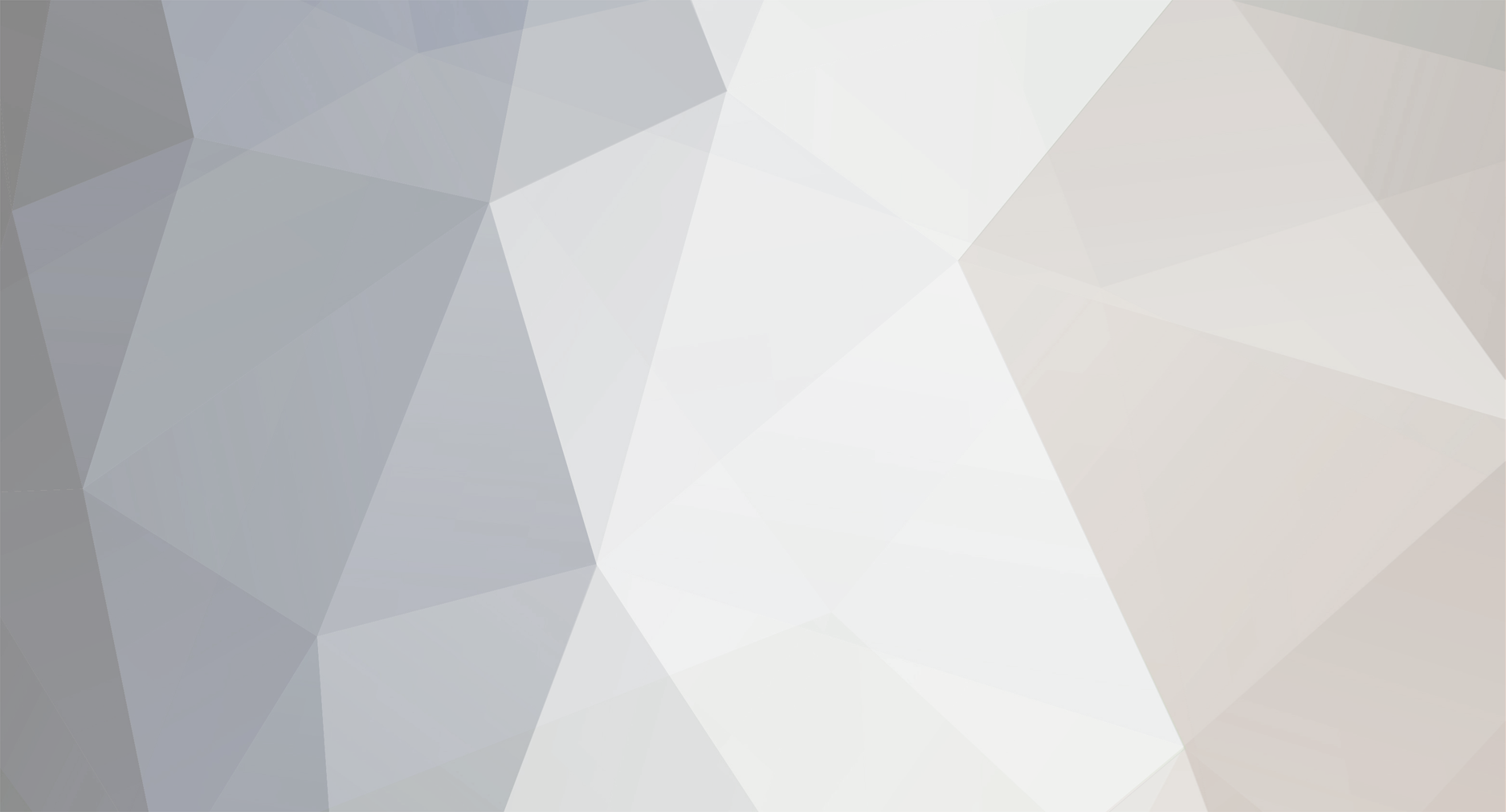
Jessica
-
Posts
8,968 -
Joined
-
Last visited
-
Days Won
41
Posts posted by Jessica
-
-
Well, you can't do that... That means key => value.
Maybe make a class, then an array of instances of it and loop through there.
Or for a simpler version,
[code]
$person = ('name'=>'andrew', 'age'=>21, 'loc'=>'UK');
$people[] = $person;
$person = ('name'=>'joe', 'age'=>23, 'loc'=>'USA');
$people[] = $person;
foreach($people AS $person){
$age = $person['age'];
$name = $person['name'];
//etc...
}
[/code] -
Sounds like the problem is on their end. If it suddenly stopped being able to connect...maybe you should contact them?
-
Can you post some code?
-
If you want something to change after the page has loaded, you need javascript. It's okay, it doesn't bite too hard.
I don't know if PHP can create animated gifs - I know it does gifs, but the animation I don't know about. The only other option I see is to make an animated gif counting down.
Just use javascript ;) -
The suggestion was simply have the same action page and use POST to determine what to do, instead of having different action pages.
-
You probably have magic_quotes_gpc turned on. You need to explore deeper into the world of sql injection. :)
(Edit: I misread about you using mysql_real_escape_string. Looks fine to me.)
Also, cookies can be easily edited. Don't rely on JUST cookies for anything. -
I second Thorpe.
-
Are you meaning you want their username to be displayed in the username input?
<p align="center">Username: <input type="text" name="username" value="'.$info['Username'].'"></p> -
That is $_GET['id'];
-
What do you think it would be? How many hours can there possibly be offset? How many digits is that? If you're storing it like 1 hour offset, 2 hours, etc, don't you think it's kind of obvious what the maximum is?
If you're storing it another way, please explain. -
Thanks!
-
[code]if(!preg_match("/^[A-Za-z.]+$/", $this->username)){
$valid = 0;
}else{
$valid = 1;
}[/code]
I also want to allow the - and a single space.
Help? -
Have you tried other hotmail accounts?
-
You'll want to make an AJAX call to your php file. Check out javascript frameworks like Mootools or script.aculo.us.
-
Are you asking how to use mail()?
-
If you upload a zip, that is ONE file. If you upload an image, that is ONE file. It does not matter how many files were in the zip, it is now ONE file. Treat it as such.
If you then want to extract the images, you'll probably need some sort of add-on. -
What is @file? I thought that was in Perl, not PHP.
-
You're calling the query twice. Remove the first one.
[code]
echo "<table border=\"1\" width=\"100%\" id=\"table3\">\n" . "<tr>\n" . "<td>Storm ID</td>" . "<td>Status</td>" . "<td>Updated</td>" . "<td>Valid On</td>" . "<td>Conditions</td>" . "<td>Location</td>" . "<td>Likelihood</td>" . "<td>Risk</td>" . "<td>Alert Mode Consideration</td></tr>" . "<tr><td>1</td>";
$q = mysql_query("SELECT * FROM `swc_alertanalysis`") or die (mysql_error());
while($row = mysql_fetch_assoc($q)) {
//Is status a field in your table? If so...
$status = $row['status'];
if ($status == 'active') {
...
[/code]
Now you need to know WHY.
Please go read some of the tutorials. Seriously. They're written for a reason.
You can clean up your html several ways. First, you don't need to keep concatenating strings. You can have one long string.
You can even close your PHP and just write HTML.
[code]
<table border="1" width="100%" id="table3">
<tr>
<td>Storm ID</td>
<td>Status</td>
<td>Updated</td>
<td>Valid On</td>
<td>Conditions</td>
<td>Location</td>
<td>Likelihood</td>
<td>Risk</td>
<td>Alert Mode Consideration</td>
</tr>
<?
$q = mysql_query("SELECT * FROM `swc_alertanalysis`") or die (mysql_error());
while($row = mysql_fetch_assoc($q)) {
//Is status a field in your table? If so...
$status = $row['status'];
if ($status == 'active') {
...
[/code] -
Line 9: $status - where does this variable come from?
-
"Should I make two functions? One for "edit" (into which one puts new info) and one for "added" (which displays the new info with a choice to further edit)?"
From what you explained, I'd try that first. -
-
No problem.
Hit the solved button ;) -
This is the code you posted.
[code]echo "<td align=center bgcolor=red><font face=Arial color=#FFFFFF>VERY LIKELY</font></td>";
}
if(mysql_num_rows($q) == 0) { echo "<tr><td align=center bgcolor=gray><font face=Arial color=#FFFFFF>-</font></td><td align=center bgcolor=gray><font face=Arial color=#FFFFFF>INACTIVE</font></td></tr>"; }
") or die(mysql_error());
?>[/code]
You don't see how this:
[code]") or die(mysql_error());[/code]
is not valid code? What do you think this does?
You were supposed to put that on the end of your query. Instead you put it at the end of your code.
This symbol: " starts and ends a string. So, you have a STRING being started on that line and it never ends. So php can't compile the code.
Just remove the line. I'm not trying to be rude, but you need to take a minute and read through the code and say, okay, what does this do? Is this causing the error? If I comment out this line, does the error still occur? Ok, it doesn't, so this is the line that is causing this error. Oh wait, this line doesn't make any sense. -
Look at the code you posted last. Do you see anywhere where you have a string beginning a line and you never close it? A string is surrounded by quotes. Look at the line right above your closing tag. I know you are a beginner which is why you need to understand what the code means, not just let us keep fixing it for you :)
[SOLVED] Get page url
in PHP Coding Help
Posted