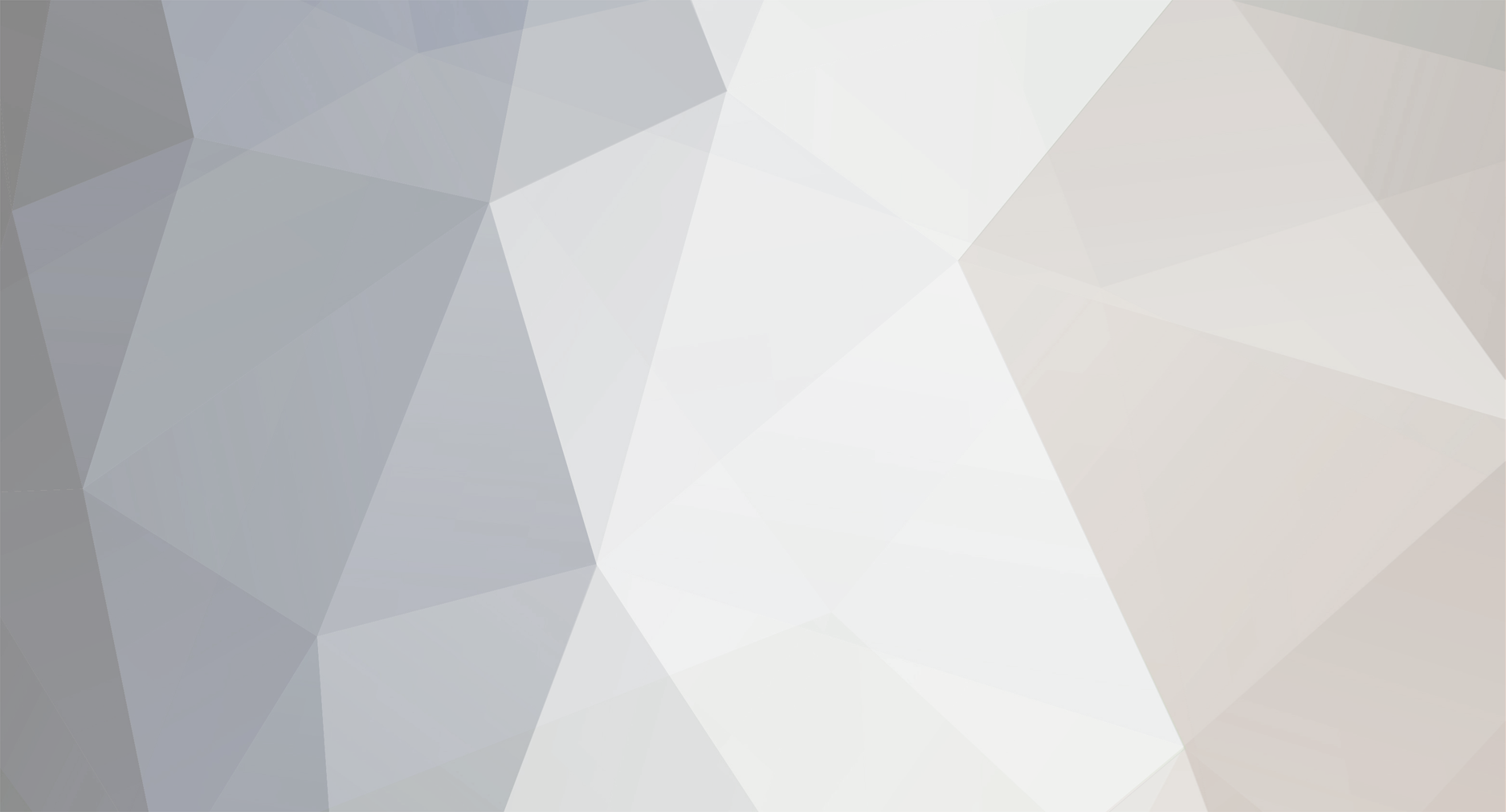
Phi11W
Members-
Posts
163 -
Joined
-
Last visited
-
Days Won
12
Everything posted by Phi11W
-
I might suggest that it could be because you are jumping between HTML and PHP with appalling rapidity. I prefer to write PHP code that builds or outputs strings that just happen to contain HTML. echo '<select name="YYYY_Entry" style="width:60px">'; if($MM == '1' && $DD < 7) printf( '<option value="%1$s"%2$s>%1$s</option>' , $PreviouseYear , ( ( $_SESSION["DE_YYYY_Entry"] == $PreviouseYear ) ? ' selected="selected"' : '' ) ); printf( '<option value="%1$s"%2$s>%1$s</option>' , $ThisYear , ( ( $_SESSION["DE_YYYY_Entry"] == $ThisYear ) ? ' selected="selected"' : '' ) ); if($MM > '10') printf( '<option value="%1$s"%2$s>%1$s</option>' , $NextYear , ( ( $_SESSION["DE_YYYY_Entry"] == $NextYear ) ? ' selected="selected"' : '' ) ); echo( '</select> ' ); Regards, Phill W.
-
The architecture is completely different. VB programs run on the user's computer and so have access to everything connected to the user's computer. PHP applications run on a web server computer somewhere "out there", on The Web. Your PHP application cannot know anything about any printer, much less interact with them [directly] in any way. To "print" things these days, your PHP application sends document content to the user's web browser (running on the user's computer) and the user can choose to print it from there. For example: https://stackoverflow.com/questions/52410546/create-write-and-download-a-txt-file-using-php Regards, Phill W.
-
You've made the closing of the anchor tag conditional on the user being set. It shouldn't be. Assuming you want an anchor element that lacks its href attribute if the user isn't set, then use something like this: printf( '<a class="custom-btn custom-btn--small custom-btn--style-4"%s>Member Login</a>' , ( ( isset( $user ) && '' !== $user ) ? ' href="https://www.paratuberculosis.com/login.php"' : '' ) ); Personally, I'd omit the whole element, like this, but YMMV: if ( isset( $user ) && '' !== $user ) echo '<a class="custom-btn custom-btn--small custom-btn--style-4" href="https://www.paratuberculosis.com/login.php">Member Login</a>' ; Regards, Phill W.
-
Store date values in Date fields. MySQL knows how to do "date things" with Date fields, including sorting them. It doesn't know how to do "date things" with Varchar fields. Convert your data [once], store it correctly, and wave goodbye to [almost] all your date-related problems. Regards, Phill W.
-
. . . And your question is? Regards, Phill W.
-
php update mysql database with form/checkbox
Phi11W replied to tonypoli783's topic in PHP Coding Help
Short answer: Your HTML is incorrect. You're missing the closing '>' on the Checkbox's input element. I'd also recommend using quotes on HTML attributes. The cases where you need them far outnumber those where you can get away without them. I'd suggest something more like this: echo '<form method="post" action="#">'; while( $row = mysqli_fetch_assoc( $result ) ) { printf( '<center>' . '%1$s %2$t<br>' . '%3%s %4$s<br>' . '%5$s[%6$s] vs %7$s[%8$s]<br>' . '<input type="checkbox" value="%5$s" name="team"> . '</center>' , $row['data'] , $row['time'] , $row['country'] , $row['lega'] , $row['team1'] , $row['pos_t1'] , $row['team2'] , $row['pos_t2'] ); echo '<input type="submit" value="submit"></form>'; Regards, Phill W. -
NOT NULL cannot be omitted if the field must never contain NULLs. Remember that the DEFAULT clause only applies when inserting new records and where this field value is not specified. Including NOT NULL prevents the value from being set to NULL at some later point, i.e. this statement would fail: update .. set quantity = NULL , TYPE = NULL where ... Without NOT NULL, it would work. Regards, Phill W.
-
how to hide data behind the = (page.php?id=xx) for the user
Phi11W replied to wildware's topic in PHP Coding Help
If you're using Sessions (not everybody does) you could capture the given QueryString Argument, save it into the Session, then redirect to the same page without the QueryString Argument, this time taking the id value from the Session. This may go some way to achieving what you want: if ( isset( $_GET[ 'id' ] ) { $_SESSION[ 'id' ] = $_GET[ 'id' ] ; http_response_code( 302 ); header( 'location: .../same_page_without_querystring_arguments' ); return; } if ( ! isset( $_SESSION[ 'id' ] ) ) { // No id available! header( 'location: .../errorpage.php' ); return; } $id = $_SESSION[ 'id' ]; // Display rest of page. Of course, it's not foolproof - anything that can be built can be broken and, at the end of the day, the browser simply has to know this value in order to request it! Regards, Phill W. -
Which field in Charters identifies the driver in question? SELECT chtr.id, chtr.charter_name, chtr.fleet_number, chtr.driver, chtr.customer_name, chtr.customer_number, chtr.dep_date, chtr.status /* \/ \____/ Which of these is the id into the users table? */ , usr.id, usr.fname FROM charters AS chtr LEFT JOIN users AS usr ON chtr.id = usr.id /* \/ Should this, perhaps, be chtr.driver? */ I would expect every table to have its own, unique id field and those ids are completely independent of one another (by which I mean Charter .id=6 is a completely different thing to Users .id=6). Regards, Phill W.
-
What does the fetch() function return? I think you need something more like this: $row = $stmt -> fetch(); echo( $row[ 'N1' ] ); Also, avoid using "select *" in Application code. Whilst you might not have a lot of columns in that table [yet], databases are inherently shared entities and you never know when someone [else] might add a dozen columns full of gigabytes of stuff that this query simply doesn't care about. Always select just the columns that you specifically need. Regards, Phill W.
-
What time does your hosting server (i.e. computer) think it is? That's what the time() function returns and if the clock on your server has "wandered" a bit, you'll get that "wandered" value. If it was out by exactly an hour, either way, I'd be thinking Timezone issues instead. Do you have a working NTP service running on your computer? That should keep your clock properly synchronised with the rest of the world. Regards, Phill W.
-
This is almost always the wrong way to do things. You cannot guarantee that this update process will run every, single day. This is Technology - stuff happens. Updating every record is a lot of [unnecessary] work for your database to do and will, almost certainly, lock the entire table, causing other issues. Showing stuff to Users is not the database's job. You'll write an Application that the Users interact with and that will show them your "remaining time". I'm sorry, but why? Users these days want instant responses, not arbitrary and artificially-enforced delays. If you are interested in a particular date & time, then work out when that is and store that. You never need to change it, "in bulk" or at all, Your application can calculate how long it is until "Real Time" catches up with it and show that duration to the User, no matter what they do in the meantime (refreshing, logging off-and-on again, etc. ), and You can easily tell once you have reached it in a SQL query. Keep it Simple ... Regards, Phill W.
-
I would go further and say you must not "select data in order to decide to insert or update it". That road leads to Race Conditions. Whist "on duplicate update" exists and works well, I would suggest making a conscious decision about which is the more likely condition to occur. In this case, I would say that updates are far more likely that inserts (with new pages only being added occasionally) so I would code the update first, and check to see whether zero rows were updated by it and, if so, insert the new row. Regards, Phill Ward.
-
The path is relative to where it starts from. Without any qualification ("inc/header.php"), it starts from the current directory, i.e. where the file doing the including is. With a leading slash ("/inc/header.php"), it will start at the root of the site. You might also be able to navigate "upwards", e.g. "../inc/header.php", but that's actively barred in some systems and will drive you mad if you have to refactor the site significantly. Regards, Phill W.
-
Five of your queries use "SELECT *". Do not do this in Production code. Databases are intrinsically shared entities and table structures can be changed [by anyone] at any time. Retrieving more fields than you actually need leaves you open to expected slow-downs, not of your making. Your remaining four queries could be combined into two. Taking the first pair, you can retrieve both aggregated values in one query: SELECT date_format(Date,'%Y') as month, COUNT(*) COUNT FROM calibrationdata WHERE Branch = '$userbranch' group by year(Date) order by year(Date) SELECT date_format(Date,'%Y') as month, sum(amount) FROM calibrationdata WHERE Branch = '$userbranch' group by year(Date) order by year(Date) // Can be combined into SELECT DATE_FORMAT( `Date`,'%Y' ) as month , COUNT( * ) as tally , SUM( amount ) as total FROM calibrationdata WHERE Branch = '$userbranch' GROUP BY year( `Date` ) ORDER BY year( `Date` ) Execute the query once, retrieve the values into an intermediate variable, then display that at the relevant point on the page. Also,. make sure that you have a database index supporting querying this table by Branch. Also, take a look at Parameterised Queries (Prepared Statements) to protect against SQL Injection Attacks. Obligatory XKCD Reference: Little Bobby Tables. Regards, Phill W.
-
Or, perhaps better (i.e. safer) ... switch( $_POST[ 'siti' ] ) { case: 'FIUMI' : case: 'MOLINI' : case: 'VITA' : $category = $_POST[ 'siti' ] ; break; default: throw new \Exception( 'Invalid category!' ); } Why? Just because you send the User an HTML SELECT list to use does not guarantee that the value you receive comes from that list! Regards, Phill W.
-
How does the User tell your code which "category" a file belongs to? Presumably, that would be another Form field, passed at the same time as the uploaded file itself. [Validate and then] Use that value to construct the target path for the file and pass that value to move_uploaded_file(). // pseudo-code if ( isset( $_POST[ 'btn-upload' ] ) ) { if ( validatePostArguments( $_POST ) ) { $targetFile = buildTargetPath( $_POST[ 'category' ], $_FILES[ 'file' ] ); move_uploaded_file( $file_loc, $targetFile ); } } Regards, Phill W.
-
You'll also note that my function returns a string which is then displayed by echo(). It's a subtle distinction but means that you can send that string result anywhere you want. You should retrieve the data up front and pass it to the templating "system", not the other way around. Having the templating "system" reaching out to get its own data whenever it needs it will cripple the application. You could wind up running dozens (or hundreds!) of queries where one would do just as well. The principle I'm trying to demonstrate here is that data retrieval (from the database) and creation of content (based on a "template") need to be separate functions and you use PHP code to get the data you want from one into the other. The "front-end" must be parameterised to take the data you pass it and apply those values to the template HTML. The "back-end" must retrieve the required data and put it in a form that you can pass to the "front-end". In my example, I used individual parameters, mainly for clarity. It sounds like you'd be better off passing an array, with key-value pairs containing the data. This allows the templating "system" to take whichever values it wants and use them and "ignore" any that it doesn't need. (This is the classic "XML" principle; a great idea, as long as you don't have to worry about security!). Regards, Phill W.
-
I think this is something like what you're after: function data () { //Abbrieviated code.... SELECT title, category, description, image_link FROM TABLE1 WHERE product_id=1; return $row ; } function template( string $title, string $category, string $description, string $image_link ) : string { $tmp = '<div class="t">TITLE %s</div>' . '<div class="l">CATEGORY %s</div>' . '<div class="m">DESCRIPTION %s</div>' . '<div class="r">IMAGE LINK <img src="%s"/></div>' ; return sprintf( $tmp, $title, $category, $description, $image_link ); } function go() { $row = data(); echo template( $row['title'] , $row['category'] , $row['description'] , $row['image_link'] ); } Regards, Phill W.
-
MySQL should be able to cope with loading 31000 rows with ease. What error(s) are you getting when try to load it? I would suggest loading the whole file into a "staging" table and then transferring data from that into your "proper" tables. Regards, Phill W.
-
In your login page, you need to extract the data from the data record you've retrieved and store it into the session, as you do for the username. $query = 'SELECT username, phone FROM users WHERE username=? AND password=?'; // bind parameters $result = mysqli_query($con, $query) or die(mysql_error()); $rows = mysqli_num_rows($result); if ($rows >= 1) { $_SESSION['username'] = $username; $_SESSION['phone'] = $result[ 'phone' ]; . . . Learn to use parameterised queries (which is much easier with PDO) to protect against SQL Injection attacks. Obligatory XKCD reference: Little Bobby Tables Never use "select *" in Production code. If somebody [else] adds some multi-Giga-byte columns holding the User's life story in video form, your super-quick login page suddenly slows to a crawl, having to read those massive fields that you've absolutely no interest in. Kudos for storing hashed passwords. Regards, Phill W.
-
PHP 8.1 How can I stop warning messages from displaying?
Phi11W replied to SLSCoder's topic in PHP Coding Help
You should be checking every single input value that comes from the client because you cannot trust anything that it sends to you! You have no control over the client's machine so you must regard it as completely untrusted. Just sending particular HTML to it does not count as control - just fire up the [free, installed-as-standard] "Developer Tools" in your web browser if you don't believe me. You must validate everything server-side and that means checking and cleansing every single input datum as it arrives. Would that not turn them on for every single User? Cue wide-spread confusion, complaints and bug reports! Yes, upgrades can be disruptive but I'd be surprised if every upgrade causes you problems. Or, perhaps, your codebase is simply older - the more versions you have to "jump" across, the more likely you're going to have problems. Sadly, software upgrading is like a diesel-powered hamster wheel - once you climb onto it and start running, it's very very hard to stop. (Unless you use VB6, of course - abandoned by "Our Friends in Redmond" in 2002 but still going strong today. 😉 ). To me, this sounds like you're either working with highly volatile data structures that change a lot or you have a lot of "dead" code handling data items that no longer exist. All the more reason to guard against missing elements properly. Regards, Phill W. -
Not sure about best, but this should do the trick: <?php sacconicase_post_thumbnail(); $am = get_the_author_meta( 'discount', $post->post_author ); if ( $am ) printf( '<div class="mostrasconto"> -%s%%</div>', $am ); ?> Regards, Phill W.
-
I don't think you need password_verify() at all here. When saving the password, hash the entered, plaintext, password and store the hashed value. When logging in, hash the entered, plaintext, password and compare that to what's in the database table. Saving password (as you currently have): $hashed_password = password_hash($password, PASSWORD_DEFAULT); . . . $sql = "INSERT INTO users (first_name, last_name, email, password_hash) VALUES (:first_name, :last_name, :email, :password_hash)"; . . . $stmt->bindParam(':password_hash', $hashed_password); Logging in: $email = $_POST['email']; $password = $_POST['password']; $hashed_password = password_hash( $password, PASSWORD_DEFAULT ); $sql = "SELECT a, b, c FROM users WHERE email = :email and password_hash = :hashed_password"; . . . $stmt = $pdo->prepare($sql); $stmt->bindParam(':email', $email); $stmt->bindParam(':hashed_password', $hashed_password ); $stmt->execute(); $user = $stmt->fetch(); If you get no rows back, either the username or entered password is wrong. Regards, Phill W.