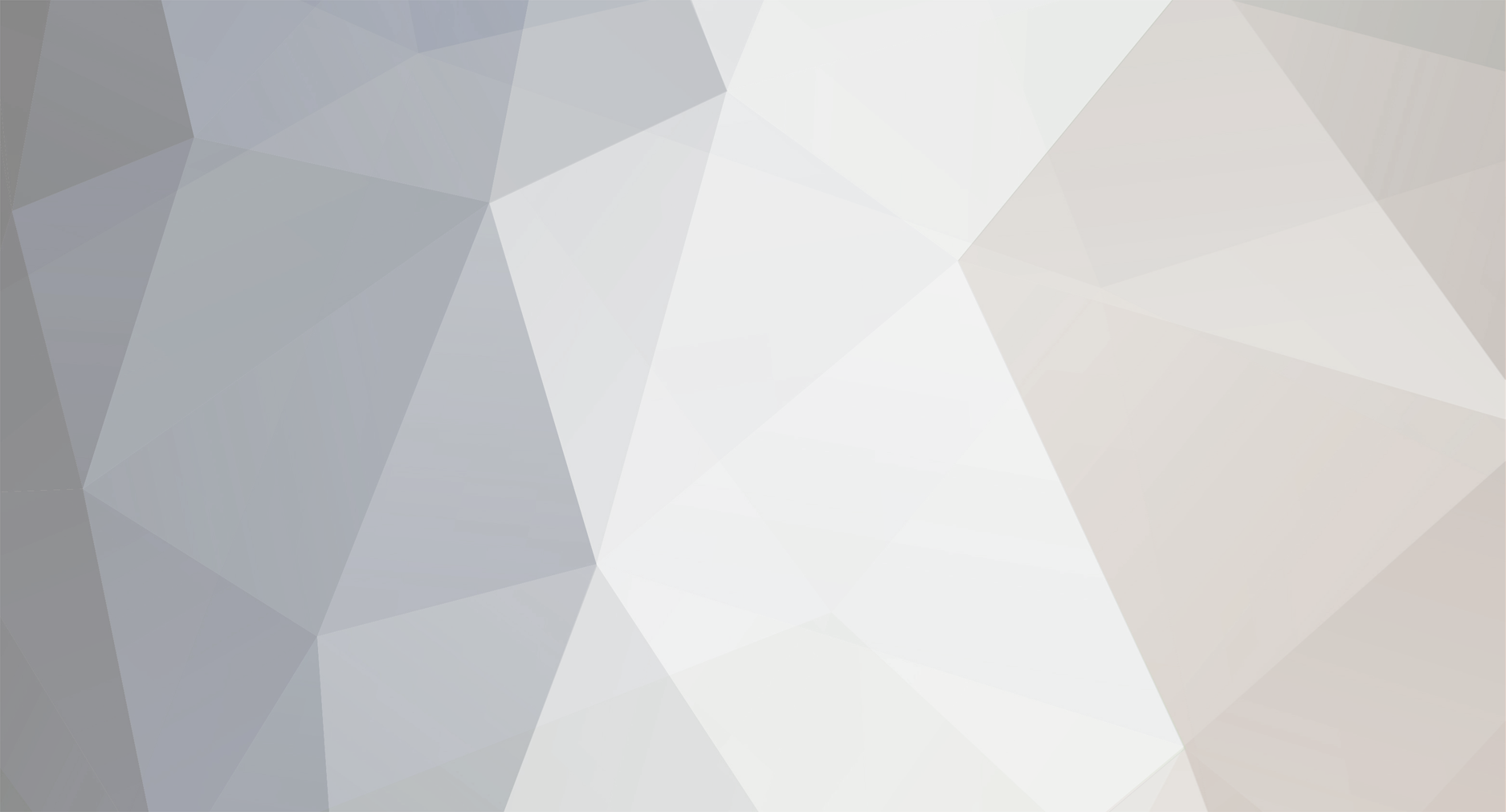
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
Hardcore oop enthusiast looking for appropriate framework
ignace replied to andrewgauger's topic in Other Libraries
It depends on your OO knowledge (principles, patterns) how well (or how bad) you'll get along with the Zend framework. I am currently playing with a few popular frameworks (Zend, CI, Cake, Symfony, ..) Our own CMS system runs on Zend, while one of our products runs on CodeIgniter I am going to regret this in the future, I'm sure. -
How exactly do you mean connect multiple servers? Why do you need these different servers in the first place?
-
Google BigTable, that algorithm has proven it's worth.
-
Hardcore oop enthusiast looking for appropriate framework
ignace replied to andrewgauger's topic in Other Libraries
IMO the most important decision you have to make is if you want a glue or a full-stack framework. A glue framework like Zend provides you with components which you can use either way you want and does not restrict you to use any of them. A full-stack like CodeIgniter (and Yii?) does the opposite, it restricts you to it's feature-set but it requires less effort on your end to write the application as bootstrapping and resource loading is all done for you (it runs out-of-the-box). I don't think there is much else I can add to this to be more relevant to you, download a few frameworks of which you think you like and try them, create something small, amuse the masses [ot]PS: Developers of the modern age get no respect. We are always the blunt of jokes, the first to blame, and people hardly talk to us. I wouldn't trade it for the world. -- love it [/ot] -
class CameraDAO { private $db; public function findById($id) { $row = $this->db->fetchRow('..'); return new Camera($row['id'], $row['price'], ..); } public function findByPriceRange($low, $high) { $rows = $this->db->fetchAll('.. BETWEEN $low AND $high'); foreach($rows as $row) { $cameras[] = new Camera($row['id'], $row['price'], ..); } return $cameras; } public function save(Camera $c) { $row['id'] = $c->getId(); $row['price'] = $c->getPrice(); .. $this->db->insert('table', $row); } } class Camera { private $id = 0; private $price = 0.0; private $angle = 0; } When you design a system you ideally want the classes to not know the names of the tables/columns because in the future these may be adjusted and for some reason (switch from MySQL to XML for example) these may not be the same which leads to the problem that you would have to find all occurrences of these column names in your classes. In the Java world they use a DAO (Data-Access Object) which fills the gap between your models and your database. As you can see only my DAO knows what the names of the columns are, my Camera class doesn't. All Camera knows is the attributes it shall have but not how it is named. For further information: http://en.wikipedia.org/wiki/Data_access_object http://en.wikipedia.org/wiki/Object-relational_mapping
-
UML is big, what is it exactly that they are asking? a class diagram? Have you got any experience creating ERD schema's? When you create a class diagram you think about the relations between each class (parent, child, contributor, ..). http://www.digilife.be/quickreferences/QRC/UML%20Quick%20Reference%20Card.pdf + = public - = private # = protected Composition = create the object inside an object Aggregation = create the object outside the object and pass it to the object paremeters are shown as: <variableName> : <variableType> the same goes for class variables <visibility> <variableName> : <variableType> association: A -----> B class A { private $B;// regardless if composition or aggregation } ---|> is inheritance (specialization)
-
Instead of: function Process(){ global $session; Use: function Process( Session $session ){ Which is a far better practice
-
If you are using this many objects already why not switch to OOP? Like before mentioned buy PHP Objects, Patterns, and Practice. Buy Domain-Driven Design afterwards. The first book will learn you how to program OOP, the second will tell you how to do it properly.
-
Your design is flawed. cURL has no direct relation to your Auction besides that cURL provides the service it provides (reading the HTML source, for which other tools are available). In the below example DomDocument provides the reading service, AuctionDAO does his part by parsing the source and translate it into an array. AuctionRepository stores an array of Auction's and facilitates data iteration. Auction is the representation of an auction. class Auction { // auction specific details } class AuctionRepository { private $auctions = array(); public function __construct( $auctions = array() ) { $this->addAll( $auctions ); } public function addAll( $auctions ) { foreach( $auctions as $auction ) { $this->add( $auction ); } } } class AuctionDAO { public function findById( $auctionID ) { $auctions = array(); if( $this->dom->loadHtmlFile( 'http://example.com/auction.php?id=' . urlencode( $auctionID ))) { $xpath = new DomXPath( $this->dom ); foreach( $xpath->query( '//auction' ) as $auction ) { $details = array(); foreach( $auction->getChildren() as $tag => $value ) { $details[$tag] = $value; } $auctions[] = $details; } } return $auctions; } } Explain what you are trying to do and what you want to achieve and we can further assist you.
-
I think this can be done BETTER in PHP and JavaScript.
-
I like the design but it misses the goal you are trying to achieve: sell websites. When you are focusing on web-design, you should have a stunning portfolio showcasing your skill and your success. For example http://www.albertlo.com/ -- this website makes prospecting clients confident this is the candidate they were looking for. A good design of course isn't everything eg http://www.gummisig.com/ -- great design but the text is a bit off.. What is it that you actually want to make? An online portfolio? An online resume? What is the goal? What do you want to achieve with this website? Try to find questions to further define your concept and then translate the answers to a design.
-
Smarty is not "garbage", you just need to understand it's non-OO plugin-system. That said though I dont use Smarty anymore.
-
Is it possible to change the color of the select? I've seen a website do it but couldn't figure out how (:select perhaps or something?) -- found it http://css-tricks.com/overriding-the-default-text-selection-color-with-css/
-
Post some screens and relevant code.
-
I agree, put some weight to your decision. CI is indeed a good choice as it has a small learning curve, the documentation is well-written and easy-to-understand. However CI and full-stack frameworks in particular are a bad choice when you in the future may switch from MVC to some other implementation MVP, MVVC or HMVC. ZF becomes the better choice when your development team already has a sound knowledge on OO methodology where-as CI almost entirely hides it.
-
Create a Smart-Object (select all layers including masks et cetera) of your original and create a copy. On your copy right-click edit contents, a new window opens, adjust as needed and save (the changes are reflected in your main document). Besides this advantage does it also allow you to stretch your graphic without loss of data (it does not become blurry and pixelated). This will also solve the other problems. PS It would help if you included an image of the current button so we can visualize your text.
-
if (1 <= $input['number'] && $input['number'] <= 20) // 1 <= x <= 20 { switch ($input['type']) { case 'square_root': $output = factory('square_root')->calc($input['number'])->toString(); // 'The square root of $input['number'] is $x' break; case 'square': $output = factory('square')->calc($input['number'])->toString(); // 'The square of $input['number'] is $y' break; case 'cube': $output = factory('cube')->calc($input['number'])->toString(); // 'The cube of $input['number'] is $z' break; } echo $output; } Should get you started.
-
$iterator = new RecursiveDirectoryIterator( new DirectoryIterator( 'path/to/directory' ) ); while ($iterator->valid()) { if ($iterator->isDot()) continue; echo $iterator->key(), "<br>\n"; } Play with it and modify (extend) to suit your needs.
-
Here's an article that explains encoding and issues: http://www.phpwact.org/php/i18n/charsets. If anyone has some better resources when it comes to encoding and stuff, please share.
-
Best advice ever!
-
When choosing a framework, you have 2 options: 1. You go for a glue-framework 2. You go for a full-stack framework The difference lies in flexibility and the way you use it. CodeIgniter, RoR and CakePHP are full-stack frameworks, they provide you with everything you need to get going fast. CodeIgniter has a small learning curve and beats CakePHP on performance. The catch with full-stack framework is that because they provide you with everything you need to get going fast, they also restrict you in freedom. It's for example not possible to switch from an MVC approach to some other architecture. A glue framework provides you with components that can be used individually and do not force you to use them in a specific way. Zend framework is a glue framework but it has a hard learning curve. You can't rely on the ZF manual for answers as not all components are documented, leaving you with a lot of questions. Not all of ZF's components will fit your application, which is normal but due to a low abstraction and absent refactoring will you find yourself copy-pasting a lot of a class internals only to change a few lines to fit your needs. Nevertheless ZF is the best PHP glue framework around. ZF is backed by a company and therefor you can find typical company support: 1. certification (ZF certified engineer exam) 2. training 3. consulting There are other glue frameworks around, some claim to be a glue framework while they are in fact full-stack frameworks. A limited knowledge on glue vs full-stack will make you find out the hard way.
-
echo $advertisers->advertisers->advertiser[0]->advertiser-id; If you use a syntax highlighting IDE then you should have noticed that advertiser-id showed the - as an operator (advertiser - id): advertiser-id
-
I think it would be best to have a CarImage with a class variable of type CarColor as that makes the most sense. This allows you to query the color of the car in the image. It does not make much sense to put the CarColor in the CarModel because as the CarImage changes, so does the CarColor.