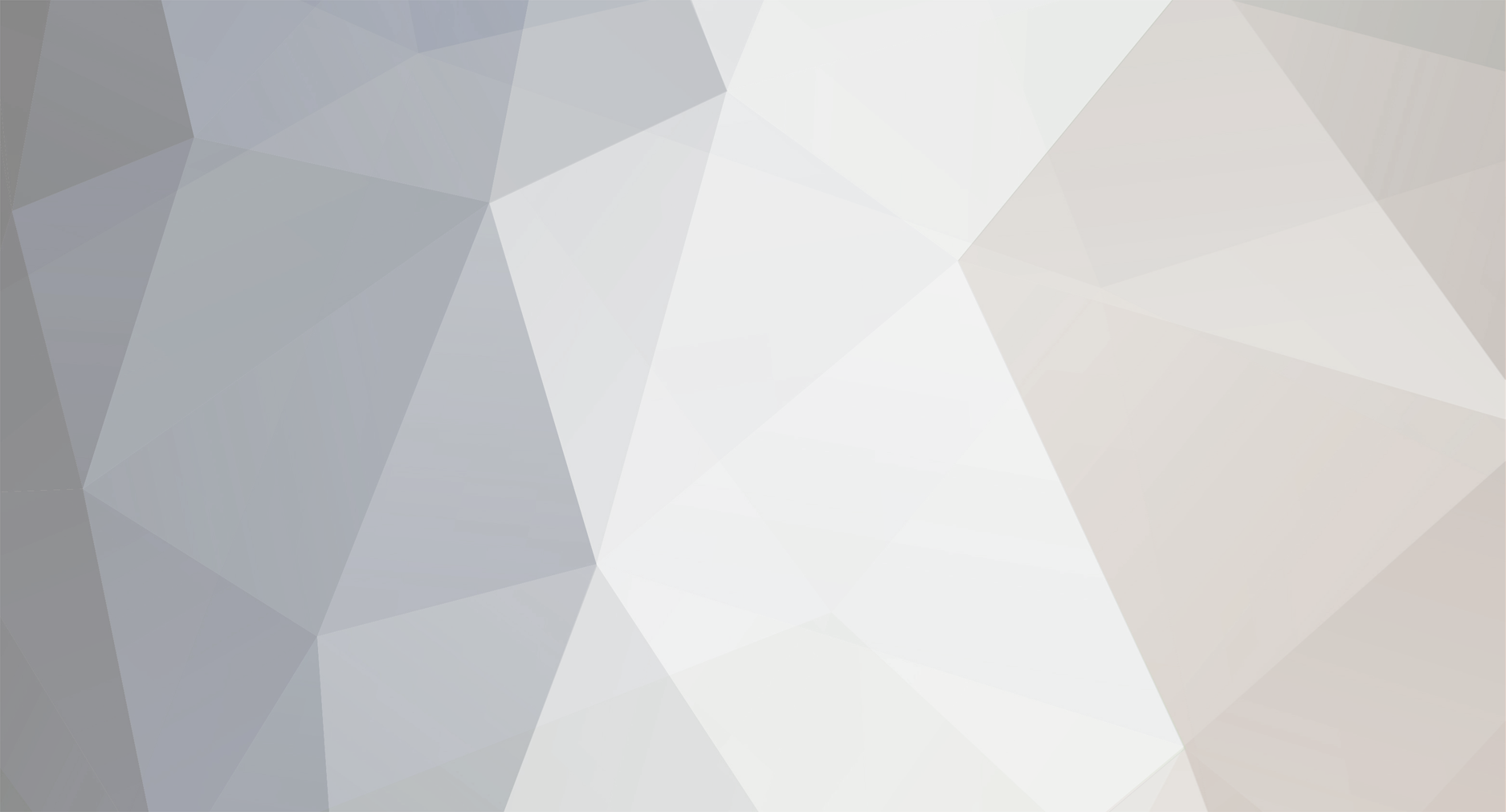
dcro2
Members-
Posts
489 -
Joined
-
Last visited
Never
Everything posted by dcro2
-
[SOLVED] allowing users to update/change thier data
dcro2 replied to Rother2005's topic in PHP Coding Help
This should give you an idea. You might need to edit the cookie name and the MySQL field names as well. [code]<?php // Connects to your Database mysql_connect("localhost", "root", "") or die(mysql_error()); mysql_select_db("booze") or die(mysql_error()); if(!$_POST['submit']) { $data = mysql_query("SELECT * FROM member WHERE Username ='".$_COOKIE['ID']."'") or die(mysql_error()); $info = mysql_fetch_array( $data ); echo "<form name=\"form1\" method=\"POST\" action=\"\"> <p align=\"center\">Username: <input type=\"text\" name=\"username\" value=\"{$info['Username']}\"></p> <p align=\"center\">Password: <input type=\"text\" name=\"password\"></p> <p align=\"center\">First Name: <input type=\"text\" name=\"firstname\" value=\"{$info['FirstName']}\"></p> <p align=\"center\">Surname: <input type=\"text\" name=\"surname\" value=\"{$info['Surname']}\"></p> <p align=\"center\">Address 1:<input type=\"text\" name=\"address1\" value=\"{$info['Address1']}\"></p> <p align=\"center\">Address 2:<input type=\"text\" name=\"address2\" value=\"{$info['Address2']}\"></p> <p align=\"center\">Town: <input type=\"text\" name=\"town\" value=\"{$info['Town']}\"></p> <p align=\"center\">County: <input type=\"text\" name=\"county\" value=\"{$info['County']}\"></p> <p align=\"center\">Postcode: <input type=\"text\" name=\"postcode\" value=\"{$info['Postcode']}\"></p> <p align=\"center\">Tel No: <input type=\"text\" name=\"telno\" value=\"{$info['TelNo']}\"></p> <p align=\"center\">Mobile: <input type=\"text\" name=\"mobile\" value=\"{$info['Mobile']}\"></p> <p align=\"center\">Email: <input type=\"text\" name=\"email\" value=\"{$info['Email']}\"></p> <p align=\"center\"><input type=\"submit\" name=\"submit\" value=\"Edit Details\"></p></form>"; } else { $username=$_POST['username']; $password=$_POST['password']; $firstname=$_POST['firstname']; $surname=$_POST['surname']; $address1=$_POST['address1']; $address2=$_POST['address2']; $town=$_POST['town']; $county=$_POST['county']; $postcode=$_POST['postcode']; $telno=$_POST['telno']; $mobile=$_POST['mobile']; $email=$_POST['email']; $sql = "UPDATE member SET Username='$username', Password='$password', Firstname='$firstname', Surname='$surname', Address1='$address1', Address2='$address2', Town='$town', County='$county', $Postcode='$postcode', TelNo='$telno', Mobile='$mobile', Email='$email' WHERE Username = '".$_COOKIE['ID']."'"; mysql_query($sql) or die(mysql_error()); echo "Your info has been updated"; } ?> [/code] A little note, you don't need a while() loop for mysql_fetch_array() if you're only going to process one row. Off topic: Also, I'm 14, that's why I wasn't out getting "wrecked" as you posted yesterday. ;) -
You need to use complete addresses such as http://mydomain.com/index.php?false=$error, it doesn't support relative paths.
-
Yes, sorry, I don't like explaining too much :P
-
[SOLVED] allowing users to update/change thier data
dcro2 replied to Rother2005's topic in PHP Coding Help
That should be right, start some code and we'll help you if you can't get something. -
You need to use global in all of the functions in order to access them within that function. [code]function view() { global $DBUser, $DBPass;[/code]
-
Yes, put session_start() before line 7 of your script. You can't send any headers after you start outputting to the browser.
-
You need to use that function before anything is output to the browser, preferably at the top of the script. Or use ob_start() and ob_end_flush() in your script. http://www.phpfreaks.com/forums/index.php/topic,37442.0.html
-
I'm not sure you understand what addslashes() does... [quote]Returns a string with backslashes before characters that need to be quoted in database queries etc. These characters are single quote ('), double quote ("), backslash (\) and NUL (the NULL byte).[/quote] Or maybe I don't understand what you're doing with commas.
-
You're missing a ending curly brace ( } ) to end the if:else
-
You need to end the PHP area with ?> before </body>
-
Yup. Eastern Time. New Year in 4 minutes.
-
[SOLVED] Im trying to write a PHP script that says....
dcro2 replied to forumnz's topic in PHP Coding Help
So nothing's showing up? -
Only 11:52 here ;D
-
[SOLVED] Im trying to write a PHP script that says....
dcro2 replied to forumnz's topic in PHP Coding Help
Have you set any session variables? -
OK... [code]$username = stripslashes($username); $hour = time() + 3600; setcookie("ID_my_site", $username, $hour); setcookie("Key_my_site", $password, $hour); header("Location: members.php"); } ?> </body>[/code]
-
[SOLVED] Im trying to write a PHP script that says....
dcro2 replied to forumnz's topic in PHP Coding Help
[code]if (!$_SESSION['username']){echo('Log In');} else {echo('Log Out');}?>[/code] ??? -
Well, you need to end the PHP tag before you put HTML in. [code]$username = stripslashes($username); $hour = time() + 3600; setcookie("ID_my_site", $username, $hour); setcookie("Key_my_site", $password, $hour); header("Location: members.php"); ?> </body>[/code]
-
You can just replace that line with [code]$username = stripslashes($username); $hour = time() + 3600; setcookie("ID_my_site", $username, $hour); setcookie("Key_my_site", $password, $hour); header("Location: members.php");[/code]
-
Well, the <meta> tag goes in the <head>. If you use the header() method, you can't output anything to the browser before it's called. Anything output to the browser after header() will probably not be seen anyways.
-
Because you're making those arrays a string, each index of it is a character in the string. $array[0] = "Y" $array[1] = "u", etc... You can do that with any string.
-
You can use <meta http-equip="Refresh" content="5; members.php"> 5 is the time to wait in seconds, members.php is the URL. Or.. If you're not going to echo anything on the page, then use header("Location: members.php"); to instantly redirect them.
-
Heh, well... never read any books ;D. Sorry. Good luck with everything else.
-
I see what's happening... the password field in the database is a char, you say, it needs to be a VARCHAR with a limit of 255 characters. Then re-register and remove that line about the original password and database password.
-
And another one at line 41... I guess I can't transform while() into a normal statement right.