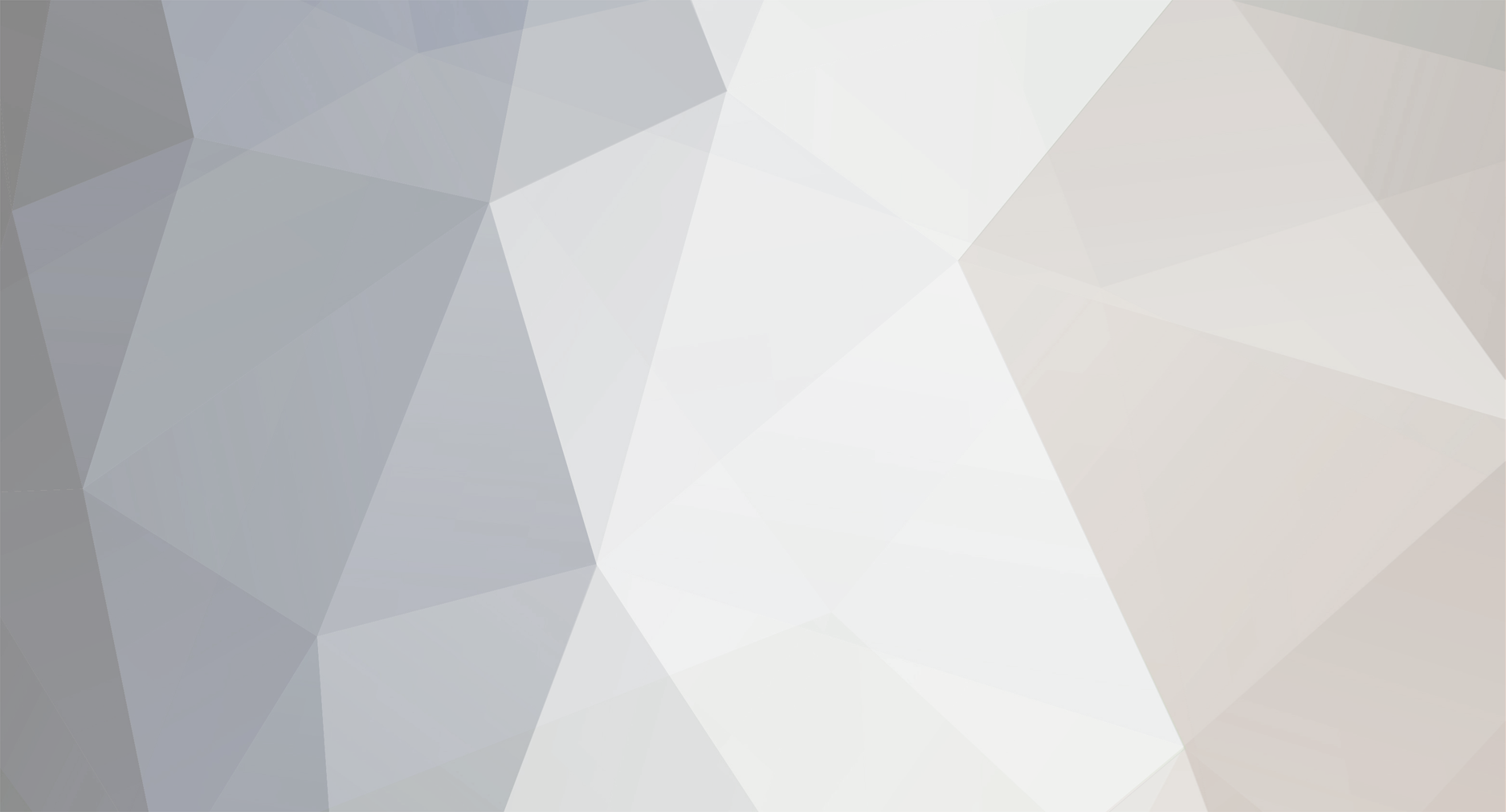
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
Your class has one major disadvantage. Consider the following scenario: you have a login form so you naturally create the methods to validate the data. Your website also has a registration form so you add in the extra validation.. your website has even some more forms and thus an equal number of validation methods, as your class is now 2 or 3k in lines you split them up into smaller chunks and you extend from a base validation class, validation methods that are needed across a number of classes are added in to the base class. However not all derived classes actually need the username/password validation but registration and login does so a lot of classes now have unneeded methods... Sure you can create another branch in your already huge validation hierarchy but maybe it can be simpler? So, how do you mitigate this problem? Let each validation rule be it's own class and have a separate class to chain them together: class Validate_Username implements Validate_Interface { // Username is a mix of validation rules public function isValid($username) { /* ..code.. */ } } class Validate_Password implements Validate_Interface { public function isValid($password) { /* ..code.. */ } } class Validator { public function addValidator($name, array $validator) { /* ...code... */ } } $validator = new Validator(); $validator->addValidator('firstname', array(new Validate_Strlen(6, 12), new Validate_InArray(array('John', 'Jane'))); $validator->addValidator('username', array(new Validate_Username())); $validator->addValidator('password', array(new Validate_Password())); if($validator->isValid($_POST)) { /* ..code.. */ } Instead of having one giant object full of methods that could possibly be needed you can now assemble the code/validation you need, removing pollution.
-
Why wouldn't you be allowed to use echo in your view or UI-layer? An application is divided up into 4 layers: Presentation, Application, Model, and Infrastructure. Presentation is your UI (HTML/CSS and the PHP to echo the passed variables or your template). Application knows about the Model and Presentation layer and makes the decisions regarding your application flow (like: performing file upload after form submission) The Model is the one who will actually perform the work (validating the upload, storing it in some directory, etc..) Infrastructure is your DB/Frameworks, everything that does not belong in the above 3 layers If you view an application as 4 different layers you'll be able to better determine what you can or "can't" do. It wouldn't make sense to echo stuff in your Application layer because why would you otherwise need the Presentation layer?
-
You can find a code sample for Zend_Acl at the Zend framework website (scroll down to example #1). Add your roles (instructor, admin) to the Acl object: $acl->addRole(new Zend_Acl_Role('instructor')); $acl->addRole(new Zend_Acl_Role('admin')); Then check against the acl if the user has access: $acl->isAllowed($identity->user_type, 'nameOfTheResource')) { The advantage of this approach is that your role names are dynamic (eg you can rename admin to Administrator without your code breaking)
-
The error means: user_id doesn't exist. So you probably forgot to assign it. PS: Zend also has a Zend_ACL component for all your access needs it makes this manual checking for instructor and administrator redundant. if($acl->isAllowed($user->getRole(), 'ResourceYouAreAboutToAccess')) {
-
Personally I think it has become so boring for you because you no longer think about creating cool stuff (make the client feel that working with your application is a breeze) and over-deliver but instead you think in snippets, you have become your own repository. During a meeting with a client your mind jumps from snippet to snippet and at the end you literally cut-and-paste the entire application together, you can almost automate this process as everything is a routine (lines 12-35 from file x paste at line 38-61 of file y) with some cunning you could (or hire someone to do it) tick/untick a few checkboxes and build your client's application. You have made yourself part of an assembly line that you have helped built. They also made a movie about this "force" where people who very very strongly believe in it can move stuff...
-
I thought I had already answered this question.
-
Whether you use a framework or not. Your code should always have a clear separation between retrieving and transforming and displaying information. Your application should transform the display to inform the user of it's state which could be as something simple as: class MyHTMLPage { public function showDialog($title, $message) { // do stuff } } $html->showDialog('No such username.');
-
The Registry pattern is a global object and thus best avoided. Martin Fowler himself notes it should be a last resort instead of your first weapon of choice. How is that abusive? A Registry is used to store objects.
-
The preferred ways are: composition: class Beta { public function __construct() { $this->alpha = new Alpha(); } } aggregation: class Beta { public function __construct(Alpha $alpha) { $this->alpha = $alpha; } } inheritance: class Beta extends Alpha {} As you notice $GLOBALS isn't part of them.
-
Like gizmola already pointed out error/exception handling and input handling are 2 different things. You don't have to crash your application because someone typed in wrong credentials. Instead, if using a Form class you could add the message to the form telling the user what went wrong or use some general messaging class to inform the user of your application's state: "wrong credentials", "profile updated", ... If you are using an MVC approach you can add it directly to your view: $view->showMessage('username/password match does not exist.');
-
add return $this; to hello() and world() remove the reference-operator (&) as objects are passed by reference by default. Sure it does, especially when passing big array's.
-
My initial introduction to programming was with COBOL and Assembler, my room just wasn't big enough to hold the computer otherwise. You don't have to. You can find CSS designs all over the net and use that as your base. Not sure this still holds truth but search engines have trouble properly indexing your website with frames. The problem with frames is that search engines index your navigation and content as different pages which means people can end up on your main content with no navigation or on a page with only navigation.
-
Well to say the least the website is seriously dated. Here are some resources to freshen your design: http://sixrevisions.com/tutorials/photoshop-tutorials/how-to-create-a-clean-web-20-style-web-design-in-photoshop/ http://www.freecsstemplates.org/css-templates/
-
I forgot to add the constructor, here it is again: class DbMySQLi { protected $connection; public function __construct() { $this->connect(); } public function connect() { $this->connection = new mysqli('localhost', 'root', '', 'login'); } } class Query extends DbMySQLi { private $result; public function runQuery() { $this->result = $this->connection->query('SELECT * FROM bla'); } }
-
public static function load() That solves your problem. If you pass an array with the first parameter being a string it is assumed to be a static call versus: $autoloader = new Autoloader(); spl_autoload_register(array(&$autoloader, 'load')); This will use the existing object and call a method load($class) upon it.
-
Because in your code you call: $this->connect()->query('SELECT * FROM bla'); If you don't return the value the above wouldn't work. You could rewrite it making the connection in the constructor or something and making the connection protected and call it like: $this->connection->query('SELECT * FROM bla'); Your code would look then like: class DbMySQLi { protected $connection; public function connect() { $this->connection = new mysqli('localhost', 'root', '', 'login'); } } class Query extends DbMySQLi { private $result; public function runQuery() { $this->result = $this->connection->query('SELECT * FROM bla'); } }
-
Your class can have multiple methods and class variables you just have to make sure the methods are cohesive (related) towards the class and one another.
-
It isn't, I just extended upon your example.
-
If you want to persist information between requests you should use sessions.
-
No, a class is a container of variables and functions. I could establish the DB connection in the constructor and all methods would have access to the DB connection.
-
public function getPage($p = $_GET['p']) Does not work as you are only allowed to specify static values. Depends on your opinion versus standards and best practices.
-
Include the code: $myclass = new myClass; $myclass->$variable = "hello world"; In the file myClass.class.php
-
You are not returning or saving the connection: class DbMySQLi { private $connection; public function connect() { if(is_null($this->connection)) { $this->connection = new mysqli('localhost', 'root', '', 'login'); } return $this->connection; } } class Query extends DbMySQLi { private $result; public function runQuery() { $this->result = $this->connect()->query('SELECT * FROM bla'); } } $query = new Query(); $query->runQuery();
-
I think you are referring to array_filter as array_values will just return all values from an array including zero's and other false-like values.
-
- In Latest Listings the description is cut-off which is fine but you should add a title attribute so the complete description is shown on hovering - If a user hovers over an item in Latest Listings stop the rotating and allow previous/next (I was hovering over an item for it's long title when it changed) - The image "No Fees, Sell 4 Free" loads very slowly, big image? Nice website!