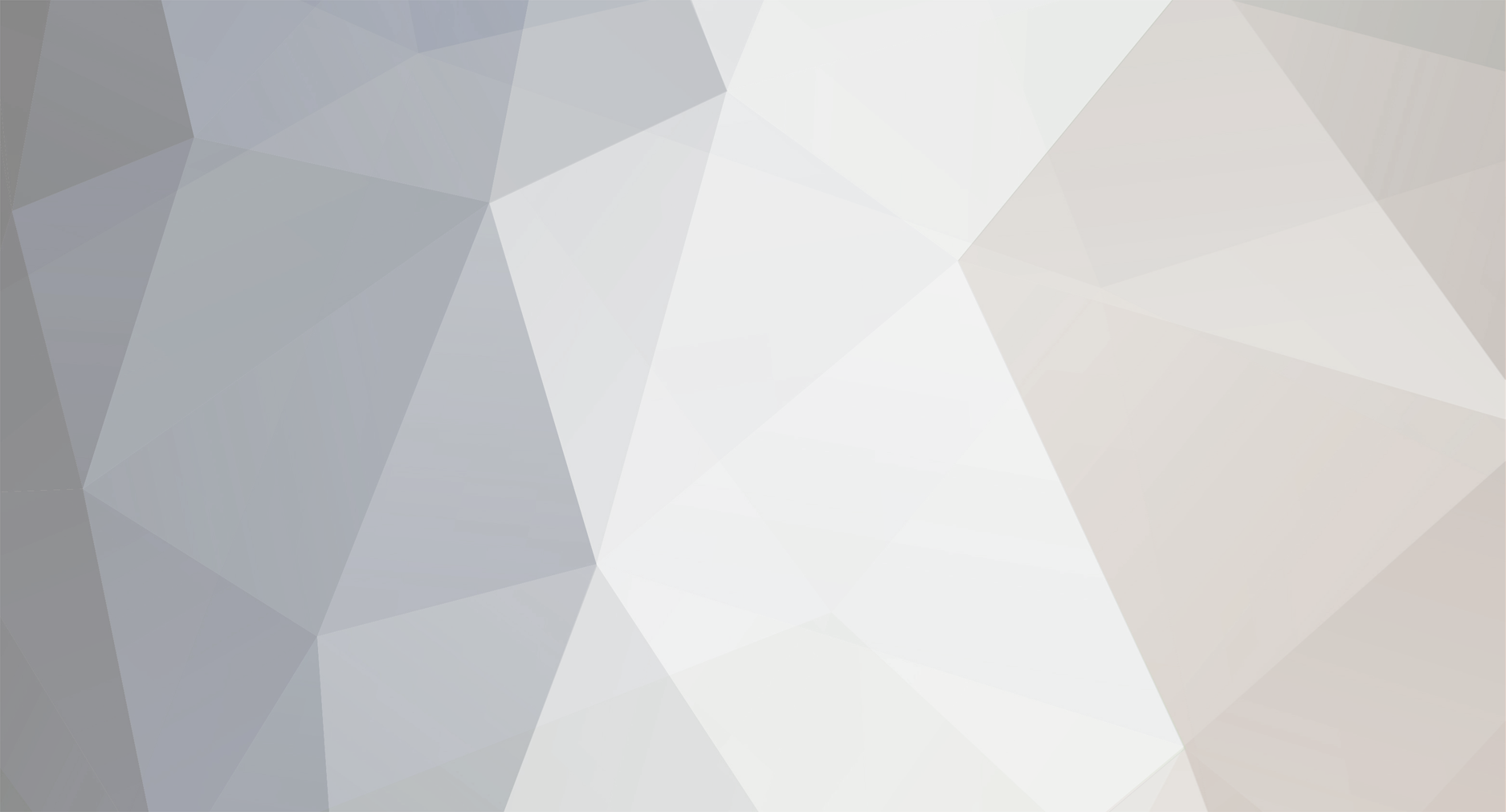
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
using the date function to find last week start and end.
GingerRobot replied to eon201's topic in PHP Coding Help
I think you'll find thats what my script does: Last Monday: 2007-11-19 Last Sunday: 2007-11-25 If you wanted to test it further, then add some time just after the call to the time() function: <?php $offset = 60*60*24*7;//pretend 'now' is one week in the future echo 'Last Monday: '.date('Y-m-d',strtotime('last monday',time()+$offset-60*60*24*6)).'<br />'; echo 'Last Sunday: '.date('Y-m-d',strtotime('next sunday',time()+$offset-60*60*24*); ?> Produces: Last Monday: 2007-11-26 Last Sunday: 2007-12-02 -
using the date function to find last week start and end.
GingerRobot replied to eon201's topic in PHP Coding Help
If i understand what you want, this should do: <?php echo 'Last Monday: '.date('Y-m-d',strtotime('last monday',time()-60*60*24*6)).'<br />'; echo 'Last Sunday: '.date('Y-m-d',strtotime('next sunday',time()-60*60*24*); ?> We use the strtotime function to find the two dates. You may wonder why it says next sunday. That is because we set the current time for the strtotime as a week ago. Otherwise, last sunday is one day before last monday. Which i dont think is what you want. You'll then we wondering why we actually only go back 6 days for last monday, or 8 days for next sunday. That is because we need to be careful of the situation where it is monday or sunday today. If it is monday today, and we go back 7 days, then last monday is in fact two weeks ago. Similarly, if today is sunday, and we go back a week, next sunday is in fact today. -
It could be done, since you can use the GD library to find the color of each individual pixel: imagecolorat It woudln't be pretty, but i would imagine that you could, at least theoretically, run through all the pixels looking for the that grey colour, and try to find patterns which you could associate with letters. It would indeed take a fair bit of effort, and wether or not the results would be entirely satisfactory, im not sure. The script would probably also take a little while to run. In your favour is the fact that the font is always the same. If you could identify the font, you could create some images with the same font and colour to analyse before you start work.
-
Why would you want to? I think you need to reconsider your approach. This is not how a database is designed to work. To take but one example. What happens when you want to gather information about many different users? You'd have to perform lots of separate queries and UNION the results. That would be VERY slow.
-
Yes. You use the $_GET array. For example, this might be your display of results: <?php $sql = mysql_query("SELECT `id`,`title` FROM `yourtable`"); while($row = mysql_fetch_assoc($sql)){ echo '<a href="info.php?id='.$row['id'].'">'.$row['title'].'</a><br />'; } ?> And this might by info.php: <?php if(isset($_GET['id'])){ $id = (int) $_GET['id']; //type casting - we only want integers for our ID. This would prevent any SQL injection attack }else{ echo 'No ID selected!'; exit; } $sql = mysql_query("SELECT * FROM `yourtable` WHERE `id`=$id") or die(mysql_error()); $row = mysql_fetch_assoc($sql); //print out the information ?>
-
Help needed with an array loop... please
GingerRobot replied to Solarpitch's topic in PHP Coding Help
You don't start a foreach loop in the same was as a for loop - it wouldn't make sense. Try: foreach($gethistory as $k => $v) { echo "menu1[".$k."]='<a href='http://www.johnlockes.com/articles.php?article_id=".$v[0]."'>".$v[2]."</a>'"; } Of course, you could always just leave it as a for loop. -
Actually, the percentages you gave are for if you DO care which order they come in. If you choose 3 cards, the probability of any one of them being rare is 24.3%: 0.1*0.9*0.9 (rare, not rare, not rare)+ 0.9*0.1*0.9 (not rare, rare, not rare)+ 0.9*0.9*.0.1 (not rare, not rare, rare) =0.243*100 = 24.3% Or in terms of a binomial probability: 3 nCr 1 * 0.1^1 * 0.9^2 = 0.243 *100 = 24.3% Anywho, dewey:witt: we need exactly what you want to find the probabiliy of before we can give you a proper answer
-
Yes, you can and should do it. And you can go as deep as you like. Though it might get a bit messy after a while.
-
[SOLVED] Simple Blog type functionality
GingerRobot replied to phatgreenbuds's topic in PHP Coding Help
Not with the code you posted it doesn't. When you submit the form, all it does is update the database. Nothing else. Thats assuming the code you postes is for blogedit.php By the way, i missed something out. I forgot to add the id in the url of the form's action: <?php if(isset($_GET['id'])){ $id = $_GET['id']; if(isset($_POST['update'])){ $text = $_POST['Entry']; mysql_query("UPDATE blogger SET blogtext = '$text' WHERE id = $id") or die(mysql_error());//i hope there's no need for the limit clause, since id should be a unique field } $sql = mysql_query("SELECT * FROM blogger WHERE id=$id") or die(mysql_error()); confirm_query($target); // no idea what this does, but ill leave it in $row = mysql_fetch_assoc($sql); //no need for a while statement - we're only working with one row $userx = $row['user']; $blogtextx = $row['blogtext']; $datex = $row['date']; ?> <form action="blogedit.php?id=<?php echo $id; ?>" method="post"> Date: <br><input name="Date" type="text" value="<?php echo $datex; ?>"><br> <br> Name: <br><input name="Name" type="text" value="<?php echo $userx; ?>"><br> <br> Entry: <br><textarea name="Entry" cols="100" rows="10" wrap="PHYSICAL"><?php echo $blogtextx; ?></textarea><br> <br> <input type="submit" name="update" value="update"> </form> <br><br> <?php }else{ echo 'No ID selected'; } ?> You can't do the error checking AFTER you've queried the database. It kinda defeats the object of the error checking. -
I think your issue is just with the use of strtotime. It does not accept english formatted dates. You must use mm/dd/yy and not dd/mm/yy. Switch it around and give it a try.
-
[SOLVED] Simple Blog type functionality
GingerRobot replied to phatgreenbuds's topic in PHP Coding Help
Well, firstly there's a syntax error in your query. It should be: mysql_query("UPDATE blogger SET blogtext = '$new' WHERE id = '$selected' LIMIT 1"); You don't use single quotes around table and column names, and there's no need to specify the table name since we're only using one table. Second, $selected is undefined. Whilst it is defined when the page originally loads, this value isn't passed along when you submit the form. Thirdly, you need to restructure your code otherwise the changes wont be shown at first, since the update gets done after the form is displayed. Try: <?php if(isset($_GET['id'])){ $id = $_GET['id']; if(isset($_POST['update'])){ $text = $_POST['Entry']; mysql_query("UPDATE blogger SET blogtext = '$text' WHERE id = $id") or die(mysql_error());//i hope there's no need for the limit clause, since id should be a unique field } $sql = mysql_query("SELECT * FROM blogger WHERE id=$id") or die(mysql_error()); confirm_query($target); // no idea what this does, but ill leave it in $row = mysql_fetch_assoc($sql); //no need for a while statement - we're only working with one row $userx = $row['user']; $blogtextx = $row['blogtext']; $datex = $row['date']; ?> <form action="blogedit.php" method="post"> Date: <br><input name="Date" type="text" value="<?php echo $datex; ?>"><br> <br> Name: <br><input name="Name" type="text" value="<?php echo $userx; ?>"><br> <br> Entry: <br><textarea name="Entry" cols="100" rows="10" wrap="PHYSICAL"><?php echo $blogtextx; ?></textarea><br> <br> <input type="submit" name="update" value="update"> </form> <br><br> <?php }else{ echo 'No ID selected'; } ?> You'll notice i put the code to display the form inside the if statement that checks if $_GET['id'] was set, since we only want to show the edit form if this is the case. Also, you'll want to add some error checking into that. -
You're missing a double quote when you define the charset: change: <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> to: <meta http-equiv="Content-Type" content="text/html; charset="utf-8" />
-
Does $renamedimage contain the image name and extension? Is that the image you trying to load? If so, you'll need to use one of the imagecreatefromgif/jpeg/png functions depending on the type. Once yo've done that, you can then use the other gd functions.
-
[SOLVED] removing text between two characters in a string
GingerRobot replied to sasquatch69's topic in PHP Coding Help
Did you try my suggestion? It uses regular expressions and would work with any times. -
You have to. You have to store the information somewhere. An array is not something which keeps information throughout many requests. If it did, it would be permanent storage. You either have to store the mesages in a database or a text file.
-
[SOLVED] removing text between two characters in a string
GingerRobot replied to sasquatch69's topic in PHP Coding Help
Are these the only two times in the data? If so, something like this might work for you: <?php $str = "other text <TD>12:00PM - 2:00PM</TD> other text"; $str = preg_replace('% - [0-9]{1,2}:[0-9]{2}(A|P)M%','',$str); echo $str; ?> -
Barand: Your code wouldn't display the right thing for something like 9.8 which should be rounded to 10 first. I think you do need to round to a half first: <?php function toStars ($n) { $n = round($n*2,0)/2; $star = floor($n/2); $half = $n - ($star*2) >= 0.5 ? 1 : 0; $blank = 5 - ($star + $half); return str_repeat("<img src='star.gif'>", $star) . str_repeat("<img src='halfstar.gif'>", $half) . str_repeat("<img src='blank.gif'>", $blank); } echo toStars(0.98).'<br />'; // 1 half, 4 blank echo toStars(9.62).'<br />'; // 4 stars, 1 half echo toStars(9.;// 5 stars ?> Your solution was much neater however - there's a surprise. Not!
-
No idea. It'll be an html/css issue.
-
Only if you happen to have a file named test.txt with the text 'This is a test file with test text.' in the same folder as the php script. Personally, i dont
-
convert 1,2,3,4 to 1st, 2nd, 3rd, 4th etc
GingerRobot replied to delphi123's topic in PHP Coding Help
What does this actually do? It makes use of the date function's format to add the suffix onto the end of the date. The format jS is the day of the month, followed by the relevant suffix. The second parameter is to set a particular date for this. It uses strtotime to create the unix timestamp based on a date, which is an arbitary year and month (though, of course, you need to use a month with 31 days!) followed by the day of the month, which is the number we want. Quite a clever approach if you only need to go up to 31. -
Thats about the only way of doing it. Of course, if you want to show the error message on any page when the website is offline, then you'd be better off storing this: <?php //connect to datase $sql = "SELECT `website_online` FROM `yourtable`"; $result = mysql_query($sql) or die(mysql_error()); $row = mysql_fetch_row($result); if($row[0] == 'OFFLINE'){ echo 'Website is currently offline'; exit;//stop running the rest of the script } ?> In a file, and requiring it in every page.
-
Something like: <?php function to_stars($num){ $half_star = false; $stars_echoed = 0; $rating = round($num*2,0)/2; //achieves rounding to a half $full_stars = floor($rating/2); //the number of full stars if($rating % 2 != 0){//if we have a half star $half_star = true; } while($full_stars > 0){//output full stars echo '<img src="path/to/your/full/star" />'; $full_stars-= 1; $stars_echoed++; } if($half_star === true){//output half star if needed echo '<img src="path/to/your/half/star" />'; $stars_echoed++; } while($stars_echoed < 5){//if we've output less than 5 stars, output the remaining as empty stars echo '<img src="path/to/your/empty/star" />'; $stars_echoed++; } } to_stars(0.98);//half a star to_stars(9.62);//4 and a half stars; ?>
-
If you store the function's result to a variable first, you can do it: function getFields() { return array("zero","One","Two","Three"); } $array = getFields(); echo $array[1];
-
The number of letters in each word is the number at each successive decimel point: que: 3 . j: 1 aime: 4 etc Clever!
-
[SOLVED] Learning Functions. Don't understand example.
GingerRobot replied to foo's topic in PHP Coding Help
Yeah, thats right. In these examples, the output would be with a comma between each part of the date. Yes, that is indeed an example of that. The reason why you need to use the mktime function is that the second parameter for the date() function is an integer. The mktime function takes the various elements that compose a date and time, and returns an integer which is the number of seconds since unix epoch. Your second example would actually return an error. As is, it would find an unexpected comma after the 7. If you quoted the date, it would return an error stating that the second parameter of date() is not the expected form. This is merely the convention that is followed in the prototype of the function. It actually makes sense if you think of anything being inside the square brackets as optional - if the comma were outside, then that would imply that you would use the comma without specifying the timestamp, which, of course, you dont. You say that in the previous example, the comma is outside - that's not the case. Its simply that when using the function, there is nothing to show which parameters are optional. You simply use a comma to separate all arguments. Thank you. Its not too complex - it grabs the track listing from my last.fm webpage (which is generated from an application which monitors what i play through WMP), and creates the image with that text on it.