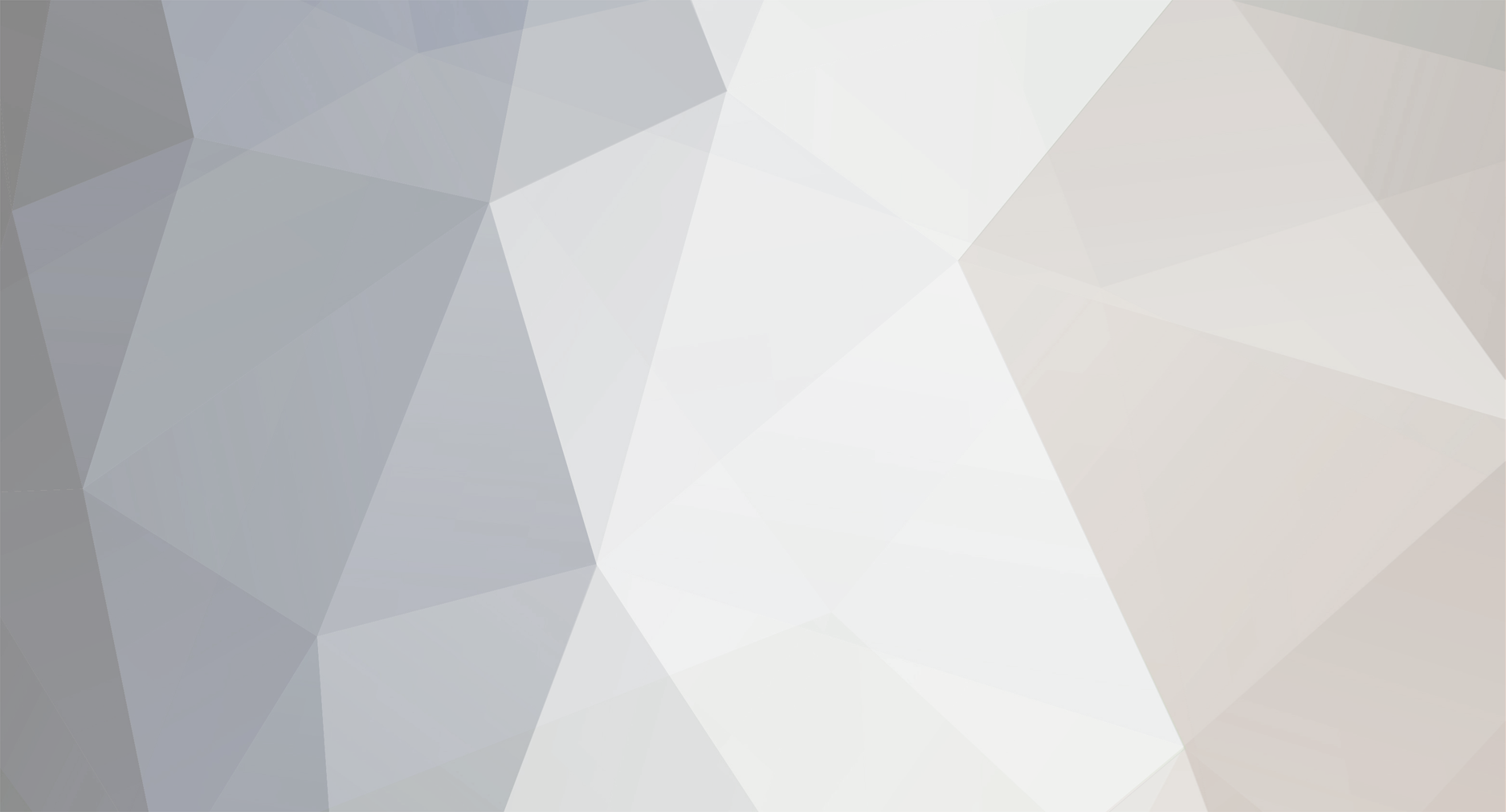
trq
Staff Alumni-
Posts
30,999 -
Joined
-
Last visited
-
Days Won
26
Everything posted by trq
-
Anywhere really. If your going to start building a small library of classes though, you'll want to stick to a common naming convention and make sure each class is in it's own file preferably somewhere on your include_path.
-
using php to sleect value from drop down menu not working
trq replied to runnerjp's topic in PHP Coding Help
Not with the information you have provided, no. -
This topic has been moved to Third Party PHP Scripts. http://www.phpfreaks.com/forums/index.php?topic=358486.0
-
Getting Exception thrown within the exception handler!
trq replied to jayuk20's topic in Third Party Scripts
Not without seeing some relevant code. -
login() is not a static method. You need to create an object in order to use it properly. $auth = new authentication; $login = $auth->login( $_POST['username'], $_POST['password'] );
-
Yeah, there is a lot more to OOP than simply grouping functions into classes. Code libraries and frameworks need these kinds of infrastructure to be able to work together and be extendable. Without them, things get very messy. As you (hopefully) can see, a few simple tricks and tweaks can often make your code a whole lot more robust. The idea above is just one simple design, but it has already made your class compatible with any type of database, without anyone ever having to hack your classes internal code.
-
No it's not wrong. And it's not wrong to create code that relies on it, especially if its just for your own use. I was merely trying to point out that in order to make your code more flexible and useful there are design practices that can make this easy. A database abstraction layer doesn't necessarily meen you need to implement some full blown DBL (like I'm sure you've been reading about). There are very simple methods you can use to make your class more flexible in regards to what databases it can be used within. In your case, you only really use two database functions. One for executing a query, and one that returns the number of results found. If you define an interface that forces these two methods to exist, you can make your code allot more flexible. eg; <?php interface DB { public function query($sql); public function num_rows(); } Now, you can force your class to require an object that implements this interface (note that I also changed the name of your class to something more fitting). <?php class UserLogin { private $db; public function __construct(DB $db) { $this->db = $db; } // rest of your code } Within your class your would now use $this->db->query() instead of mysql_query() and $this->db->num_rows() instead of mysql_num_rows(). Now, all you need is a database object that implements the DB interface. In this case it's simple: <?php class MyDb implements DB { public function query($sql) { return mysql_query($sql); } public function num_rows() { return mysql_num_rows(); } } Then, when you want to use your UserLogin class, just pass it an instance of your MyDb object: <?php $userLogin = new UserLogin(new MyDb); Now, say someone comes along and want's to use your class with an Sqlite database. All they need to is implement the same DB interface (note that this class needs more work, but that is all sqlite specific stuff and is besides the point. It still only needs to implement the query() and num_rows() methods in order to be compatible with your class). <?php class SomeOtherDB implements DB { private $conn; private $result; public function __construct($file) { $this->conn = sqlite_open($file, 0666); } public function query($sql) { $this->result = sqlite_query($this->conn, $sql); return $this->result; } public function num_rows() { return sqlite_num_rows($this->result); } } Now, suddenly your class can use a sqlite database without ever needing to edit it's internals. <?php $userLogin = new UserLogin(new SomeOtherDB('sqlite.db'); This is justa simple example of the advantages of decent design. It's probably riddled with errors, but hopefully it's pretty easy to understand. This is the power of OOP at it's simplest.
-
Well, it needs to be configurable somehow, and hacking the code itself is never advised.
-
Firstly, that won't work. That would be ../home/run.php Learn about file system paths. This really has little to do with php.
-
There are lots of issues with such a design. The first is the fact that you define a bunch of properties up front, but expect a user to edit them before they can use your class. Classes should be designed in such a way that you don't need to edit them before you can use them. You can do this by providing methods to set these properties. The other big issue I have is your classes reliance on the mysql extension. Your class should be using a database abstraction layer of some sort, and this dependency should be (again) set via some *setter* method, or even via the construct. This is called *dependency injection*, Google it. There are a few other issues I have (like having the class be responsible for sending headers) but otherwise, I guess it does the job.
-
I would change that then. PHP6 development has been borked for a long time. It is not to be used.
-
You should probably look into some form of *build* tool. I personally use Phake as it's very simple. There is an example of it's usage in my framework, https://github.com/proem/proem/blob/develop/Phakefile There would be nothing stopping you from creating a task that would upload your code coverage results somewhere. Assuming your using version control as well you could also setup a hook to do the same.
-
You also mention two different files install.php and installer.php, which is it?
-
Whatever. Here is an example without any error handling written for PHP5.4. function findIndex($array, $value) { return array_reverse($array)[$value]; } Should give you the idea without handing you the code.
-
This topic has been moved to JavaScript Help. http://www.phpfreaks.com/forums/index.php?topic=358408.0
-
Seems pretty straight forward, where exactly are you stuck? Sorry, but that statement makes little sense.
-
PHP + MYSQL: best way to handle connections for autosuggest
trq replied to j1982's topic in Applications
Because each request is a separate request it doesn't really matter if you do it yourself or not as php will close the connection for you anyway. -
The idea of this forum is not to help yourself generate traffic to your own site, but to share with the community here. If you think you can help, your welcome to post your solution right here.
-
Take a look at substr.
-
Phpunit can produce a html page that displays your code coverage. All you need do is put that on a public facing server.
-
This topic has been moved to JavaScript Help. http://www.phpfreaks.com/forums/index.php?topic=358359.0
-
This topic has been moved to PHP Coding Help. http://www.phpfreaks.com/forums/index.php?topic=358349.0
-
Sorry, but your post is unreadable.