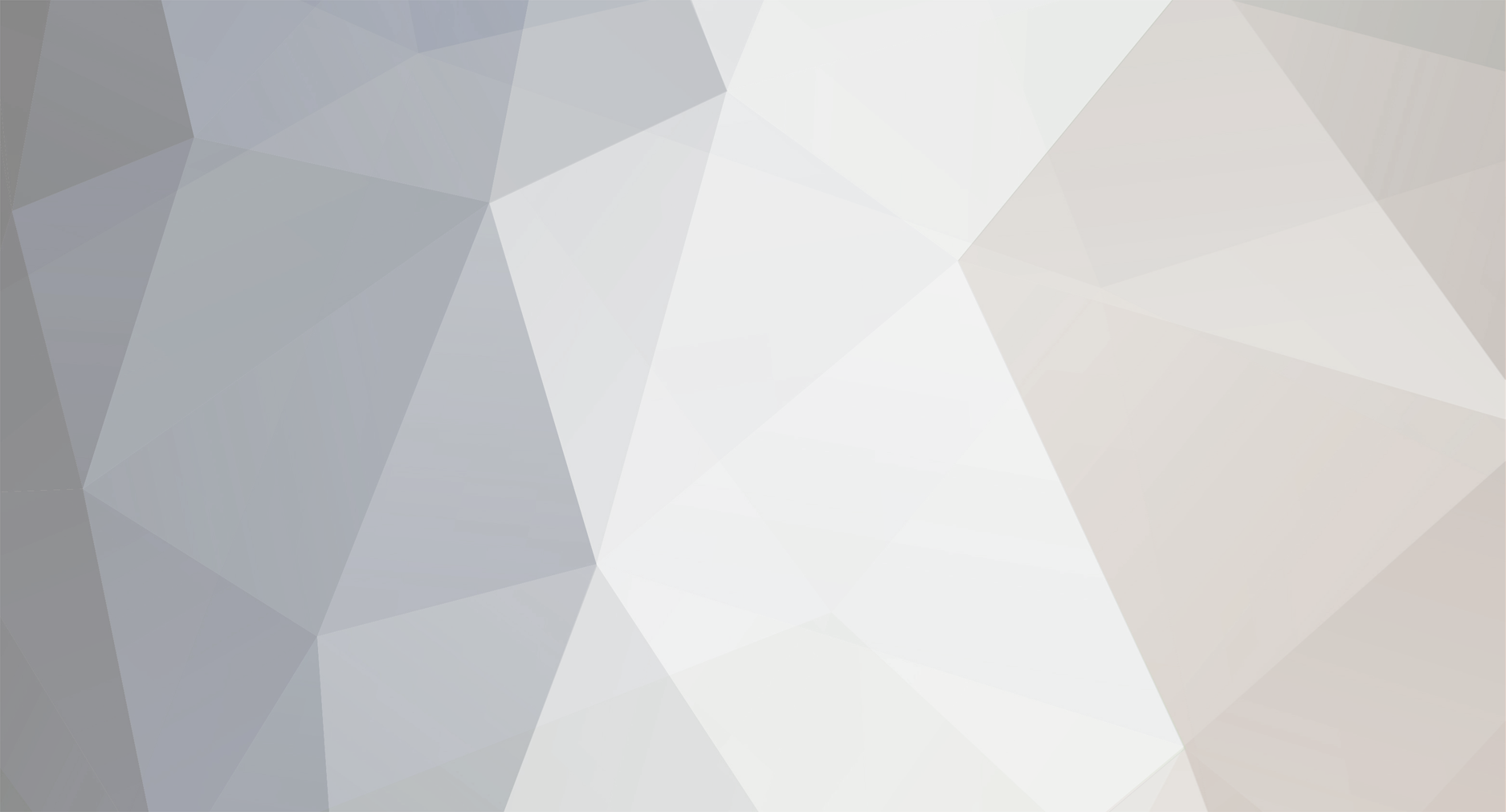
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
[SOLVED] php server configuration different from xampp ?
GingerRobot replied to j33baS's topic in PHP Coding Help
Whenever you are having mysql issues, add an or die statement to the query: $titleresult = mysql_query($titlequery) or die(mysql_error()); This will tell you the error mysql is giving. -
<?php $fields = array('firstname','lastname','position'); $sql = 'SELECT * FROM tbl'; $where = ''; foreach($fields as $v){ if(isset($_POST[$v]) && strlen($_POST[$v]) > 0){ $where.=' '.mysql_real_escape_string($v).' = '.mysql_real_escape_string($_POST[$v]).' AND'; } } if($where != ''){//if there is a where clause $where = substr($where,0,strlen($where)-4);//strip out last AND $sql .= $where; } $result = mysql_query($sql) or die(mysql_error()); while($row = mysql_fetch_assoc($result)){ //echo out results } ?> I should really stop trying to help before i go to bed - i'd make so many less mistakes.
-
If you google, you will find hundreds of registration and login tutorials. I'm sure they can show you. If you get stuck, then come back and tell us what you're stuck with, rather than asking us to make this for you.
-
If you're using PHP 5: <?php $xmlstr = file_get_contents('http://www.xfire.com/xml/sgteversmen/screenshots/'); $xml = new SimpleXMLElement($xmlstr); $games = array(); foreach($xml->screenshot as $v){ $game = (string) $v->game; $games[$game][] = (string) $v->filename; } foreach($games as $k => $v){ echo $k.'<br />'; foreach($v as $v2){ echo '<img src="http://screenshot.xfire.com/screenshot/small/'.$v2.'" />'; } echo '<br /><br />'; } ?> I'll leave you to format
-
Can i see the updated code, including the form code?
-
It should be fine $_SERVER['HTTP_USER_AGENT'] returns 'Mozilla/4.0 (compatible; MSIE 7.0; Windows NT 5.1)' when i'm using IE7
-
The code i gave you should work as is for the select boxes city, and paper (assuming you rename cities to city). I said you'll need to add to that, because you said in your original post that you had three dropdowns. In which case, you need to add an element which is the name of the select box(which should be same as the field name) So, in short, the first $options was correct. I think the best thing is just to try it - see what happens. you'll learn best that way.
-
[SOLVED] Displaying table row data as Columna
GingerRobot replied to telsiin's topic in PHP Coding Help
However, if you insist on carrying on this way, then the following code should help: <?php $sql= "SELECT * FROM Product where CustomerID='2'"; $result = mysql_query($sql) or die(mysql_error()); while($row = mysql_fetch_assoc($result)){ foreach($row as $k => $v){ if($v != 0){ echo $k. ' : '.$v.' <br />'; } } } ?> -
[SOLVED] Displaying table row data as Columna
GingerRobot replied to telsiin's topic in PHP Coding Help
Actually, the link had everything to do with what you are trying to accomplish. You are storing your data in a very bad way. Sure, you might eventually get this particular problem sorted. But you will have great difficult each and every time you have to do something. Thats not to mention that inefficiency of it all. -
[SOLVED] PHP Error, but data goes into table
GingerRobot replied to karatekid36's topic in PHP Coding Help
The issue is with these lines here: $query = "SELECT id FROM calendar WHERE ev_date='$my-$mm-$md' AND ev_locn='$l'"; $result = mysql_query($query); if (mysql_num_rows($result) == 0) { Basically, your select query is failing. mysql_num_rows() is returning false and the above error. The data is being inserted because 0 is equal to false, unless you also check for type (===). So, add an or die statement to your query, and find out what the problem is: $query = "SELECT id FROM calendar WHERE ev_date='$my-$mm-$md' AND ev_locn='$l'"; $result = mysql_query($query) or die(mysql_error().'<br />Query:'.$query); if (mysql_num_rows($result) == 0) { The header errors result from the above mysql error - fix that, and you'll fix the header errors. -
Thats because $rowob contains the number of rows returned by the query, not an array of the returned row. I assume you meant: <?php if (isset($_GET['topic'])) { $boardB = $_GET['topic']; $loolo = 'SELECT * FROM topics WHERE ID = '.$boardB; $lolo = mysql_query($loolo) or die(mysql_error()); $rowob = mysql_fetch_assoc($lolo); $titler = $rowob['Name']; if (isset($titler)){ echo "yup"; }else{ echo "nope"; } echo $boardB; echo $titler; } ?> p.s. You should learn to indent your code - it'll save you a hell of a lot of time and hassle in the future.
-
Two options. 1.) Have php echo the above line 2.) Turn off the short_open_tags setting in your php.ini, and replace all your use of the short tag(<?) with the full tag(<?php) - that'd be my prefered method, since i don't liket the short opening tags because we don't get syntax highlighting when we place the code in short tags on the forum
-
That doesn't affect anything i said. The first part of my response with with regard to how you are searching for keywords, which you may wish to reconsider. The second part gave you the code, and asked you to rename your select fields so they have the same name as the field in the database that they relate to e.g. your field is called city, not cities, so change: <select name="cities"> To: <select name="city">
-
A better method would be to stick all of the unwanted user agents into an array, and loop through: <?php $block = array('MSIE','firefox');//fill out as required $useragent=strtolower($_SERVER['HTTP_USER_AGENT']); foreach($block as $v){ if(strstr($useragent,$v)){ header("Location: http://www.thesite.com/blocked.php"); exit(); } } ?>
-
Ok, well the first thing i suggest you do is to name all or your form elements with the same name as the field they refer to in the database. You can then use code similar to: <?php $fields = array('firstname','lastname','position'); $sql = 'SELECT * FROM tbl'; $where = ''; foreach($fields as $v){ if(isset($_POST[$v] && strlen($_POST[$v]) > 0){ $where.=' '.mysql_real_escape_string($v).' = '.mysql_real_escape_string($_POST[$v]).' AND'; } } if($where != ''){//if there is a where clause $where = substr($where,0,strlen($where)-4);//strip out last AND $sql .= $where; } $result = mysql_query($sql) or die(mysql_error()); while($row = mysql_fetch_assoc($result)){ //echo out results } ?> You'll need to add to the array of fields and change the table name etc, but apart from that, it should all work.
-
Unknown column 'OpponentMAP' in 'field list' error
GingerRobot replied to ondi's topic in MySQL Help
Well, if you run the query: ALTER TABLE KYL_opponents ADD OpponentMAP VARCHAR( 255 ) NOT NULL ; You'll create the field called OpponentMAP. Or you can do it through phpMyAdmin if you have access to it. Wether or not that is going to make everything work, i couldn't tell you - the missing field in the database could just be the start of your problems. Since you're missing at least this field, i assume you didn't create the code yourself? It might be better to contact the person that made this if you're having issues. -
Unknown column 'OpponentMAP' in 'field list' error
GingerRobot replied to ondi's topic in MySQL Help
The error tells you everything: the field, OpponentMAP doesn't exist. Is it supposed to exist? If the error you've posted is generated by the above code, then it would seem that $imgname most contain the string 'MAP', since you dont actually use the field OpponentMAP anywhere, but you add that variable onto the end of 'Opponent'. -
Haha, a PHP answer to a PHP question on a PHP forum? Surely not!
-
It's an Apache setting. Find this line in your http.conf: AddType application/x-httpd-php .php And add a line below, changing .php to the extension you wish to use.
-
The problem could be the sheer volume of queries you are making. If there are over 750 lines in that text file, then that's potentially over 1500 mysql queries - which is quite a few. You could remove all of the unneeded update queries quite easily: run a query to select all the values of simbol from the database, and put them into an array. In your loop, see if $arr[2] is in the array. If its not, skip straight to the insert, rather than doing an update first. Also removes the need for the call to mysql_affected_rows();
-
Eugh, Dreamweaver code and nested tables? What were you thinking? After a closer look, its even more confusing/messy than i thought! So ill just show you what you're missing, and ill leave you to sort out the layout. Basically, you need a loop - to loop through the returned results: <?php $query_web_templates = "SELECT * FROM templates"; $web_templates = mysql_query($query_web_templates, $Epic_Creation) or die(mysql_error()); $totalRows_web_templates = mysql_num_rows($web_templates); while($row_web_templates = mysql_fetch_assoc($web_templates)){ echo 'Name: '.$row_web_templates['name'].'<br />'; echo 'Author: '.$row_web_templates['author'].'<br />'; echo 'Price: '.$row_web_templates['price'].'<br />'; echo 'ID: '.$row_web_templates['ID'].'<br /><br />'; } ?>
-
www.php.net/imagerotate That is, as alin19 points out, assuming you know how much the image needs to be rotated.
-
Rather than trying to change the file association - how about deleting it? It's not a long term solution, but it'd at least show us what the issue is. If you're using XP, go to control panel > folder options > file types. Wait for the field to populate. If you type php, it'll find the relevant one. And then hit delete.
-
One way would be to explode by the pipe, and extract the relevant parts: <?php $lines = file('DATfiles/text.dat'); $firstLine = explode(' ', $lines[0]); $first10words = array_slice($firstLine, 0, 10); $string = implode(' ', $first10words); $parts = explode('|',$string); $message = $parts[1].' : '.$parts[2]; echo $message; ?>