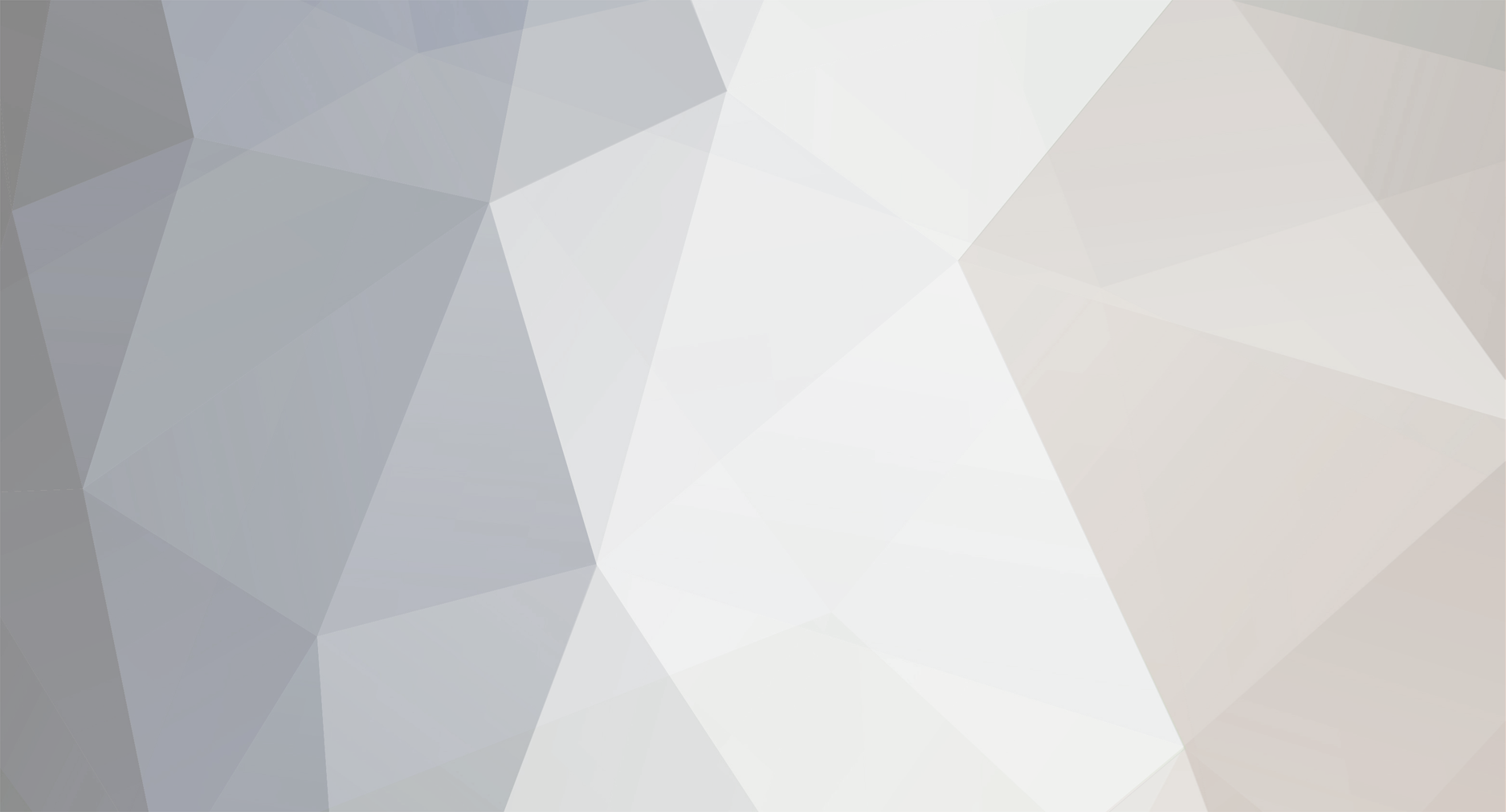
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
Well i dont think anyone is just going to do something for you. And like i said, we'd need to know a bit more information about what you are trying to do before we can help you. What is the site that you are trying to get information from?
-
Well, generating a list of all the files in the directory is reasonably straight forward. Take a look at the examples on the php website for readdir() You could then use the fread() function to get the contents of each file and find your name.
-
So you are looking to get the contents of another website, find a table within that website, and print out that particular table? Depending on the website you are getting the contents from, you can either use file_get_contents() or you may have to use cURL and php. Once you have the contents of the page in a variable, you will need to use regular expressions to find your particular table. The preg_match() function is what you'll need. To be able to give you any more help, we're probably going to need a bit more detail.
-
Given that your waning to get the index of the duplicates, i think your best bet is to cycle through the array and create a new array. Before you enter data into this new array, you will check if it is already in the array: <?php $myarray = array('0123456789first line','0123456789second line','0123456789first line');//just an example to test $new_array = array();//we're going to put new values into here $duplicates = array();//and we'll put the keys of any duplicate values here foreach($myarray as $key => $value){ $value = substr($value,10);//strip out first 10 characters if(!in_array($value,$new_array)){//check if the value is in our new array $new_array[] = $value; }else{ $duplicates[] = $key; } } ?> So you end up with two arrays, $new_array which is effectively what you would have got from using the array_unique() function on your original array - each value is in the array only once, and you have $duplicates which contains the keys from your original array of the duplicated line(s)
-
Insert string into String based on number of chars
GingerRobot replied to zfred09's topic in PHP Coding Help
Just need to use substr(): <?php $string = "I swear I have seen this before."; $addme = '**'; $add_after = '10'; $new_string = substr($string,0,$add_after).$addme.substr($string,$add_after); echo $new_string; ?> -
[SOLVED] ForEach limited for two items per column
GingerRobot replied to forzatio's topic in PHP Coding Help
Im not 100% sure from your description, but i think this is what you are after: http://www.phpfreaks.com/forums/index.php/topic,95426.0.html -
As i said above: If you look at the error message, it says that it assumes that you wanted to use the string "available". Therefore, php is capable of dealing with the error - basically it just guesses what you wanted. The default setting for error_reporting prior to php 5 did not report errors of this kind. It just attempted to deal with them. Edit: Whoops, saw there was added messages but only registered redarrow's . Ah well, ill leave this here - perhaps it explains a bit further. Glad you understand.
-
Im not 100% sure about the mysql errors, but the problem with the undefined constant is that you've closed the quotes - so php thinks your wanting to use a constant called Available. Try: <?php $query = "SELECT Class.ClassName, Class.StartDate, Site.SiteName FROM Class as a JOIN Site as b ON (a.ClassId = b.SiteId) WHERE a.ClassId='".$Classid."' AND a.Space='Available'"; $result = mssql_query($query,$db) or die ("mysql_error") echo $query; ?> They changed which errors were reported in php 5 to include undefined constants and variables. Hence why you've only just seen the error, even though it was there all along.
-
MySQL is a database - it is not part of php. However, chances are, if your server has php installed then it will also have mysql installed. If you create a php file with just this in: <?php phpinfo(); ?> And run it on your server, you should find a section on mysql. If so, mysql is installed. You dont have to use mysql - there are other databases. However, most people do tend to use it. On the otherhand, you dont HAVE to use a database at all. You COULD use text files. However, you'll probably find it far easier to use mysql anyway - working with text files isn't the easiest thing. Membership systems probably aren't too hard a thing to learn, although it does depend on how much you have done already. You might find it better to try and write a few little things, and perhaps get used to using mysql a bit, before you try. However, having something which you're aiming to do will probably help you learn. There's an introduction to mysql here: http://www.phpfreaks.com/tutorials/33/0.php And a tutorial on membership systems here: http://www.phpfreaks.com/tutorials/78/0.php
-
[SOLVED] updating multiple rows in a table in mysql
GingerRobot replied to gevo12321's topic in PHP Coding Help
Where is the data going to be coming from? A form? When/why will the number of rows change? Without anymore details, we will largely be guessing, but assuming you're using a form, and the data is in arrays, a while or foreach loop will do fine: <?php foreach($ids as $value){ $name = $names[$value]; $source = $sources[$value]; mysql_query("UPDATE `layout` SET `name`='$name', `source`='$source' WHERE `id`='$id'") or die(mysql_error()); } ?> So this is assuming you have 3 arrays(ids, names, sources) where the id being updated is the key of the names and sources array. But it all depends on how you want to do this. -
functions which are not defined but which they can be called
GingerRobot replied to radalin's topic in PHP Coding Help
I cant really think of anyway to do what your after; and i really cant see the point of it either. Perhaps if you showed where you were actually planning on using a technique like this, someone might be able to suggest a way of doing it? -
Try: <?php function dbConnect($type) { if ($type == 'query') { $user = 'paul';//you need to enclose your strings in quotes $pwd = 'mouse'; } else { exit('Unrecognized connection type'); } $conn = mysql_connect('sql1.bravehost.com', $user, $pwd) or die ('Cannot connect to server<br />'.mysql_error());//its always useful to put in the mysql_error() function in your die statements. That way you can see exactly what mysql had a problem with. mysql_select_db('fiction-1261311') or die ('Cannot open database'); return $conn; } As was said above, if you want to put a piece of text into the function, you'll need to put it in quotes - however, given that you've defined the variables $user and $pwd, im assuming you want to use those. When you define those, you still need to enclose the string in quotes though. Edit :Finally, if those are your real username and password, its unwise to post them.
-
php include statments runs all functions?
GingerRobot replied to calmchess's topic in PHP Coding Help
Are you calling your functions inside your included page or just defining them? Your functions should not run unless you call them somewhere. -
No not at all. Get variables are passed to a page in the URL. If you specify the form method as get, all it actually does it put the contents of the form in the url. You would make your links something like: echo "<a href='deleteuser.php?id=$row[CallID]'>$row[CallID]</a>";
-
Well im guesing that your 'CallID' field is a unique field? If so, just pass that into the query string, as you do for your editUser.php page, and delete the row: <?php $id = $_GET['CallID']; mysql_query("DELETE FROM `person` WHERE `CallID`='$id'") or die(mysql_error()); ?>
-
[SOLVED] Can someone help me find the error please.
GingerRobot replied to HaLo2FrEeEk's topic in PHP Coding Help
If you are trying to check if a variable is empty, use the empty() function. -
Yep, thats right. It's completely unreliable. Some firewalls also prevent/have an option of preventing the referrer being sent.
-
Dont mean to be picky, but you should use the strstr() function for this as its faster.
-
So basically what your saying is that at the top of your script, you are checking for errors - which if there are any you want to echo the result. Further down, you also might redirect someone(presumably if there are no errors?) If so, i would store the error text in a variable, and perhaps set another variable, $errors for example to the value yes: else { $error_text .= "<font color=\"#FF0000\">The Promotional code that you have entered does not exists or has already been redeemed.</FONT><BR><BR>"; $errors = 'yes'; } Then, wherever you would want to show these errors, you would use: <?php if($errors =='yes'){ echo $error_text; } ?> Meh, beaten to it - ill post anyway since ive typed out the example.
-
As far as im aware, mysql is case insensitive anyway.
-
Ah, i've finally got it - this was really annoying me. Once the first function in your if statement returns false, php doesn't bother to evaluate the second part of it(and therefore the function does not run) - obviously it would be a waste of processing power since it has already found out that the if statement will overall be false. What you'll have to do, is fun the two functions and store the output into variables: <?php $email_success = validate_email($email); $password_success = validate_password($password); if($email_success === true && $password_success === true){ //both true }else{ //one or more false } ?>
-
Edit: Scrap that, 'twas wrong
-
Your answer lies in javascript. You should be able to find it easily if you google it.
-
Yes, that was entirely my fault. Should have been a while statement. No idea where the foreach came from!
-
I think what you're after is: <?php $var1 = 'Hello'; $var2 = 'Hello again'; for($a=1;$a<=2;++$a){ $var = 'var'.$a; echo $$var; } ?>