language compiler' (of which PHP is neither). A language compiler is often a third party application that auto generates a particular language's syntax to speed up the code writing process. A language compiler can also be an application that takes readable syntax and reduces it to assembly language. A compiled language is often a language who's run-time syntax is in binary form, C#, C++ .... etc. PHP (as it is offered by the official project maintainers) is an interpreted language. Meaning that PHP is a set of files that has the ability to parse other files that use a particular set of syntax (scripts). The inherit sign of an interpreted language is that the syntax of the language cannot be executed alone, it requires an 'interpreter' to translate the readable syntax into assembly/machine language for the computer to understand it. There is an official port of the PHP core called PHP CLI (command line interface) that allows PHP syntax execution outside of the dependencies of a typical execution environment (a web server like Apache). Even so, it is still interpreted because the CLI core is interpreting the syntax into assembly or machine language. Oh, but wait Ryan ... What about the Zend Encoder ... that makes PHP compiled right? Wrong. The Zend encoder does encrypt and encode the syntax however it does not 'compile' the syntax into assembly or machine language. Why? Because you still need the Zend Encoder on the server in order to interpret the 'encoded' scripts. The Zend Encoder is more for code security and optimization ... it isn't a 'compiler'. In closing, even though there may be programs that 'auto -generate' PHP syntax or encode PHP syntax PHP is and always has been an interpreted language.
-
[SOLVED] Having problem with IMAGE UPLOAD (hack)
phporcaffeine replied to andz's topic in PHP Coding Help
The only other thing I can think of is to RegEx the PE Header inside the content of the upload. -
I'm not sure I understand the question but I'll answer from every angle I can think of. phpinfo(); is a helpful (and dangerous) read-only core method within the PHP language. The displayed results will give very detailed info about the configured php runtime environment on your server and all the things available. register_globals() is a function that you can call in your scripts to tell php to make a variable available to all parts of a script regardless of scoping. It is mostly discouraged from use not because it is a faulty function but because of a general lack in understanding of the function and how to use it responsibly (as it can be a very dangerous function when used in a vulnerable script or incorrectly).
-
Also, make sure that in every instance where you are using unset that you are also using the appropriate/desired array index. For example, if I accidentally used unset($_SESSION) ... I would wipe out all sessions for that user, regardless of the array indexes.
-
[SOLVED] Having problem with IMAGE UPLOAD (hack)
phporcaffeine replied to andz's topic in PHP Coding Help
You could also break out a RegEx manual and then file_get_contents() the upload a do a regex search for the PE header. That is a more definitive solution but just verifying the mime type should do the trick. -
Drowzor, You shouldn't have too many compatibility issues (if any) between the minor releases. The most major compatibility issue that PHP saw in recent times was the split from the 4 branch and into the 5 branch. Overall though I don't think there was too much fuss in the community. We are due to repeat though as PHP6 is on the horizons.
-
Drowzor, I also produce a podcast series dedicated to the teaching and the proliferation of PHP. It may be of some help to you. You can find it in iTunes by searching for, "The CodeTree" or "Ryan Huff". Additionally the RSS link is: http://codetree.rthconsultants.com/feed/podcast/?format=codetree
-
Absolutely not .... by sending text you are in effect, sending a header to the browser. Sending subsequent headers is not possible. Some alternatives maybe to use some creativity with iFrames.
-
echo $_SERVER['PHP_SELF'] .... (assuming an Apache server)
-
[SOLVED] If File Doesn't Exist Statement
phporcaffeine replied to mcmuney's topic in PHP Coding Help
$path = "photos/".$date."/".$id.".jpg"; @unlink($path); $sql2 = mysql_query("DELETE FROM images WHERE id = '$id'"); In the above example regardless if the file was present for unlink to delete, the query statement is ran. The '@' symbol simply squelches any error messages that you would get when unlink tried to remove a file that wasn't there. Not the most elegant, but the quickest. -
[SOLVED] Having problem with IMAGE UPLOAD (hack)
phporcaffeine replied to andz's topic in PHP Coding Help
So you are trying to prevent users from uploading something to your server? A file upload? Can you be a little more specific? -
Firstly, you won't find anything in the realm of a 'php compiler' ... php is an interpreted (non-compiled) language. There are many available resources on the internet for learning PHP. The best way to learn in my opinion is to start with some simple php scripts (the ole 'hello world' standby is a good one) and then reverse engineer those scripts to figure out how they work.
-
Where one issue may be in your code is references to: $_SESSION[mailorder] ... it should be $_SESSION['mailorder'] (notice the use of the single quote). Other than that it should work fine.
-
Your right, it isn't needed but I do it to adhere to coding standards in the project that I am working with.
-
Here is what I am trying to do: Script creates an .xls file on the fly, forces a download of that file and then redirects the browser window to another page. I have a working example below. The example will create and force a download to the browser but I just can't figure out how to get it to redirect after the download? I know that since I am using the headers to force the download that is what is causing my issue so if someone could show me how to still be able to redirect after the download or maybe a different way to do the download it would be greatly appreciated! <?php function xlsBOF() { echo pack("ssssss", 0x809, 0x8, 0x0, 0x10, 0x0, 0x0); return; } function xlsEOF() { echo pack("ss", 0x0A, 0x00); return; } function xlsWriteLabel($Row, $Col, $Value ) { $L = strlen($Value); echo pack("ssssss", 0x204, 8 + $L, $Row, $Col, 0x0, $L); echo $Value; return; } function excelHeader($filename) { header("Pragma: public"); header("Expires: 0"); header("Cache-Control: must-revalidate, post-check=0, pre-check=0"); header("Content-Type: application/force-download"); header("Content-Type: application/octet-stream"); header("Content-Type: application/download");; header("Content-Disposition: attachment;filename=$filename"); header("Content-Transfer-Encoding: binary "); return; } function createOutputFile($extractRows) { excelHeader("contacts.xls"); xlsBOF(); $row=1; foreach ($extractRows as $value) { xlsWriteLabel ($row,0,$value); $row++; } xlsEOF(); } if (isset($_POST['name'])) { $extractRows=array(); $extractRows[]="Some example data 1"; $extractRows[]="Some example data 2"; $extractRows[]="Some example data 3"; $extractRows[]="Some example data 4"; createOutputFile($extractRows); } else { print "<form method=\"post\" id=\"customerdetails\" name=\"calendar_form\" id=\"datesForm\" action=\"$formActionURL\">\n"; print "Extract your contact"; print "<input type=\"text\" id=\"name\" name=\"name\">"; print "<input type=\"submit\" id=\"submitbutton\" value=\"Extract contacts\">"; print "</form>"; } ?>
-
Here is the code: <?php class xmlreaders { var $_data; var $_white; var $_xml_url; function xmlreaders ($xml_url = "") { $this->_white = 1; if (trim($xml_url) != "") $this->set_xml_url ($xml_url); } function set_xml_url ($url) { $this->_xml_url = $url; } function read () { if (!$this->_xml_url) $this->error ("XML File is not assigned."); $fp = fopen ($this->_xml_url, "r"); while (!feof ($fp)) $this->_data .= fgets($fp, 4096); fclose ($fp); $this->_data = trim ($this->_data); } function parse () { $this->read(); if (trim ($this->_data) == "") $this->error ("Data not ready."); $vals = $index = $array = array(); $parser = xml_parser_create(); xml_parser_set_option($parser, XML_OPTION_CASE_FOLDING, 0); xml_parser_set_option($parser, XML_OPTION_SKIP_WHITE, $this->_white); xml_parse_into_struct($parser, $this->_data, $vals, $index); xml_parser_free($parser); $i = 0; $tagname = $vals[$i]['tag']; if ( isset ($vals[$i]['attributes'])) { $array[$tagname]['@'] = $vals[$i]['attributes']; } else { $array[$tagname]['@'] = array(); } $array[$tagname]["#"] = $this->xml_depth($vals, $i); return $array; } function xml_depth($vals, &$i) { $children = array(); if ( isset($vals[$i]['value'])) { array_push($children, $vals[$i]['value']); } while (++$i < count($vals)) { switch ($vals[$i]['type']) { case 'open': if ( isset ( $vals[$i]['tag'])) { $tagname = $vals[$i]['tag']; } else { $tagname = ''; } if ( isset ( $children[$tagname])) { $size = sizeof($children[$tagname]); } else { $size = 0; } if ( isset ( $vals[$i]['attributes'])) { $children[$tagname][$size]['@'] = $vals[$i]["attributes"]; } $children[$tagname][$size]['#'] = $this->xml_depth($vals, $i); break; case 'cdata': array_push($children, $vals[$i]['value']); break; case 'complete': $tagname = $vals[$i]['tag']; if( isset ($children[$tagname])) { $size = sizeof($children[$tagname]); } else { $size = 0; } if( isset ( $vals[$i]['value'])) { $children[$tagname][$size]["#"] = $vals[$i]['value']; } else { $children[$tagname][$size]["#"] = ''; } if ( isset ($vals[$i]['attributes'])) { $children[$tagname][$size]['@'] = $vals[$i]['attributes']; } break; case 'close': return $children; break; } } return $children; } function traverse_xmlize($array, $arrName = "array", $level = 0) { foreach($array as $key=>$val) { if ( is_array($val)) { traverse_xmlize($val, $arrName . "[" . $key . "]", $level + 1); } else { $GLOBALS['traverse_array'][] = '$' . $arrName . '[' . $key . '] = "' . $val . "\"\n"; } } return 1; } function error ($str) { print get_class ($this)." ".$this->version()." => $str"; exit(); } function version () { return "1.0"; } } $xml = new XMLReader(); $xmlurl = "stocksvol.xml"; $xmlreaders = new xmlreaders ($xmlurl); $xml = $xmlreaders->parse(); $new = array(); foreach ($xml['TTI_transmission']['#']['q'] as $value) { //FORMULA = $value['#']['s'][0]['#'] (WHERE 'S' IS THE NODE THAT YOU ARE TRYING TO GET THE VALUE OF $net = $value['#']['l'][0]['#'] - $value['#']['o'][0]['#']; echo $value['#']['l'][0]['#'] . "-" . $value['#']['o'][0]['#'] . "=" . $net . "<br />"; $num = floatval($net); $new[] = array($num => "<net>$net</net><q><s>" . $value['#']['s'][0]['#'] . "</s><l>" . $value['#']['l'][0]['#'] . "</l><c>" . $value['#']['c'][0]['#'] . "</c><p>" . $value['#']['p'][0]['#'] . "</p><h>" . $value['#']['h'][0]['#'] . "</h><o>" . $value['#']['o'][0]['#'] . "</o><h>" . $value['#']['h'][0]['#'] . "</h><t>" . $value['#']['t'][0]['#'] . "</t><d>" . $value['#']['d'][0]['#'] . "</d><t>" . $value['#']['t'][0]['#'] . "</t></q>"); } $date =$xml['TTI_transmission']['#']['datestamp'][0]['#']; unset($xml); unset($xmlreaders); sort($new); $cnt =0; $export = " <?xml version=\"1.0\" ?> <!DOCTYPE TTI_transmission [ <!ELEMENT TTI_transmission (q*,datestamp)> <!ELEMENT datestamp (#PCDATA)> <!ELEMENT q (s?,l?,c?,p?,v?,o?,h?,w?,d?,t?)> <!ELEMENT s (#PCDATA)> <!ELEMENT l (#PCDATA)> <!ELEMENT c (#PCDATA)> <!ELEMENT p (#PCDATA)> <!ELEMENT v (#PCDATA)> <!ELEMENT o (#PCDATA)> <!ELEMENT h (#PCDATA)> <!ELEMENT w (#PCDATA)> <!ELEMENT d (#PCDATA)> <!ELEMENT t (#PCDATA)> ]> <TTI_transmission> "; foreach ($new as $key => $value) { foreach ($value as $val) { $cnt++; if ($cnt >= 100) { break; } $export .= $val . "\n"; } } $export .= "<datestamp>" . $date . "</datestamp> </TTI_transmission>"; fwrite(fopen('export.xml', 'w+'), $export); ?>