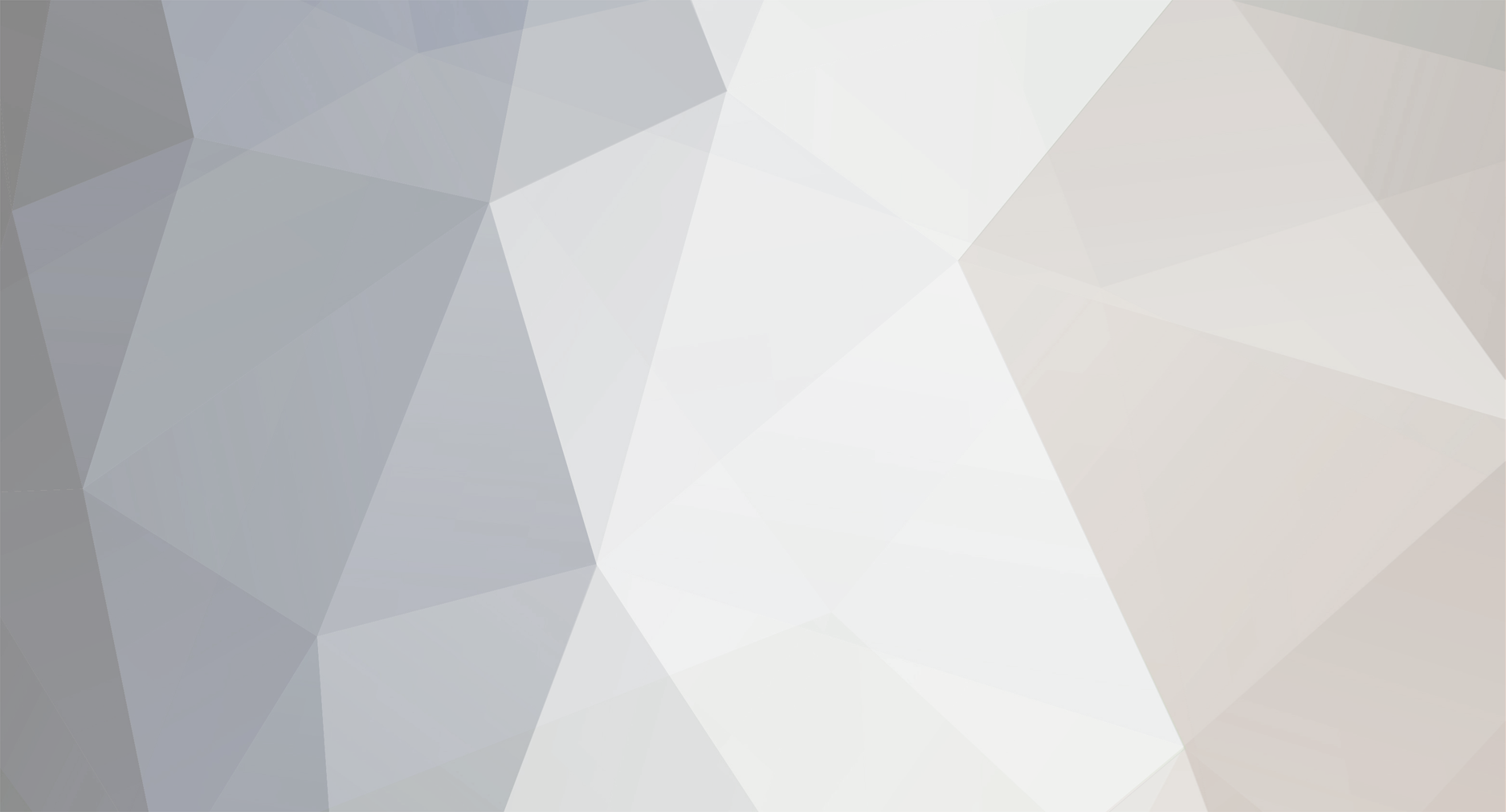
NotionCommotion
Members-
Posts
2,446 -
Joined
-
Last visited
-
Days Won
10
Everything posted by NotionCommotion
-
Who uses ON DELETE CASCADE in a PHP application?
NotionCommotion replied to NotionCommotion's topic in MySQL Help
Yea, my first two statements with an "Agree?" question were more of a SQL question. Added them trying to give context, but probably shouldn't have. But the last part was where I need help. You imply that not using ON DELETE CASCADE results in highly visible, easy to change, one place in code. Presumably, using ON DELETE CASCADE may result in the opposite? Not trying to go round and round in a debate; just trying to better cement my own approach. I believe that it does matter who executes a query. If I have multiple applications (or even more relevant, multiple people) performing queries, then ON DELETE CASCADE likely makes sense. Do you know of any other reasons why one would recommend using ON DELETE CASCADE? -
This is more of a PHP question (or at least an application question) and not really a SQL question, so please read on. First, would like some opinions of my assumptions... ON UPDATE CASCADE should typically be used on natural keys but never on surrogate key regardless of whether a human or PHP is executes the queries as the natural key values may be changed in the future. ON DELETE CASCADE should typically be used on surrogate keys where a human executes queries, but most likely never on natural keys regardless of whether a human or PHP executes the query as it just seems wrong. Disagree? If so, why? So, my question.... Should ON DELETE CASCADE (or maybe even ON DELETE SET NULL for that matter) ever be used when PHP solely executes the queries? Please provide rational why or why not. Thank you
-
Permission denied to make directory
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Well, I feel silly. PHP is being ran as apache. Probably better to use something like www-data so it is simpler to change websevers if desired. Still odd that I earlier wasn't able to create the directory. I changed permissions to 0775, and apache belonged to the NotionCommotion group. Oh well, all is good now, and probably best not to use groups like I did for this. -
Permission denied to make directory
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Yes, I recognize that permission is denied. Why? As I showed on the original post, the parent directory is 0755 and is owned by the PHP user. -
I am sure it is a silly mistake, but don't see it. Can anyone tell me why I cannot create a directory? Thanks echo('filepath1: '.$filepath1.'<br>'); echo('filepath2: '.$filepath2.'<br>'); echo('fileowner: '.posix_getpwuid(fileowner($filepath1))['name'].'<br>'); echo('fileperms: '.substr(sprintf('%o', fileperms($filepath1)), -4).'<br>'); echo('current_user: '.get_current_user().'<br>'); echo('filepath1 exists: '.(file_exists($filepath1)?'Yes':'No').'<br>'); echo('filepath1 is a directory: '.(is_dir($filepath1)?'Yes':'No').'<br>'); echo('filepath2 exists: '.(file_exists($filepath2)?'Yes':'No').'<br>'); if(!file_exists($filepath2)){mkdir($filepath2, 0755);} filepath1: /var/www/main/user_resources/documents/30 filepath2: /var/www/main/user_resources/documents/30/01 fileowner: NotionCommotion fileperms: 0755 current_user: NotionCommotion filepath1 exists: Yes filepath1 is a directory: Yes filepath2 exists: No An error occurred in script '/var/www/main/ application/classes/application.php' on line 197: mkdir(): Permission denied (error no: 2). [12.34.56.789]
-
Agree a port needs to be open to hear through that port. Doesn't websockets by default use 80 and 443? I don't claim to know, and base this on https://en.wikipedia.org/wiki/WebSocket. If so, could a server that responds to websockets not act as a webserver? Needless to say, I am very new to sockets!
-
Thanks 0X00, Sorry for being cryptic, but I am not allowed to communicate the application. What I heard you say is "yes, this is possible, but not really with PHP and will require some lower level language such as C, etc." If I misread you, please advise. PS. I like your comment "All networks are based on sockets, e.g. Berkley Sockets, WinSock, etc..." It confirms what I somewhat already suspected. Thanks again, Michael
-
Yes, I totally agree. I tried to make that clear in the original post. Assuming that server B initiated the connection, will http://php.net/manual/en/book.sockets.php work? My initial review indicated that they are not sophisticated enough to pass header information to return to originating client. If not, how can this be accomplished?
-
Don't want port forwarding or a DMZ. For arguments sake, lets say Server B is located behind my home router, and my router has a semi-static public IP as defined by my IP provider. If I am using a browser behind my home router, it is my understanding that I could initiate a websocket to a server, and later receive pushes from that server. If a browser could do it, why could it not be done without one?
-
But websockets uses port 80/443 by default, no? How does it accomplish it?
-
Maybe something like the following... <?php $data=array(); $query=''; foreach(array('name','phone') as $key) { if(isset($_POST[$key]) && $_POST[$key]!=''){ $data[$key]=$_POST[$key]; $query.="$key=:$key,"; } } if(!empty($data)){ $sql = 'UPDATE myTable SET '.trim($query, ",").' WHERE id=:id'; $data['id']=123; $q = $conn->prepare($sql); $q->execute($data); }
-
Thank you Scootstah, No, there is no web browser client, and I will check sockets out. Something I've always planned on doing, but never really did in earnest. Note that assuming the firewall is configured to allow communication on standard ports such as 80 or 443 is acceptable, but setting up port forwarding to a given local IP is not. It sounds like I am okay in this regard, but if you feel differently, please advise. Thanks again! EDIT. Will some network configurations prevent this? Somehow Server A needs to get back to Server B, and without port forwarding or equal, the local IP needs to be somehow bundled in the response.
-
I have two server/clients. Server B initiates communication to Server A. Afterwards, both servers need to be able to initiate communication to one another. The router in front of Server B should not require any special configuration such as port forwarding. Is this possible using PHP? I recognize maybe PHP is maybe not the preferred language, but wish to use it as proof of concept. If possible, please provide very high level steps on how it can be accomplished. Thank you
-
You will also need to use $_SESSIONs and password hashing (http://php.net/manual/en/faq.passwords.php) Also, for better security, look into using a single atomic operation (http://forums.phpfreaks.com/topic/293061-validate-username-and-password/?p=1499458)
-
I have three classes which each have their own method "pm()". Each of them call mainMethod(), and pass $a and $b. All of them also need to pass a value for x and y except for one that also needs to pass a value for z, and as such, I was planning on passing an array for these "extra" values. mainMethod is the same for all of them except one small section. It is possible that mainMethod() will be called by more than once in a given class and will require different functionallity for this "small section". Originally, I planned on having the pm() methods pass mainMethod() an anonymous function, but have since learned the error of my ways. I recognize I am not providing "all the information", but am hoping that I have provided enough to get advice whether I am doing this the right way. Thanks <?php class class1 extends mainClass { public function pm() { //some script $extra=['x'=>1,'y'=>2]; $this->mainMethod($a,$b,'pm_callback',$extra); } protected function pm_callback($extra,$n,$m) { //some script that uses $this->model, $extra, $n, and $m } } class class2 extends mainClass { public function pm() { //some script $extra=['x'=>1,'y'=>2]; $this->mainMethod($a,$b,'pm_callback',$extra); } protected function pm_callback($extra,$n,$m) { //some script that uses $this->model, $extra, $n, and $m } } class class3 extends mainClass { public function pm() { //some script $extra=['x'=>1,'y'=>2,'z'=>3]; $this->mainMethod($a,$b,'pm_callback',$extra); } protected function pm_callback($extra,$n,$m) { //some script that uses $this->model, $extra, $n, and $m } } class mainClass { protected function mainMethod($a,$b,$callback,$extra) { $model=new model(); //some script $this->$callback($extra,$n,$m); //some script } } ?>
-
So don't even do them? Or do them, and know they are not comprehensive and will only deter amateurs?
-
Why?
-
I wish to make it difficult to upload files except for given types such as Word/Excel/PDF or whatever. Yes, I will reject it if MIME doesn't match either $_FILES['...']['type'] or the extention or both. The purpose it to provide reasonable protection to other users which might download the files. Users will download the files directly from the web server (or maybe XSendFile if I am using Apache) and it will need to be configured to not parse PHP so I don't think I have “application/dangerous-php-script” risk.
-
Both being $_FILES['...']['type'] and mime, or file extention and mime? On a related side note, do you know of a maintained class that quickly takes into account $_FILES['...']['type'], extention, and $_SERVER['HTTP_USER_AGENT'] to pre-screen files?
-
I have the following amazing script. I see that the file type is "application/vnd.ms-excel". I will later use FILEINFO_MIME_TYPE to make extra sure. My question is whether I should even look at $_FILES['myFile']['type']. It come from the client, right? furthermore, I've seen some browsers provide different types. Maybe best to go just off extention and mime? <?php echo('<pre>'.print_r($_FILES,1).'</pre>'); ?> <!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <title>Testing</title> </head> <body> <form method="post" enctype="multipart/form-data"> <input type="file" name="myFile"> <input type="submit" value="Upload"> </form> </body> </html> Array( [myFile] => Array ( [name] => contacts.csv [type] => application/vnd.ms-excel [tmp_name] => /tmp/php6c1Knk [error] => 0 [size] => 137271 ))
-
Passing Anonymous Function to a function
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Thanks again for your replies. For my case, the logic belongs in bar1() and bar2(), and not other(). That being said and given your advice, I agree with both of you that I shouldn't be doing this way, and will move a small amount of script from other() to bar1() and bar2() thereby eliminating the need for a call back and making the script much more straightforward. -
Passing Anonymous Function to a function
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
I have two methods, bar1() and bar2() which do different things. They both use method other() and use it 99% the same way and the only difference will be a conditional IF statement. Instead of using an anonymous function, I "could" pass some value to other() and put the logic to use one of the two conditional checks there. Would you recommend doing it that way, with an anonymous function, or some other way all together? <?php class foo { public function bar1() { $a=123; $callback=function($b) use ($a){ return ($a==$b); }; $this->other($callback); } public function bar2() { $a=246; $callback=function($b) use ($a){ return ($a==2*$b); }; $this->other($callback); } private function other($cb) { $B=123; if($cb($B) ) { echo('$a == $b'); } } } -
Passing Anonymous Function to a function
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
I figure I should go crazy with them for a while, and then go back to reality! Yes, I could store it as a temporary variable. I take it that is the "right" way to do it? -
Am I doing this correctly? How would I do it if I wished to pass $a['x'] instead of $a, and wanted a normal variable within the callback to represent the passed $a['x']? <?php class foo { public function bar() { $a=123; //$a['x']=123; $callback=function($b) use ($a){ return ($a==$b); }; $this->other($callback); } private function other($cb) { $B=123; if($cb($B) ) { echo('$a == $b'); } } } $foo=new foo(); $foo->bar(); ?>