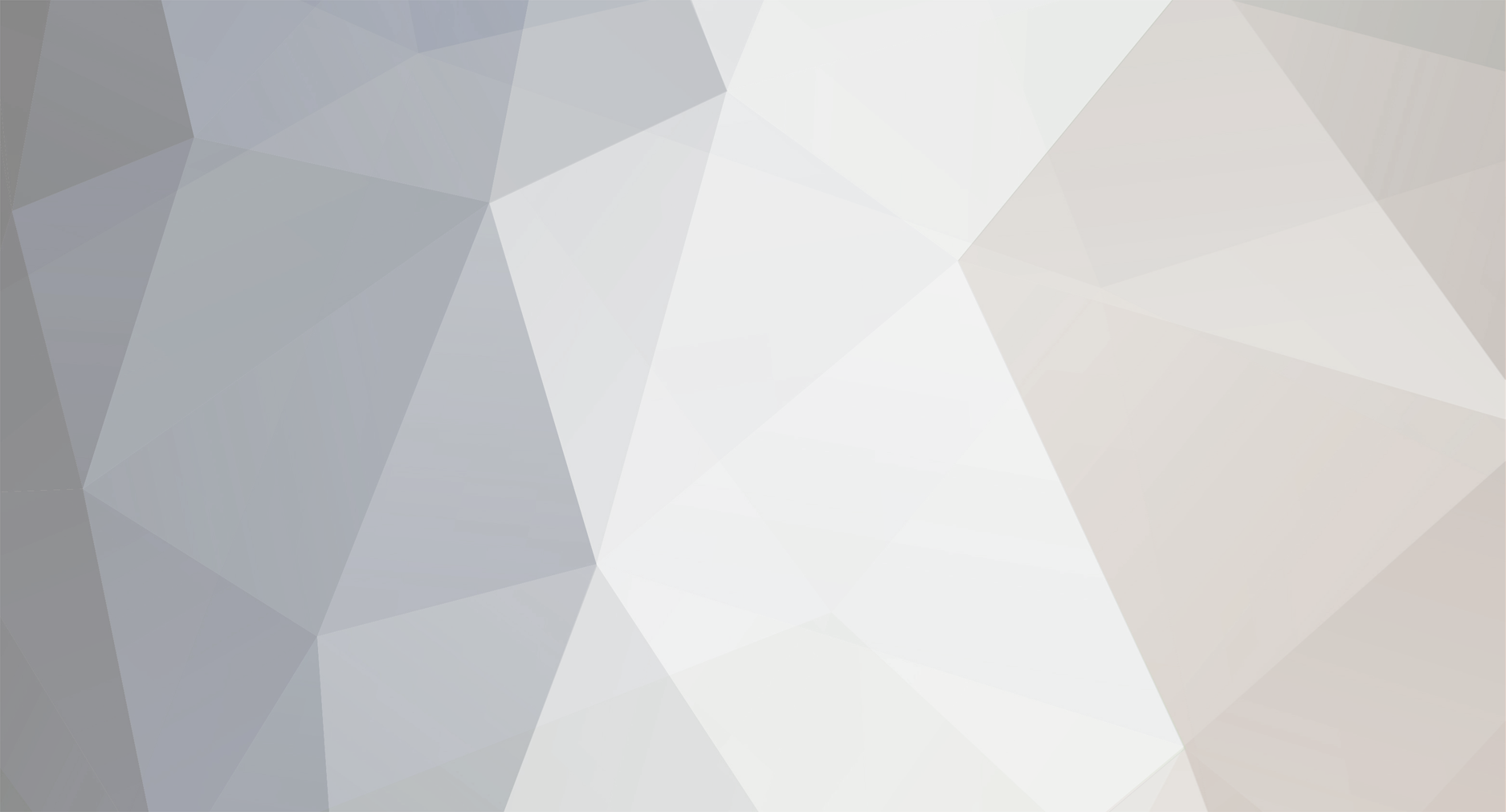
NotionCommotion
Members-
Posts
2,446 -
Joined
-
Last visited
-
Days Won
10
Everything posted by NotionCommotion
-
I don't know. Maybe he is really interested in cats and dogs, and your solution is perfect.
-
But it is the thought that counts
-
Thanks ignace, Yes, I was thinking of something similar. May I ask whether you actually do so in practice? What is the purpose of type hinting (PDO and PageFactory) the arguments sent to __construct()?
-
Thanks mac_gyver. I've never used constant() before. Looks like it is only needed when you wish to dynamically assemble the constant name (No point in using echo constant("MAXSIZE"); as described by http://php.net/manual/en/function.constant.php, right?). The array approach would work, however, I would need to use a global variable if used in multiple scripts and there is no way to prevent them from inadvertently being changes, so they are not true constants, right? Maybe a singleton class which is accessed as const::const('someConstant') or const::const('someGroupConstant','someGroup')? Or is what I am asking not typically desired, and maybe I should re-think my needs?
-
Hi Rafal, Its been a long time since I haven't used PDO (and you really should check it out), however, it looks like you are okay with sql injection. Most sites inform the user that the username and/or password is invalid as it prevents a bad buy from first knowing they have a valid username and then trying random passwords with it. Your approach informs them if they have a valid username but invalid password, and is actually more code intensive (not a big deal) as it queries the database twice. Your choice. In regards to session hijacking, you probably want to rely on others for final judgement.
-
Often in the very beginning of index.php, I will define a bunch of constants. To make sure I can quickly identify them as being one of my defined constants, I will often include some sort of prefix. define ('xzy_somecontant',123); define ('xzy_anothercontant',321); Sometimes I have a bunch of constants that are related; define ('xzy_id_for_page1',123); define ('xzy_id_for_page2',231); define ('xzy_id_for_page3',312); It would be nice to somehow group them into say "xzy_page_ids", and then access them by some index such as "page1" or "1" (or whatever makes sense for the given naming structure). Is this possible? Is there another defacto way of doing so such as a static class or something? Thanks
-
SQL injection will only occur when you interface with the database. Since you didn't show this scope, we wouldn't know. Note that SQLinjection is most easily prevented by using PDO prepared statements. If the data isn't escaped, the "data" desired to be inputted into the DB can change the SQL query to perform some unintended result. I also don't believe your script indicates whether session hijacking can or can't be accomplished. I suppose if this is your only script and a user has no ability to add JS to the content, you should be okay. You might want to look at password_hash() instead of hash(). Also, I don't think using a cryptic key for your session array (i.e. $_SESSION["e64X96ea"]) provides any protection.
-
I could be wrong, but it sounds like he is trying to learn regex, and wanted to use regex for this example. I messed around with it for a bit, but I am really bad at regex.
-
I know it is totally unrelated to your question, but I recommend not opening and closing PHP tags as often. Maybe there is some performance savings (or maybe the opposite), but any potential savings will greatly pale to the lost time in your life trouble shooting it (if others disagree, please comment). foreach($FinalName as $key => $item) { echo('<tr><td><input type="checkbox" name="fSelected[]" value="'.htmlspecialchars($FinalID[$key]).'" />'.($FinalID[$key] & $item).'</td></tr>'); } As for your specific question, I would probably do one of the following: Don't use Ajax, but submit each item to your server, and have it update your session value and send back the appropriate HTML. Don't use Ajax but just JavaScript (or jQuery which is JavaScript for noobs like me) to add hidden inputs on the existing page. If you do wish to use Ajax to update your session, you will need to send with it the session ID so that the server knows the session file to update.
-
Check if server cpu or memory is overloaded
NotionCommotion replied to Issam's topic in PHP Coding Help
Issam, I tend to agree with Requinix's questions/recommendations that you might be chasing the wrong carrot. If you really wanted to do so, my first inclination was to use some sort of native operating system function (are you running Linux/Windows/etc?) along with PHP's exec(), and then parse if if necessary. On a whim, I Goggled "php find cpu load", and the first hit was http://php.net/manual/en/function.sys-getloadavg.php, so you might want to check out this as well. Before doing so, however, you should find out what is causing over usage and deal with it. -
Thanks requinix, On my original post, I said less than 500 documents per user. But what if I am wrong? I will change to something that spreads things out evenly. For now, I won't save the full path. If I ever need to, the paths can be derived from the ID. Thanks for your help
-
So, you would not create a separate folder for each user, correct? Why or why not? Two character will result in 256 sub-folders per folder instead of 16, however, I guess this is better and agree. I actually wasn't planning on storing the hash, just the following four fields, and and the fifth if the full path was saved. Saving the full path just seems anti-normalized, however, maybe it makes sense. id: 5 name: blabla.pdf users_id: 27 date_uploaded: 2014-11-15 13:59:59 full_path_to_file: /bla/bla/user_files/e4/da/3b7fbbce2345d7772b0674a318d5
-
Ah, you've redeemed my faith in Newbies! So I condensed the output to make it simpler. It contains two primary elements: transactions which is in turn an unassociated array containing (7) associated arrays, and lastblock which is a string. When you apply the foreach on it, you or iterating over these two elements, yet it is obviously that you need to iterate over the transactions array (and when you do, $tx will be one of those 7 associated arrays, and sure enough category, confirmations, address, amount, and txid are all there - at least I hope so because I didn't check While this didn't not specifically solve your issue, it gives you the clues to quickly figure it out. Array ( [transactions] => Array ([0] => Array(...),[1] => Array(...),[2] => Array(...),...,[7] => Array(...)) [lastblock] => 00000000000000000db5d7c653732396fa7345bb4f97cbab73720e29d9c0b7bd )
-
I have a bunch of users in a database (id, name, etc). I have a bunch of documents which belong to users (id, filename, users_id, etc), and expect 500 or less per user. The documents will be renamed to the document_id, and X-Sendfile (since they are stored under the document root) will be used to retrieve them and a header will be used to return them to their original name. Is it recommended to make a separate folder for each user and store each individual user's documents in that folder, or create one folder for all documents? If I go with the one folder approach, I will need some method from keeping the total files per folder below some reasonable limit (1,000?). My thought is to estimate the maximum potential number of folders, and creating subfolders under the main document folder. I will likely hash the ID, and use the first character to create the first subfolder, the second character to create a second subfolder in the first subfolder, and continue as long as needed to accommodate the maximum potential documents (if there are 1,000,000 potential folders, then three levels will keep the maximum per folder under 244). Please provide rational for one approach over the other. Thank you
-
header(Location: blah) not redirecting
NotionCommotion replied to trevzilla's topic in PHP Coding Help
It doesn't take much! Glad to be of help. -
header(Location: blah) not redirecting
NotionCommotion replied to trevzilla's topic in PHP Coding Help
You sent content to the browser before calling the header (a big no-no). Happened right between when you call session_start() and include your password.php file. -
Cookie priority with common names
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Thank you all for your replies. Subdomains turned out to be just the ticket (I hope!). Step 1: I set up my DNS for mysite.com, *.mysite.com, and *.*.mysite.com to go to the same file. Step 2: Apache redirects www (or PHP just strips it off), and deals with ServerAlias. Step 3: Do the following: $domain=explode('.', $_SERVER['HTTP_HOST']); switch($domain) { case 1: //Display front site. case 2: //If $domain[1] exists in DB, display user site, else display warning page. case 3: //If $domain[1] exists in DB AND $domain[2]==admin name (as stored in DB for $domain[1]), display admin site, else display warning site. default: //display warning page } Questions Do I have the correct workflow? (see question 4 which might indicated that I need to change it) Can I rely on $_SERVER['HTTP_HOST']? It is provided by the client (bad), but verified by the webserver (good), so it should be fine, right? If I use sessions on all three and don't change the name, for the admin site, I will have (3) cookies all named PHPSESSID with the same path but different domains. Will the cookie with the same domain as the admin page always take precedent? Should I rename all three to have unique names? By the way, this was the original intent of what turned out to be an off-topic title to this thread. Currently, a user cannot add content (and thus JavaScript) to mysite.com and joes_site.mysite.com, so I have no risks, but that might change in the future. As stated in Question 3, admin site includes its own session cookie as well as session cookies for main site and user site, and user site includes its own session cookie as well as session cookies for main site. Jacques1 recommends using domains joe.user-sites.mysite.com and joe.site-admin.mysite.com (and I suppose I could main.mysite.com as well from the "corporate" site). I guess this makes sense, but I obviously don't want the user to have to enter "user-sites" in the URL. Just use Apache to rewrite and add it? Also, Jacques1 indicated that there is a risk for “global” cookie for .users.yoursite.com which is also valid for other user sites. How is this mitigated? Thank you all for your help! -
Guess so, but they are missing out on some fun!
-
I am sure you are correct, however, I always say "look at what the server is telling you by using var_dump or print_r (and without saying, error detection)". I've posted a dozen similar replies, and have not heard once that it was of any help. Shouldn't (especially beginners) be doing this often?
-
Cookie priority with common names
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Thanks Kicken. I will check out ServerAlias! I was able to get a subdomain working earlier today, but had to go to my domain name host (1&1), and create a subdomain for my primary domain and point that name to my server. I will contact 1&1, but hopefully there is a better way. Thanks Jacque1. As you could tell, I am very new to subdomains, but very excited on the opportunities that they might provide. -
if ($tx['category']!='receive') continue; Notice: Undefined index: category in C:\xampp\htdocs\content\cron\check_deposits.php on line 18 $tx does not have the element 'category'. When you get these errors/notices, back up a bit. Use either var_dump($txs) or my favorite echo('<pre>'.print_r($txs,1).'</pre>');. Is the array what you expected?
-
Cookie priority with common names
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Thanks kicken, I wasn't implying that a separate copy of my application will be used for each user, but that something unique would be made available for each user, and the application would configure things as appropriate. Sounds like we are on the same page. I take it that "configuring a wild-card vhost" is a Apache (or similar) configuration, and will it send all requests regardless of the subdomain to the same file, right? So then, I don't need a separate folder for each user? Sounds great! My virtual host is already created with a wildname, no? (see below). But this will not magically allow janedoe.mysite.com to work. Can you give me some points on where to? NameVirtualHost *:443 <VirtualHost *:443> bla bla bla Thank you -
Cookie priority with common names
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
I am obviously way over my head. Currently, a user logged on to john.yoursite.com can do very little. They can view information, and do a couple of heavily validated SELECT menu post updates. That's not to say this is a smart approach as things seem to change and maybe it will not be so safe in the future. The admin user does have the ability to add HTML, however, I am using http://htmlpurifier.org/ to in theory make it safe. I guess I (kind of) understand the part about a good guy visiting a bad guy's site, and getting a cookie. But what if the session array was broken down by site accessed name, and the application only allows access based on the URL? also, is my http://mydomain.com/index.php?site=folder1&type=admin idea totally fubar? -
Cookie priority with common names
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Understand the recommendation about managing session credentials via code. Easy enough. Does this also provide reasonable protection between front and admin sites? In regards to each user site having its own sub domain, how is this implemented? My wildest dreams suggest 100,000 subdomains . If I don't have 1,000 subdomains, I failed. Do you know if that is how wordpress.com does it? For instance, I have a wonderful site https://notioncommotion.wordpress.com/. Think it is a unique domain? Think they created some folder just for me? Or is wordpress.com's implementation inherently insecure, and I should not attempt to mimic it? Or maybe I shouldn't even be creating separate folders for each site. Instead, I have something like http://mydomain.com/index.php?site=folder1 and http://mydomain.com/index.php?site=folder1&type=admin, and use the webserver to rewrite it. Can it be implemented securely? If so, please provide general description of approach. Thank you -
Cookie priority with common names
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Thanks Jacques1, I agree that when things get weird and hacky, it is usually a flawed implementation, and would appreciate some more input. I posted a somewhat related question http://forums.phpfreaks.com/topic/292405-how-to-structure-website-with-accompanied-microsites/ which is not exact, but provides some background. I have a public website where people can look at the application, and signup if they want. Currently, I have this at http://mydomain.com/index.php. Sessions are only used to support the site creation phase (you've done part, and only need to click a link on your email), and doesn't deal with user logons. If they sign up, they select a "domain name" (not really a domain name, but it differentiates their site kind of like a WordPress.com or Facebook account does) and they get two websites. Assuming they picked the name "bobs_site", their two sites will be http://mydomain.com/bobs_site/index.php and http://mydomain.com/bobs_site/administrator/index.php. The two sites are completely separate and an admin user doesn't have access to the frontend using their admin username/password. Normally the user who signed up will only visit the administrator portion, and the user's customers will visit the other portion, however, the user will likely wish to see what the other portion looks like so may create a frontend logon for himself and log on as well. You provided the following advise: Could you elaborate on this topic, and how it might apply to my scenario? Thank you