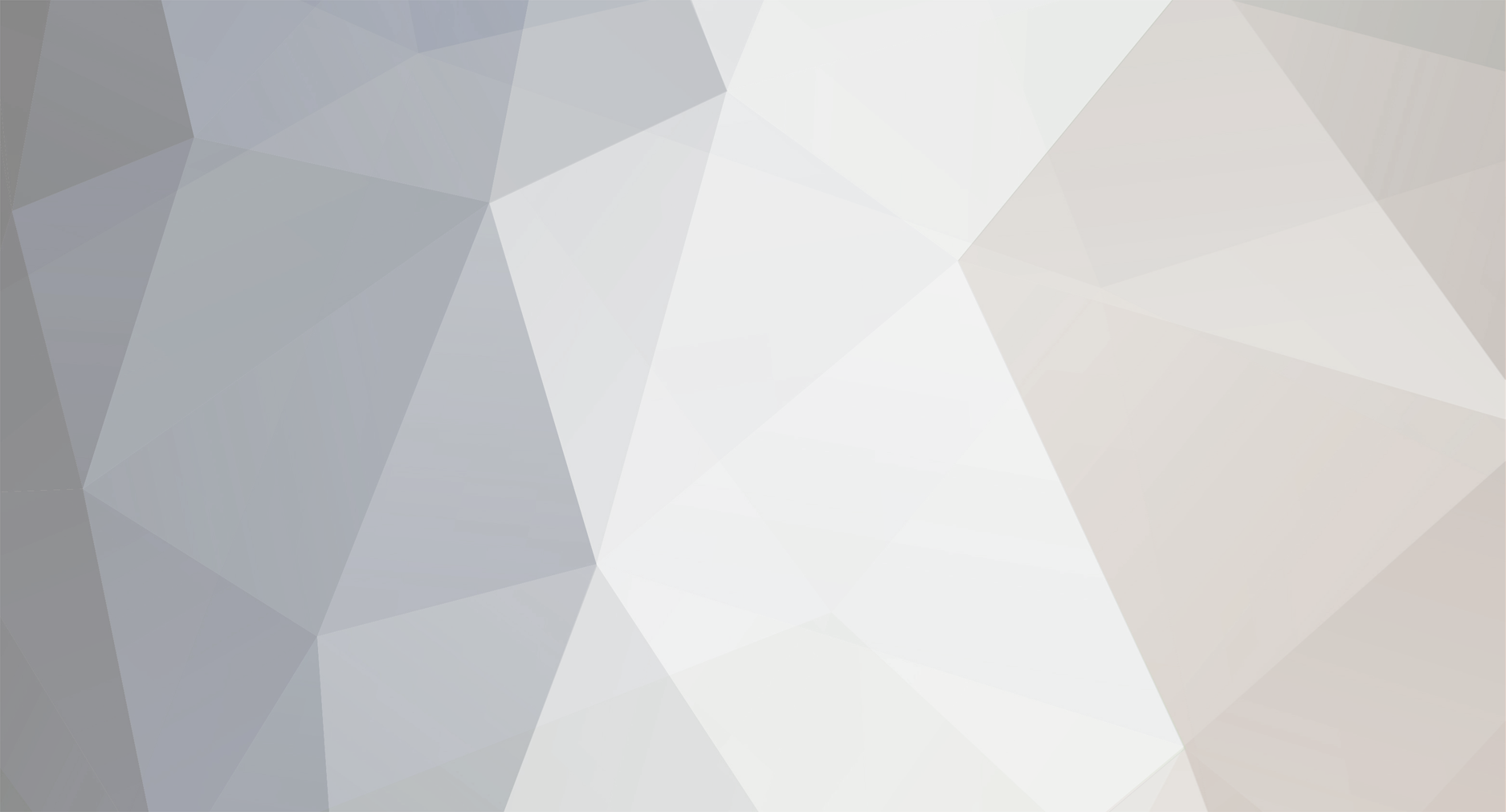
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
Okay. Well, the first thing i notice is that you're using keywords to search. Are you aware of how the mysql search works? If a user were to put in say 'keyword1 keyword2' as their keywords, then , your current query would only return rows where the description was exactly 'keyword1 keyword2'. So, if a row had the descrition 'some words keyword1 keyword2', then it would not be returned. For this kind of thing, you want to be at least using the LIKE operator, with wildcards. So, for example, your query would be: $qry = "SELECT * FROM table WHERE description LIKE '%$keywords%'"; A % sign is the wildcard for any character, any number of times An _ can be used to signify any character, but just once Using the above example, this would return all rows where 'keyword1 keyword2' are contained anywhere within the description. However, it would still not return something like: 'keyword1 somewords keyword2'. If that is an issue, you have a few options. Firstly, you could place the % sign between the keywords. However, this would still only help you if all the words were contained in the description. Or, you could search word by word, using an OR clause. Finally, the best results would be returned by using a full text search, which is more complex - i suggest you google. So, you'll need to decide what quality of search results you're happy with. Back to the question: The first thing to do is rename your select fields to use the name of the field in the database they relate to. For instance, cities should be renamed to city. You could then use this code: <?php $options = array('paper','city');//define an array of all the fields that select boxes exist for $qry = "SELECT * FROM table WHERE description LIKE '%$keywords%'"; foreach($options as $v){ if($_POST[$v] != 'all'){ $qry.= ' AND '.mysql_real_escape_string($v).' = '.mysql_real_escape_string($_POST[$v]); } } echo $qry; ?> Hope that helps.
-
I've never worked with pdfs from PHP. But since i know you can create them, it would seem logical that you could open and modify them. You may have to do some digging, but this would be worth a look: www.php.net/pdf
-
Extracting only text within a certain tag
GingerRobot replied to denhamd2's topic in PHP Coding Help
This is a bit neater: <?php $schedule = 'Test<span>Some Text</span> Text not in a span tag <span> Bit more text here inside a span</span> Blah Blah Blah'; preg_match_all('|<span>(.*?)</span>|',$schedule,$matches);//put anything between span tags into an array $schedule = implode('',$matches[0]);//join them together echo $schedule; ?> -
You'd be better off using the the id of the product as the key of the array, and the quantity required as the element. You could then use a foreach loop and do whatever you need to do.
-
Extracting only text within a certain tag
GingerRobot replied to denhamd2's topic in PHP Coding Help
How's about: <?php $schedule = 'Test<span>Some Text</span> Text not in a span tag <span> Bit more text here inside a span</span> Blah Blah Blah'; $schedule = preg_replace('|</span>(.*?)<span>|','</span><span>',$schedule);//strip out anything between span tags $schedule = strstr($schedule,'<span>');//take out anything before the first span pag $rev = strrev($schedule);//reverse string, to find 'first' occurrance of closing span tag $rev = strstr($rev,strrev('</span>'));//take out anything before it $schedule = strrev($rev); echo $schedule ?> -
Trying to remove a space from a data call -- help?
GingerRobot replied to illusiveone's topic in PHP Coding Help
Ah, i got it. Change your code to: <?php echo $data['fname']."'"; if (substr($data['fname'],-1)!= "s"){ echo "s"; } ?> The space being generated wasn't being echoed by PHP, it was in the HTML. That happened because there was a space between closing the first bit of PHP and opening the second. -
You just need a return statement. A return statement literally returns control of the script back to where the function was called.
-
Me too. Interesting information there. After a bit more digging, it looks like i was wrong; Java is indeed used as the starter language at most of the universities i applied too. Having said that, the first language you use at Bristol university is C and that's looking like the place im going to end up.
-
Hmm, is that the case throughout universities in the US, including the better ones? I've just applied for Computer Science in the UK, and it seems that most still teach C in the first year, although perhaps alongside Java. Quite a lot of them use Haskell as a starter language too - apparently it's good for teaching. Anyone happen to have any experience of that?
-
Trying to remove a space from a data call -- help?
GingerRobot replied to illusiveone's topic in PHP Coding Help
Firstly, welcome to the forums! I can't see anything wrong with that (apart from the general messy code). I can only assume that it is the the element of the array, $data['fname'], that has a space on the end of it. You could test that by comparing the length of the string and the string itself. <?php echo $data['fname'].' has a length of: '.strlen($data['fname']).'<br />'; ?> So, for example, if the first name is Ben, and the length of the string is 4, we know we have a rogue space in there. p.s. Try to remember to use the tags for your PHP code (and, where possible, full opening tags - we then get syntax hightlighting making it much easier to read) -
The in_array() function expects two parameters, you are only providing one. Not entirely sure what you're trying to achieve, so im not sure of the fix.
-
No problem. Can you mark this as solved please?
-
Ok, well this would do it based on a date: <?php $d = '29'; $m = '01'; $y = '2008'; $diff = strtotime("$m/$d/$y") - strtotime("$m/01/$y"); $weeks_diff = floor($diff/(60*60*24*7))+1;//rounding down and adding 1 to allow for the date being the 1st of the month //rounding up would return 0 echo $weeks_diff; ?> So we just need to use date() to generate the date: <?php $d = date('d',time()-60*60*24*14); $m = date('m',time()-60*60*24*14); $y = date('Y',time()-60*60*24*14); $diff = strtotime("$m/$d/$y") - strtotime("$m/01/$y"); $weeks_diff = floor($diff/(60*60*24*7))+1; echo $weeks_diff; ?>
-
Personally i wouldn't bother using strtotime() - i'd just take away the number of seconds in two weeks from the current time: <?php $weekNum = date("W",time()-60*60*24*14); echo $weekNum; ?> Also, you don't need all that to get the current week number. This would suffice: <?php $weekNum = date("W"); ?> Edit: misread this - didn't realise you were asking for the week of the month
-
It's certainly not possible with PHP. PHP is a server side language - by the time the HTML is displayed in the browser, the PHP code has been executed. Though im no javascript expert, I would imagine it wouldn't be too difficult to create using javascript, using the onClick and onKeyPress events. Perhaps if you head over to the javascript forum, someone with a bit more javascript knowledge might be able to point you in the right direction.
-
Well, im a little confused with the code you've attempted, and the problem. Im not sure why there are so many string functions, when we're dealing with numbers. However, if the problem is as btherl stated, then this might give a solution: <?php function numbers($start,$finish,$n,$dps=3){ if($n <= 1 || $start >= $finish){ return false; } $increment = ($finish - $start)/($n-1); //echo $increment; uncomment this line $array = array(); while($start <= $finish){ $array[] = number_format($start,$dps); $start += $increment; } if(count($array) < $n){ $array[] = $finish;//comment out this line } return $array; } echo '<pre>'.print_r(numbers(0,157,516),1).'</pre>'; ?> Note: There's always going to be a slight issue here. With such large numbers, and the rounding that occurs, the penultimate number generated + $increment is actually a touch larger than the value for $final. That was why i added that last if statement. As an example, using 0-157 in 516 steps, the last number generated is 156.695145631069 (to 12 d.p.), and the number we are incrementing by is 0.304854368932. Add them together, and you get 157.000000000001. So the last number (157) is not added to the array. If you follow the comments and uncomment and comment where required, you'll see what i mean.
-
I've no idea. What does it do? What doesn't it do? What's it supposed to do?
-
[SOLVED] cURL remote server, no browser PLEASE HELP
GingerRobot replied to candeman's topic in PHP Coding Help
That topic IS this topic. If i view my last posts, the title of the topic is different, but it links to this one. I guess SMF just had a funny 5 minutes. -
[SOLVED] cURL remote server, no browser PLEASE HELP
GingerRobot replied to candeman's topic in PHP Coding Help
Yes, i know - i've not gone completely insane, i've just absolutely no idea what happened here. When i posted, my reply was the first - and the question being asked was rather different. I came back later and checked the topic only to find that lots of people had posted before me and the question had changed. All very bizarre. -
Tell your client they're a pain Anyways, i'd advise you to keep the bits of information separate in the database, and only join them together to form the order ID when you output the data. So, you'll have 3 columns: username,date,order_today (or whatever you prefer). You can then do something like: <?php $sql = "SELECT MAX(order_today) FROM tbl WHERE username = '$user' AND `date` = DATE(NOW())"; $result = mysql_query($sql);; $order_today = mysql_result($sql,0) + 1; $sql = "INSERT INTO tbl (username,`date`,order_today) VALUES ('$user',DATE(NOW()),$order_today)"; mysql_query($sql); ?> Edit: PFMaBiSmAd has a valid point. I've assumed you're using a database.
-
Is there any particular reason why you're giving your orders and order ID like this? Things would be much simpler if you just used a number.
-
Yes, you need to use wildcards, and for that, you'll need the LIKE comparison: $query_Student = "SELECT Name, `Class` FROM skill_cards WHERE skill_cards.`Class` LIKE 'Bob%'"; This will return rows where class begins with Bob. If you want to match records where Bob occurs anywhere within the string, place a % character at the start of the string too. The % wildcard matches any character any number of times. You can also use the _ wildcard, which matches any character, but just once.
-
[SOLVED] cURL remote server, no browser PLEASE HELP
GingerRobot replied to candeman's topic in PHP Coding Help
If i understand your question correctly, you can use cURL. See here -
You can explode() the data returned from cURL (making sure you have the curl option, RETURNTRANSFER, set to true, so the file is returned to a string, rather than being echoed). That will generate the array of lines for you. e.g.: <?php $output = curl_exec($ch); $lines = explode("\n",$output); for($x=0;$x<3;$x++){ echo $lines[$x]."<br />\n"; } ?> However, you may find it easier to use the file() function, which will return an array of the lines in the file.
-
Whats wrong with this is that your inserting inside your for loop. If you only want one entry, the database insert needs to be done outside of the loop