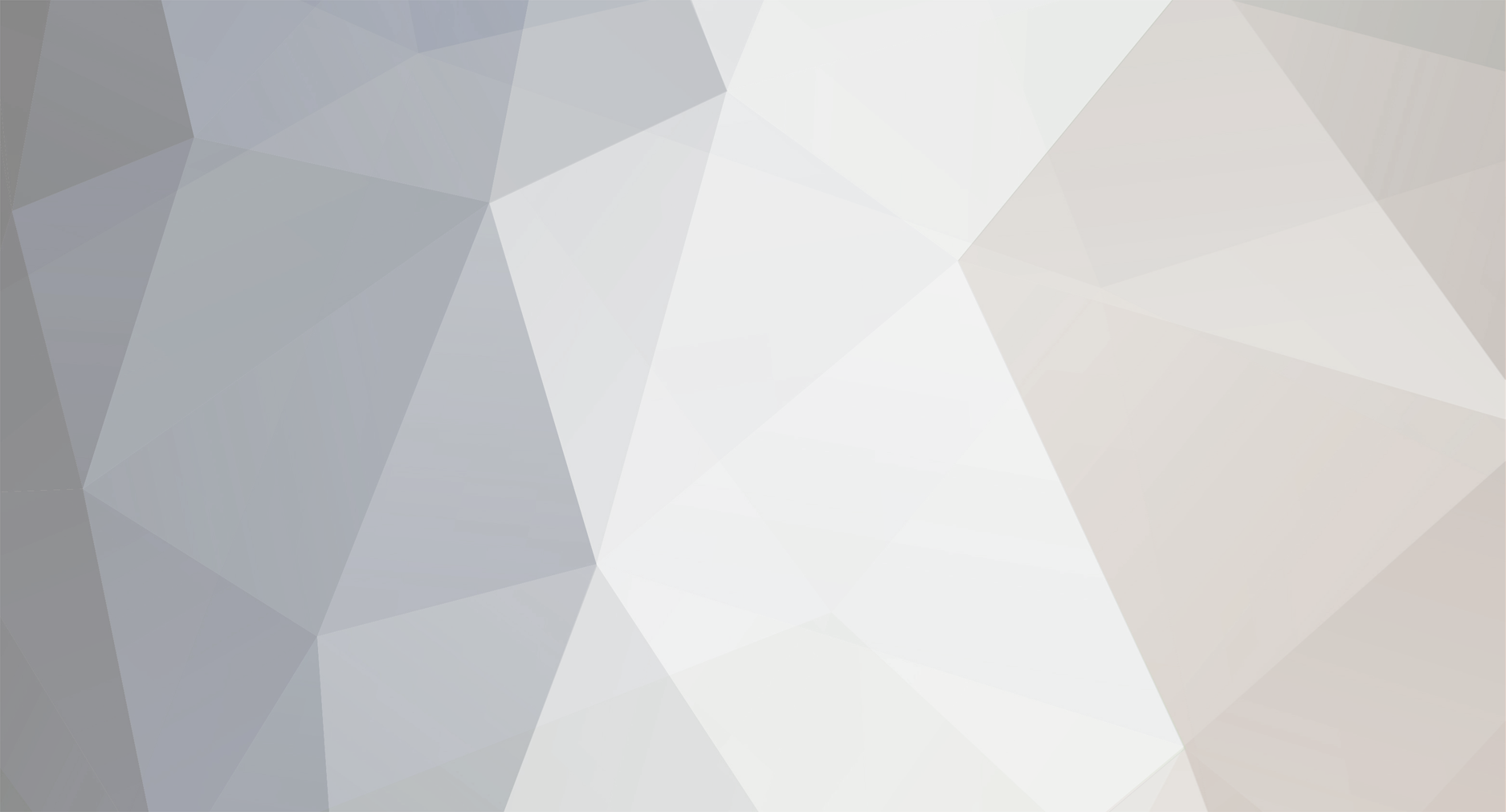
dcro2
Members-
Posts
489 -
Joined
-
Last visited
Never
Everything posted by dcro2
-
You mean... continue? It'll skip the rest of the loop and begin the next iteration. $i = 0; while($i < 10) { if ($i == 7) { //Do Function } else { $i++ continue; //Go back to beginning of loop } //More if statements here. }
-
Using a HTML template with includes can't read from $_GET
dcro2 replied to rpmorrow's topic in PHP Coding Help
Try using the method I posted. Like this for your DisplayPage function: <?php function DisplayPage($page_id, $page_type) { //the two variables here are used to select the right info from database, and which template to use ob_start(); include_once($_SERVER['DOCUMENT_ROOT'].'/templates/home-template.htm'); $output = preg_replace("/~~PAGECONTENT~~/",$row['page_content'],ob_get_clean(),1); echo $output; ?> Worked perfectly for me. Use [ php ] for php code btw. [ code ] doesn't color php code unless you wrap it in <?php ?> -
How do you expect it come out exactly? The way you have it set up right now you'll just have a ton of columns with one link inside each. <tr> is a row. <td> is a column. Each <tr> (row) of a table can have multiple <td> (column) inside, and of course you have to end the <tr> in order to get a new row.
-
If you have PHP5, you might as well just use the FILE_IGNORE_NEW_LINES flag of file. It might not make any difference if the file is small but, I don't know, I like cleaner code . $sites = file('file.txt', FILE_IGNORE_NEW_LINES);
-
Using a HTML template with includes can't read from $_GET
dcro2 replied to rpmorrow's topic in PHP Coding Help
I *think* if you use output buffering you could somehow get this to work. For example, call ob_start() right before include()ing the html file. Then, call ob_get_clean() to get what the included file outputted and clean the buffer so it doesn't output to the browser. ob_start(); include('somehtmlwithphpcode.html'); $output = ob_get_clean(); That'll let the file actually execute and $_GET stays the same. -
Put 'em into two arrays? //might have scope problems otherwise? $approvedurls = array(); $unapprovedurls = array(); foreach($vanity_url as $v){ if($v->approved){ $approvedurls[] = $v->url; } else { $unapprovedurls[] = $v->url; } } echo "Approved vanity urls:"; foreach($approvedurls as $url) echo $url; echo "Unapproved vanity urls:"; foreach($unapprovedurls as $url) echo $url; I'm still confused how you got that output without any newlines however.
-
I think you want to use strrpos instead of strpos. It searches for the last occurrence in a string.
-
SUBSTRING_INDEX: $query = "SELECT id, title, SUBSTRING_INDEX(content, ' ', 101) FROM news ORDER BY id DESC"; You could also get the whole field, explode() by spaces and then join the first 100 or something. I figure something directly in MySQL will work faster though.
-
Is sendmail setup correctly? Is this on your own machine/server or is it on shared hosting? Php has to use a smtp server to send mail succesfully. Also, make sure you check your spam folder since these emails could come from a strange source (from hotmail's point of view). PS: you might want to take out your email from your post. Spambots and whatnot.
-
My bad. Meant to post this as the second link: http://www.gidforums.com/t-1816.html. Good luck! Edit: sorry, that only matches links starting with http(s):// Here's something that might work with some modifications: $t = " ".preg_replace( "/(([[:alnum:]]+:\/\/)|www\.)([^[:space:]]*)". "([[:alnum:]#?\/&=])/i", "<a href=\"\\1\\3\\4\" target=\"_blank\">". "\\1\\3\\4</a>", $t); From here: http://snipplr.com/view/29556/php-parse-url-mailto-and-also-twitters-usernames-and-arguments/
-
You might be missing single quotes around the user_id here unless it's a number: $qq = "SELECT name FROM campaigns WHERE (user_id={$_SESSION['user_id']} AND keyword='$kw')";
-
No problem, just remember that my example was pretty simple and will fail on a lot of links that aren't written the same way. There's some more advanced regular expressions here for example. As for what you're asking for, I would probably convert all <a> tags into plain urls and then use something like this to convert them all back along with the unlinked ones.
-
I think if you just do something like this it could work: $timetoadd = "10"; //from your text field $time = "1500"; //from database $newtime = date('Hi', strtotime($time." +".$timetoadd." minutes")); I couldn't get it to work with something like 00:10:00 for some reason, but this is the concept. You can do +10 minutes, hours, months, years, etc. Note: I used 'Hi', not 'hi' because lower-case h is 12-hour time, so you would get 0310.
-
imagecreatefromjpeg() is looking for Rainbow-code-1_blck.jpg in the directory your php script is in. Include the whole path like you did with file_exists(): $filename = "Rainbow-code-1_blck.jpg"; if (file_exists(sfConfig::get('sf_upload_dir') . '/rainbowcode/images/profilepics/'.$filename)) { echo "file found"; $source = imagecreatefromjpeg(sfConfig::get('sf_upload_dir') . '/rainbowcode/images/profilepics/'.$filename); }
-
Err.. sorry, I got confused. What I meant to say is it will only get the url from the first <a>. It will replace all occurrences. You could get all the urls like this: <?php $text = '<a href="http://theurl.com/">link</a>\n<a href="http://theurl2.com/">link2</a>'; $replacement = 'the replacement here'; //put the urls inside $urls if we find a match if(preg_match_all('/<a href="(.*?)">/i', $text, $matches)) { //$matches[1] contains all matches to the first subpattern $urls = $matches[1] } //replace all <a> tags with $replacement $text = preg_replace('/<a href=".*?">.*?<\/a>/i', $replacement, $text); ?>
-
Something like this maybe: <?php $text = '<a href="http://theurl.com/">link</a>'; $replacement = 'the replacement here'; //put the url inside $url if we find a match if(preg_match('/<a href="(.*?)">/i', $text, $matches)) { $url = $matches[1]; } //replace the whole <a> tag with $replacement $text = preg_replace('/<a href=".*?">.*?<\/a>/i', $replacement, $text); ?> It will only replace the first occurrence in your text.
-
I'm a little confused if you want the shape or class, but I think you want to put a subpattern in with parentheses: preg_match('/shape=\'(.*)\'/sU',$value,$matches); Then the match inside the parentheses will be inside $matches[1] Here's more about subpatterns: http://www.php.net/manual/en/regexp.reference.subpatterns.php
-
Well, you can't put WHERE clause in an INSERT, but I don't know what you're trying to do... [code]INSERT [LOW_PRIORITY | DELAYED | HIGH_PRIORITY] [IGNORE] [INTO] tbl_name [(col_name,...)] VALUES ({expr | DEFAULT},...),(...),... [ ON DUPLICATE KEY UPDATE col_name=expr, ... ][/code] Are you trying to find which table has that value? If so, you should search for it beforehand.
-
Would you care to share some code? Also remember $_POST['uploadedfile'] contains the path, such as "C:\swffile.swf".
-
If you use fopen() with write access, the file will automatically be created. [code]<?php $o = fopen($_POST['uploadedfile'].".txt", "w"); fclose($o); ?>[/code] You can also strip the extension from the file to get just .txt, not .swf.txt
-
If you have PHP 5, you can use scandir() [code]$dir = scandir("folder/"); $rand = rand(0, count($dir)-1); $song = $dir[$rand];[/code]
-
If you include an external address, you will only be including the HTML source of it. The php file has to be in the local server in order to include the source of it.
-
[SOLVED] allowing users to update/change thier data
dcro2 replied to Rother2005's topic in PHP Coding Help
Well, uh, it's trying to set them all to a blank value, check all the variable name and field names. -
You're missing a starting brace for [b]else[/b] here [code]<p align="center"><input type="submit" name="register" value="Enter Details"></p></form>';} //27 else //checks if user has input text[/code] and then you're missing a ending brace for each of these [code]if ($username=$_POST['username'] == "") { die ("No Username Input"); if ($password=$_POST['password'] == "") { die ("No Password Input"); if ($firstname=$_POST['firstname'] == ""){ die ("No Fristname Input"); if ($surname=$_POST['surname'] == "") { die ("No Surname Input"); if ($address1=$_POST['address1'] == "") { die ("No Address Input"); if ($town=$_POST['town'] == "") { die ("No Town Input"); if ($county=$_POST['county'] == "") { die ("No County Input"); if ($postcode=$_POST['postcode'] == "") { die ("No Postcode Input"); if ($email=$_POST['email'] == "") { die ("No Email Input");[/code] What happened to your previous topic?
-
Maybe use Javascript to time them, and then make a cookie? PHP is only a pre-processor.