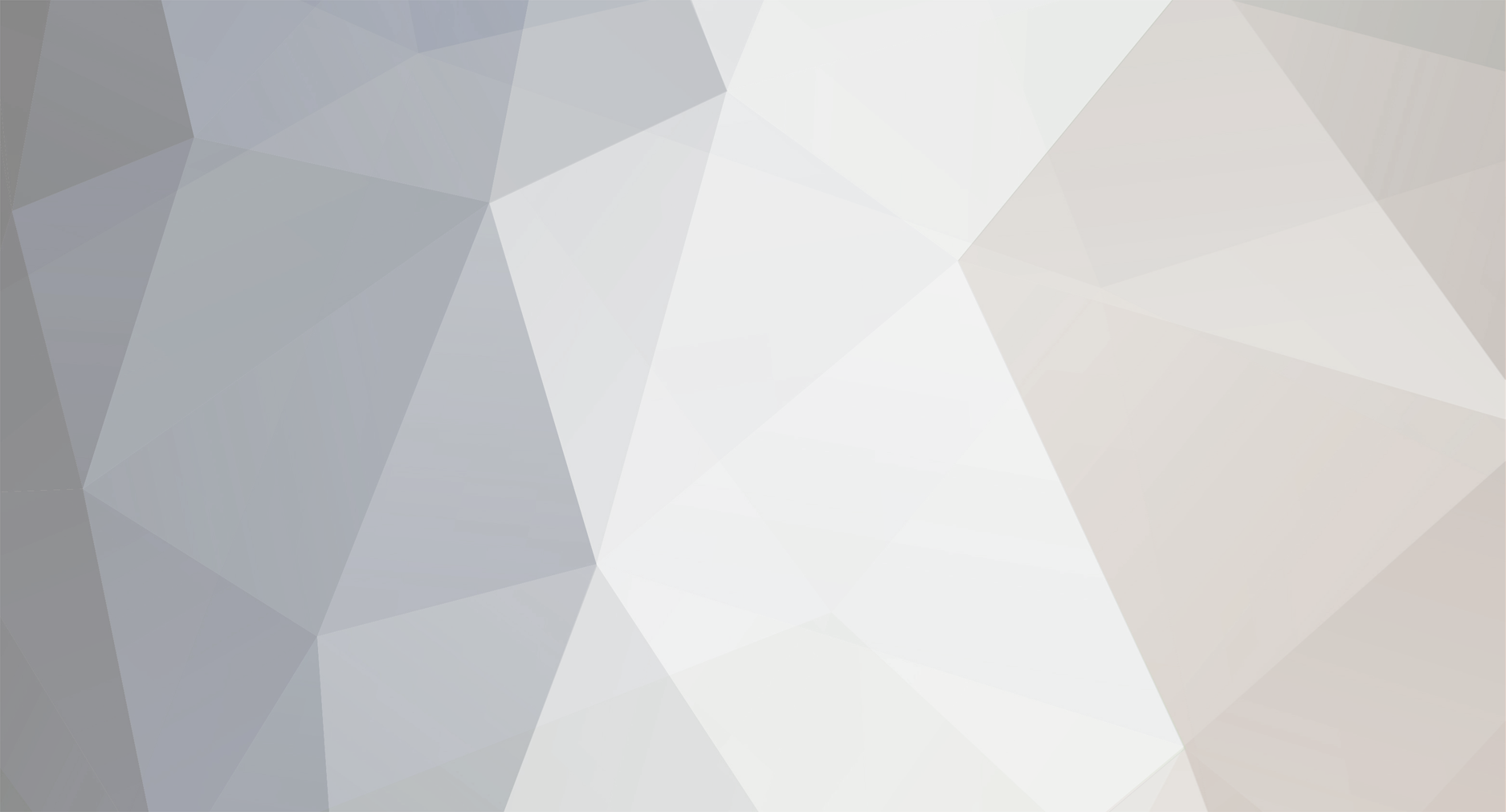
Alex_
Members-
Posts
37 -
Joined
-
Last visited
Everything posted by Alex_
-
The absolute first thing you should do is create a template that you're satisfied with, as in HTML CSS and Javascript. Backend code (such as PHP) is kind of a time wasting thing to do without a template for a project like you're explaining. At least that's how I usually think about it. Once I have a template ready, I can browse the template myself and just see what I want to build next, and know how to do it. In general, forums aren't "too" hard to create either, depending on how far you want to go with it. The further you go, the more difficult it gets, of course. Things like Plugins for instance can be a challenge at times. I'd start of with a template, and the functionality of making Threads/Posts in different sections of the forum. That's a good start.
-
By frontend and backend I do mean client / server. For me, Entities Mappers and Services are a given in an MVC application, but obviously it's just a preference for each developer. I prefer it for the ease, and organizied code. Let me give you an example Controller public function doUpdate() { $data = $this->request->putJSON(); $myService = new MyService(); $updatedUser = $myService->updateUser($data); return static::ok($updatedUser); } Service public function updateUser($data) { $User = new User($data); //Entity $User->convertOriginalFormat(); //Just an example function on an Entity, that can do what you wanted to do. return $this->_myMapper->update($User); } Mapper public function update(User $user) { //Only accept a User entity $params = $user->toValidatedArray(); $query = implode(' ', array($this->update, $this->keySearch, $this->returning)); return $this->db->query($query, $params); } So i keep logic in the service. Most often an Entity is able to hold "helper" functions, such as formatting and stuff as well. The Entity can be thought of as the Model in the frontend. Contains data with getters and setters, and helper functions. The mapper can then use an Entity for queryBuilding and creates a proper update Query. I wouldn't advice using this MVC pattern on an existing application because it may be a lot to rebuild, but for a future project, I'd say it's worth giving it a try.
-
I'm confused what you mean by "comparing" two variables since that's what you did yourself in the main post. Also you should use tripple '=' operator, not double, if you want to be sure the two values are actually the same, because then it makes sure the type of the variable is the same as well. For instance a string of "1" and an integer of 1 would be "the same" when using double '=' operator. Example: var first = "1"; var second = 1; console.log(first == second); //true console.log(first === second); //false, different types. But could you please elaborate more what you mean by "comparing" two variables ? Because like I said, it looks like you did it yourself in the main post.
-
Assuming the PHP Script is run on the same page you're loading, and above the actual <script> import tag, you could make it easy on yourself. A lot of applications use their own global object to keep some form of data that is fairly unionized throughout more than one page. A way you can do this is something like this: <script> MyApplication = { meta: {} }; //Global object MyApplication. For this example, with 'meta' MyApplication.meta.MyVariable = '<?php echo $timezone_offset;?>'; </script> <script src="path/myJsFile.js"></script> And then in your external JS file you do var myVariable = MyApplication.meta.MyVariable; And you have access to it. http://i.imgur.com/VKUBfLm.png You can also do this if you have an array of items you want accessible in the frontend, by doing something like this.. <script> MyApplication = { meta: {} }; <?php foreach($myList as $key => $value) { echo "MyApplication.meta." . $key . " = '".$value."';"; } ?> </script> Hope it helps.
-
What 'tryingtolearn' said is true, should be done in the backend. The reason I wanted to make a post is to present the way my MVC application is built, perhaps it sounds like a lot more work, but it's a lot more organized and easier to know where things are done, and why. Frontend View: Self-explanatory, but associates with Model/Collection, handles what the user can & should see. Model: Contain data, validation, helperfunctions (Like format a value to money format) Collection: Same as model, but several. Backend - Controller: Receive requests, (Only logic here is extracting request data & returning correct response, IE 200) - Service(s): Handle Logic -- This is where I would, in my application, convert the values back that you mentioned - Entitiy(ies): Used to handle each model as an object. Getters/setters/validations. Also used for queryBuilding (Insert, update, delete, etc) - Mapper(s): Handle database operations. (With potential entities) Maybe a useless post, but perhaps the design could give you some form of idea. A Controller in my personal view should do nothing but actually handle the request, no actual datahandling or logic in that sense. For me, that's the service & entities job.
-
Passing Parameters as Variables vs Passing Parameters as An Array
Alex_ replied to NotionCommotion's topic in PHP Coding Help
It's a preference, I guess. Most people I know prefer using objects rather than a large quantity of parameters. Although when opting for using an Array as the parameter, you should probably change it out to function myNewFunction(Array $data = array()) { So that any other type of value is not accepted by the function. Right now you could call myNewFunction('Test'); and it will attempt to merge an array with a string. Since you asked for personal preferences as well, I'll mention that I'd always opt for the object parameter option, simply because it's easier, far more readable, and like you just demonstrated yourself it's the perfect parameter when used for things like Configurations/Options (Database, for instance). It's especially nice when using getters/setters in a class as well. For instance: class MyClass { private $_defaults = array(); //Default values public function __construct() { $this->setData($this->_defaults); } public function myNewFunction(Array $data = array()) { $data = array_merge($this->_defaults, $data); $this->setData($data); } public function setData(Array $data = array()) { foreach($data as $key => $value) { $this->set($key, $value); } } public function set($key, $value) { $this->$key = $value; return $this; } public function get($key) { return $this->$key } } I know that wasn't part of your question, but it's a related example. -
The .closest() function only looks for parents, not "any nearest" element in the DOM, so to speak. One alternative is to do something like this since the container seems pretty static.. $(document).on('click', '#select', function() { var mainContainer = $(this).parents()[1]; //<div class="names dropdown"> -- Index 1, since static. var inputField = $(mainContainer).find('input')[0]; //Index 0, because it's right below the <div> tag. console.log(inputField); }); Obviously it isn't great practise tho, if you had more dynamic content or happened to add/change a field you'd have to change the indexes as well. But assuming that's a pretty static layout (aside from the dynamic <li> tags), it should work just fine.
-
Haven't used jquery.validate myself but from your snippet I'm assuming jquery.validate is expecting a simple bool return value, so you should be able to just match your regularexpressions in the validIPurl function. Something like jQuery.validator.addMethod('validIPurl', function(value) { var ip = ^([01]?[0-9]{1,2}|2[0-4][0-9]|25[0-5]).([01]?[0-9]{1,2}|2[0-4][0-9]|25[0-5]).([01]?[0-9]{1,2}|2[0-4][0-9]|25[0-5]).([01]?[0-9]{1,2}|2[0-4][0-9]|25[0-5]); var URL = /^(http|https)?:\/\/[a-zA-Z0-9-\.]+\.[a-z]{2,4}/; var ipMatcher = new RegExp(ip); var urlMatcher = new RegExp(url); return ipMatcher.test(value) || urlMatcher.test(value); } }, 'Invalid Address'); Assuming I understood what you meant. It will accept either a valid IP, or a valid URL.
- 1 reply
-
- jquery
- javascripts
-
(and 1 more)
Tagged with:
-
if page contains an exact image src then redirect to another page
Alex_ replied to toolman's topic in Javascript Help
That seems overly complicated Xaotique, considering he has an ID on the <span> tag it's kind of a waste to loop over all images on the page. Try something like this: //HTML <span id="bannerhold"><img src="http://www.website.com/images/banners/banner1.gif"></span> //JS $(document).ready(function() { var pageContainsImage = $('span#bannerhold > img[src="http://www.website.com/images/banners/banner1.gif"]').length > 0; if(pageContainsImage) { window.location.href = 'http://google.com'; } }); -
Oh yeah I forgot to mention, sorry.. The data comes from two different databases. Basically one Database is the User's own database (Well, postgres schema), while the second Database is a union DB for all Users, where sensitive data is held. So unfortunately union statements aren't an option either, considering it's two different connections.
-
Hey. So imagine this example... class FormulaEvaluator { ...... //Construct & variables here.. public function exec() { //Missing some lines here, but you get the idea $formula = $this->formula; $context = (array) $this; ob_start(); $res = eval('return ' . $formula . ';'); } } $formulaEvaluator = new FormulaEvaluator(); $formula = '10 * (($Test * 2) + ($Test2 / 3))'; $formulaEvaluator->formula = $formula; $formulaEvaluator->Test = 20000; $formulaEvaluator->Test2 = 300000; $res = $formulaEvaluator->exec(); echo $res; //1400000 The code we have is working as intended, but we want to avoid using eval. I know it's a pretty common question, and the answer is usually the same, but is there any way to do something similar without using eval?
-
Hey. So the issue I'm having is consecutive loops on semi-large arrays, over and over. Consider this array: $firstArray = array( 'row1' => array( 'dates' => array( '2014-01-01' => array('key1' => 'value1', 'key2' => 'value2', 'key3' => 'value3', 'key4' => 'value4', 'key5' => 'value5', 'key6' => 'value6', 'key7' => 'value7', 'key8' => 'value8', 'key9' => 'value9', 'key10' => 'value10'), '2014-01-02' => array('key1' => 'value1', 'key2' => 'value2', 'key3' => 'value3', 'key4' => 'value4', 'key5' => 'value5', 'key6' => 'value6', 'key7' => 'value7', 'key8' => 'value8', 'key9' => 'value9', 'key10' => 'value10'), '2014-01-03' => array('key1' => 'value1', 'key2' => 'value2', 'key3' => 'value3', 'key4' => 'value4', 'key5' => 'value5', 'key6' => 'value6', 'key7' => 'value7', 'key8' => 'value8', 'key9' => 'value9', 'key10' => 'value10'), '2014-01-04' => array('key1' => 'value1', 'key2' => 'value2', 'key3' => 'value3', 'key4' => 'value4', 'key5' => 'value5', 'key6' => 'value6', 'key7' => 'value7', 'key8' => 'value8', 'key9' => 'value9', 'key10' => 'value10'), '2014-01-05' => array('key1' => 'value1', 'key2' => 'value2', 'key3' => 'value3', 'key4' => 'value4', 'key5' => 'value5', 'key6' => 'value6', 'key7' => 'value7', 'key8' => 'value8', 'key9' => 'value9', 'key10' => 'value10'), '2014-01-06' => array('key1' => 'value1', 'key2' => 'value2', 'key3' => 'value3', 'key4' => 'value4', 'key5' => 'value5', 'key6' => 'value6', 'key7' => 'value7', 'key8' => 'value8', 'key9' => 'value9', 'key10' => 'value10'), '2014-01-07' => array('key1' => 'value1', 'key2' => 'value2', 'key3' => 'value3', 'key4' => 'value4', 'key5' => 'value5', 'key6' => 'value6', 'key7' => 'value7', 'key8' => 'value8', 'key9' => 'value9', 'key10' => 'value10'), ) ), 'row2' => array( 'dates' => array( '2014-02-01' => array('key1' => 'value1', 'key2' => 'value2', 'key3' => 'value3', 'key4' => 'value4', 'key5' => 'value5', 'key6' => 'value6', 'key7' => 'value7', 'key8' => 'value8', 'key9' => 'value9', 'key10' => 'value10'), '2014-02-02' => array('key1' => 'value1', 'key2' => 'value2', 'key3' => 'value3', 'key4' => 'value4', 'key5' => 'value5', 'key6' => 'value6', 'key7' => 'value7', 'key8' => 'value8', 'key9' => 'value9', 'key10' => 'value10'), '2014-02-03' => array('key1' => 'value1', 'key2' => 'value2', 'key3' => 'value3', 'key4' => 'value4', 'key5' => 'value5', 'key6' => 'value6', 'key7' => 'value7', 'key8' => 'value8', 'key9' => 'value9', 'key10' => 'value10'), '2014-02-04' => array('key1' => 'value1', 'key2' => 'value2', 'key3' => 'value3', 'key4' => 'value4', 'key5' => 'value5', 'key6' => 'value6', 'key7' => 'value7', 'key8' => 'value8', 'key9' => 'value9', 'key10' => 'value10'), '2014-02-05' => array('key1' => 'value1', 'key2' => 'value2', 'key3' => 'value3', 'key4' => 'value4', 'key5' => 'value5', 'key6' => 'value6', 'key7' => 'value7', 'key8' => 'value8', 'key9' => 'value9', 'key10' => 'value10'), '2014-02-06' => array('key1' => 'value1', 'key2' => 'value2', 'key3' => 'value3', 'key4' => 'value4', 'key5' => 'value5', 'key6' => 'value6', 'key7' => 'value7', 'key8' => 'value8', 'key9' => 'value9', 'key10' => 'value10'), '2014-02-07' => array('key1' => 'value1', 'key2' => 'value2', 'key3' => 'value3', 'key4' => 'value4', 'key5' => 'value5', 'key6' => 'value6', 'key7' => 'value7', 'key8' => 'value8', 'key9' => 'value9', 'key10' => 'value10'), '2014-02-08' => array('key1' => 'value1', 'key2' => 'value2', 'key3' => 'value3', 'key4' => 'value4', 'key5' => 'value5', 'key6' => 'value6', 'key7' => 'value7', 'key8' => 'value8', 'key9' => 'value9', 'key10' => 'value10'), '2014-02-09' => array('key1' => 'value1', 'key2' => 'value2', 'key3' => 'value3', 'key4' => 'value4', 'key5' => 'value5', 'key6' => 'value6', 'key7' => 'value7', 'key8' => 'value8', 'key9' => 'value9', 'key10' => 'value10'), ) ) ); Originally the data comes from ~2-3 database tables, of course. But to ilustrate the point, this is how the main array looks like. This array usually contains anywhere between 10-50 rows, each row containing at least 10 dates, with 10 key/values each. And after setting up all the data, it needs to be processed. Currently this is how a friend of mine did it.. $placeDataHere = array(); foreach($firstArray as $key => $dates) { foreach($dates as $date => $values) { foreach($values as $key => $value) { $placeDataHere['DV_' . $date]['SM_' . $key] = 'KS_' . $value; //Followed by another ~50-70 lines of processing the 3 loop's data.. ... ... .... .... .... .... .... .... } } } Obviously this isn't good practise, but we can't seem to figure out a better way of doing it, since both the data and the loops are horribly nested. This loop and setup of $firstArray is run anywhere between 10-20 times/request, due to amount of users we wish to process. So, the result is that this code can take up to over 2-3 minutes to complete, which isn't really optimal performance. In short my question is, are there any better methods of handling this with the data setup we currently have?