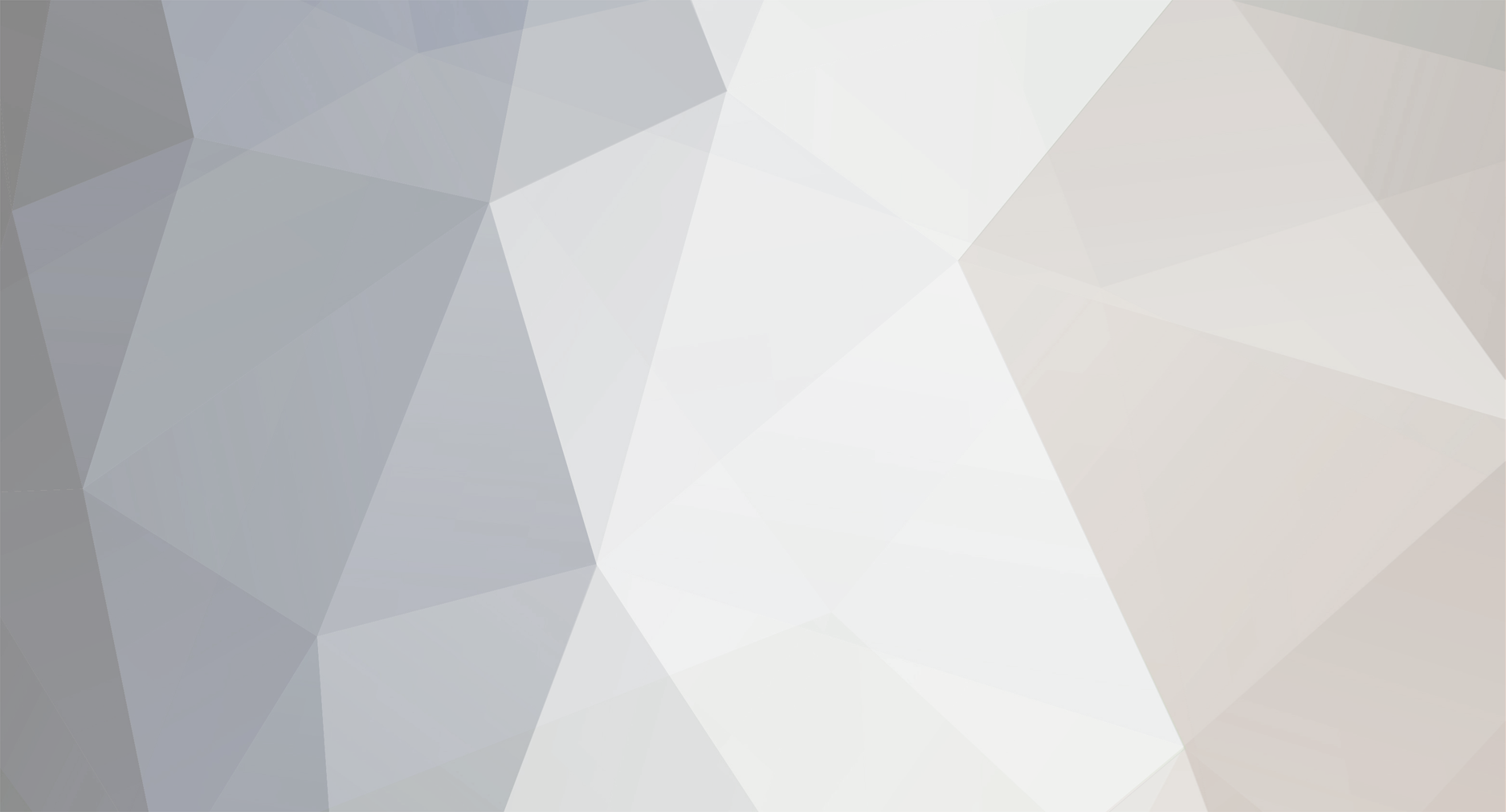
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
Well if you were wanting to select all of your friends with images (which i think is what you wanted?), you can do: <?php $sql = "SELECT friendname,image FROM friends,users WHERE friends.username='runnerjp' and friends.friendname=users.Username ORDER BY friendname"; $result = mysql_query($sql) or die(mysql_error().'<br />Query was:'.$sql); while(list($friendname,$image) = mysql_fetch_row($sql)){ echo $friendname.'<br />'.$image.'<br />'; } ?>
-
If gameid is an integer: $result = mysql_query("SELECT * FROM myscore2 WHERE gameid=$_GET['gameid'] ORDER BY score DESC") Otherwise: $result = mysql_query("SELECT * FROM myscore2 WHERE gameid='".$_GET['gameid']."' ORDER BY score DESC")
-
Well we might need to see the actual code. Because you shouldn't have the new keyword there either, and you'd get an error message if you do have it there.
-
Because you close the while loop before you execute the second query. The result: only the last friend gets queried. In any case, you dont want to do it like that. Use one query. If you post up your database structure (firends and users table as well as a bit of sample data) ill show you how.
-
[SOLVED] Form to kill two birds with one stone..
GingerRobot replied to ag3nt42's topic in PHP Coding Help
Unless your posting to another website, then why not? You can handle the form's on one page and redirect someone to the appropriate page after. -
I would imagine the problem relates to that fact that zip_open doesn't return false or error, it returns an error number. So, you should be doing: $test = zip_open($zip_name); if (is_resource($test)){ $zip_close(); echo 'ok'; } else { echo 'failed'; } And courtesy of saulius at solmetra dot lt from http://www.php.net/zip_open (strangely there appears to be no official documentation on the error numbers, unless im being blind) : function zipFileErrMsg($errno) { // using constant name as a string to make this function PHP4 compatible $zipFileFunctionsErrors = array( 'ZIPARCHIVE::ER_MULTIDISK' => 'Multi-disk zip archives not supported.', 'ZIPARCHIVE::ER_RENAME' => 'Renaming temporary file failed.', 'ZIPARCHIVE::ER_CLOSE' => 'Closing zip archive failed', 'ZIPARCHIVE::ER_SEEK' => 'Seek error', 'ZIPARCHIVE::ER_READ' => 'Read error', 'ZIPARCHIVE::ER_WRITE' => 'Write error', 'ZIPARCHIVE::ER_CRC' => 'CRC error', 'ZIPARCHIVE::ER_ZIPCLOSED' => 'Containing zip archive was closed', 'ZIPARCHIVE::ER_NOENT' => 'No such file.', 'ZIPARCHIVE::ER_EXISTS' => 'File already exists', 'ZIPARCHIVE::ER_OPEN' => 'Can\'t open file', 'ZIPARCHIVE::ER_TMPOPEN' => 'Failure to create temporary file.', 'ZIPARCHIVE::ER_ZLIB' => 'Zlib error', 'ZIPARCHIVE::ER_MEMORY' => 'Memory allocation failure', 'ZIPARCHIVE::ER_CHANGED' => 'Entry has been changed', 'ZIPARCHIVE::ER_COMPNOTSUPP' => 'Compression method not supported.', 'ZIPARCHIVE::ER_EOF' => 'Premature EOF', 'ZIPARCHIVE::ER_INVAL' => 'Invalid argument', 'ZIPARCHIVE::ER_NOZIP' => 'Not a zip archive', 'ZIPARCHIVE::ER_INTERNAL' => 'Internal error', 'ZIPARCHIVE::ER_INCONS' => 'Zip archive inconsistent', 'ZIPARCHIVE::ER_REMOVE' => 'Can\'t remove file', 'ZIPARCHIVE::ER_DELETED' => 'Entry has been deleted', ); $errmsg = 'unknown'; foreach ($zipFileFunctionsErrors as $constName => $errorMessage) { if (defined($constName) and constant($constName) === $errno) { return 'Zip File Function error: '.$errorMessage; } } return 'Zip File Function error: unknown'; } //making your code: $test = zip_open($zip_name); if (is_resource($test)){ $zip_close(); echo 'ok'; } else { echo 'failed<br />'; echo zipFileErrMsg($zip); }
-
[SOLVED] Form to kill two birds with one stone..
GingerRobot replied to ag3nt42's topic in PHP Coding Help
Why bother? Give the submit buttons different names and handle them on the same page. -
No. Most definitely not. Your first way is correct. Try reading up on database normalization. See this topic to get started.
-
Sorry? Are you trying to insert data or select data?
-
Or even quicker: function function2($string1, $string2) { $array1 = str_split($string1); $array2 = str_split($string2); $count1 = count($array1); $count2 = count($array2); if(count(array_intersect($array1,$array2))==$count1 &&$count1==$count2){ return true; }else{ return false; } } Interestingly, the speed difference is more noticeable with a set of numbers than a set of letters. Not sure why that should be, though. <?php $string1 = 'abcdefghi'; $string2 = 'ihgfedcba'; $string1 = '1234567'; $string2 = '7654321'; function function1($string1, $string2) { $array1 = str_split($string1); $array2 = str_split($string2); sort($array1); sort($array2); if($array1 == $array2){ return true; }else{ return false; } } function function2($string1, $string2) { $array1 = str_split($string1); $array2 = str_split($string2); $count1 = count($array1); $count2 = count($array2); if(count(array_intersect($array1,$array2))==$count1 &&$count1==$count2){ return true; }else{ return false; } } $t1 = microtime(true); for($x=0;$x<=10000;$x++){ function1($string1,$string2); } $t2 = microtime(true); for($x=0;$x<=10000;$x++){ function2($string1,$string2); } $t3 = microtime(true);//end time //now output results printf ("1st Method: %0.8f<br>2nd Method: %0.8f<br>Ratio (1st method/2nd method): %0.2f", $t2-$t1, $t3-$t2, ($t2-$t1)/($t3-$t2)); ?>
-
Why use a form? Wouldn't it be easier to just have a link with a target of _blank?
-
Did some benchmarking -- my method is about twice as quick: <?php $string1 = 'abcdefghi'; $string2 = 'dbceghifa'; function function1($string1, $string2) { $array1 = str_split($string1); $array2 = str_split($string2); sort($array1); sort($array2); if($array1 == $array2){ return true; }else{ return false; } } function function2($string1, $string2) { $lenStr1 = strlen($string1); $lenStr2 = strlen($string2); if ($lenStr1 != $lenStr2) { // invalid if not the same length return false; } else { for ($i=0; $i<$lenStr1; $i++) { $str1[$i] = substr($string1, $i, 1); } for ($i=0; $i<$lenStr2; $i++) { $str2[$i] = substr($string2, $i, 1); } sort($str1); sort($str2); $str1 = implode('', $str1); $str2 = implode('', $str2); if ($str1 == $str2) { return true; } return false; } } $t1 = microtime(true); for($x=0;$x<=10000;$x++){ function1($string1,$string2); } $t2 = microtime(true); for($x=0;$x<=10000;$x++){ function2($string1,$string2); } $t3 = microtime(true);//end time //now output results printf ("1st Method: %0.8f<br>2nd Method: %0.8f<br>Ratio (1st method/2nd method): %0.2f", $t2-$t1, $t3-$t2, ($t2-$t1)/($t3-$t2)); ?>
-
Well, you could have made your code a lot shorted by converting the strings to arrays, sorting and then checking for equality. Something like: <?php $string1 = 'abc'; $string2 = 'acb'; function strings_contain_same_chars($string1, $string2) { $array1 = str_split($string1); $array2 = str_split($string2); sort($array1); sort($array2); if($array1 == $array2){ return true; }else{ return false; } } if(strings_contain_same_chars($string1,$string2)){ echo 'true'; }else{ echo 'false'; } ?> Wether or not it's any more efficient, im not sure.
-
Doing something to every second result from query
GingerRobot replied to garry's topic in PHP Coding Help
See this FAQ/code snippet. Its just what you're after. -
Sorry, what's the problem? If you're inserting into 3 different tables then you have you to use 3 separate queries.
-
You dont. You reply with it. There's a short time limit on modifications.
-
How about 'Old hat'? Or maybe 'Beaten horse'? Seriously though, what does your site do differently? Perhaps you could base your name on that.
-
Eugh. Same thing is happening in the UK too. Im sick of it. They now wish to record and save every phonecall made, and every email sent, within the UK. Not only is this pointless for stopping terrorism ('we're gonna blow it up you say?', 'yeah', 'tomorrow - be there or be square') and is a complete invasion of privacy, our government doesn't exactly have a great track record when it comes to keeping our personal data secure. I recently had to do a speaking test for my french A-level in which you had to to take a fairly extreme stance on a subject. Mine happened to be CCTV cameras and security. My point of view was that we were half way toward totalitarianism. Now, that was in part to tick said 'clear stance' box, but it's not far from the truth to be honest. We're a tiny country, yet we have over 4 million CCTV cameras. Paranoid? How dare you! We're rapidy become a state that wishes to police every damn aspect of our lives. We can't even be unhealthy anymore. Let alone speak your mind. </political rant> Re: the article; i particularly like the part where it points out that pretty much all routers and firewalls cound be considered illegal. Lock up the whole damn country i say.
-
Sounds like what you need to do is order by the item_id then keep track of the item_id and check it each time the loop is run. If it's the same as last time, dont show the item info. Something like: $currmapquery = "SELECT * FROM lesson_plan LEFT JOIN plan_items ON FIND_IN_SET(plan_items.item_id, lesson_plan.item_id) WHERE plan_items.item_type = 'STA1' ORDER BY item_id"; $currmap = @mysql_query($currmapquery, $connection) or die(mysql_error()); $prev_item = ''; while($row = mysql_fetch_assoc($currmap)) { $plan_id = $row['plan_id']; $item_id = $row['item_id']; $item_name = $row['item_name']; $item_desc = $row['item_desc']; $date = $row['date']; if($prev_item != $item_id){ echo "<br />\n".$item_name.' || '; }else{ echo ', '; } $prev_item = $item_id; echo $plan_id; }
-
Well, if you hadn't set any other cURL options then $data wouldn't have contained the returned string, it would have just been output to the browser. You need to set RETURN_TRANSFER to true if you wish to work with the returned data rather than just outputing it straight away. curl_setopt($ch,CURLOPT_RETURNTRANSFER,TRUE); There's no reason why you should have to write it to a text file. I can only assume that in doing that you added the above option?
-
Replace this bit: $message=nl2br($message); With: $message=bbcode($message);
-
Something like this? <?php $page = file_get_contents('yourfile.html'); $page = preg_replace('|<!-- DONT SHOW START -->.*?<!-- DONT SHOW END -->|is','',$page); echo $page; ?>
-
Change this: return $output; To: return nl2br($output);
-
Yeah. You just didn't echo it: echo bbcode('stuff here [b]bold text[/b]');
-
you mean something like that? - not sure i needed telling twice, thanks anyway though Err, no. Thats a