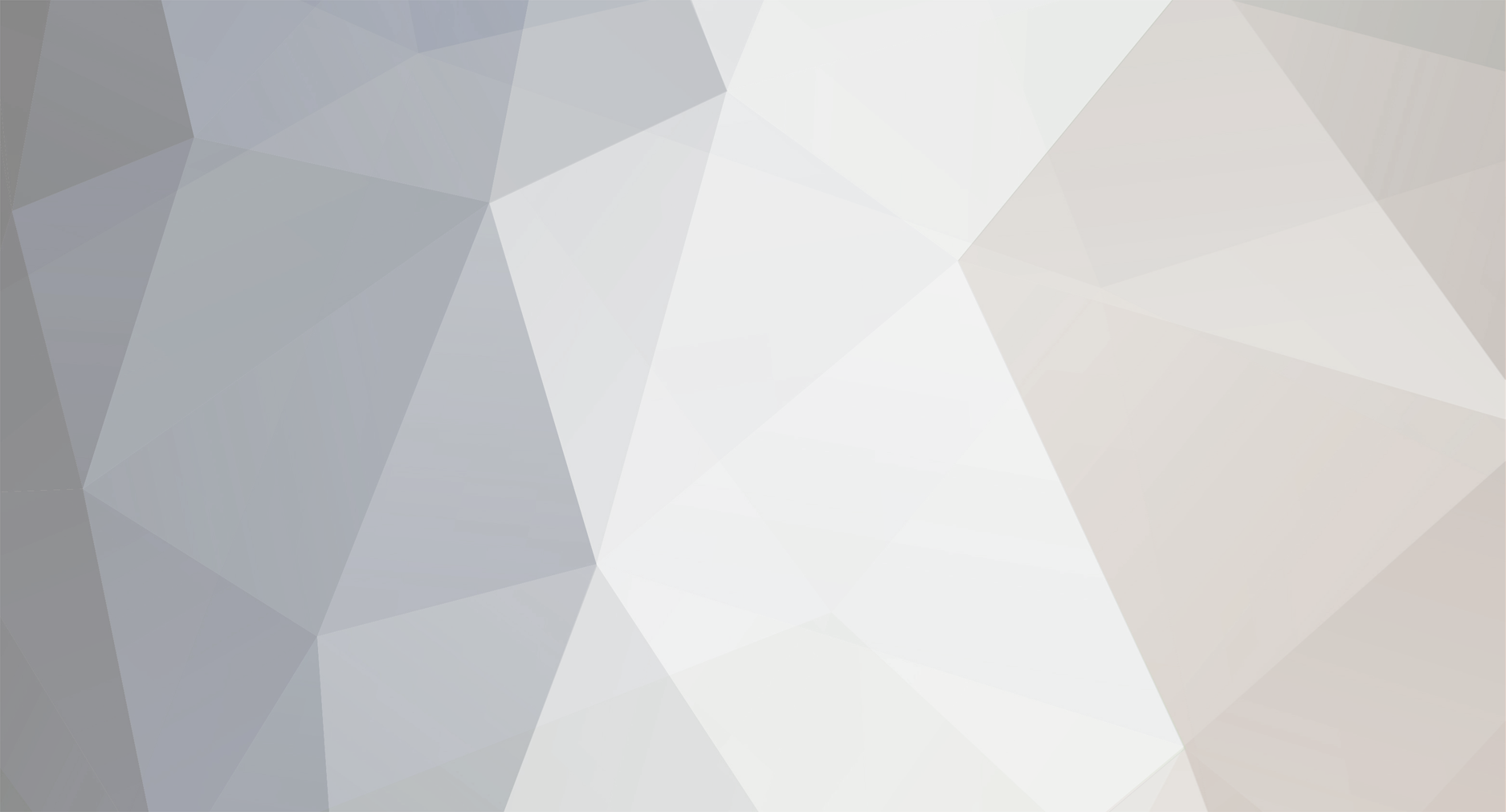
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
The use of type casting to prevent sql injection is actualy a recommended process by Zend. If you define a variable as an integer, then attempt to assign a string to it, the integer will be 0. <?php $myvar = (int) 'test'; echo $myvar; //echos 0 ?>
-
To simply check for the presence of a character, use the strpos() function. However, to create proper validation for email addresses, look into using regular expressions.
-
I forgot that if one of them is null, the last character wouldn't be a comma. So this now checks to see if there is a comma in the string. If there isn't, it simply appends the current part(first name, last name or suffix) to the end of the string. If there is, it replaces the comma with the current part. <?php if($creator['Fname'] !=Null){ $name = $creator['Fname'].','; } if($creator['Lname'] !=Null){ $name =(strpos($name,',')===false) ? $name .= ' '.$creator['Lname'].',' : substr_replace($name,' '.$creator['Lname'].',',strlen($name)-1); } if($creator['suffix'] !=Null){ $name =(strpos($name,',')===false) ? $name .= ' '.$creator['suffix'].',' : substr_replace($name,' '.$creator['suffix'].',',strlen($name)-1); } echo $name; ?>
-
I would suggest that you build a string as you go when you do this part: <?php foreach($creators as $creator){ if($creator['Fname'] !=Null){ echo " ".$creator['Fname'].""; } if($creator['Lname'] !=Null){ echo " ".$creator['Lname'].""; } if($creator['suffix'] !=Null){ echo " ".$creator['suffix'].""; } } ?> Where you add a comma after each part of the name, and then strip it out if the next part is not null. Something like: <?php if($creator['Fname'] !=Null){ $name = $creator['Fname'].','; } if($creator['Lname'] !=Null){ $name = substr_replace($name,' '.$creator['Lname'],strlen($name)-1).','; } if($creator['suffix'] !=Null){ $name = substr_replace($name,' '.$creator['suffix'],strlen($name)-1).','; } echo $name; Edit: Hang on, this needs modifying slightly.
-
I may have missed the point, but if you are just using static data(ie, you wont be retrieving any data from a database after the selection of a checkbox) then you might as well just use javascript to do this. If you will be quering the database based on a selection and want to change a part of your page based on the result, use AJAX.
-
Ok, so if javascript is definately being used, then you could use ajax to load a php script to do all the server side error checking. If there are no errors, you can submit the form to another php page(which will not need to do any error checking), to deal with the data. If there are errors, alert the user and do not submit the form.
-
Got bored, so i put this together: <?php function no_of_files($start_dir,$sub_dir=FALSE){ $no_of_files=0; if($sub_dir === false){ $handler = opendir($start_dir); }else{ $start_dir = $start_dir.$sub_dir; $handler = opendir($start_dir); } while(false !== ($file = readdir($handler))){ if($file != '.' && $file != '..'){ if(is_dir($start_dir.'\\'.$file)){//if this is a directory, recall the function with the subdirectory defined $dir_name = '\\'.$file; $no_of_files += no_of_files($start_dir,$dir_name); }else{//is a file, so increase our counter $no_of_files++; } } } return $no_of_files; } echo no_of_files('C:\Documents and Settings\Ben\My Documents\My Music'); ?> Tested and compared with the number of files reported by windows. Seems to work fine to me.
-
I dont think you are really going to find a good way of doing this. Any sort of pop-up message to the user would have to be given by reloading the page with a particular variable defined(e.g. : yoursite.com/yourscript.php?errors=yes&errormsg=incorrect user name or password) To then produce the pop up you would have to have php echo some javascript. Therefore, if the user doesn't have javascript turned on, then they wont ever see the error message. As far as i can see, there will always have to be alternative error messages for people who do not have javascript turned on. However, it may be that you could you ajax to test for any errors that the php script would generate(such as an incorrect username/password) and display them nicely for users with javascript.
-
Passing sensitive information using GET or POST
GingerRobot replied to mattd55's topic in PHP Coding Help
You could always post the data to the second website using cURL. Im not sure if there are any particular security issues with this sort of method however. -
Well, you can use variable variables: <?php $var1 = 'foo'; $var2= 'bar'; for($x=1;$x<=2;$x++){ $var_name = 'var'.$x; echo $$var_name.'<br />'; } ?> However, you are probably better off using multidimensional arrays. For example, this: <?php $arrays = array(array('something','somethingelse'),array('anothersomething','somethingmore')); echo '<pre>'; print_r($arrays); echo '</pre>'; ?> Would produce: Array ( [0] => Array ( [0] => something [1] => somethingelse ) [1] => Array ( [0] => anothersomething [1] => somethingmore ) ) As you can hopefully see, you could cycle through the various "dimensions" of the array.
-
Take a look into php and AJAX. You'll need to use ajax to fill the second drop down box after searching the database.
-
User is a reserved word in PostgreSQL. Having only ever used mysql, i cant guarantee it, but i would guess the quickfix is to use backticks (`) around your field names: $result = pg_exec($dbconn, "UPDATE `user` SET `name` = '$username', `password` = '$userPassword' WHERE id = '$userID'"); The proper solution is to not use reserved words as table or column names.
-
Haha, i was annoyed there wasn't an array_average() function built in
-
Perhaps a shorter way to do it: <?php $book = array("newbook" => array(10, 20, 30), "usedbook" => array(15, 25, 35)); foreach($book as $key => $value){ $average = array_sum($value) / count($value); echo "Average of: $key = $average <br />"; } ?>
-
I felt the easiest way to answer your questions was to create a working example. If you set up a table(called users in my example) with two fields , id and username. Populate it with a few bits of data. Or you can import this using phpMyAdmin if its easier: CREATE TABLE `users` ( `id` int(20) NOT NULL auto_increment, `username` varchar(100) NOT NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=latin1 AUTO_INCREMENT=7 ; -- -- Dumping data for table `users` -- INSERT INTO `users` (`id`, `username`) VALUES (1, 'tom'), (2, 'dick'), (3, 'harry'), (4, 'jim'), (5, 'bob'), (6, 'bill'); Then, the script: <html> <head> </head> <body> <?php include("test_db_connection.php"); if(isset($_POST['submit'])){//form has been submitted, so process it if(count($_POST['edituser']) > 0){//first check to see if we are trying to edit anyone. otherwise we get an error foreach($_POST['edituser'] as $key => $value){ //as the index of each element of the array, $key will contain the user ID $new_username = $_POST[username][$key]; mysql_query("UPDATE `users` SET `username`='$new_username' WHERE `id`=$key") or die(mysql_error()); echo "User $key# has changed their name to $new_username <br />"; } } if(count($_POST['deleteuser']) > 0){//first check to see if we are trying to delete anyone. otherwise we get an error foreach($_POST['deleteuser'] as $key => $value){ mysql_query("DELETE FROM `users` WHERE `id` ='$key'") or die(mysql_error()); echo "User $key# has been deleted<br />"; } } } ?> <form action="phpfreaks.php" method="post"> <table border="1"> <tr><td>User ID# </td><td>Username</td><td>Edit?</td><td>Delete?</td></tr> <?php $sql = mysql_query("SELECT * FROM `users`") or die(mysql_error());//grab all our users from the table while($row = mysql_fetch_assoc($sql)){//cycle through each user echo "<tr><td>$row[id]</td><td><input type='text' name='username[$row[id]]' value = '$row[username]' /></td><td><input type='checkbox' name='edituser[$row[id]]' /></td><td><input type='checkbox' name='deleteuser[$row[id]]' /></td></tr>"; //notice how i use the id as the index for all the arrays. This is because this is the the one thing that uniquely identifies each person } ?> <tr><td colspan="4"><input type="submit" name="submit" value="Edit/Delete" /></td></tr> </table> </form> </form> </body> </html> Its just a quick piece of code to show you how it all works. But its tested and does work. Play around with it , i hope it will answer your questions. If there's any of the code you dont understand, feel free to ask.
-
There is an FAQ for multi column results from a database. Although you are getting your information from a text file, the same methods still apply. Take a look at: http://www.phpfreaks.com/forums/index.php/topic,95426.0.html Hopefully you'll be able to work it out from that.
-
Well, first off, i suggest you try and learn a little about arrays. There's an introduction to them here: http://www.phpfreaks.com/tutorials/42/0.php Edit: plus there's a section from a free online php book about arrays here: http://hudzilla.org/phpwiki/index.php?title=Arrays Try reading through those first and see if you can understand the way this might work.
-
Projects in PHP and MySQL Web Development: User Authentication and Personalization Shopping Cart Content Management System Web-Based Email Service Mailing List Manager Web Forums Generating PDFs Using XML and SOAP I must admit, ive not really gone through any of the large projects in the book, i tend to use it more for reference. However, i find it well written, easy to read and informative. Ive not tried any of the other books.
-
Why do you not want to use arrays? It would be far easier. You could go down the route of hidden fields etc, but arrays are a much better idea.
-
For your first question, yes its fine to use non-numeric indexes. As for your second question, im not entirely sure what you mean. Could you post the code you have?
-
Edit : Whoops, i didn't read your post properly. If, as i would guess, you have a number of these form elements that you wanting to put into a database, you can use arrays. An example might be: <input type="text" name="name[0]" /> <input type="text" name="name[1]" /> <input type="text" name="name[2]" /> You can then use a loop on the array: <?php $name= $_POST['name']; foreach($name as $value){ //insert the name into the database etc } ?>
-
Hmm, well, you could always store the time that the teacher starts the timer, and wether or not you are accepting data. Then, each time any data is supposed to be put into the database, you first check to see if you are supposed to be accepting data, and then you can check to see if the timer was started more or less than 20 minutes previously. If it is less than, you can put the data into the database, if it more than, you can update the field in the database to not accept data, and reject the data. Perhaps you could improve this with cron jobs. Ive never used them so im not too sure. Definately. The GD library in php can be used to create graphs. One of the staff members on the site, barand, has written a class for creating charts. You might find it useful: http://members.aol.com/barryaandrew/baaChart/baachartguide.html
-
You're going to need to use regular expressions for this. Although, as you have done, you can replace parts of the text with str_replace, if you use the ereg() or preg_match() functions, you can also return text between the two things. There are a couple of tutorials on this site for this sort of thing too. You might find them useful: http://www.phpfreaks.com/tutorials/141/0.php http://www.phpfreaks.com/tutorials/123/0.php
-
Try using: if ($id == "") {$id = "noid";} rather than: if ($id == "") {$id == "noid";} == is the comparison operator - so you use it to compare two values for equality = is the assignment operator - you use this to give a variable a value.
-
Surely this line here: if (is_array($errors)){ Should be: if (!is_array($errors)){ After all, you only want to execute the next bit of code if there are no errors.