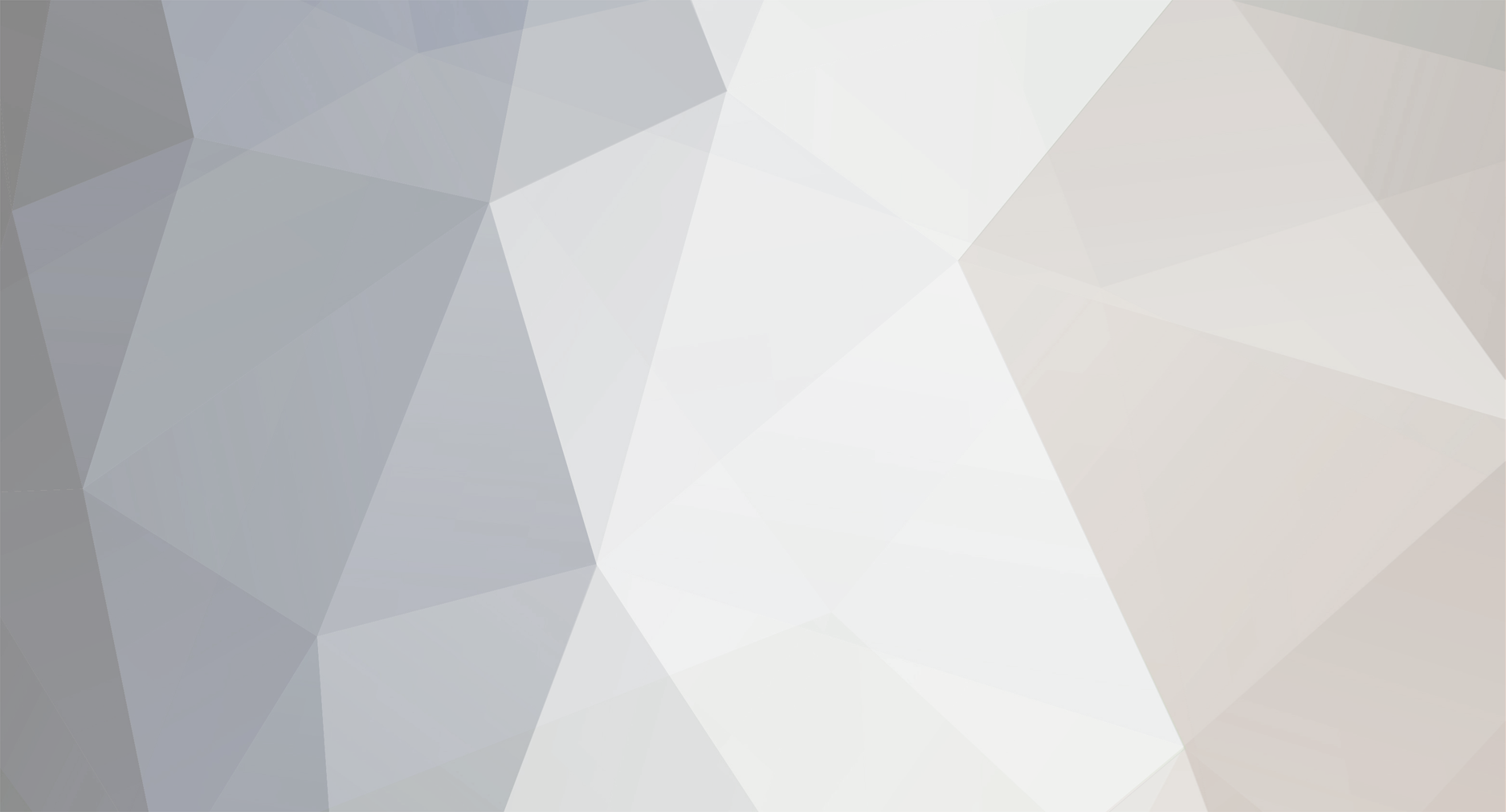
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
Yes. You pass a variable in the URL string. Fore example: <a href="yourpage.php?yourvar=somevalue&foo=bar&id=5">your link</a> yourpage.php: <?php $yourvar = $_GET['yourvar'];//contains somevalue $foo = $_GET['foo']; //contains bar $id = $_GET['id'];//contains 5; ?> As you can see, you separate the differant variables in the URL with an ampersand(&)
-
[SOLVED] $end ? WOE (What on Earth :P )
GingerRobot replied to maxudaskin's topic in PHP Coding Help
Its not too long a script. Post it up in tags. Let us take a look. -
Without having any idea of how your code currently works, its all going to be a bit difficult to say how you shoud do this. How are you storing the users profiles? Do you set sessions when someone logs in? Typically, you would have two pages. One would be something like editprofile.php. The other, viewprofile.php. editprofile.php would get the current user's profile from a database. You would search for their profile based on which user is currently logged in. Something like: <?php $user = $_SESSSION['user'];//presumably you are setting some sessions on login //here you would check to see if a form has been posted to a page, if so, check the new profile and update the databse $sql = mysql_query('SELECT `profile` FROM `users` WHERE `user`='$user'"); $profile = mysql_result($sql,0,"profile"); echo "<textarea name='profile'">$profile</textarea>"; //the rest of your form ?> Whislt viewprofile.php would be something like: <?php $id = $_GET['id'];//you would pass the id of the person's profile you want to look at through the url //e.g. viewprofile.php?id=123 $sql = mysql_query('SELECT `profile` FROM `users` WHERE `userid`='$id'"); $profile = mysql_result($sql,0,"profile"); echo $profile; ?> Its quite difficult to give anything definitive here. It all depends on how you are already doing things.
-
The equality comparison (==) will return true if two variables are equal. The identical comparison (===) will return true if the variables are equal AND of the same type. <?php if(0=='0'){ //true } if (0 === '0'){ //false } ?>
-
As you can see, you'll run into issues with anything like '~After' as the translator will think thats all a word and will try to translate the whole thing. However, the bigger issue is that you are only ever going to get a gisted translation from an online translator. Whilst a french speaker could probably understand the majority of the translated text, it wont read very well, and there will be plently of gramatical errors(particularly those relating to tenses). If online translators were better, i'd do far better in my french A-level
-
A newline character is not the same as an HTML line break. Whenever you OUTPUT your data that you have stored, you'll need the nl2br() function. You dont use it before you enter the data into the database.
-
I think this is more of an XHTML standards question. Every documentation that ive seen uses double quotes for enclosing the attributes of a tag. On the other hand, they all tend to just say that the attribute value must be enclosed in quotes(they do not specify double quotes) - but as i say, they all use double quotes in their examples.
-
If you know that there will always be 5 pieces of data for each line (that is, you will always have y co-ordinates 1-5 for each x co-ordinate), then i think this is fairly simple. If you sort your query by X then by Y you will get them in the order you need. When you loop through the data, you can then check to see if new rows are needed in your table dependant on the value of Y: <table> <?php $sql = mysql_query("SELECT * FROM `map` SORT BY `x` ASC, `y` ASC") or die(mysql_error()); while($row = mysql_fetch_assoc($sql)){ if($row['y']==1){ echo '<tr>'; } echo '<td>'.$row['type'].'</td>'; if($row['y']==5){ echo '</tr>'; } } ?> </table> This might need to be more complex - it depends how your maps work.
-
I think you misunderstood. Thorpe was asking what you meant when you said "its not working". What's not working? Do you get an error message?
-
d: sıɥʇ punoɟ noʎ ʍoɥ ɹǝpuoʍ oʇ ǝʌɐɥ ı
-
If you are unsure of your database name and password, you'll need to contact your host. However, you say that you know your database is called dbtables. How do you know this? Do you log in somewhere(eg. phpMyAdmin) and it shows you this? If so, its likely that the username and password you enter here is the ones you need. The server will most likely be localhost. Again though, you might need to contact your host/look through their FAQs
-
[SOLVED] how do I ensure that numbers are entered into a variable?
GingerRobot replied to kr3m3r's topic in PHP Coding Help
Using regular expressions to check for numeric data is a bit unnecessary. There are quicker methods. -
Im now a little confused. Are you trying to empty a form of all its values? Or are you trying to not display any more information? If its the latter, then i think its already been explained. You need to do something like: <?php //no output before this line if($_POST['yourfield'] == 'b'){ echo 'b'; }else{ //ALL the rest of your code } ?> Or, alternatively: <?php //no output before this line if($_POST['yourfield'] == 'b'){ echo 'b'; exit; } //the rest of your code ?>
-
Use the nl2br() function when you output data that came from a text box. It converts all new lines(\n) to html <br /> tags.
-
Yep. If your method is post and the name of the text area was textarea: <?php if(strlen($_POST['textarea']) > 100){ //too long }else{ //length ok } ?>
-
If you dont have the ability to run a cron, then just store the last active time as was suggested, and then for your users online page, select only those users who were last active less than 5/10 minutes ago.
-
Use the strlen() function.
-
grabbing select information from a page
GingerRobot replied to android6011's topic in PHP Coding Help
Its not that confusing Take a look at the file_get_contents() and the preg_match() functions -
File and Directory Lister. Not working (Fully)
GingerRobot replied to AbydosGater's topic in PHP Coding Help
You might find this useful. I made this for another topic somwhere: <?php function no_of_files($start_dir,$sub_dir=FALSE){ $no_of_files=0; if($sub_dir === false){ $handler = opendir($start_dir); }else{ $start_dir = $start_dir.$sub_dir; $handler = opendir($start_dir); } while(false !== ($file = readdir($handler))){ if($file != '.' && $file != '..'){ if(is_dir($start_dir.'\\'.$file)){//if this is a directory, recall the function with the subdirectory defined $dir_name = '\\'.$file; echo $dir_name.'<br />'; $no_of_files += no_of_files($start_dir,$dir_name); }else{//is a file, so increase our counter $no_of_files++; } } } return $no_of_files; } echo no_of_files('C:\Documents and Settings\Ben\My Documents\My Music'); ?> This was to return the number of files(not folders) in a given directory and all its sub directories. Could be modified to echo names and include folders etc -
Take a look at the sticky here In your script, you must be sending something to the browser before you call session_start(), which you cannot do.
-
[SOLVED] Display only single record set title on page
GingerRobot replied to yandoo's topic in PHP Coding Help
Well im not entirely sure from your question, but i think the problem is that you close the table inside of your loop. You need to close the table outside of it: <?php if (mysql_num_rows($res) > 0) { echo"<table width=\"700\" border=\"1\" class=\"border_bottom\"> \n"; echo "<tr> \n"; echo "<td>"; echo "<strong>Loan ID</strong>"; echo "</td>"; echo "<td>"; echo "<strong>Client Name</strong>"; echo "</td>"; echo "<td>"; echo "<strong>Student Name </strong>"; echo "</td>"; echo "<td>"; echo "<strong>Laptop Name</strong>"; echo "</td>"; echo "<td>"; echo "<strong>Username</strong>"; echo "</td>"; echo "<td>"; echo "<strong>Date</strong>"; echo "</td>"; echo "<td>"; echo "<strong>Complete</strong>"; echo "</td>"; echo "</tr>"; while($result = mysql_fetch_array($res)) { echo "<tr>"; echo "<td width=\"75\" style =\"text-align: left\""; ?> <em><a href="search_loan_details.php?recordID=<?php echo $result['LoanID']; ?>"><?php echo $result['LoanID']; ?> </a></em> <?php echo " </td>"; echo "<td>"; echo "<em>"; echo $result['Client']; echo "</em>"; echo "</td>"; echo "<td>"; echo "<em>"; echo $result['StudentName']; echo "</em>"; echo "</td>"; echo "<td>"; echo "<em>"; echo $result['LaptopName']; echo "</em>"; echo "</td>"; echo "<td>"; echo "<em>"; echo $result['Username']; echo "</em>"; echo "</td>"; echo "<td>"; echo "<em>"; echo $result['Date']; echo "</em>"; echo "</td>"; echo "<td>"; echo "<em>"; echo $result['Complete']; echo "</em>"; echo "</td>"; echo "</tr> \n"; } echo "</table> \n"; echo"<br>"; } else echo 'no result'; -
Has anyone else created(or is planning on creating) a team for the fantasy football on the premier league website (www.premierleague.co.uk) If you've not heard of it, and you follow english football, then take a look at it. You basically create a dream team of players for a set budget, and the players earn points depending on their performance over the course of the season. I'm just wondering if its worth starting a phpfreaks league on there
-
my login doesn't write to banip.txt or to my hacklog
GingerRobot replied to CBaZ's topic in PHP Coding Help
Well, as far as i can see, you dont actually attempt to write to your file. You need the fwite() function. Also, you should be getting an error when your run this script anyway. Where you have this: <?php //echo "sql: ".$query." "; ?> it will produce an error. The double forward slashes are single line comments - so by having this statement broken over two lines, the "; will still be parsed, giving an error. Try: //echo "sql: ".$query.""; -
While it is often useful having plenty of the source code, its more useful having the relevant bit highlighted too us too Also, for future refernce, if you use full php opening tags(<?php) we get syntax highlighting which makes it a whole stack easier to read. Anyways, there is an FAQ on exactly this subject: http://www.phpfreaks.com/forums/index.php/topic,95426.0.html Hopefully that might guide you in the right direction.
-
Well, if you're only retrieving data from the post array then its not a problem is it?