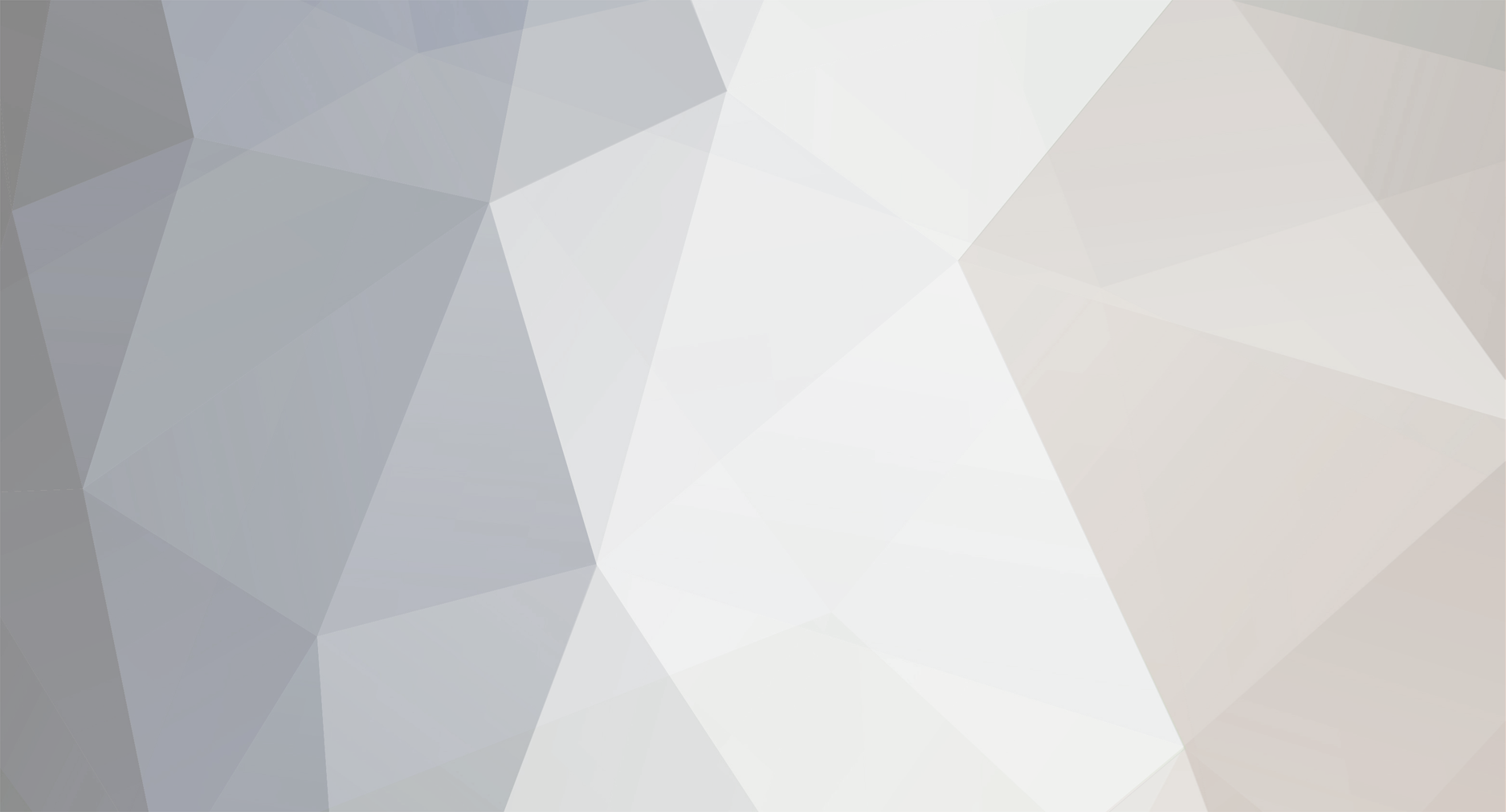
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
Removing numbers from a set of numbers
GingerRobot replied to thefollower's topic in PHP Coding Help
At it's simplest: <?php $choices = array(1,2,3,4,5,6,7,8,9,10); $used = array(5,7); $possible = array_diff($choices,$used); $possible = implode(',',$possible); echo $possible; ?> It really depends on what its for as to how you would actually do it. -
You need to use the in_array() function to determine if a particular value is in an array: <?php $used = array("0","1","2","3","4","5","6"); $number = rand(0,10); while(in_array($number,$used)){ $number = rand(0,10); } echo 'Number: '.$number; ?> Of course, the above code would enter into an infinite loop should all the possible numbers gererated by the rand() function be in the $used array.
-
That'd be pretty pointless. No need to query the database another time. Just use the same value that you used to update the database to change the value of the variable, $Username.
-
So do some sites use a particular CSS file and other sites some other CSS file?
-
Just a simple bit of maths with the sizes: <?php $sql = "SELECT SUM(size) AS sum_size FROM `uploads` WHERE uploader = 'steviez' "; $result = mysql_query($sql) or die(mysql_error()); $i = mysql_fetch_array($result); $space_used = $i['sum_size']; $max_space = 100 * 1024 *1024; //100MB if($space_used > $max_space) echo "You are using too much!"; else echo "You are ok, you are using ".ByteSize($space_used)." out of allowed ".ByteSize($max_space)." which is ".number_format($space_used/$max_space*100,0)."% of your allowed space"; $mb_used = ByteSize($space_used); function ByteSize($bytes) { $size = $bytes / 1024; if($size < 1024) { $size = number_format($size, 2); $size .= ' KB'; } else { if($size / 1024 < 1024) { $size = number_format($size / 1024, 2); $size .= ' MB'; } else if ($size / 1024 / 1024 < 1024) { $size = number_format($size / 1024 / 1024, 2); $size .= ' GB'; } } return $size; } ?>
-
Definitely. No point worrying about something you can't change. On the (sort of) subject of salaries, i went to an interview at Imperial College London yesterday (it's a universtiy, if you didn't know). During a presentation, they mentioned that one of the companies that takes 2nd year students from Imperial on work placements pay them around £19,000 for a 6 month internship, which works out as being able to pay off most of my student debt, and is also just under the average annual starting salary for Imperial graduates - which already has the highest average starting salary for graduates of any UK universtiy. I think it was about then when i really decided i could put up with living in London for 4 years!
-
Well, if you HAVE to use this sort of algorithm, you're going to have to do this within PHP, you wouldn't be able get mysql to do much of the work for you. What i propose would only be a viable solution if there aren't thousands of results to search through, since we must work out the number of consecutive words in each result, and that involves cyling through all our search terms. The more search terms and the more results there are, the slower this would be. However, this should work, including when we have a result with two consecutive words twice, which would be more relevant than two consecutive words once, but less relevant than 3 consecutive words once (i assume anyway). <?php $search = 'one two three four'; $results[0] = 'two three four five'; $results[1] = 'one two three four'; $results[2] = 'one two four three'; $results[3] = 'one two five three four'; $search_terms = explode(' ',$search); $matches = array(); foreach($results as $result_key => $result){//cycle through our results foreach($search_terms as $key => $term){//use each word in the search terms to find how many consecutive matches we have $temp = $term;//temporary string we're searching for $found = 0;//how many words we've found $iterations = 0;//how many times while loop has run while(strstr($result,$temp)){//run this while the temporay string is within each result $found++; if(!isset($search_terms[$key+1+$iterations])){//if the next word doesn't exist, break - we've used all our search terms break; } $temp.=' '.$search_terms[$key+1+$iterations];//if it DOES exist, change the temp string to include the next word $iterations++; } $matches[$result_key][] = $found;//we store EVERY consecutive number of matches we find, to allow for sorting where w words occur twice } } //echo '<pre>'.print_r($matches,1).'</pre>'; function sort_array($a,$b){//user defined comparison function to order our results rsort($a);//sort the two arrays of matches we are comparing rsort($b); if($a[0] > $b[0]){//if the first term in one is greater than, or les than, we can easily sort return -1; }elseif($a[0] < $b[0]){ return 1; }else{//otherwise, they're equal, and we have to continue looking through the array untill we find an unequal pair foreach($a as $k=>$v){ if($a[$k] == $b[$k]){ continue; } return ($a[$k] > $b[$k]) ? -1 : 1; } return 1;//if the arrays are identical, we need to return something - cannot order them in any meaningful way. } } uasort($matches,'sort_array'); //echo '<pre>'.print_r($matches,1).'</pre>'; echo 'Search term relevance:<br />'; foreach($matches as $k=>$v){ echo $results[$k].'<br />'; } ?> Edit: I've commented that quite heavily, but if you don't understand any of it, then just ask.
-
You'd have to grab the contents as suggested above, but instead of printing it, you'll need to evaluate it Personally, i'd find another host.
-
Hmm, well i spose i silently swore at a drawing of a sack of potatoes yesterday if that counts? I have to wonder, apart from sleeping with your wife, why would you need to swear at a potato?
-
Yes, as protection for pointless robots it might work. Of course, if this became common, all the robot would need to do would be to compare the background colour of the input field and the page, and then not fill it in if it is the same. However, it would do nothing for any script targetted at your form - which would be written not to fill in this other field. It seems to me that if you're going to use some form of captcha, you might as well use the one that would block all types of automated script submissions - not just some. Hence why i said it seemed a little pointless to me.
-
There is simply no way of doing this. Every way suggested can be faked or will cause problems for some users. The only reason why anyone would ever need to do this is because they are not doing proper validation. Validate your forms properly. That is the only solution.
-
Seems kinda pointless to me. Anyone who was deliberately trying to write a script to access your site wouldn't have much trouble in noticing that the value of the field isn't required. Edit: And, what about people who use tab to skip between fields? That could just get confusing.
-
My two cents on your questions: 1.) As PFMaBiSmAd said, the contents of $_POST and $_GET are sent by the browser. Each request is separate, so no, there cannot be any 'overlapping' of data between users. 2.) A session, in itself, does not secure an area of your site - it is the way that you use them that does. Obviously you have a login system which sets certain session variables which you will check on all pages requiring a login. You can use other session variables to pass other variables around, regardless of wether or not the user is logged in. It's often a matter of preference as to wether or not you set a session or add a variable onto the end of your header redirects which you then retrieve from the $_GET array. 3.) Yeah, global variables are exactly how you described them - if a variable is global, then it is in scope inside and outside a function. There is a slight difference as regards to functions defined with the global keyword, and the superglobal arrays (GET,POST,SERVER,COOKIE,SESSION). To use a variable defined outside a function inside that function, you need to use the global keywords, whilst the superglobals are available inside the function without any explicit use of a global keyword. 4.) As mentioned above, yes you could use GET to pass variables rather than sessions. You mention with particular reference to including files - if you want to pass something in the URL of an included file, you must use an absolute rather than a relative path. However, often you dont actually need to pass any variables to an included file. Remember, the process of including a file acts as if you take out all the code from the included file and dump it in the file doing the including. Therefore, any variables defined in the original file are still defined within the included code. 5.) That depends on what you mean. If, for example, you have something along the lines of check_login.php which checks the login of the user (duh), then yes - you must included it in every file that required login. If, on the other hand, we have a database connection script, which we include in file A and another file, file B - which also requires the database, but is also included in file A, then we don't need to incldue the database connection script within file B, so long as the database script is included before file B in file A. I hope at least some of that makes some sense and is useful.
-
I've tidied it up a little bit since we seem to be repeating the same checks a few times: <?php $fields = array('First Name' => 'firstName','Last Name'=>'lastName','Graduation Year' => 'gradYear','Full date of birth'=>'birthYear','Full date of birth'=>'birthMonth','Full date of birth'=>'birthDay'); $errors = 0; $dob_error = FALSE; foreach($fields as $k => $v){ if(!isset($_POST[$v]) && !empty($_POST[$v])){ $errors++; if($k == 'Full date of birth'){//these checks are needed since we have 3 DOB fields but only want the error message shown once if($dob_error === FALSE){ echo $k." is required<br /> \n"; }else{ $dob_error = TRUE } }else{ echo $k." is required<br /> \n" } } } if($errors == 0){//if there were no errors we can add the record // add the record $objAlumni = new Alumni(); $objAlumni->First = $firstName; $objAlumni->Last = $lastName; $objAlumni->GradYear = $gradYear; $objAlumni->BirthDate = new QDateTime($birthDate); $objAlumni->Save(); // print a line indicating that the record was added print('Record added for ' . $_POST['lastName'] . ', ' . $_POST['firstName']); } ?> On a side note, if you're working with each element in an array, you'll probably find it easier to use the foreach statement (as i did above) rather than a while statement. If you do use a while statement, you would need to use the next() function at the end of the while statement - which was one of the issues with your code.
-
You need to be passing the tag variable in the URL in all of your pagiation links - each time the page loads, it searches the database dependant on that variable, so it is always needed. You need to make your links like: echo " <a href='{$_SERVER['PHP_SELF']}?pagenum=1&tag={$_GET['tag']}'> <<-First</a> "; You'll need to change all the other links to also pass tag in the URL.
-
I'd reverse the string, find the first occurence after the given offset, then work out the position in the unreversed string: <?php $str = 'aaCaBaaaCaaaBaB'; $char = 'C'; $start = 12;//the position of second to last B $start_rev = strlen($str) - 1 - $start;//position of character in reversed string $str_rev = strrev($str); $pos = strpos($str_rev,$char,$start_rev); $original_pos = strlen($str) - 1 - $pos;//the position of the character in the original string. echo 'The first occurence of '.$char.' before the character at position '.$start. ' is at postion '. $original_pos; ?>
-
[SOLVED] simple php code displaying white page
GingerRobot replied to aebstract's topic in PHP Coding Help
Ah ok - i misread. Glad to help. -
[SOLVED] simple php code displaying white page
GingerRobot replied to aebstract's topic in PHP Coding Help
Two things: 1.)$page is undefined - if it comes from the URL, take it out of the $_GET array, if its posted, take it out of the $_POST array 2.)You need to quote your strings in your if statement, like if($page == 'home'){ The reason for the blank screen is that your script produces a parse error (for the reasons given above) and you have php set up not to display errors - therefore you don't even see the error. -
Another way to do it is to set up apache to parse .jpg files as .php files. If you were to stick the following code in a .htaccess file in a sub-directory: AddType application/x-httpd-php .jpg Apache would parse your .jpg files in that folder as php files. Thats why i say put it in a sub-folder, otherwise none of your normal jpg images would work! It's how i got the picture in my signiture to work.
-
[SOLVED] any reason why this simple image upload isnt working?
GingerRobot replied to scarhand's topic in PHP Coding Help
You're missing a couple or parts from your form. You need to specify an enctype of '"multipart/form-data", and you also need so specify a maximum file size in your form(not sure how far you'll get with your testing before this actually becomes a necessity, but it will at some point). Try: <form method="post" enctype="multipart/form-data"> <input name="MAX_FILE_SIZE" type="hidden" value = "1000000" /> <input name="myphoto" type="file" class="normalinput"> <br> <input name="submit" type="submit" value="Submit Registration"> </form> -
Ever had to work on code that you had written a few years back?
GingerRobot replied to zq29's topic in Miscellaneous
Hahaha -
Ever had to work on code that you had written a few years back?
GingerRobot replied to zq29's topic in Miscellaneous
If i tried to work on some of the stuff i wrote more than a year ago i'd be pretty stuffed. I think it would probably be quicker to rewrite the stuff than to try and figure out what it all did - not to mention an awful lot more efficient. I still have the code for a text based game i wrote whilst in the early stages of learning php - the sheer messyness of the code is quite scary! -
If you want to do this without reloading the page/redirecting, you'll have to use AJAX. Google AJAX and you'll see that you'll make javascript make a request to a php page which can handle the query to your database.
-
You have your or die statement on the wrong part. Try: <?php $db_host = "localhost"; $db_user = "auction_asset"; $db_pwd = "xxxxxx"; $db_name = "auction_asset"; mysql_connect($db_host, $db_user, $db_pwd); mysql_select_db($db_name); ?> <?php $sql = "SELECT * FROM equipment WHERE tag=$lot"; $query = mysql_query($sql) or die('Query failed: ' . mysql_error()); $tag = $row['tag']; $descrip = $row['descrip']; while($row = mysql_fetch_array($query)){ echo "<center>"; echo "$tag<br>"; echo "$descrip<br><br>"; echo "Is the above information correct?<br>"; echo "<form method=\"post\" action=\"bidrec2.php\">"; echo "<input type=\"hidden\" name=\"tag\" value=\"$tag\">"; echo "<input type=\"hidden\" name=\"descrip\" value=\"$descrip\">"; echo "<input type=\"submit\" value=\"Continue\">"; echo "</form>"; } ?> The reason for the blank screen is that your previous code gives a parse error - evidently you have display_errors set to off, and hence recieve no error message - just a blank screen.