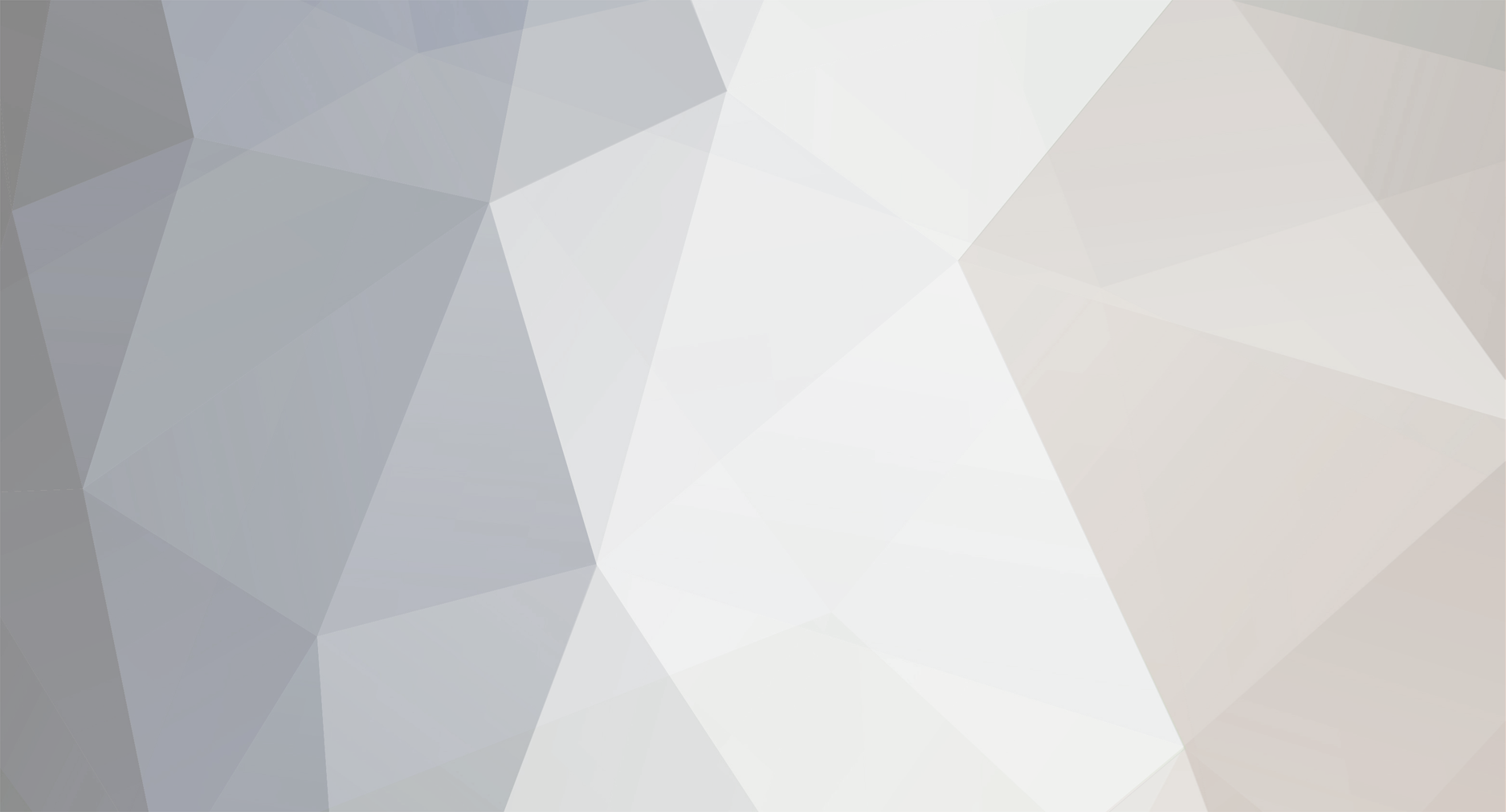
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
[SOLVED] Undefined property: stdClass :: $titel
GingerRobot replied to patrickm's topic in PHP Coding Help
No worries. Always the little things that trip you up. -
Image verification for registration form help
GingerRobot replied to zhinigami's topic in PHP Coding Help
To check to see if the gd library is installed, run a script with this in: <?php phpinfo(); ?> You should find a section titled, GD. You may find that you have an old version running, or perhaps you have don't have FreeType support, which may well be required for the above examples. An alternative way of debugging the captcha scripts is to take out the lines which are similar to: header("Content-type: image/png"); imagepng($im); And add in the following lines, at the top of the script ini_set('display_errors','On'); error_reporting(E_ALL); Then navigate directly to the captcha script in your browser, and see if there are any errors being produced. -
[SOLVED] Taking a picture from another site.
GingerRobot replied to Ryaan's topic in PHP Coding Help
Regular expressions are your friend: <?php $str = '" ... </table> <img class="centeredImage" src="/nothingnice/comics/20080121.gif"> <table width="100%" border="0" cellpadding="0" cellspacing="8"> ... "'; preg_match('|<img.*?class="centeredImage".*?src="(.*?)"|',$str,$matches); echo $matches[1]; ?> This would assume that there is only one image with the class centeredImage in that page. If there's not, then you either need to find something else which identifies that particular picture, or see if you can work out how many there are with that class, and if there are always a fixed number of others before it in the page. -
Search multiple values within one field without using like?
GingerRobot replied to hannibal's topic in MySQL Help
Normalize your database. If people will set multiple locations, you should have a separate table. See here for an introduction to database normalization. -
If you're using php 5: www.php.net/simplexml - i think example 9 is what you're after. Otherwise: www.php.net/xml Must admit, i didn't read the whole post, but it might point you in the right direction.
-
Well, apart from anything else this: if(!is_int($page)){ Will always be true. The is_int() function checks a variable's type. All variables in the $_GET and $_POST array are strings(yes, i suppose you could argue that they can also be arrays, but they are ultimately arrays of strings). The function to use is ctype_digit(), if you want to check a string contains an integer.
-
Well, you can order by RAND(), so if you add this as the second field to order by, it should work ok: $data = mysql_query("SELECT * FROM shirts WHERE active='true' AND approved='1' ORDER BY $field $direction, RAND() LIMIT $from, $max_results") or die(mysql_error());
-
Ah ok, i see what's happening. Firstly, i suggest you add the order by clause: <?php mysql_select_db($database_pedrograncha, $pedrograncha); $sql = "SELECT fotos_datas.nome, fotos.big, fotos.thumb FROM fotos, fotos_datas WHERE fotos_datas.id_data=fotos.id_nome ORDER BY fotos_datas.nome"; $result = mysql_query($sql, $pedrograncha) or die(mysql_error()); ?> Then, we just need to add a little piece in: <?php $curr_name = ''; $cols = 4;//number of columns in picture display $x=0; echo '<table width="100%" border="0" cellspacing="0" cellpadding="10">'; while(list($nome,$big,$thumb) = mysql_fetch_row($result)){ if($curr_name != $nome){//if the name of the row is not the same as the last one, we need to echo out the new name if($curr_name != ''){//if this isn't the first name change, we need to finish off the last row of data'; if($x < $cols && $x != 0){ for($i=$x; $i<$cols;$i++){ echo "<td> </td>"; } } $x = 0; } echo '<tr><td colspan="'.$cols.'" class="style3">'.$nome.'</td></tr>'; $curr_name = $nome; } if($x==0){//new row echo '<tr>'; } echo '<td><img src='.$thumb.'></td>'; if(++$x == $cols){ echo "</tr>"; $x=0; } } if($x < $cols){ for($i=$x; $i<$cols;$i++){ echo "<td> </td>"; } } echo '</tr></table>'; ?> The problem was that if the new name coincided with a new row , we were filling in blank row with blank cells.
-
The $i is defined by the for loop. The idea behind that is that we set it equal to the current value of $x. The value of $i will then be increased by one each time the loop runs, until it reaches the number of columns. It should be correct. As for the problem, do you think you could post up the code you are now using, just to be clear? If you've got the code running on a server i could see, that'd be a useful too.
-
Just had a thought - im quite surprised this has been working perfectly, since the data really ought to be ordered by the name. Perhaps you did that anyway. Explanation? Sure thing. Each row of returned data from the database will contain the name of the user, and the photo. We cycle through this row by row. All of the rows for one person will be together (since we should have been ordering by the name). Therefore, we have a loop which checks to see if the name of the current row is different from the name during the last run of the loop. If it is different, then we show the new name. Either way, we always show the photo - otherwise we'd be missing some. The code is a little more complicated because of the multiple rows. The basic idea behind that is that we will place the images into the same row, until our counter ($x) is equal to the maximum number of images we want in one row ($cols). I've also commented the code some more. <?php $sql = "SELECT table1.name,table2.foto FROM table1,table2 WHERE table1.id=table2.name_id ORDER BY table1.name";//only select the bits you want - more efficient $result = mysql_query($sql) or die(mysql_error()); $curr_name = '';//we need to define $curr_name because we need to know if the loop is being run for the first time //also prevents any undefined variable nitces $cols = 2;//number of columns in picture display $x=0;//this is our counter that we use so we know how many images have been echoed in each row of the table echo '<table>'; while(list($name,$foto) = mysql_fetch_row($result)){ //the list function assigns a name to each of the elements of a given array if($curr_name != $name){//if the name of the row is not the same as the last one, we need to echo out the new name if($curr_name != ''){//if this isn't the first name change, we need to finish off the last row of data if($x < $cols){//we need to check to see if the number of images in the previous row is less than the number //there are supposed to be in a row for($i=$x; $i<$cols;$i++){//we use a for loop to echo out blank cells to fill up the row in the table echo "<td> </td>"; } } $x = 0;//we must reset the counter if a new name has been found } echo '<tr><td colspan="'.$cols.'">'.$name.'</td></tr>';//echo out the new name $curr_name = $name;//assign this name to $curr_name, so we know when this changes } if($x==0){//new row - we create a new row whenever $x is 0 echo '<tr>'; } echo '<td>'.$foto.'</td>';//show the photo if(++$x == $cols){//we must check to see if the current row is now full. If it is, we close the row, and reset $x echo "</tr>"; $x=0; } } if($x < $cols){//once the while loop is finished, we tidy up the table by finishing off the last row if it needs it for($i=$x; $i<$cols;$i++){ echo "<td> </td>"; } } echo '</tr></table>';//close the table ?> Between the comments and the above description, i hope that helps you understand. If not, tell me which bits you're not sure of, and ill try and explain a bit more. If you do now understand, can you mark the topic as solved?
-
Whoops. Try: <?php $sql = "SELECT table1.name,table2.foto FROM table1,table2 WHERE table1.id=table2.name_id";//only select the bits you want - more efficient $result = mysql_query($sql) or die(mysql_error()); $curr_name = ''; $cols = 2;//number of columns in picture display $x=0; echo '<table>'; while(list($name,$foto) = mysql_fetch_row($result)){ if($curr_name != $name){//if the name of the row is not the same as the last one, we need to echo out the new name if($curr_name != ''){//if this isn't the first name change, we need to finish off the last row of data'; if($x < $cols){ for($i=$x; $i<$cols;$i++){ echo "<td> </td>"; } } $x = 0; } echo '<tr><td colspan="'.$cols.'">'.$name.'</td></tr>'; $curr_name = $name; } if($x==0){//new row echo '<tr>'; } echo '<td>'.$foto.'</td>'; if(++$x == $cols){ echo "</tr>"; $x=0; } } if($x < $cols){ for($i=$x; $i<$cols;$i++){ echo "<td> </td>"; } } echo '</tr></table>'; ?>
-
Yes, you can have it all on one page. You'll need to employ some ajax to do it, however. See this FAQ for something which is at least a little similar: http://www.phpfreaks.com/forums/index.php/topic,155984.0.html It's done without a database, and its all on one page, so you might find it a little confusing. However, if might give you the general idea. Also, see www.ajaxfreaks.com/tutorials for some basic tutorials on AJAX.
-
[SOLVED] Populating individual database rows.
GingerRobot replied to Siggles's topic in PHP Coding Help
So $gottenid is defined inside a different while loop? If so, then that is why it is only even updating the last id. Once that loop has finished, $gottenid will obviously contain the last id. Surely it should be defined in the loop that is doing the updating. -
[SOLVED] Populating individual database rows.
GingerRobot replied to Siggles's topic in PHP Coding Help
1.) $gottenid looks like its undefined. 2.) you need to assign the returned value of the calc_score function to $sum, otherwise this is undefined too. -
Give this a go: <?php $sql = "SELECT table1.name,table2.foto FROM table1,table2 WHERE table1.id=table2.name_id";//only select the bits you want - more efficient $result = mysql_query($sql) or die(mysql_error()); $curr_name = ''; $cols = 2;//number of columns in picture display $x=0; echo '<table>'; while(list($name,$foto) = mysql_fetch_row($result)){ if($curr_name != $name){//if the name of the row is not the same as the last one, we need to echo out the new name if($curr_name != ''){//if this isn't the first name change, we need to finish off the last row of data'; if($i < $max_columns){ for($i=$x; $i<$cols;$i++){ echo "<td> </td>"; } } $x = 0; } echo '<tr><td colspan="'.$cols.'">'.$name.'</td></tr>'; $curr_name = $name; } if($x==0){//new row echo '<tr>'; } echo '<td>'.$foto.'</td>'; if(++$x == $cols){ echo "</tr>"; $x=0; } } if($x < $cols){ for($i=$x; $i<$cols;$i++){ echo "<td> </td>"; } } echo '</tr></table>'; ?>
-
[SOLVED] Undefined property: stdClass :: $titel
GingerRobot replied to patrickm's topic in PHP Coding Help
The error message would suggest that there is no field called 'titel' in your database. Perhaps a typo? -
[SOLVED] PHP MySQL populated drop down box issue.
GingerRobot replied to $username's topic in PHP Coding Help
In this line: $Owners = "$Contactfname, $Contactlname"; You have a space after the comma. In this line: $selected = ($row150['UserFirstName']","$row150['UserLastName']==$Owners )?" selected=\"selected\"":""; You dont. Personally i'd use an AND operator anyway: $selected = ($row150['UserFirstName'] == $Contactfname && $row150['UserLastName']==$Contactlname )?" selected=\"selected\"":""; -
Use bb tags rather than allowing html tags. Much safer.
-
Sorry, it should have been 'greater than' rather than 'greater than or equal to' in the query: <?php $sql="SELECT ID FROM art_product WHERE is_active=1 AND collection_ID=$cid AND ID > $pid ORDER BY ID LIMIT 1"; $result = mysql_query($sql) or die(mysql_error());//i assume $pid refers to the ID field? if(mysql_num_rows($result) > 0){//we are not on the last item $nextid = mysql_result($result,0); }else{//current item is the last, we require the first $sql="SELECT ID FROM art_product WHERE is_active=1 AND collection_ID=$cid ORDER BY ID LIMIT 1"; $result = mysql_query($sql) or die(mysql_error()); $nextid = mysql_result($result,0); } //pass $nextid in the url as the new pid ?>
-
Oh, sorry - ignore my previous post. I misunderstood.
-
Can't say that i know an awful lot about it, but isn't this where full text searching comes in?
-
Question 1.) No. Question 2.) You'll need to join the tables. Lets say you have a field called id in both, which the join can be performed on. Lets also say downloads and installs are in table1 and views and comments are in table2: SELECT (table1.downloads+table1.installs+table2.views*0.5+table2.comments) as total, table1.id as id FROM table1,table2 WHERE table1.id=table2.id ORDER BY total DESC
-
recursive function woes (reading multi-dim array)
GingerRobot replied to nikefido's topic in PHP Coding Help
I think the main problem was you weren't apending the returned from the function to $output when it was being called from itself. I rewrote to tidy it up a lil bit: <?php function arrayToXml($array,$start=TRUE){ if($start === TRUE){ $output = "<structure>\n"; } foreach($array as $v){ if(is_array($v)){ $output .= "<directory>\n".arrayToXml($v,FALSE)."</directory>\n"; }else{ $output .= '<file>: '.$v."</file>\n"; } } if($start === TRUE){ $output .= "</structure>\n"; return htmlentities($output);//only apply htmlentities on last return } return $output; } echo arrayToXml($array); ?> -
The first thing i notice is that when i click any of the thumbnails on the page you listed, there is no cid passed in the URL, only the pid. However, the way i would do it is this: <?php $sql="SELECT ID FROM art_product WHERE is_active=1 AND collection_ID=$cid AND ID >= $pid ORDER BY ID LIMIT 1"; $result = mysql_query($sql) or die(mysql_error());//i assume $pid refers to the ID field? if(mysql_num_rows($result) > 0){//we are not on the last item $nextid = mysql_result($result,0); }else{//current item is the last, we require the first $sql="SELECT ID FROM art_product WHERE is_active=1 AND collection_ID=$cid ORDER BY ID LIMIT 1"; $result = mysql_query($sql) or die(mysql_error()); $nextid = mysql_result($result,0); } //pass $nextid in the url as the new pid ?>
-
Even with the above, if a user were to enter 'metalica ban' the query would not find rows matching 'metalica'. One way around this is to explode the search term by the space, and search the database to see if any of the words match. However, that would then lead you into issues of relevant results. You may wish to look into full text searching.