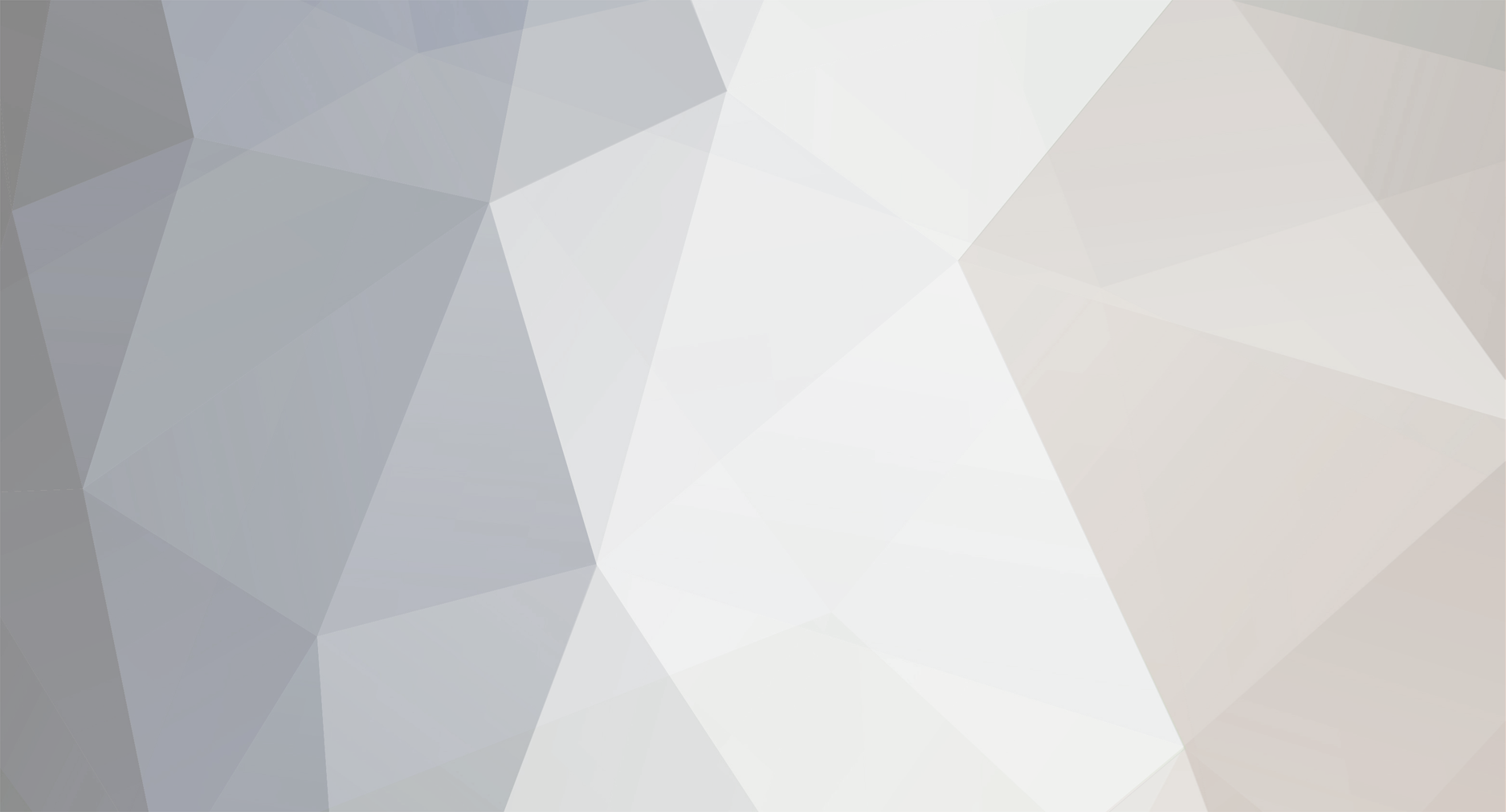
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
That's because you decided to listen to the less experienced person while thorpe gave you the answer: class MyObjectThatAlsoHappensToInteractWithADatabase { private $db = null; public function __construct(Database $db) { $this->db = $db; } } Another option you could opt for is to use a factory: class MyObjectThatAlsoHappensToInteractWithADatabase { private $db = null; public function __construct() { $this->db = AppAssetFactory::getDb(); } } That saves you the trouble of having to pass $db all the time.
-
+1
-
You should allow cURL to create and send cookies as I noticed csrftoken=2b4f94f8d03e0b00ea81e99fe90d53f1 In your cookie header. I think CURLOPT_COOKIEJAR does that.
-
Show us the code where you call ::authenticate() with the username and password. PS You may want to brush up on your OO since their is nothing OO about your code. 1) You use globals 2) All your properties are public 3) Every single method is static You could have saved yourself the trouble of having to write "class" everytime and just wrote procedural instead.
-
passing multiple function in codeigniter uri segment
ignace replied to linux1880's topic in Frameworks
Please read the CodeIgniter manual. As it contains answers to all your questions regarding CodeIgniter. -
Set the X-Requested-With header on your cURL object, anything else without the header will get a 404. Edit: nevermind I just noticed they use a X-CSRFToken which means that unless you know the token you can't get the information.
-
Nice, I really like the way it loads the next page (and tells you the page you are on) when you scroll down
-
Indeed, you will learn more by what you teach then any other forms of learning. My colleagues still don't understand why I try to give a presentation almost every once a week.
-
It doesn't matter how you turn it, the answer remains: NO. Using an array to store information like configuration variables and languages remain a popular technique among programmers. Passing this array around limits your program to only an array to store this information while at some point you'll realise that using an array to store languages is unmaintainable yet you used the array everywhere in your application so you have to go through the horror of finding and removing unused translations. Using a class instead gives you more flexibility as illustrated below: interface Translator { public function get($variable); } class ArrayTranslator implements Translator { public function __construct(array $array) { $this->_array = $array; } public function get($variable) { return $this->_array[$variable]; } } In my application I use it like: public function setTranslator(Translator $tl) Now i have come to the point where I realise that using an array to store my translations was a bad idea so I'm switching to Gettext and use the awesome tool POEdit to sync my translations. class GetTextTranslator implements Translator {/*actual code omitted*/} In my Factory I replace it with my new GetTextTranslator: class ApplicationFactory { public function getConfig() { if (is_null($this->_config)) { $this->_config = new ArrayConfig(include 'path/to/config.php'); } return $this->_config; } public function getTranslator() { if (is_null($this->_translator)) { $this->_translator = new GetTextTranslator('path/to/gettext/directory'); } return $this->_translator; } } I switched my entire application from using an array for translation data to gettext in one single line of code. How long would it have taken you to do the same using global $config or global $translate? Maybe it wouldn't have taken you too much time, if the code was properly written, but can you also switch between languages on the fly without leaving too much of a mess? $translate->_('Hello'); $translate->_('Good morning', 'fr'); // this should always be in French
-
Are they mad? Delegation is good practice.
-
JCBones made a very good example as why globals are bad: for($num = 0; $num > 10; $num++) { echo discount($prices[$num]); } Functions are meant to be black boxes, that is also how we use them: pass in information, get back the expected information. If it doesn't we have to go figure out why. You could easily solve this if this bug is found during development but what if this bug is discovered after 6 months, or a year? What if your company has expanded and you hired a few recruits? Surely they won't know $num is a global variable used in discount() would they? You could solve this problem partially by writing all global variables UPPERCASE to avoid overwriting it in normal application flow. I say partially because as your application grows more and more functions will be using/change the global variables which is prone to creating bugs. You could solve this even further by grouping all global variables and the functions that use them in a single file but then how do you avoid other functions/libraries of overwriting or writing to your global variable? What if we had a data structure that grouped functions and variables together and did not allow other libraries to overwrite or write to my variable, something like say.. a class?
-
Oh, how I wish that was possible
-
Your adventure has only yet started and you already have 3 options: MySQL, Lucene, or Sphinx? And that's only scratching the surface when it comes to selecting a DB
-
PHP and MySQL, but only the very basics since you don't know if PHP/MySQL is the way to go. Like I stressed and KingPhilip mentioned: your DB is the key part here.
-
Works best? PHP can work with any DB not just MySQL nor is PHP optimized to perform better when using MySQL as a back-end.
-
More important will be the database and -engine you'll use to store and retrieve the information from. For example if you go for MySQL, you would want to use MyISAM for it's FULLTEXT indexing. But if your searches are fulltext anyway then Lucene may be a better alternative? PHP is then just the middle-man to display the search results. SE typically have lots of text queries, no simple ID-select, so DB performance is critical.
-
@dougjohnson how does that answer his question on Since you only provide him the code on how to download the file. @OP You can't hide the URL since the download client needs a location to read the file from. If you want to hide the URL to avoid people downloading from the direct link. You can create a token that lasts for one hour so that they need a valid token in order to dowload from it. Combine that with checking the IP-address of the download and the User-Agent and the chance becomes really small multiple people are downloading from one and the same URL. This isn't a 100% guarantee but about 99.8% give or take.
-
Try phpwcms support, they can assist you much better: http://www.phpwcms.de/index.php?id=4,0,0,1,0,0
-
Installation is then complete. No button 'Go to website' or something below or above the sql queries? Otherwise go to the main website and try to login to the admin panel.
-
If you have phpMyAdmin access then log in, select the database you chose to install phpwcms in. Click on the SQL tab and copy-paste all queries. And press Execute of course.
-
You can't use self in a non-static context. Like mentioned: using die() is a bad habit even in procedural programming. In OO we use Exception, these are more flexible than a die() statement, once an Exception is thrown it will fall back till the Exception is handled (try..catch..) or PHP will show an uncaught exception error. global is bad, don't use it. It goes against the basic idea of encapsulation.
-
What prevents users from running your cron.php
ignace replied to clumsygenius's topic in PHP Coding Help
Checking on every request whether their is a job puts more strain on your server. Not ideal if you have around 20k to 30k users per day. -
Finding purpose for Developing Applications on a higher level
ignace replied to kanidrive's topic in Application Design
In short: write code, and provide estimates. The long version would be something like: - Implement classes - Write Unit-Tests - Refactor Code Since the developer writes code he's required to have a good understanding of OO: Inheritance, Polymorphism, Abstraction, and Encapsulation. Furthermore he should also understand UML, Patterns, (Principles?). Kick the architect's ass when the design is wrong or the sysadmin's when the build- or test-server breaks. A good set of acronyms and buzzwords is also required. As you go up the chain more and more responsibilities are added: it's not like that when you are a lead developer you suddenly only get to do the cool stuff. You won't find much procedural code in an enterprise environment because OO is easier (cooler *AHUM*) to model. There is no real difference between an enterprise PHP developer and just some regular PHP-joe. As a programmer you should know what you are talking about regardless if you are working in an enterprise or not. Buzzwords are great to make you look cool but most don't truly understand what they are talkikng about (I'm not excluding myself here in any way). Every now and then you'll see a trend in buzzwords like Dependency Injection a while back. Although DI is great, it's not something new. The concepts behing DI go way back to 1980 as is common in our profession. Having a good working knowledge on different subjects and in different areas helps you to see through the BS. -
PHP 5 Objects, Patterns, and Practices by Matt Zandstra is still the best around I think: http://www.apress.com/9781430229254