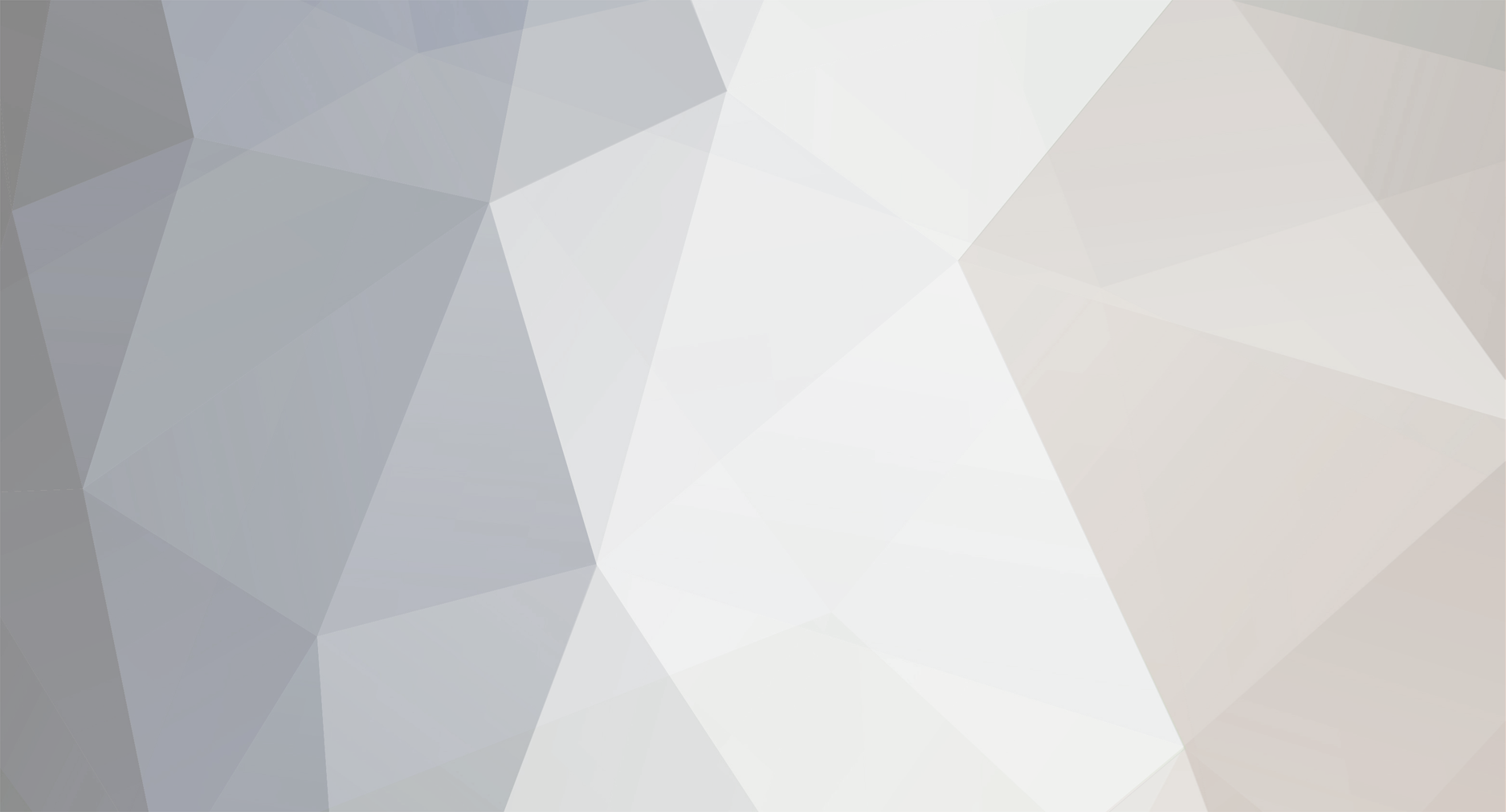
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
Use the basename() function: <?php foreach(glob('path/to/files/*.mp3') as $v ){ echo basename($v).'<br />'; } ?> If you don't want the extension either, then use basename($v,'.mp3')
-
If you're using windows and your PHP version is 5.2.2 or higher, then see: imagegrabwindow()
-
The problem is that you are sending white space to the browser. Anything that is outside of PHP tags will be sent to the browser as plain text. Try: <?php require_once("session.php"); require_once("functions.php"); require_once("databasec.php"); $username = $_POST['username']; $password = $_POST['password']; $hashedpassword = sha1($password); $query = "SELECT *"; $query .= "FROM users "; $query .= "WHERE username = '{$username}' "; $query .= "AND hashedpassword = '{$hashedpassword}' "; $query .= "LIMIT 1"; $result_set = mysql_query($query); confirm_query($result_set); if (mysql_num_rows($result_set) == 1) { $found_user = mysql_fetch_array($result_set); $_SESSION['user_id'] = $found_user['id']; $_SESSION['username'] = $found_user['username']; redirect_to("login.php"); } else { $message = "Username/password incorrect. <br /> Please make sure your caps lock key is off and try again."; echo $message; } ?>
-
You'll probably make life easier for yourself if you have a database. Though it might not be necessary for what you want to do right now, it will make things easier to add in the future. For example, what happens when you want to display your archives month by month? Or what happens when you want to add a search feature? You might want to take a look at a CMS. It could save you lots of time on the coding, though it will take time to get it to loook how you want.
-
If you use the glob() function, you can specify a pattern for the file name to retrieve from a given directory: <?php foreach(glob('path/to/files/*.mp3') as $v ){ echo $v.'<br />'; } ?>
-
As it stands, you'll run into issues with capitalization. You can overcome this by changing this line: if ($usercurrentword == $currentword) To: if (strtolower($usercurrentword) == $currentword) And making sure every word in your dictionary is in lower case. Though you will still have issues with punctuation.
-
Well, if you were to set up your checkboxes like: Blue: <input type="checkbox" name="colours[]" value="Blue" /><br /> Red: <input type="checkbox" name="colours[]" value="Red" /><br /> //etc You could then do something like: <?php $colour = "'".join("','",$colours)."'"; $sql = "SELECT * FROM products WHERE Collection LIKE '$collection' AND color_e IN ($colours) AND...." ?>
-
You need a comma between each field you would like to update. You also appear to have a typo. It should be UPDATE and not UPADTE.
-
The reason why you are getting that error message is that there is output prior to sending the header. You must change headers prior to output. Whilst you can use output buffering, it can often make things messy. Its more likely that you need to restructure your code. Perhaps if you post up what you've got, we can make some suggestions.
-
Sounds like you need to look into some AJAX. The basic idea is that javascript makes a request to another php file 'behind the scenes' then updates the page with the output from that page. I suggest you google for some AJAX tutorials to get you started.
-
No - the modifier on the end of the pattern (thats the bit after the foward slash) - means the pattern is case insensitve. See[http://uk3.php.net/manual/en/reference.pcre.pattern.modifiers.php]the manual [/url]
-
TEST POST FOR QUOTES A.K.A a post count increaser?
-
Take a look at the glob() function. The examples there show you how to cycle through a list of files from a folder.
-
This line: $result = mysql_query("SELECT * FROM private_messages WHERE 'sendto'='$username'", $con); Should be: $result = mysql_query("SELECT * FROM private_messages WHERE sendto='$username'", $con); Weather or not that is the problem, i dont know - i've not read the whole thread, just saw the error. I assume $username is defined in session.php?
-
At present, your sorting may well cause you a problem. If your episode numbers are not all 3 digits, then you have an issue. You would be much better off just storing the episode number in an INT field, and dropping the text 'episode'. Apart from anything else, its constant - so its a waste of storage space. For example, if you were to have the episodes: 101 and 99, then 99 would be sorted AFTER 101 - because the column is being sorted as a string. Now, you may be able to do some regex and just sort by the numbers, but it would be far easier (and the query woudl be quicker) to drop the 'episode' text and use a INT field. As for the rest, its fairly straight forward: <?php //connect to database $sql = "SELECT addyEpisodeNumber,addyLocationTitle,addyTitle FROM Addresses ORDER BY addyEpisodeNumber"; $result = mysql_query($sql) or die(mysql_error()); $x = 0; while($row = mysql_fetch_row($result)){ $array[$x] = $row; $x++; } echo $array[0][2];//the first row's title echo $array[12][0]; //the 12th row's episode number ?>
-
How do I get array elements to populate textboxes?
GingerRobot replied to octacon's topic in PHP Coding Help
Well, you're going to need some javascript to handle the buttons to move things around. But before anyone could help you, we'd need to know a little more about the structure of the text file. You mention moving forwards and backwards. Im assuming this is through the data - in which case, how is is stored? Does the text file contain a long list of values, all comma separated? Or is there 3 values on each line? I would suggest having PHP read the file into an array in javascript. You would then be able to move backward and forward though the data nicely. -
1.) session_start() must be called before any output. Move it right to the top of your script. 2.) As i said earlier, $mysuername is undefined. If its in the session, extract it: <?php session_start(); $myusername = $_SESSION['myusername']; //put the above right at the top of your script. Everything else can follow ?> All the errors are caused by those two problems.
-
There are quite a few applications geared towards turning PHP scrips into stand-alone applications. For example: http://www.priadoblender.com/index.php?layout=main&cslot_1=2 http://www.scriptol.com/apollo.php http://gtk.php.net/ Never having used them, I dont know about the effectiveness and limitations of these.
-
I can't see anything really wrong - are you sure your php setup supports the short tags? Try changing the <? for the full PHP opening tags - <?php You also ought to close the table row inside your while loop - otherwise you're going to be getting a funny looking table. Change this line: echo("<td>" . $row["users.firstname"] . "</td>"); To: echo("<td>" . $row["users.firstname"] . "</td></tr>");
-
Well this kind of thing realy is a job for sessions. A user could quite easily see the ID by taking a glance at the source and it is easily modified too. Sessions shouldn't behave like that - they are user independant, so i suspect something else is going on here. Is that the actual query you were using? There doesn't appear to be a field that you're leaving blank which would correspond to the auto-incremented ID. I take it this wasn't two users on the same computer?
-
[SOLVED] Insert multple records in a table!!!!!
GingerRobot replied to flforlife's topic in PHP Coding Help
The idea wasn't that you add the code Barand provided to the beginning of your script, it was a replacement. The only thing i see missing is that the query that is built doesn't get executed. Try this: <?php if (isset($_POST['btnSub'])) { $sql = "INSERT INTO mytable (place,team, wl, gb, pcage, rs, ra, ags) VALUES\n "; foreach ($_POST['entry'] as $data) { $dataArray[] = "('" . join ("','", $data) . "')"; } $sql .= join (",\n", $dataArray); echo '<pre>', $sql, '</pre>'; // view query mysql_query($sql) or die(mysql_error()); } ?> If you have any problems, try providing us with the output of the above - the query is being printed to the screen to help debug any errors. Also, when you post code/output please place it inside tags. -
The only thing i can see immediately wrong is that $myusername appears to be undefined. Is this stored in a session?
-
What do you mean by a lot of 'repeating variables' ? The variables in the $_POST and $_GET files depend entirely on the data send with the request to the page. Perhaps you could show as an example of what you're doing.
-
I assume you're referring to the $id value? Pass it in the URL and retrieve it from the $_GET array: header("location:page4.php?id=".$_POST['id']); //page 4 echo 'ID: '.$_GET['id']; However, wouldn't it be easier to track the ID with a session? Then you wouldn't have to worry about passing it around all over the place and, assuming the ID isn't supplied by the user, you wouldn't have to worry about it being changed by the user.
-
discomatt: the is_int function will return false on all information from the GET and POST arrays. The function checks the variable's type, which will always be a string from these sources. You can use the ctype_digit() function, however. Though for things like the id of a row in a databases, i prefer to use type casting: $id = (int) $_GET['id']; Edit: Sorry, ignore. This applies with the is_int function, not is_numeric.