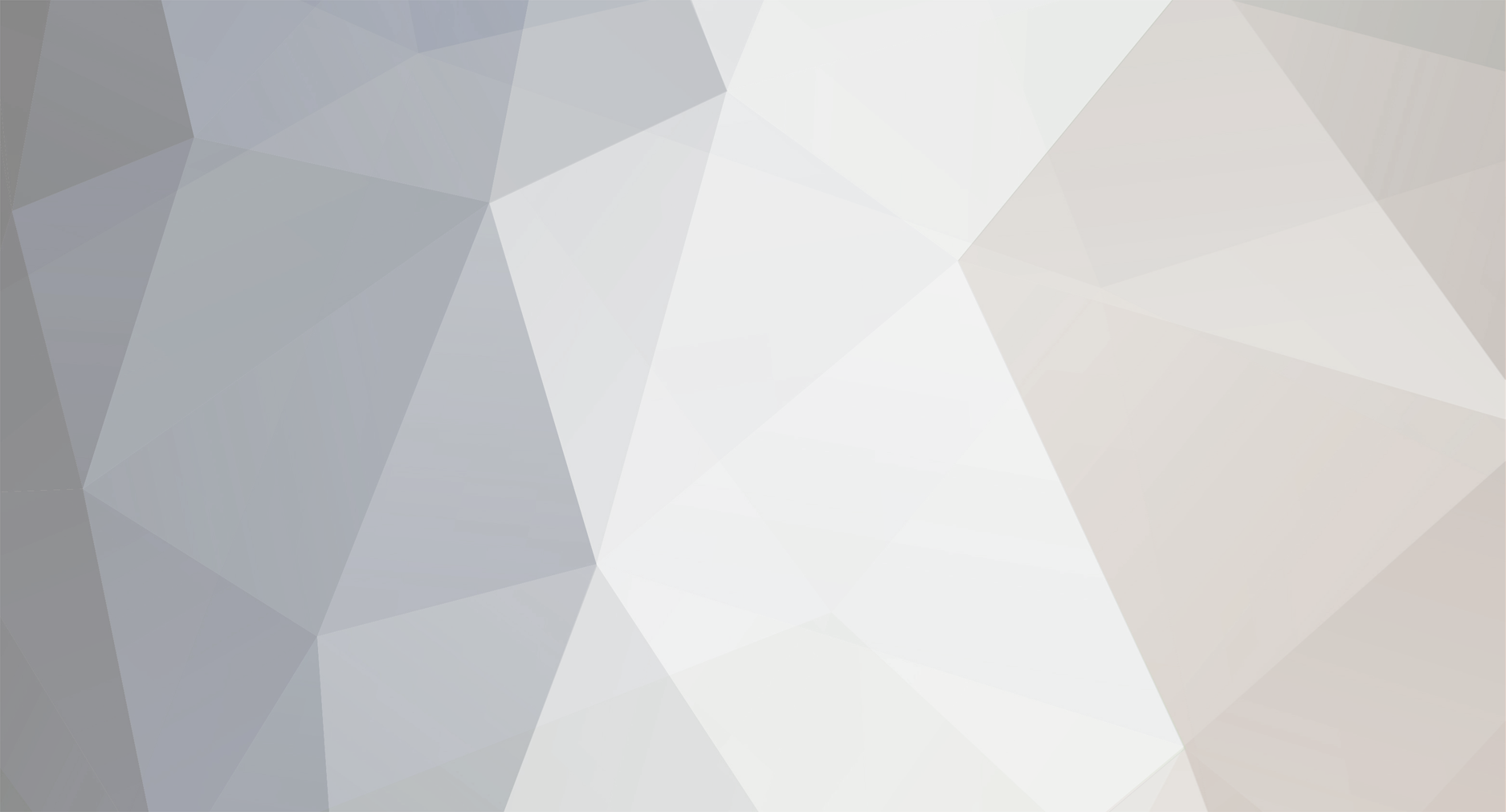
NotionCommotion
Members-
Posts
2,446 -
Joined
-
Last visited
-
Days Won
10
Everything posted by NotionCommotion
-
Okay, I add something to a queue <?php // Ajax file //Connecting to Redis server on localhost $redis = new Redis(); $redis->connect('127.0.0.1', 6379); $rs=$redis->rPush('stack', 'Hello!'); var_dump($rs); Now need to get it back. Reading http://redis.io/commands/rpoplpush, I don't wish to just pop it off as it is not reliable. Instead, I should use rpoplpush (or brpoplpush if I want it to be a blocking function which I do). So, I did the following and it appears to work. <?php //Consumer //Connecting to Redis server on localhost $redis = new Redis(); $redis->connect('127.0.0.1', 6379); $rs=$redis->bRPopLPush('stack','stack2',0); var_dump($rs); It is my understanding that the first argument to bRPopLPush is the variable name, and the last is the timeout time and I am thinking that 0 is indefinite. Correct? What is the second argument all about?
-
Thanks Kicken! and not 3.2 based on doing: yum install redis32u.x86_64? Also, yes, I see how I did in fact include a client. Turns out I needed to only add the following to php.ini. Probably questions later, but am now talking! extension = redis.so
-
I wish to use redis as a queue. The way I envision it working is an ajax call to a PHP script will push something on the queue, and then another PHP script will have a blocking command (i.e. http://redis.io/commands/brpoplpush) to receive the something, and deal with it. I've installed it as shown below. Note that I have not edited /etc/redis.conf or /etc/php.ini, nor installed any additional sorts of redis clients. yum remove redis.x86_64 yum install redis32u.x86_64 cat /etc/redis.conf yum install php56u-pecl-redis.x86_64 # Extension for communicating with the Redis key-value store yum install php56u-pecl-redis-debuginfo.x86_64 # Debug information for package php56u-pecl-redis yum install redis32u-debuginfo.x86_64 # Debug information for package redis32u #Requires tcl (already installed) chkconfig --add redis chkconfig --level 345 redis on phpinfo() shows redis 2.2.8as being installed. Why not redis3.2? Do I need a redis client? For instance, one of http://redis.io/clients#php? If so, which one? I've tried $redis = new Redis();, however, the class doesn't exist. What next? Thanks
-
Allow ability to NOT attach file in PHP mail form
NotionCommotion replied to spastickyle's topic in PHP Coding Help
Looks like your form name was "attach1", however, something is wrong with your form as you received error 4 (UPLOAD_ERR_NO_FILE) -
Allow ability to NOT attach file in PHP mail form
NotionCommotion replied to spastickyle's topic in PHP Coding Help
Hi spastickyle, While not a direct answer, the following will show you what the problem is. I typically start off with print_r() and the pre tags as I showed unless I am working with json in which I don't use the pre tags. Sometimes, you want more information, and should use var_dump() instead of print_r(). Try this, and display what it prints out. $files = array(); echo('<pre>'.print_r($_FILES,1).'</pre>'); foreach($_FILES as $name=>$file) { $file_name = $file['name']; $temp_name = $file['tmp_name']; $file_type = $file['type']; $path_parts = pathinfo($file_name); echo('<pre>'.print_r($path_parts,1).'</pre>'); $ext = $path_parts['extension']; if(!in_array($ext,$allowedExtensions)) { die("File $file_name has the extensions $ext which is not allowed"); } array_push($files,$file); } -
Thanks gizmola, I will checkout Redis. Isn't Websockets just sockets implemented with a web browser so that JavaScript can access them? If so, I am not sure if it is applicable.
-
There is so much I don't know about PHP! Thanks, let me look into it.
-
Yes, it does have a couple of faulty =s. Thanks. I kinda came up with this strategy myself (with you guys pointing me in the right direction), and whenever I solely come up with something, it always proves to have issues. Is what I am attempting to do fairly common? Never used inotify before, but looks interesting. I was just using a file as an example, however, and planned on using a database instead. What do you think would be best? I will have a maximum of 1,000 clients located behind firewalls and one server. The server will receive ajax requests which will contain the applicable client identifier and the desired action, and the server must get the desired action to the appropriate client.
-
Thanks kicken and requinix, Good point kicken about an Apache server being a specialized socket server. Maybe I knew that, but I didn't realize that Both of you recommend initially just a simple polling strategy. Okay, that is what I will do. But why make the user wait? I gave a narrative at the end of my last message, and started implementing it below. Do you see any issues? <?php // Script running as a service on computer located behind firewall $poll_time=300; //seconds $url='http://example.com/remote.php'; $data=['id'=>123]; if ($data) {$url = sprintf("%s?%s", $url, http_build_query($data));} $options=[ CURLOPT_URL=>$url, CURLOPT_RETURNTRANSFER=>true, CURLOPT_CONNECTTIMEOUT => $poll_time, CURLOPT_TIMEOUT=> $poll_time ]; $ch = curl_init(); curl_setopt_array( $ch, $options ); while (true) { if($rsp = curl_exec( $ch )) { if($error=curl_errno($ch)) { $rsp='Error: '.$error; } else { $code=curl_getinfo($ch, CURLINFO_HTTP_CODE); if($code=200) { //process results $rsp='Valid response from remote: '.$rsp; } elseif($code=204) { $rsp='No action required by remote'; } else { $rsp="Error $code response from remote: $rsp"; } } } else { $rsp='No response from remote'; } echo($rsp); //curl_close( $ch ); //should I close? } <?php // http://example.com/remote.php // This script is run by the webserver and is located outside of the firewall // If the user wants to send something to the computer located behind the firewall, the database will be updated with the appropriate content function getCommand($id) { /* Query DB and see if there is a command. If $id is invalid, return false If no commands, return NULL If command is pending, delete it from database and return it. */ // Fake database for example $file=__DIR__.'/database.json'; $database = json_decode(file_get_contents($file),true); if(!array_key_exists($id,$database)) { $rsp=false; } elseif(!$database[$id]){ $rsp=null; } else { $rsp=$database[$id]; $database[$id]=null; file_put_contents($file, json_encode($database)); } return $rsp; } $poll_slow=300; //seconds $poll_fast=250000; //microseconds if(!empty($_GET['id'])) { $code=204; //Maybe something else? $rsp=null; for ($i = 0; $i <= 1000000*$poll_slow/$poll_fast; $i++) { $rsp=getCommand($_GET['id']); if(!is_null($rsp)) { if($rsp===false) { $code=404; $rsp='Invalid identifier'; } else { $code=200; } break; } usleep($poll_fast); } } else { $code=404; $rsp='Missing identifier'; } header('Content-Type: application/json;'); http_response_code($code); json_encode($rsp);
-
Thanks for your patience and all your help. Sorry for being a little dense, but I've never needed to think through these workflows before and the inner workings of TCP/IP and BSD are still a mystery to me (yes, just like regex, I know I need to master them one day). Okay, I think I understand. More later. In a perfect world, instant, however, a few minutes would be acceptable. Current implementation.... Human utilizes a browser to interact to ComputerLocal indirectly by going through ComputerRemote. ComputerLocal polls ComputerRemote every 60 seconds to either send some data or ask how things are going. If ComputerRemote says human wants to talk, poll rate goes up to every 5 seconds until no new news for 300 seconds. ---------------------------------------------- Maybe I don't need sockets, and this is more simpler than I thought. ComputerLocal makes a cURL POST request whenever it wants to to ComputerRemote to send data, and ComputerRemote just replies back that it was received (and doesn't try to reply back that it has something new to say). ComputerLocal also independently makes a cURL GET request every 5 minutes? to ComputerRemote to ask whether ComputerRemote wants ComputerLocal to do anything. If ComputerRemote doesn't reply within 299 seconds, the request is somehow canceled, and the next GET request goes out one second later. If ComputerRemote responds, ComputerLocal processes the response and then initiates another cURL GET request without waiting for the 5 minutes to expire. Maybe???
-
Thank you requinix for your comprehensive reply. I also agree my confusion results in poor terminology. You earlier indicated that In networking terminology, the "client" is the one doing the connecting and the "server" is the one being connected to. I originally felt a client requests something and a server provides something regardless of who connects. So, if computerA connects to computerB and computerA waits for computerB to do something, computerA is still considered the client? Also, I am still confused regarding HTTP servers and socket servers. Originally, I was going to ask the question on how sockets deals with virtual hosts. But now I am starting to realize that both a webserver and a socket server can't be bound to the same IP and port else a client would get two different responses (okay, not "response" since this is a HTTP thing, but what do I call it for the socket server?). And then there are clients... Yes, I understand that a webbrowser is a HTTP client. I think that cURL is also a HTTP client, but it could also be a sockets client, right? And then one can build a sockets client using stream_socket_client() or the like. I guess this makes sense, but I am still confused when one transitions from one technology to another. Maybe if I better explain why I am doing this and what I am hoping sockets can provide, it will better uncover my misunderstandings. I have ComputerLocal located behind my customer's firewall and ComputerRemote located outside of it. ComputerLocal needs to regularly send data to ComputerRemote. ComputerRemote needs to every great while send data to ComputerLocal. Note that ComputerRemote also operates a HTTP server with several virtual hosts for other purposes. The firewall is maintained by my customer's IT group, and while they are friendly, they are not willing to change any configuration settings as a mater of principle. Outgoing on Port 80 is allowed, however, direct access of ComputerLocal from outside the firewall is not. My first thought was to add a new virtual host to ComputerRemote. ComputerLocal would periodically perform a cURL request to https:// NewVirtualHostOnComputerRemote.com:80 and send the data, and if ComputerRemote happens to have something queued up which it needed to send to ComputerLocal, it would take the opportunity to send it in the reply. Okay, this works, however, it is kind of a kludge. Furthermore, ComputerRemote needs to wait to be polled by ComputerLocal before sending the data. Is this a good opportunity for sockets? By the way, a finite number of connections is okay. How would one implement it? For instance... ComputerLocal still periodically perform a cURL request to https:// NewVirtualHostOnComputerRemote.com:80 and sends the data? ComputerRemote has a sockets server bound to Port 80 and receives the request and stores the data? Note that should a HTTP server be require, a new server will be required to host it since Port 80 is now being used by the sockets server. Every time ComputerLocal is sending the data, it ensures that a sockets server? client? is working and bound to Port 80? ComputerRemote sends a message to ComputerLocal whenever necessary? Yikes, those last 4 steps are confusing me more!
-
Hi kicken, Your reply snuck by my and I didn't see it until now, but do feel it will be useful going over, and appreciate you sending it over. Why do you recommend using a library instead of creating from scratch, and if a library, any recommendations of a good one? EDIT. I see you referenced http://reactphp.org/ in this post: https://forums.phpfreaks.com/topic/302371-php-client-to-periodically-make-curl-requests-to-other-servers/ Thanks
-
I've since read how http://php.net/manual/en/function.socket-accept.php will halt the code until a response is received. Ah, this makes sense. I assume http://php.net/manual/en/function.stream-socket-server.php is the same even the documents do not say so? But to implement this, there seem to be so much more required. How are multiple connections dealt with? If communication is lost, how is it reestablished? How does the client be able to continue operating as a client (through the use of a timeout?) instead of just entering an eternal state of listening? Will I just be reinventing the wheel, or do you know if there are existing classes?
-
Thanks requinix, I agree with your three options, and wanted to investigate your option b (A establishes a persistent connection to B and waits for activity). Would a "persistent connection" be a socket? Should A establish a stream_socket_client() or stream_socket_client() connection? Would some sort of endless loop on A be need to allow it to "wait for activity"? When A sends a request to B, typical firewall configurations will allow B to reply to A, and will this behavior persist? This is all very new territory for me, and I would appreciate any advice.
-
Never used sockets, and would like some advise whether I am on the right track. PHP Client A (or maybe will be changed to Python or something else) is behind a fire wall with reasonable settings, has a dynamic IP, and periodically makes cURL or sockets requests to PHP Server B. Later, Web client C makes a request to PHP Server B. How can I forward Web Clients C's request to PHP Client A? Also, should I be using the stream_ sockets functions? Thanks PHP Client A | FIREWALL | PHP Server B | Web Client C
-
Extracting only specific array elements
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Or array_flip() -
Extracting only specific array elements
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
You beat me by 1 second! Would you recommend doing it your way if there are only a couple of elements, but using array_intersect_key() if there are more than say 5? -
Extracting only specific array elements
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Obvious being $clients[]=['name'=>$source['name'],'guid'=>$source['guid']]; with maybe some isset checks? -
Recommendations on the best way to extract only specific elements from an array? What about the following? Thanks while($source=$stmt->fetch(\PDO::FETCH_ASSOC)) { if($source['guid']) { $clients[]=array_intersect_key($source, ['name'=>null,'guid'=>null]); } else { $servers[]=array_intersect_key($source, ['name'=>null,'ip'=>null,'port'=>null,'encrypt_key'=>null]); } }
-
Contact Form sends only 'message' field info only ...
NotionCommotion replied to Chrisj's topic in PHP Coding Help
You said: What does that mean to you? It means to me... First, I should do one more (or even more) reality checks of what the client is sending to the server to ensure what I am witnessed is reality. 80% of the time it isn't. Assuming 1 passes, this has nothing to do with the server (unless it is supplying faulty HTML), and I should be looking at the client (i.e. the form and/or js). I shouldn't be going down a rabbit hole troubleshooting the email class as it is not the issue, but should focus solely on the client. That I should definitely vote against Trump as the script will not run correctly if he is elected. -
How to make php.ini changes stick?
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Perfect! This works: sudo /etc/init.d/php5-fpm restart -
Contact Form sends only 'message' field info only ...
NotionCommotion replied to Chrisj's topic in PHP Coding Help
Sorry, meant $_POST. My point is to break your application up and test each part. -
How to make php.ini changes stick?
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
No, I expect my init.d script doesn't do more. In /etc/nginx/sites-available/default, I replaced server{ ... } with the following, and thus suspect I am using PHP-FPM, however, confess I am just guessing. server { listen 80; server_name $domain_name; root /var/www/html/; index index.html index.htm index.php; access_log /var/log/nginx/access.log; error_log /var/log/nginx/error.log; location ~\.php{ fastcgi_pass unix:/var/run/php5-fpm.sock; fastcgi_split_path_info ^(.+\.php)(/.*)$; fastcgi_index index.php; fastcgi_param SCRIPT_FILENAME $document_root$fastcgi_script_name; fastcgi_param HTTPS off; try_files $uri =404; include fastcgi_params; } } -
Contact Form sends only 'message' field info only ...
NotionCommotion replied to Chrisj's topic in PHP Coding Help
I'm kind of old school, and like to break it into pieces. Your problem is either html, js, php, networking, etc, etc. Start ruling things out. In your server script, add var_dump($_POST). What do you get? And why are you using file_get_contents("php://input") instead of the superglobal $_PRINT? -
Often I use Centos on a physical or virtual server with Apache. My normal operation is edit php.ini and then do /etc/init.d/httpd restart. Found myself using Raspian on a Raspberry Pi with nginx, so tried /etc/init.d/nginx restart, but it didn't take, and I had to reboot the server. Really, wasn't so easy, and it took me over an hour to try this How should changes to php.ini be made active?