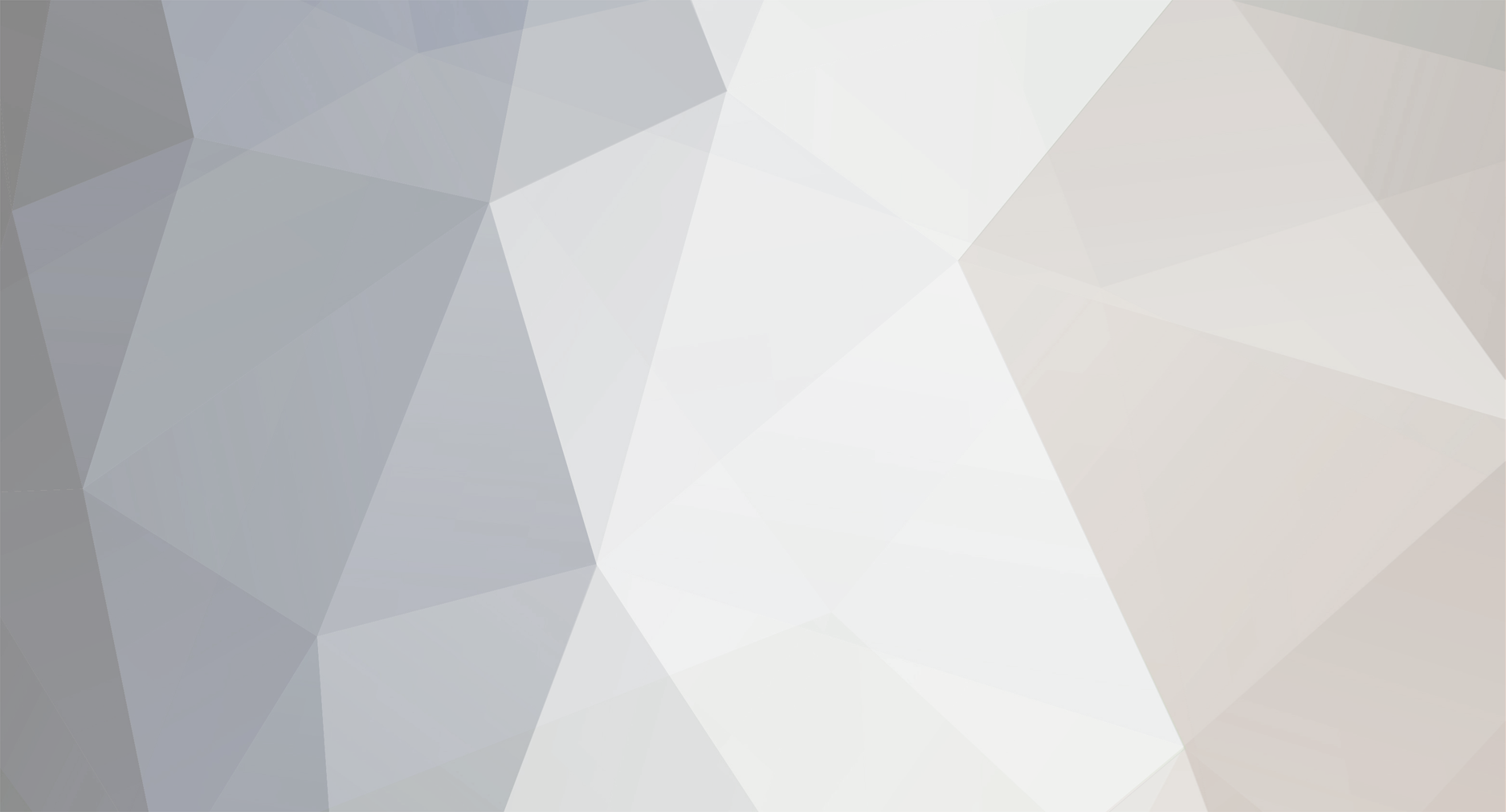
bljepp69
Members-
Posts
111 -
Joined
-
Last visited
Never
Everything posted by bljepp69
-
Needs to be: [code] <?php $do=fopen("sing/index.php",'x'); $filepath= 'sing/index.php';//don't need this $write='this is all shit man'; if($do){ fwrite($do, $write); } fclose($do); ?> [/code]
-
It's a bit more complicated than that. Here's a tutorial that describes a method for multipage forms - [url=http://www.phpfreaks.com/tutorials/145/0.php]http://www.phpfreaks.com/tutorials/145/0.php[/url] There are lots of posts on this board discussion sessions and how to implement them. I do a lot of forms where I process on the same page. You basically write your processing code at the top of the page and check for the existence of a certain POST or submit variable when the page loads. If the form has been submitted, you run your processing script, otherwise, display the form.
-
Remove the quotes around lorder in your query statement [code] $result = mysql_query( "SELECT * FROM links where sec=1 order by lorder ASC") or die( "Unable to select database"); [/code]
-
It's because you process the form in a different file. The values for $q1_x_check are set in calculate.php, but then the file with the form in it still doesn't know what those variables are. If you want to process in a separate file from where the form is, your best bet is to use session variables to pass the information back to the form page. The other option is to process the form on the same page.
-
The mail() function as you've described it above, sends a text-based email. So, you could simply type out the link like this - http://www.mysite.com - and then it's up to the recipient's email client as to whether or not it gets displayed as a link or as text. You won't have control of that. If you want to send HTML mail, it gets a bit more complicated because you have to send a bunch of headers in the mail() function. However, then you would be able to send a link, and, assuming the recipient can receive an HTML email, they will see the link as you describe it in HTML (e.g. <a href="http://www.mysite.com">My site</a>)
-
You are exactly right. I made the assumption your form method was POST, so the $_POST super global holds the value of all form variables. You are also correct on the interpretation of the format for the variables. It's basically a shorthand version of an if/then statement. It's called a ternary operator and it's defined: The expression (expr1) ? (expr2) : (expr3) evaluates to expr2 if expr1 evaluates to TRUE, and expr3 if expr1 evaluates to FALSE.
-
In the last code you posted, you had called curl_mail() like this: [quote] curl_mail($_POST['username'],"Registration",$msg,"From: Private msgs Admin <robert@usnet1.net>"); [/quote] Did that work? It should work better than what you had before because you've now defined a variable, $msg, that would contain the value of $ses. It looks like the output echoed is what you are looking for. I suspect the reason it didn't work the way you originally called curl_mail(): [quote] curl_mail($_POST['username'],"Registration","Thank you for registering when logging for the first time use: {$ses} as your password","From: Person"); [/quote] is because you literally passed "...use: {$ses} as..." and not the value of $ses. Then, when the function tried to process the comment, it tried to parse $ses and that's where you got the error.
-
Here's a method I've used to keep the radio button selections during form processing. Set up the radio buttons like this: [code] <input type="radio" name="radio1" value="Y" <?php echo $ry_check; ?>>Yes <input type="radio" name="radio1" value="N" <?php echo $rn_check; ?>>No [/code] Then use something like this in your form processing. [code] <?php $ry_check = ($_POST['radio1']=='Y') ? 'checked' : ''; $rn_check = ($_POST['radio1']=='N') ? 'checked' : ''; ?> [/code] That should keep the values selected by the user.
-
Yeah, you'll have to write it to the server and then download the file. If you're doing all that, you might as well just echo the data to the screen and then copy-and-paste into Excel on your PC. Probably faster overall, and definitely less coding.
-
You could easily write it to a "csv" file and then open that in Excel. This code should take all the POST variables and use the keys as the header row and put the values in the row beneath them. Haven't tested it though. Also, there is no error checking in this code. [code] <?php foreach ($_POST as $key=>$val) { $$key = stripslashes($val); $header .= $key.","; $data .= $val.","; } $header = substr($header,-1)."\n"; //to strip away trailing comma and add a newline character $data = substr($data,-1);//to strip away trailing comma $filecontent = $header.$data; //merge the content $handle = fopen("yourfilehere.csv","w"); fwrite($handle, $filecontent); // Write $filecontent to your opened file. fclose($handle); ?> [/code]
-
[url=http://www.php.net/rename]rename()[/url] Since you are picking the new name / location with above command, you should be able to store that in a db.
-
For checking the form submission using PHP you could try something like: [code] if (!preg_match('/[^0-9]+/',$FORMVARIABLE)) { //error code goes here } else { //process code here } [/code] I haven't tested this, but it should reject everything that's not an integer. You'll just have to fill in the $FORMVARIABLE with your actual variable and then write the error and process code.
-
Just cleaned up your code: [code] <?php $ip = $_SERVER['REMOTE_ADDR']; if ($ip == "68.xx.xx.xx") echo "<html><b><a href=\"http://xxx.no-ip.com/NewPage/news\">Main News</a><br><a href=\"http://xxx.no-ip.com/NewPage/pages/journal/news\">Journal News</a><br></html>"; else null; ?> [/code] Tested the above with my IP address, and it worked fine.
-
Here's a method I've used to show the search string in bold text: [code] <?php function formatSearch($text,$term) { return preg_replace("/{$term}/i",'<strong>${0}</strong>',$text); //note the ${0} in the replacement term keeps the original string that matched the search term } //you call the function like $formatted_result = formatSearch($search_results,$search_for); //or echo it directly echo formatSearch($search_results,$search_for); ?>[/code] Hope that works for you
-
You just have an extra ; in that line. Should be: [code]$query = mysql_query("SELECT * FROM news WHERE id=$id") or die(mysql_error());[/code]
-
Bottom line on the "Warning: mysql_fetch_array()..." is that your query ("SELECT * FROM news WHERE id='$id'") returned nothing. Therefore, a call to grab the data from the query, like what you are doing with mysql_fetch_array() is invalid. Maybe try re-formatting your query like this: [code]$query = mysql_query("SELECT * FROM news WHERE id=$id");[/code] I removed the single quotes (') from around the variable $id. Have you used something like phpMyAdmin to run the query to make sure that with a valid $id you get something?
-
Is there something in your "header.php" include file that could be causing an issue with the session? The output you show above means that your $_SESSION variable is empty. Move the 'echo...' line above the includes, and try it again.
-
Try this at the top of your page. This will show you what session variables there are and what their values are: [code] <?php session_start(); include_once'includes/header.php'; include_once'includes/db.php'; echo "SESSION:<pre>".print_r($_SESSION,TRUE)."</pre>"; ?> [/code] Then you will at least know for sure that the session variables are there.
-
Your function returns "$word1 - $word2", but your function call didn't give it anything to return that value into. [code]$var = my($word1,$word2);[/code]
-
Unless register_globals is on (in your php.ini file), which it likely isn't, you will need to specifically call the session variable "username" like $_SESSION['username']. With register_globals off, $_SESSION['username'] is NOT the same as $username. [code]<?php if($_SESSION['username']){}else{?>[/code]
-
[code]foreach ($_GET AS $key=>$val) { $getarray[$key] = stripslashes($val); }[/code] will put all the $_GET variables into an array called $getarray. The array is formatted with the GET variable name as the array key and the value as the array value.
-
I'm pretty sure the full variable is getting passed to the processing page. You can test by putting the following line at the top of the code and you will see all your $_POST variables. [code]echo "POSTS: <pre>".print_r($_POST,TRUE)."</pre>";[/code] I think your problem is that the "From:" header is looking for a little different format. Try one of these: [code] $headers = "From: {$_POST['fullname']} <$email>"; //or $headers = "From: $email"; [/code]
-
Make sure you use "session_start()" at the top of the second page as well. Otherwise, the session variables won't be passed.
-
Make CustID Problem with preg_match *SOLVED*
bljepp69 replied to scottybwoy's topic in PHP Coding Help
You can use [url=http://www.php.net/str_pad]str_pad()[/url]. Using this function, you wouldn't even have to check to see if the value is <= 9 or <= 99. [code]$rowInt = str_pad($rowInt,3,'0',STR_PAD_LEFT);[/code] This will return $rowInt with 3 character places. Any places not taken with a number will be replaced with a '0'.