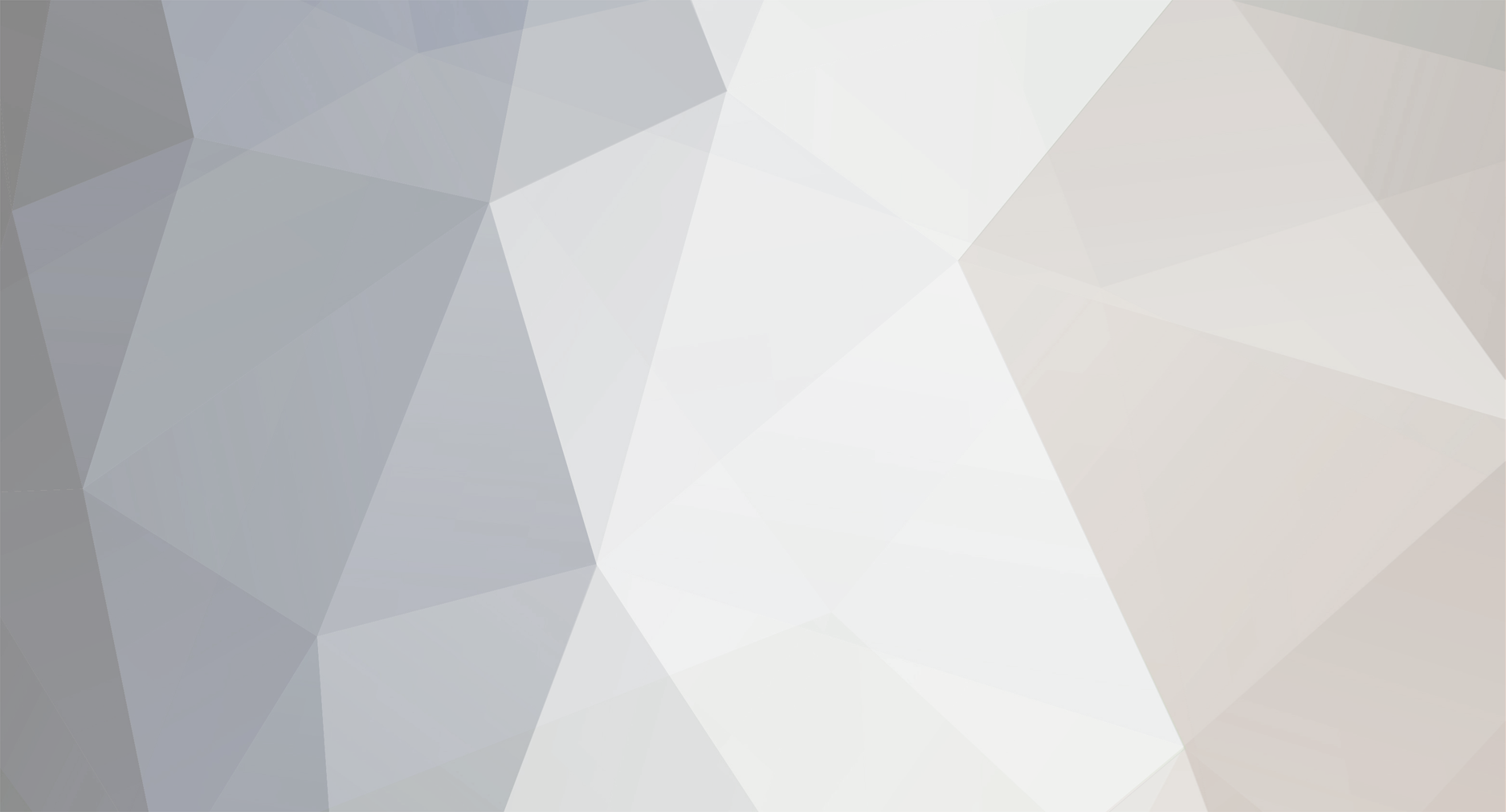
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
I think this query will suffice: SELECT * FROM shows WHERE shows_week = $week ORDER BY shows_date, shows_start Result has this order: Monday Aug 10, 00:30u Show#1 Monday Aug 10, 1:50u Show#2 Monday Aug 10, 3:30u Show#3 ... Tuesday Aug 11, ... ...
-
You can extend this further by adding a shows_type which references the primary key in the shows_types table and a shows_rating references the primary key in the shows_ratings table (rating applied by your internal reviewing crew) CREATE TABLE shows_types ( shows_types_id TINYINT NOT NULL AUTO_INCREMENT, -- increase (to SMALLINT) if you need more then 127 different types shows_types_name VARCHAR(32), PRIMARY KEY (shows_types_id) ); CREATE TABLE shows_ratings ( shows_ratings_shows_id INTEGER NOT NULL, shows_ratings_rating TINYINT, -- increase (to SMALLINT) if you need more then 127 stars shows_ratings_count SMALLINT, -- should suffice unless you receive more then 64k votes UNIQUE KEY (shows_ratings_rating), PRIMARY KEY (shows_ratings_shows_id) ); Queries: SELECT * FROM shows LEFT JOIN shows_types ON shows_type = shows_types_id INSERT INTO shows_ratings (shows_ratings_rating, shows_rating_count) VALUES ($rating, 1) ON DUPLICATE KEY shows_rating_count = shows_rating_count + 1
-
I assume: - Shows have a start and an end and between shows their is a commercial (shows_start, shows_end as the time a show takes greatly varies plus commercials). - A schedule shows all shows playing during the week CREATE TABLE shows ( shows_id INTEGER NOT NULL AUTO_INCREMENT, shows_title VARCHAR(32), shows_description TEXT, shows_date DATE, shows_start TIME, shows_end TIME, PRIMARY KEY (shows_id) ); Query: SELECT * FROM shows WHERE shows_date BETWEEN $monday AND $sunday ORDER BY shows_date Another possibility is using weeks instead of dates: CREATE TABLE shows ( shows_id INTEGER NOT NULL AUTO_INCREMENT, shows_title VARCHAR(32), shows_description TEXT, shows_week TINYINT, shows_date DATE, shows_start TIME, shows_end TIME, PRIMARY KEY (shows_id) ); Query: SELECT * FROM shows WHERE shows_week = $week ORDER BY shows_date PHP: $week = date('W');
-
http://us3.php.net/manual/en/features.http-auth.php scroll down to example #3
-
[SOLVED] Custom Built Radar Weather Tracker
ignace replied to barryhayden's topic in PHP Coding Help
$mydir = realdir('path/to/directory'); $dir = 'http://radar.weather.gov/ridge/RadarImg/N0Z/EAX/'; $images = scandir($dir); foreach ($images as $image) { if ('.' === $image[0]) continue; $fullurl = implode('/', array($dir, $image)); $fullpath = implode(DIRECTORY_SEPARATOR, array($mydir, $image)); if (!file_exists($fullpath)) { $fs = filesize($fullurl); $fh1 = fopen($fullpath, 'w'); $fh2 = fopen($fullurl, 'r'); fwrite($fh1, fread($fh2, $filesize), $filesize); fclose($fh1); fclose($fh2); } } -
echo a variable in a form based on user selection
ignace replied to jeff5656's topic in PHP Coding Help
We have a javascript sub-section: http://www.phpfreaks.com/forums/index.php/board,6.0.html -
[SOLVED] RePOSTing Search info for Paginated Results
ignace replied to kingnutter's topic in PHP Coding Help
Use a db table to store the search result along with the user's session id (to retrieve these rows). There are many tutorials that teach you how to paginate db table data, use these. Don't forget to clean this table up use an additional created_at column to delete them when they expire, also delete them as soon as the user initiates another search. -
You can't use an ini format for this if you parse it you will only get the last entry
-
<meta name="ROBOTS" content="NOINDEX, NOFOLLOW"> or create a robots.txt in your root: User-Agent: * Disallow: / This will however keep your website from every search engine out there
-
NaN means Not A Number and javascript uses it when it gets something passed that isn't a number: http://www.w3schools.com/jsref/jsref_NaN.asp
-
[SOLVED] PHP not outputting all database rows
ignace replied to jeeves245's topic in PHP Coding Help
add an AUTO_INCREMENT to your primary key -
You are using the wrong credentials for your db server
-
What do you actually mean by clonable?
-
Does your invites table have a primary (auto-incrementing) key? Or some key that is unique for each record, then modify 'id' to 'yourUniqueColumn'? <select name="attend[<?php print $info['id']; ?>]" .. $_POST['attend'] is now an array where the key represents the record <option value="1">Will be attending</option> <option value="0">Will not be attending</option> foreach ($_POST['attend'] as $pk => $willornot) { if (true == $willornot) { //assuming attending BOOLEAN NOT NULL DEFAULT FALSE $query = 'UPDATE invites SET attending = TRUE WHERE yourUniqueColumn = ' . $pk; //.. } }
-
[SOLVED] Shopping Carts differences in the same script...
ignace replied to gerkintrigg's topic in PHP Coding Help
It would be nice to know to which variable you are referring, plus have you started sessions? If not then $_SESSION is empty on each new request, which would explain why nothing is added. -
use <?php instead of <?
-
$result == 3 means nothing to some other programmer, rather use something like: define('USER_STATE_BANNED', 3); if ($result == USER_STATE_BANNED) { .. $dbarray['userlevel'] = stripslashes($dbarray['userlevel']); Don't think abstract if you are working concrete. In your application design userlevel is a number therefor: $dbarray['userlevel'] = (int) $dbarray['userlevel']; will be more appropriate. if($result == 1){ $field = "user"; $form->setError($field, "* Username not found"); } else if($result == 2){ $field = "pass"; $form->setError($field, "* Invalid password"); } else if($result == 3){ $field = "user"; $form->setError($field, "* Your account has been banned"); } $form->setError($field, "* Your account has been banned"); won't stop the user from logging in, you are not taking any measures.
-
Then my guess wasn't far off as it lists the router/switch and it's options.
-
It's pretty obvious. It lists all routers with their name, protocol, network and alot of other stuff.
-
You can't use fixed values unless you only have 1 product to sell or are all your products labeled 55 USD?
-
What does this Piece of PHP codes do?
ignace replied to natasha_thomas's topic in Third Party Scripts
127.0.0.1 is indeed a loopback address depending on who is using it. If I use 127.0.0.1 then I'll be using my own loopback address and not yours (as you will use yours and not mine). 127.0.0.1 is a reserved class B network address. Like all addresses in the format x.x.x.0 and x.x.x.255. So when you order your crawler to go to 127.0.0.1 it will do so on its own server not yours (I think this must be the technical equivalent to: go **** yourself). -
Create all conversions 100-based so you can use them as percentages, use google: 100 usd to <currency> Then divide currency through 100 (<currenty>/100) the currency conversion for usd to eur = 0.7 function country_amount($netcashgross) { global $cconv;/*currency conversions*/ $r = run_query('SELECT userid,country FROM users_details WHERE userid =149'); $country = strtoupper($r['country']); $conv = !empty($cconv[$country]) ? $cconv[$country] : 1; return $netcashgross * $conv; } print country_amount($netcashgross);
-
foreach ($matches as $file => $times)
-
setcookie('tpverify',(md5($rand_string))); Don't store stuff in cookies use sessions instead: $_SESSION['tpverify'] = md5($rand_string); if(md5($verification) != $_SESSION['tpverify'])
-
I have slightly modified the code and sorted $matches for your convenience. if (!empty($_GET['name'])) { $globQuery = implode(DIRECTORY_SEPARATOR, array(realpath('maps'), '*/*')); $files = glob($globQuery); $words = str_word_count($_GET['name'], 1); $matches = array();//array_flip($words); foreach ($files as $file) { $file = basename($file); foreach ($words as $word) { if (stripos($file, $word)) { if (!isset($matches[$file])) { $matches[$file] = 1; } else { $matches[$file]++; } } } } arsort($matches); }