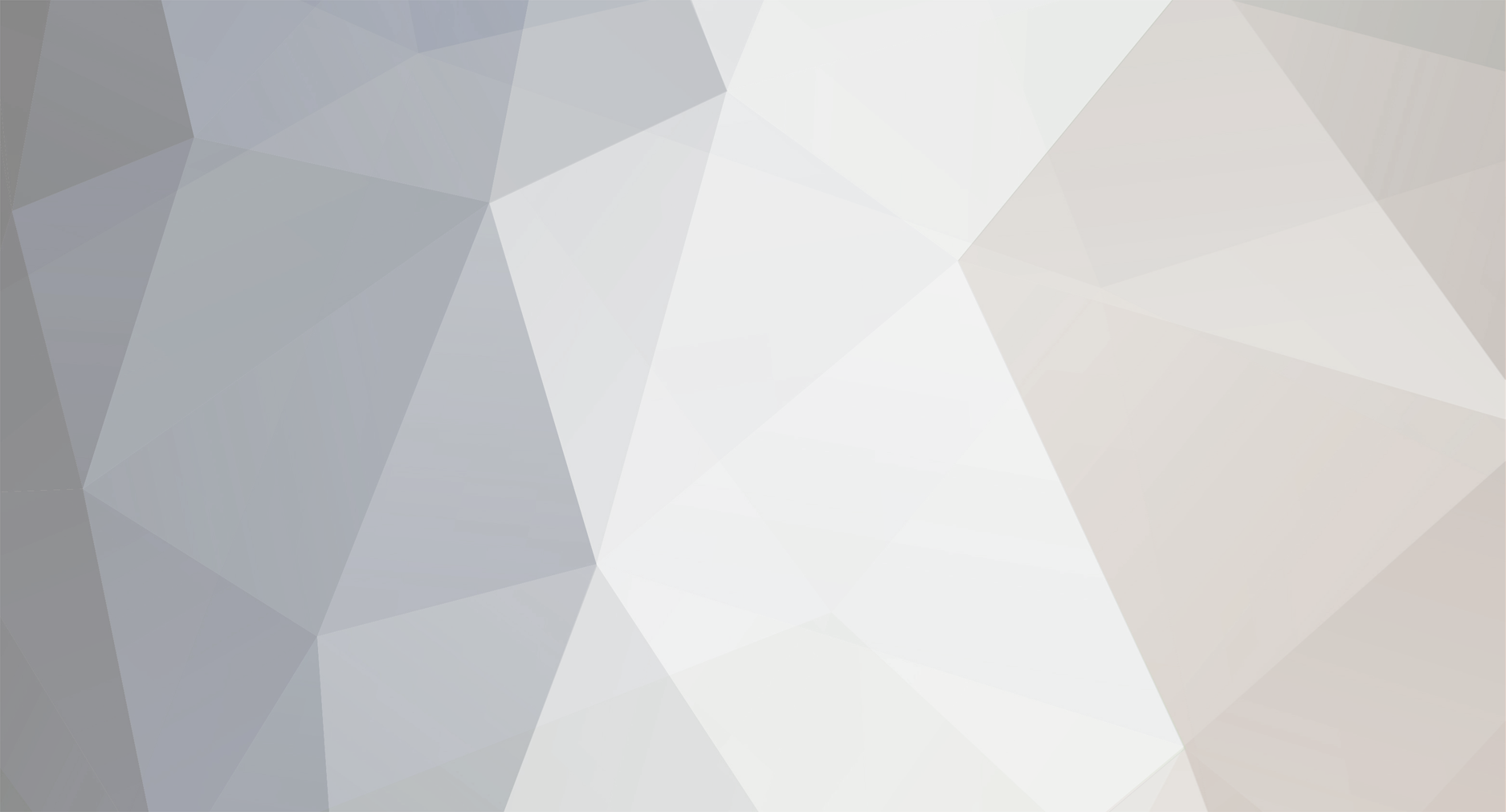
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
Your design is suffering from the illness Singletonitis. Try my Paginator class: class Paginator implements Iterator { private $key = 0; private $sizeOf = 0; private $itemsPerPage; private $data = array(); private $activePage = 1; public function __construct($array, $itemsPerPage) { $this->data = $array; $this->sizeOf = sizeof($array); $this->itemsPerPage = abs((int)$itemsPerPage); } public function getItemsForPage($pageNumber) { $pageNumber = $pageNumber > 0 ? $pageNumber : 1; $pageNumber = $pageNumber <= $this->getNumberOfPages() ? $pageNumber : $this->getNumberOfPages(); $this->activePage = $pageNumber; $this->key = $this->calculateOffset($pageNumber); return $this; } public function getActivePage() { return $this->activePage; } public function getNumberOfPages() { return ceil($this->sizeOf / $this->itemsPerPage); } public function valid() { return $this->key < $this->calculateOffset($this->getActivePage() + 1) && $this->key < $this->sizeOf; } public function key() { return $this->key; } public function next() { $this->key++; } public function rewind() { //$this->key = 0; } public function current() { return new ArrayObject($this->data[$this->key], ArrayObject::ARRAY_AS_PROPS); } private function calculateOffset($pageNumber) { return ($pageNumber - 1) * $this->itemsPerPage; } } Use it like: $rows = $db->execute('SELECT * FROM table')->fetchAll(); $paginator = new Paginator($rows, 20); $items = $paginator->getItemsForPage(1); foreach ($items as $item) { //whatever } @Jay Why did you limit your Paginator to MySQL? What if you were to use PostGre or Oracle?
-
I intended it as a joke and I feel kinda sorry you have to grow up in a neighborhood like that.
-
I wasn't furious (bare in mind I'm not a native English speaker) I just pointed out that there is no need to write a function for something that already exists like when someone writes a function to get the extension of a filename instead of using pathinfo
-
JavaScript: function changeFontSize(size) { document.body.style.fontSize = size + 'px'; } Something like this should do the trick: <a href="#" onClick="changeFontSize(16);">Normal</a> <a href="#" onClick="changeFontSize(20);">Medium</a><!-- 125% --> <a href="#" onClick="changeFontSize(24);">Large</a><!-- 150% -->
-
Where do you get this logic? Just use: echo money_format('%i', $row_getsum['SUM_value'] . "\n";
-
I don't see how that's going to be a problem. Is not a good idea, because the first 50 will remain the first 50 as all others would-be popular keywords are always reset while the first 50 will keep incrementing.
-
How much does a gun cost? Give them a healthy meal something with lots of iron.
-
I doubt he will be able to write his own version control system using something like Subversion is really his best option as all he'll have to do is: cd /path/to/web/root/ svn update You can use hooks but I do not recommend it as you don't want an unstable copy on the production server.
-
[WARNING] Question requires ULTRA PRO GODLIKE MYSQL MASTER![/WARNING]
ignace replied to this.user's topic in MySQL Help
I concur, you may want to save the id somewhere (like $_SESSION) after they have logged in to verify they are in fact logged in. And you don't need an ULTRA PRO GODLIKE MYSQL MASTER for this as someone with a few months experience could tell you this too. -
wrong choice you should have gone for AlexWD's solution. It's stupid to write something that is already built-in.
-
If by that you mean I know how to write a class, then no you don't. The concepts revolve around inheritance, abstraction, encapsulation, and polymorphism. Do you know what polymorphism means? And what encapsulation means? And I already gave you resources: http://en.wikipedia.org/wiki/Solid_%28object-oriented_design%29 SOLID OOP principles http://en.wikipedia.org/wiki/Design_pattern_%28computer_science%29#Classification_and_list OOP patterns What kind of reference? OOP? OOA&D (as you are creating a project)?
-
Try: class Database { private $connection = null; private $result = null; private $lastQuery = ''; private $affectedRows = 0; public function __construct($name, $password, $username = 'root', $host = 'localhost') { $this->connection = new MySQLi($host, $username, $password, $name); } public function execute($query, $mode = MYSQLI_STORE_RESULT) { $this->lastQuery = $query; $result = $this->connection->query($query, $mode); if (is_object($result) && $result instanceof MySQLi_Result) { $this->result = $result; $this->affectedRows = $this->result->num_rows(); } else { $this->affectedRows = $this->connection->affected_rows(); } return $this; } public function fetchRow($mode = MYSQLI_ASSOC) { return $this->result->fetch_array($mode); } public function fetchAll($mode = MYSQLI_ASSOC) { return $this->result->fetch_all($mode); } public function getAffectedRows() { return $this->affectedRows; } } $db = new Database('name', 'password'); print_r($db->execute('SELECT * FROM people')->fetchAll());
-
Yup, you are passing the query to fetchAssoc() which executes the query and then returns the first result set (all the time): mysql_fetch_assoc(mysql_query($query)); I'm not sure that you know what this means but your query is executed on each loop which means that you will always get the first result back so $rows is populated with the first row (a few thousand times). These kind of error's you should be able to pick up as a programmer as they are very hard to track and your parser does not return any syntax error's, as they are so called logical error's.
-
If you're from the US I'd be surprised you've never heard of that expression before. Where are you from? Belgium
-
Try this: function insertProduct($id, $categoryId, $productName, $note, $quantity) { if ($id != abs($id) || $categoryId != abs($categoryId) || $quantity != abs($quantity)) return FALSE; $id = (int) $id; $categoryId = (int) $categoryId; $quantity = (int) $quantity; if (0 === $id || 0 === $categoryId || 0 === $quantity) return FALSE; $productName = databaseEscape($productName); $note = databaseEscape($note); $affectedRows = databaseQuery("INSERT INTO table (id, category_id, product_name, note, quantity) VALUES ($id, $categoryId, '$product_name', '$note', $quantity)"); if (1 !== $affectedRows) return FALSE; insertSession('cart', array('id' => $id, 'category_id' => $categoryId, 'product_name' => $productName, 'note' => $note, 'quantity' => $quantity)); return databaseLastInsertId(); } function insertSession($namespace, $data) { if (!array_key_exists($namespace, $_SESSION)) $_SESSION[$namespace] = array(); $_SESSION[$namespace][] = $data; } And use: if (array_key_exist(array('id', 'category_id', 'product_name', 'note', 'quantity'), $_POST)) { $insert = insertProduct($_POST['id'], $_POST['category_id'], $_POST['product_name'], $_POST['note'], $_POST['quantity']); if (FALSE !== $insert) { //success } } To check if something is submitted.
-
@CV Yeah I mostly have a hard time understanding what you mean, like "went balls to the wall"
-
Because you are creating an Object. It's the operator for objects. RegEx (Regular Expressions) clearfix is a CSS class, more info: http://www.webtoolkit.info/css-clearfix.html getMatch() is a method of the Object $scraper.
-
I didn't say it was a private school. I said that if the information was specific to the students it would have been on a more private URL but as it isn't everyone can read it and thus everyone can/will use it. And your website should support most/all of these and provide information they seek.
-
How do I explain security/insecurity/privacy to a client?
ignace replied to webmaster1's topic in Miscellaneous
Impossible, I have no such thing as a Brain -
if ($result='$_POST["username"]') Like mikesta already said $result is a result resource and the above code assigns '$_POST["username"]' (literally) to $result due to =. You most probably are looking for: $row = mysql_fetch_assoc($result); if ($row['username'] == $_POST['username'])
-
I think you are taking this a bit too serious. In Agile software development all of these diagrams are optional. Like Eisenhower you should plan but don't exaggerate.
-
How do I explain security/insecurity/privacy to a client?
ignace replied to webmaster1's topic in Miscellaneous
I actually answered your question. You can't guarantee them that something is ever 100% secure and add some paranoia and you get the idea. You can't tell them their data will be safe. It's the same as telling to someone who is catholic that God doesn't exist (the same as telling a child that Santa-Claus doesn't exist). -
A Use-Case is not a diagram it's a description of steps, as in text (and sometimes accompanied by a diagram)
-
You create one page and just retrieve the info you want to display on that page for a particular user.
-
What is difference between Switch() and If statement ?
ignace replied to shanali4's topic in PHP Coding Help
It may be not so clear as to what Ken was trying to explain but when you pass the value a, both a and b will execute. Not default as a break has been added before default: When you pass the value b only b is executed. What I'm trying to say is that, if you don't write the break it will fall-through (and execute all code in between) until a break is reached or the switch has ended.