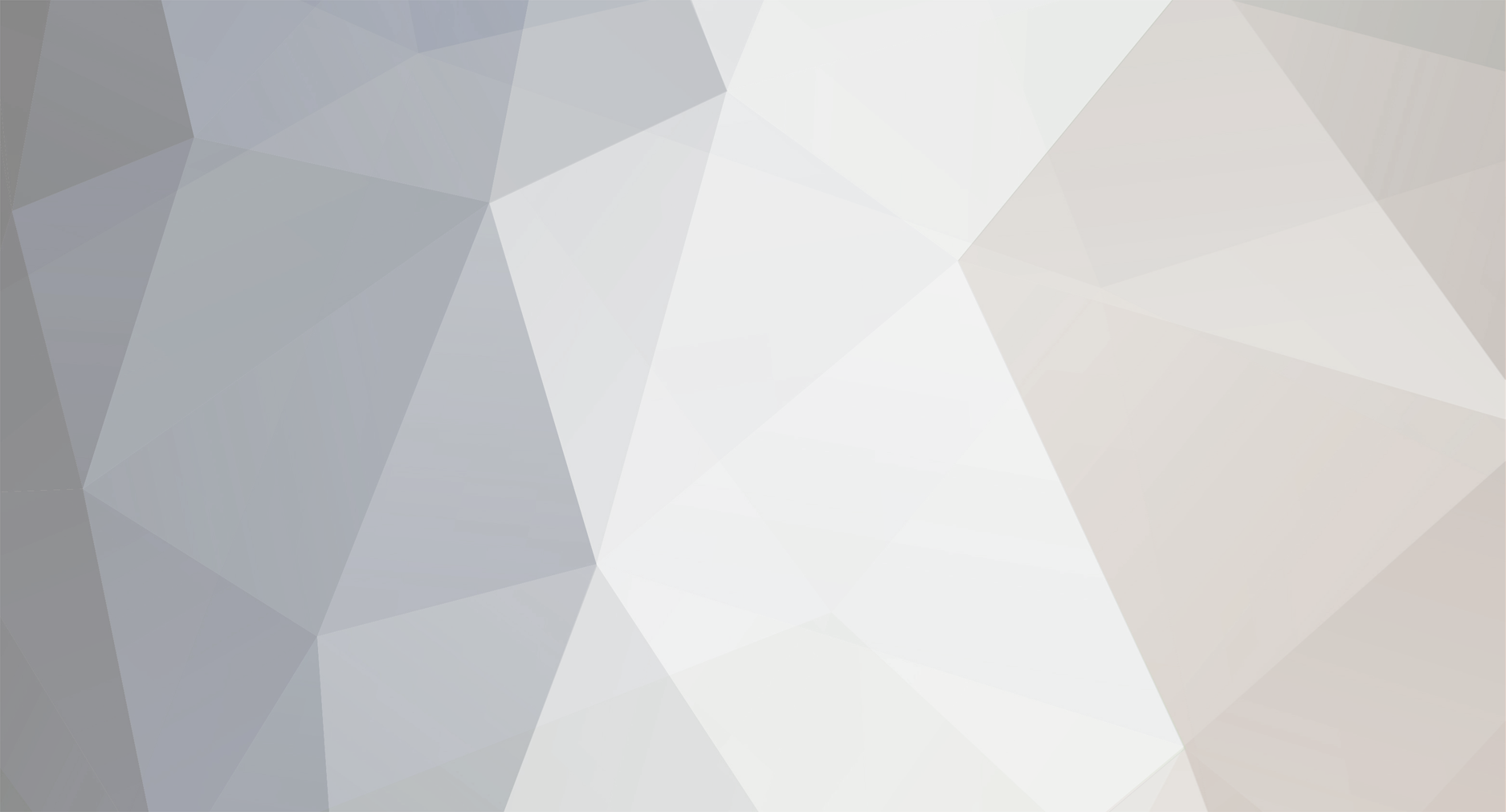
bljepp69
Members-
Posts
111 -
Joined
-
Last visited
Never
Everything posted by bljepp69
-
The function 'check_email()' returns either 'TRUE' or 'FALSE'. It returns 'TRUE' if there is already an email address in the db. So, the statement "if (check_email($addr))" is checking to see if the function is 'TRUE', or, there is already an email address. If so, then process the error and redirect using headers. If the function is 'FALSE' the code will continue processing below this particular 'if' statement.
-
construct for <div>image</div> Grid CSS PHP
bljepp69 replied to nadeemshafi9's topic in PHP Coding Help
Your logic is a bit out of whack. Once $accross is equal to 2, it won't increment any more. First iteration $accross = 0, second iteration $accross = 1, third iteration $accross = 2. From there on, $accross will always equal 2. More importantly, you are basically setting the value of $accross with each 'if' statement. If you are checking to see if $accross is equal to a value, you need to use if ($accross == 2). The statment if($accross = 2) simply returns true if it actually set $accross to the value of 2, which it always would. Try something like this... [code] <?php $accross = 0; $leftPos = 0; $topPos = 0; while($row = mysql_fetch_array($result)) { if($accross == 1) { $leftPos = 3; } if($accross == 2) { $leftPos = 6; } if($accross == 3) { $leftPos = 0; $topPos = $topPos + 4; $accross = 0; } print "". "<div style='text-align: left; position: absolute; ". "left: " + leftPos + "cm; top: " + topPos + "cm; width: 2cm; height: 4cm;'>". "<img src='img.asp?id=" + $row["id"] + "' height='80px' width='80px'><br>". $row["name"] + "<br>". $row["type"] + "<br>". $row["description"] + "<br>". "</div>"; $accross++; } ?>[/code] -
it's a good idea to put exit(); after your call to a header like that. Also, make sure you don't have any blank lines or spaces before your "<?php" Those are output to the browser and then you will get that header warning.
-
Variable defined in another page not getting accessed
bljepp69 replied to riteshn's topic in PHP Coding Help
[code] <?php require('config.php'); print_r($db_info); // 1 /* This is a complete PHP page all database related stuff goes here */ function ExecuteAndGetResult ( $query ) { global $db_info; print_r($db_info); // 2 } ?>[/code] -
You need to understand [url=http://www.php.net/manual/en/language.variables.scope.php]variable scope[/url] when using functions. Unless you specifically declare a variable to be "global" when defining it in the function, it is considered only applicable to that function. As corillo181 mentions, you can pass variables to a function and use the value, but you have to allow for that when defining the function. So, a call to 'check_email($email)' is probably the best way to do this. Your function needs to look like this. [code] <?php function check_email($addr) { $check_email = mysql_query("SELECT * FROM users WHERE email = '$addr'"); $emailcheck = mysql_num_rows($check_email); if ($emailcheck > 0) { $reason = "-The email address is already in use with another account sorry.<br />"; header("Location: index.php?page=register&reason=$reason"); } } ?>[/code] Note that you called the 'check_email() function with the variable $email, but you defined the variable in your function as $addr. Inside that function, you use the value $addr (which = $email when called as mentioned). Also, your header location and query string are going to be messy the way you are calling them. The '<br />' will be encoded and then you will have to decode it. It might even break altogether. A better way to define a function like this might be: [code] <?php function check_email($addr) { $check_email = mysql_query("SELECT * FROM users WHERE email = '$addr'"); $emailcheck = mysql_num_rows($check_email); if ($emailcheck > 0) { $err = TRUE; } else $err = FALSE; return $err; } ?>[/code] Then, you can call the function like this: [code] if (check_email($addr)) { $err_code = 'A'; header("Location: index.php?page=register&reason=$err_code"); exit(); } [/code] Finally, in the index page, you would put a section where you check for the existence of a '$_GET['reason']' and then write out a longer description for 'A', in this example.
-
You have: [code] <td><div align="right">Security Code :<br> <span class="style3">Enter The Code Thats Below </span></div></td> <td><label> <input name="key" type="text" id="key"> </label></td> </tr> <tr> <td><div align="right"> <input name="keyc" type="hidden" id="keyc" value="'.$key.'"> </div></td> <td><span class="style4">'; $RandomStr = md5(microtime()); $key = substr($RandomStr,0,5); echo '<label> <input name="key3" type="text" id="key3" disabled value="'.$key.'"> </label> </span></td> </tr> [/code] which defines a hidden input field named 'keyc' but the variable, $key, hasn't been defined yet. That comes a few lines later. When you process the script in the second code you show, the value for $_POST['keyc'] will be nothing. Define $key earlier in your code. Do a View -> Source on your HTML form to make sure the hidden value 'keyc' is filled in.
-
mysql_num_rows... not a valid MySQL result resource?
bljepp69 replied to Unholy Prayer's topic in PHP Coding Help
This line: [code]$count_sql = mysql_query('SELECT * FROM threads WHERE fid = $fid') or die(mysql_error());[/code] uses single quotes. The variable won't be processed in single quotes. Change it to: [code]$count_sql = mysql_query("SELECT * FROM threads WHERE fid = $fid") or die(mysql_error());[/code] -
Now it's time to hunt down where the error is that's causing the query to not be valid. Try this code. I have added some echo statements to send output at various points in the code so we can see what's happening. [code] <?php include('config.php'); $db=mysql_connect($db_host,$db_user,$db_pass); mysql_select_db ($db_name) or die ("Cannot connect to database"); if(isset($_GET['id'])) { $id = $_GET['id']; echo "id = $id<br />"; //check the value of $id echo "<!-- Your query was selected with a get method -->"; $query_text = "SELECT * FROM news WHERE id='$id'"; //verify that the query actually picked up the variable $id echo "query = $query_text<br />"; $query=mysql_query($query_text) or die(mysql_error()); } if (mysql_num_rows($query)>0) { echo mysql_num_rows($query)." rows returned<br />"; //see how many rows are actually returned while ($r=mysql_fetch_array($query)) { $nid = $r['id'] $title = $r['title']; $news = $r['news']; echo "<form name='edit_process' method='post' action='edit_save.php?id=".$nid."'> <p>Title : <input type='text' name='title' value='".$title."' /> </p> <p>News :</p> <p> <textarea name='news' cols='40' rows='6'>".$news."</textarea> </p> <p> <input type='submit' name='Submit' value='Save' /> </p> </form>"; } } else { echo "<p>No news items.</p>"; } ?>[/code] Also, you can get rid of the "Notice" by setting this at the top of your code... error_reporting(E_ALL ^ E_NOTICE);
-
Try formatting your SELECT statement like: [code] SELECT username, name, website, phone, email FROM users WHERE *** = {$_POST[***]} AND --- = '{$_POST[---]}' AND +++ = {$_POST[+++]} ORDER BY name [/code] Note the {} around the POST variables. If you have something like $_POST['number'], the single quotes around 'number' will screw up the parsing of the SELECT statement. Putting the {} around the variable tell PHP where the variable starts and ends.
-
That likely means that your query ($query=mysql_query("SELECT * FROM news WHERE id='$id'") returned nothing. No rows matched your selection criteria, so the resource ($query) is empty. Then the call to mysql_fetch_array is invalid. Try this: [code] <?php include('config.php'); $db=mysql_connect($db_host,$db_user,$db_pass); mysql_select_db ($db_name) or die ("Cannot connect to database"); if(isset($_GET['id'])) { $id = $_GET['id']; echo "<!-- Your query was selected with a get method -->"; $query=mysql_query("SELECT * FROM news WHERE id='$id'") or die(mysql_error()); } if(isset($_POST['id'])){ $id = $_POST['id']; echo "<!-- Your query was selected with a post method -->"; $query=mysql_query("SELECT * FROM news WHERE id='$id'") or die(mysql_error()); } if (mysql_num_rows($query)>0) { while ($r=mysql_fetch_array($query)) { $nid = $r['id'] $title = $r['title']; $news = $r['news']; echo "<form name='edit_process' method='post' action='edit_save.php?id=".$nid."'> <p>Title : <input type='text' name='title' value='".$title."' /> </p> <p>News :</p> <p> <textarea name='news' cols='40' rows='6'>".$news."</textarea> </p> <p> <input type='submit' name='Submit' value='Save' /> </p> </form>"; } } else { echo "<p>No news items.</p>"; } ?>[/code] I also think you could get rid of the "if (isset($_POST['id']..." statement. You don't have any variable called 'id' in your form and you are only using a GET method in the query string. If you wanted to make the processing code such that it didn't matter, then change the GET to REQUEST and then it will grab both POST & GET variables.
-
You're missing a ';' on line 17... $nid = $r['id'];
-
You might have to set the image path to the full server path - /home/httpd/vhosts/nicaprojects.com/httpdocs/news_images/LaSaltaDeEstanzuelaWaterfall (Small).jpg, instead of simply - /news_images/LaSaltaDeEstanzuelaWaterfall (Small).jpg. Or, if news_images is easily accessable as a relative path from image_mailer.php, try simply 'news_images/$image', or whatever relative variant, insead of the leading '/', which is trying to take it to the root directory.
-
1. You have to make sure your hosting company allows for url rewrites. 2. You also need to make sure your .htaccess file includes the following: Options +FollowSymLinks RewriteEngine On (Then do your RewriteRule here) Based on the error you're getting above, it looks like your server is honoring the fact you have an .htaccess file. Any misspelling or bad formats within the .htaccess file can cause this issue, however. There's a good tutorial on here - [url=http://www.phpfreaks.com/tutorials/23/0.php]Search Engine Friendly URLs with mod_rewrite[/url]
-
Leave 'CommentID' out of your INSERT query: $sql = "INSERT INTO `tblBlogComments` (`BlogID`, `UserID`, `Active`, `Username`, `Comment`) VALUES ('1', '1', '1', 'James', 'test')"; Since it's an auto_increment, it will increment without you doing anything to it. Every time you insert a row...increment.
-
Your .htaccess Rewrite Rule would look something like: RewriteRule ^pages/(.*) /page.php?p=$1 This tells Apache to use the file page.php with the query string 'p=pagenamehere'. Then you put the proper code in your page.php file to retrieve the file listed in $_GET['p']
-
if 'duration' is type TIME in your db, you should be able to use the DATE_FORMAT query and get the output. If you pull out a TIME type and try to do regular math, you won't get the expected results. You would have to convert it to a timestamp, then do your calcs, then convert back to time.
-
use 'str_pad' - basically, you are just going to pad the string with a 0 on the left side. Something like this: [code] <?php $sql = mysql_query("SELECT SUM(duration) AS count FROM reports"); $count = mysql_result($sql, 0, 'count'); $total = $count; $v1 = str_pad( (floor($count/60)) , 2, '0', STR_PAD_LEFT); $v2 = str_pad( ($count % 60), 2, '0', STR_PAD_LEFT); echo $v1.':'.$v2; ?> [/code] You might even be able to get the right format with just the mySQL query, but I haven't tested this. Try something like. [code] $sql = mysql_query("SELECT DATE_FORMAT(SUM(duration),'%H:%i:%S') AS count FROM reports"); [/code]
-
You could try forcing it to an integer value by specifically declaring it that way. Something like this might work: [code] $id = (int)$templates[$i]->id; ....OR.... (int)$id = $templates[$i]->id;[/code]
-
[code]\s[/code] is what you need to identify a space. Also, you need to escape the period(.) in your regex. So, try something like [code]$text=preg_replace_callback('/\[([A-Za-z0-9\¦\'\.-_\s]+)\]/i' , "findLinks", $text); [/code]
-
check out the glob(0 function - http://www.php.net/glob [code] foreach (glob("*.txt") as $filename) { echo "$filename size " . filesize($filename) . "\n"; } [/code]
-
if your function "display_item()" gets called after the user clicks "Submit" on the form, then change the value for $id in the display_item() function to : $id = $_POST['id']; I'm just making an assumption that is how the code flows.
-
I [i]think[/i] that with the new hosting configuration on GoDaddy, you can put a supplemental php.ini file in your root directory. So, you might try creating a php.ini file and putting it in your root directory. It would be a pretty simple, one line deal: allow_url_fopen = on The first time you add this file (or an .htaccess file) where there hasn't been one before, you might have to wait 30-60 minutes until they get it properly cached. Once that happens, you can make changes that are implemented immediately. Hope that works.
-
It's a bit difficult to follow, but if you are talking about not being able to retrieve values after the form shown in the "edit_what()" function is submitted, it's probably because your form is a "POST" form and so $_GET['id'] becomes $_POST['id']
-
I have a large text file with approx. 400,000 lines. The file is tab delimited and it's very easy to parse each line. I'm working to grab certain information from each line and put into a mySQL database. I'm running into a big issue where the script starts working and simply times out after processing somewhere between 1,000-2,000 lines, or so. I suspect this is an issue with max_execution_time, but I don't have access to change that and set_time_limit() doesn't seem to over-ride max_execution_time. My question is, what is the best way to deal with this large file? The best solution I've come up with is to break the file into smaller chunks and then write the script to deal with many files. Ideally, I would use a cron server to launch the script every so many minutes and just come back later when it's, hopefully, done. How do people normally deal with data files like this? Thanks for the discussion.
-
try: [code]$expression = '^[0-9]\.[0-9]$'[/code] That will look for a literal period(.) It should match numbers like 5.6, 6.7, 7.8 etc., but not 56, 56.7, 5.67, etc. You can also consider a subexpression like: [code]$expression = '([0-9]\.[0-9])'[/code] and that should match the same thing without the beginning and ending requirements.