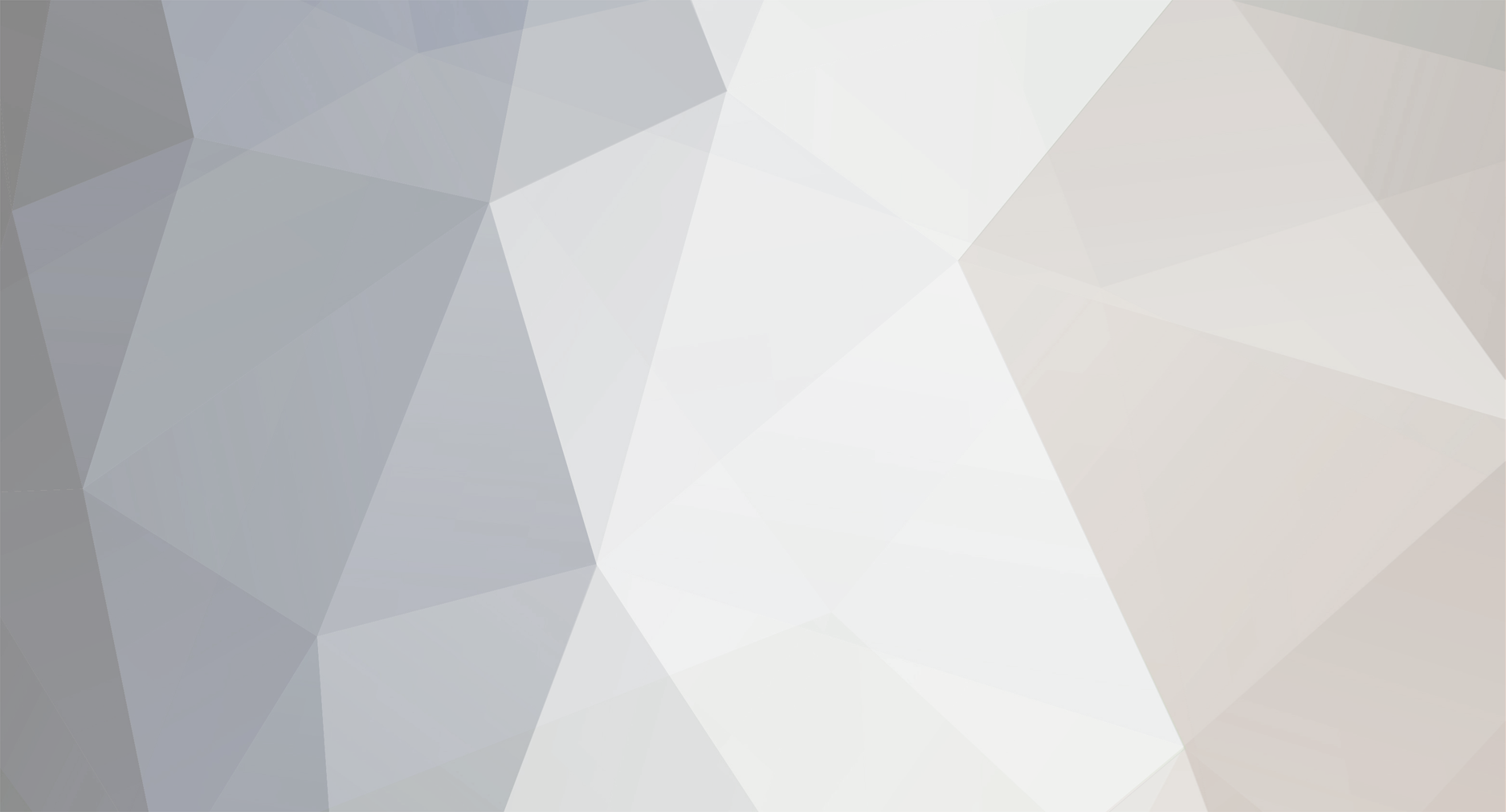
NotionCommotion
Members-
Posts
2,446 -
Joined
-
Last visited
-
Days Won
10
Everything posted by NotionCommotion
-
Is it worth storing database results in a session
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Wouldn't dream of doing so in practice, and only did so to limit the clutter for this post and focus the responses. Guess it didn't work... SELECT a.x, a.y, b.x AS b_x, c.c, e.bla, f.bla AS blabla FROM a JOIN b ON b.id=a.b_id JOIN c ON c.id=b.c_id JOIN d ON d.id=c.d_id JOIN e ON e.id=d.e_id JOIN f ON f.id=e.f_id WHERE a.something=123; -
Originally, I was going to say this question belongs in the MySQL forum and not the PHP forum, but maybe not. Why does the data in your database have a backslash before the apostrophe?
-
Let's say I have the following query, all tables are properly indexed, the resultant data is needed for every initial load and ajax request, and the associated data rarely changes: SELECT * FROM a JOIN b ON b.id=a.b_id JOIN c ON c.id=b.c_id JOIN d ON d.id=c.d_id JOIN e ON e.id=d.e_id JOIN f ON f.id=e.f_id WHERE a.something=123; Instead of running the query every request, I decided to store the results in a session. To know if the session was stale, I added a datetime column to one of the tables, and updated the value using the application whenever one of the six tables changes. I then would just first execute SELECT updated FROM a WHERE something=123;, and only use the big query if the updated value was different than the session value. Recently found things not working as expected, and later found that I forgot to update the a.updated value when one of the column values changes in one of the joined tables. Any advice from seasoned professionals whether it is worth trying to take steps such as these? If so, would you recommend using triggers? Don't think this would have even helped me on my earlier faux pas as the column which changed was also a new column, but probably better than using the application. Or maybe I should be doing this completely some different way?
-
Please elaborate. How would this work? My approach would just do the following: $sql='INSERT INTO myTable (a,b,c,d,e,f) VALUES (?,"foo","bar",NOW(),FALSE,TRUE), (?,"foo","bar",NOW(),FALSE,TRUE), (?,"foo","bar",NOW(),FALSE,TRUE), (?,"foo","bar",NOW(),FALSE,TRUE), (?,"foo","bar",NOW(),FALSE,TRUE), (?,"foo","bar",NOW(),FALSE,TRUE)'; $stmt = $conn->prepare($sql); $stmt->execute(array(4,3,6,9,6,3,6));
-
Normally, I use PDO and make a separate query for each insert. Started thinking maybe it would be best to insert all records with one query. Any advise? Thank you <?php $insert=array(4,3,6,9,6,3,6); $stmt = $conn->prepare('INSERT INTO myTable(a,b,c,d,e,f) VALUES (?,"foo","bar",NOW(),FALSE,TRUE)'); foreach($insert as $id) { $stmt->execute(array($id)); } ?> <?php $insert=array(4,3,6,9,6,3,6); $sql = 'INSERT INTO myTable(a,b,c,d,e,f) VALUES '; foreach($insert as $dummy) { $sql.='(?,"foo","bar",NOW(),FALSE,TRUE),'; } $sql=rtrim($sql,','); $stmt = $conn->prepare($sql); $stmt->execute($insert); ?>
-
Thanks Grissom, Yes, there are many, many, many solutions out there! One benefit I like about FILTER_VALIDATE_EMAIL is that it will be updated whenever I update my PHP version. I agree it will not validate whether the email is valid, and tested so just to be certain. Good point about testing for popular disposable e-mail accounts.
-
Hi ChOcu3r. So, I "could", and probably "should" use filter_var, correct? Are there any methods that are considered buggy? As I tried to example, $name is only used with other methods such as the following three: protected function required($x,$true,$name) { return (!$true || trim($x))?false:$name.' is required.'; } protected function minlength($x,$minlength,$name) { return (trim($x) && strlen(trim($x))<$minlength)?$name.' requires '.$minlength.' characters.':false; } protected function maxlength($x,$maxlength,$name) { return (trim($x) && strlen(trim($x))>$maxlength)?$name.' allows no more than '.$maxlength.' characters.':false; } In regards to !$true and !trim(), the first arguement follows jQuery validator plugin structure, and !trim() prevents it from validating as false if the variable is empty. Returning false if the variable validates allows a non-false response (i.e. the message) to be returned.
-
A while ago, I created a validation class which includes a bunch of methods to validate various types of data. For instance, the method to validate an email looks like: protected function email($x,$true,$name) { //$name isn't used with the email method, but is used with other methods to return a response like "$name is not a bla" return (!$true || !trim($x) || preg_match('/^[_a-z0-9-]+(\.[_a-z0-9-]+)*@[a-z0-9-]+(\.[a-z0-9-]+)*(\.[a-z]{2,3})$/i', $x) )?false:'Invalid email.'; } I recently started looking into http://php.net/manual/en/book.filter.php. Looks like I could do the same thing using: protected function email($x,$true,$name) { return (!$true || !trim($x) || filter_var($x, FILTER_VALIDATE_EMAIL) )?false:'Invalid email.'; } Assuming I am running PHP version 5.5.24, Should I be using the extention all times when available? Anything I should keep an eye out for? Thanks
-
Thanks CroNIX, Yes, I am doing so in my original post.
-
Thanks Muddy, Whether a DB or a flat file, I still need to detect the error and run some script, no? So I would just replace error_log ($message); with a query. Am I missing something? In regards to whether I display a nice page or don't even inform the user something went wrong, I am only displaying the nice page if it is a fatal error, uncaught exception, or normal error if not a notice or greater than 2047.
-
Hoping to get some advise regarding handling errors. Consider the following script. Is there any reason to explicit log errors in my_shutdown_handler? They seem to get logged automatically using the default error message. Is it typical to redirect to some static page such as error.html which politely informs the user that an error occurred, or should I be doing something else? Any other recommended changes to this script? <?php define('DEBUG',0); class start { public function __construct() { ini_set('display_errors', 0); error_reporting(E_ALL); register_shutdown_function(array($this,"my_shutdown_handler")); set_error_handler(array($this,"my_error_handler")); set_exception_handler(array($this,"my_exception_handler")); } public function my_shutdown_handler() { $error = error_get_last(); if ($error['type'] == E_ERROR) { $message = "An error occurred in script '{$error['file']}' on line {$error['line']}: {$error['message']}."; error_log ($message); //Is this necessary, or is it automatically called with critical errors? //Email error if desired if (DEBUG) {exit($message);} else {header('Location: /error.html');exit;} //Maybe don't go to a separate URL? } } public function my_error_handler($e_number, $e_message, $e_file, $e_line, $e_vars) { if (ini_get('error_reporting') != 0) { //Will be 0 if ignored using @ $message = "An error occurred in script '$e_file' on line $e_line: $e_message (error no: $e_number)"; error_log ($message); //Email error if desired if (DEBUG) {exit($message);} elseif ( ($e_number != E_NOTICE) && ($e_number < 2048)) {header('Location: /error.html');exit;} } } public function my_exception_handler($e) { $message = "An error occurred in script '{$e->getFile()}' on line {$e->getLine()}: {$e->getMessage()}. Exception code: {$e->getCode()}."; error_log ($message); //Email error if desired if (DEBUG) {exit($message);} else {header('Location: /error.html');exit;} } } $obj=new start(); //$x=$y; //throw new Exception('Division by zero.'); $obj->missingMethod(); echo('<p>done</p>'); ?>
-
No, nothing I'm not telling you No universal target. Just a reply to a normal ajax request. Still the server needs to inform the client that the server is not fond of the request.
-
Yes, an ajax request. Should have said so. Some jQuery plugins (x-editable for instance) have an error callback and are built to display a error message when receiving any response other than 200. This allows the server to do the following: http_response_code(400); echo('Your input must be between 40 and 80 degrees'); If desired, one could set up the error callback to display a general service unavailable message if code 500 is received. Alternatively, I am sure I could have the server return something like: echo(json_encode(array('status'=>0, 'error'=>'Your input must be between 40 and 80 degrees'))); and then use the plugins success callback to present the error. Just trying to better understand the implications of both approaches.
-
Exactly. Just looked into 422. Do you know if it tells the client to not try the identical request again? Was my concern about a 400 code doing this correct?
-
The user wouldn't see the header unless they had Firebug or a console open. The client would get the header, and would provide a user friendly response.
-
I've seen some client applications which use the server to respond with an HTTP header other than 200 to indicate that a request did not pass validation. It appears that a 400 header is the correct one to return. It appears that if the browser made a request, it did not pass validation and thus received a 400 header, it would not attempt to perform the request again without some modification. Is this correct? Is the request cached by the browser? Reference: https://tools.ietf.org/html/rfc7231#section-6.5.1 Reference: http://www.w3.org/Protocols/rfc2616/rfc2616-sec10.html#sec10.4.1 I then considered that validation is not always permanent. For instance: User submits a date after today, and the request doesn't meet validation today but will so in the future. User submits multiple identical requests which must have some minimum time span between them, and fails the validation preventing them from making the same request in the future. Business rules are relax so that the previous request which didn't pass validation now does. User submits a record which must be unique, attempts to submit the same record which now fails because it is not unique, deletes the record, and then attempts to re-submit the record which should no longer fail the unique constraint. Probably a lot more... Are my concerns valid? If so, are there other headers which should be used other than 400 which will allow the client to repeat the request? Also, will the client receiving a 400 status cause tracking solutions such as qbaka, trackjs, etc to log the error as a JavaScript error? I look forward to hearing any real world experience or sound hypothetical advice regarding this. Thank you
-
Maybe http://en.wikipedia.org/wiki/Primality_test
-
MySQL's LIMIT?
-
Doubling after getting data from MySQL?
NotionCommotion replied to canadezo121's topic in PHP Coding Help
Break it up in pieces. At first, forget you fancy HTML, and just view the results provided by your database. When you know what you are getting, then deal with presentation. Use functions like var_dump($yourstuff), print_r($yourstuff) or my personal favorite echo('<pre>'.print_r($yourstuff,1).'</pre>'). -
Proper way to create JavaScript object using PHP
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Depends which part of my application I have similar need multiple places. Sounds like you recommend that if the data is static, directly output the data as a JavaScript object, right? -
I've flip-flopped on this topic for a while. I need some data available to the client which is generated using PHP. Below are two options to do so. Is one preferred over the other? Is there a better option? Thank you <!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <title>Generating Serverside Generated JavaScript Object</title> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.2/jquery.js" type="text/javascript"></script> <script> //Option 1: Generate the appropriate JavaScript directly using PHP var myJSONObj_1=<?php echo json_encode(array('a'=>1,'b'=>2,'c'=>3, 'd'=>array('x'=>1,'y'=>2,'z'=>3)));?>; $(function() { //Add all my JavaScript here... console.log('myJSONObj_1 test 1',myJSONObj_1); }); </script> <script> $(function() { //Option 2: Server to just provide JSON $.getJSON( 'getMyJSON.php', function(myJSONObj_2) { //Add all my JavaScript here... console.log('myJSONObj_2 test 1',myJSONObj_2); }); }); </script> <script> //Some other imported script //No problem here console.log('myJSONObj_1 test 2',myJSONObj_1); //Opps, not so good, anything we could do? console.log('myJSONObj_2 test 2',myJSONObj_2); </script> </head> <body> </body> </html> getMyJSON.php <?php header('Content-Type: application/json;'); echo json_encode(array('a'=>1,'b'=>2,'c'=>3, 'd'=>array('x'=>1,'y'=>2,'z'=>3))); ?>
-
Doubling after getting data from MySQL?
NotionCommotion replied to canadezo121's topic in PHP Coding Help
What do you mean by "pulling the identical information twice"? It is obviously displaying two <li> elements for each record. What I like to do is get rid of all your HTML, and just display what is being returned from the databases. You are using the obsoleted PHP MySQL functions, and you should use the new improved ones or better yet PDO.