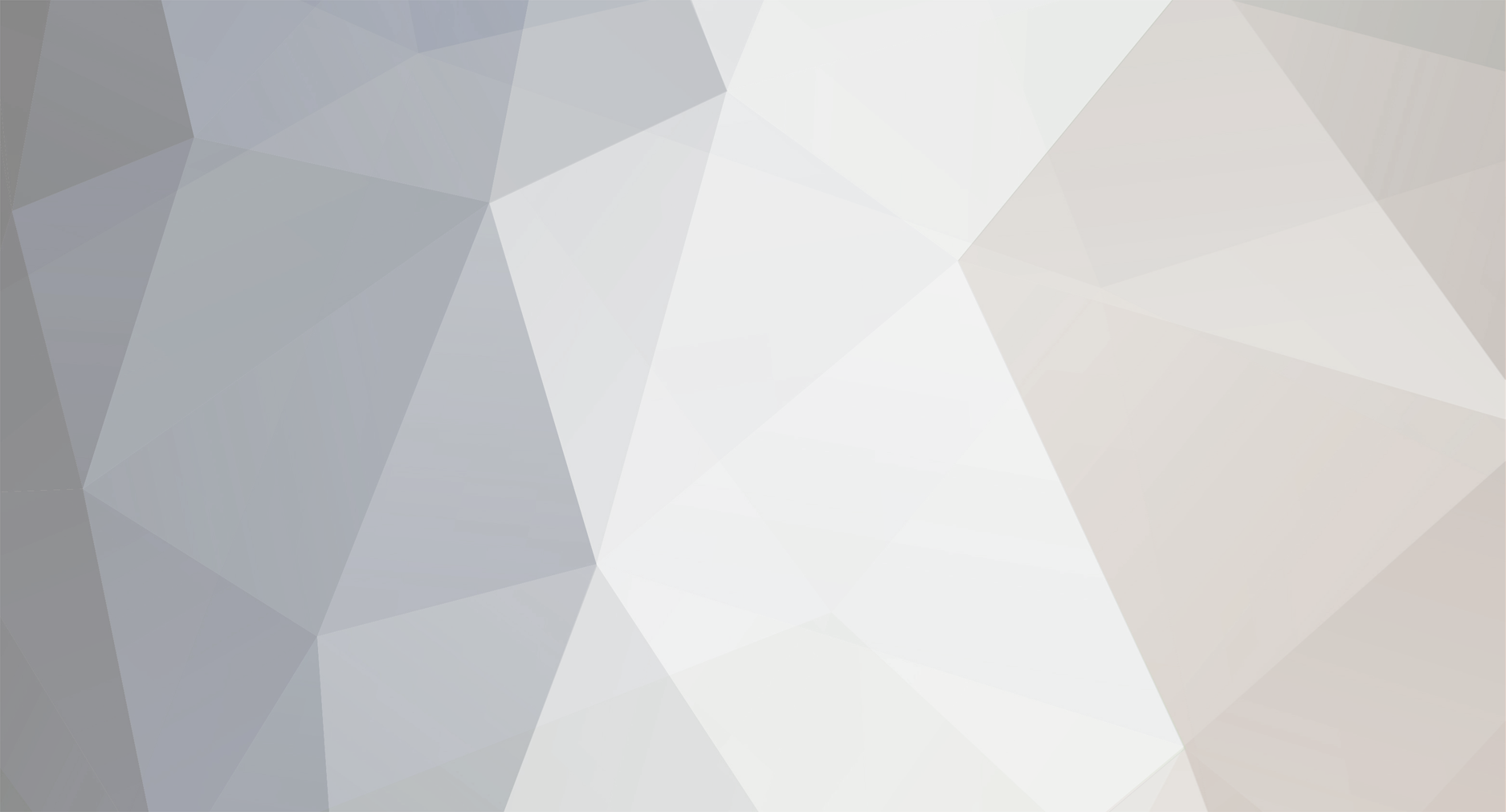
dcro2
Members-
Posts
489 -
Joined
-
Last visited
Never
Everything posted by dcro2
-
Woops, forgot a ";" on line 13 :P
-
Can you use this as your login script? Just to test it [code]<?php // Connects to your Database mysql_connect("localhost","root","") or die(mysql_error()); mysql_select_db("booze") or die(mysql_error()); //Checks if there is a login cookie if(isset($_COOKIE['ID_my_site'])) //if there is, it logs you in and directes you to the members page { $Username = $_COOKIE['ID_my_site']; $Pass = $_COOKIE['Key_my_site']; $check = mysql_query("SELECT * FROM member WHERE Username = '$Username'")or die(mysql_error()); $info = mysql_fetch_array( $check ) if ($Pass != $info['Password']) { } else { header("Location: members.php"); } } //if the login form is submitted if (isset($_POST['submit'])) // if form has been submitted { // makes sure they filled it in if(!$_POST['Username'] || !$_POST['Pass']) { die('You did not fill in a required field.'); } // checks it against the database $check = mysql_query("SELECT * FROM member WHERE Username = '".$_POST['Username']."'") or die(mysql_error()); //Gives error if user dosen't exist $check2 = mysql_num_rows($check); if ($check2 == 0) { die('That user does not exist in our database. <a href=loginpage.php>Click Here to Register[/url]'); } $info = mysql_fetch_array( $check ) $_POST['Pass'] = stripslashes($_POST['Pass']); $_POST['Pass'] = md5($_POST['Pass']); //gives error if the password is wrong if ($_POST['Pass'] != $info['Password']) { die('Incorrect password, please try again. Original Password = '.$_POST['Pass'].'. DB Password = '.$info['Password']); } else { // if login is ok then we add a cookie $_POST['Username'] = stripslashes($_POST['Username']); $hour = time() + 3600; setcookie("ID_my_site", $_POST['Username'], $hour); setcookie("Key_my_site", $_POST['Pass'], $hour); //then redirect them to the members area header("Location: members.php"); } } else { // if they are not logged in ?> <form action="" method="post"> <table border="0"> <tr><td colspan=2><h1>Login</h1></td></tr> <tr><td>Username:</td><td> <input type="text" name="Username" maxlength="40"> </td></tr> <tr><td>Password:</td><td> <input type="password" name="Pass" maxlength="50"> </td></tr> <tr><td colspan="2" align="right"> <input type="submit" name="submit" value="Login"> </td></tr> </table> </form> <?php } ?>[/code]
-
Enter the password you originally registered with instead of the already encrypted password in the database.
-
If password entered in form does not equal the password in the database then die(); If it does match, create the cookie and stuff. [edit]No... no... if you use the encrypted password from the database it will only re-encrypt it and it won't match up. Use the original password you registered with[/edit]
-
[SOLVED] How to get a php script to run on a specified date?
dcro2 replied to ted_chou12's topic in PHP Coding Help
If you really want to update the age, you could use [b]crontab[/b] (only on Linux) if your host has enabled it, to run [b]linx[/b] (text browser on Linux). Linx can access your website to run the script. Basically, crontab runs a command at specified intervals, so you can go through all the DOBs and update the age of someone if they are older. If you have cPanel, there is a control for crontab. -
Well, in the login script, you convert the submitted password to md5 and check it against the hash in the database (hash = the password in md5). Which is what I understand you're already doing...
-
Oh.. well, just do this: Replace: [code]$sql = "INSERT INTO member(Username,Password,Firstname,Surname,Address1,Address2,Town,County,Postcode,TelNo,Mobile,Email) VALUES ('$username','$password','$firstname','$surname','$address1','$address2','$town','$county','$postcode','$telno','$mobile','$email')";[/code] With: [code]$password = stripslashes($password); $password = md5($password); $sql = "INSERT INTO member(Username,Password,Firstname,Surname,Address1,Address2,Town,County,Postcode,TelNo,Mobile,Email) VALUES ('$username','$password','$firstname','$surname','$address1','$address2','$town','$county','$postcode','$telno','$mobile','$email')";[/code]
-
If you remove the slashes in the register script, and md5() and insert that into the database then do it equally in the login script. [edit]I'm sorry, I'm wrong... I think.[/edit] [edit2]Wait, I think I'm right. Sorry, I'm confused now.[/edit2]
-
I'm pretty sure strings are stored with slashes in the database, so it might not be the same without slashes.
-
AAAH!! FrontPage! Oh, the evils of FrontPage... and all that IE-only compatible code... Used it once... despised it.
-
Uh... maybe you didn't make the folder with the right permissions?
-
We probably need part of it to understand what $txt[] is...
-
Is $result gotten from mysql_query($query)? [code]$result = mysql_query($query);[/code]
-
Try renaming the field names without spaces in the database and in the script. (Address 1 -> Address1), etc.
-
I don't think we understand most of this. I don't think this is what you meant to do, though: [b]If the form was submitted then:[/b] Nothing; [b]Else If the first name is set and it is numeric then:[/b] Say 'please type in valid first name'; [b]Else If the last name is set and it is numeric then:[/b] Say 'please type in a valid last name'; $firstname= $_POST['firstname']; $lastname= $_POST['lastname']; $age= $_POST['age']; $query = "INSERT INTO info (firstname,lastname,age) VALUES ('$firstname','$lastname','$age')"; mysql_query($query); echo "thank you for your submission"; [b]If none of these things are true then: (What things?)[/b] echo mysql_error(); [b]End If[/b] print_r($query); You see, I don't think you mean to put in the first [b]elseif[/b] because it should have to go if the form was submitted. Let me suggest some code, maybe it's right: [code]<?php error_reporting(E_ALL); require_once ("./mysql_connect_databasetest.php"); if ( isset($_POST['submitted']) ) { if ( isset($_POST['firstname']) && is_numeric($_POST['firstname']) ) { echo 'Please type in valid first name.'; exit; //Only using exit as example } if ( isset($_POST['lastname']) && is_numeric($_POST['lastname']) ) { echo 'Please type in a valid last name.'; exit; //Only using exit as example } //If none of those things are true... $firstname= $_POST['firstname']; $lastname= $_POST['lastname']; $age = $_POST['age']; $query = "INSERT INTO info (firstname,lastname,age) VALUES ('$firstname','$lastname','$age')"; if( mysql_query($query) ) echo "Thank you for your submission."; }else{ echo mysql_error(); } } print_r($query); ?>[/code]
-
Are the From and Reply-To addresses showing up on Gmail?
-
I see something there... the quotes are kind of messed up. Replace [quote]VALUES ('"$_POST['username']."',$_POST['password']."',$_POST['firstname']."',$_POST['surname']."',$_POST['address1']."',$_POST['address2']."',=$_POST['town']."',$_POST['county']."',$_POST['postcode']."',$_POST['telno']."',$_POST['mobile']."',$_POST['email']."')";[/quote] With:[quote] VALUES ('".$_POST['username']."','".$_POST['password']."','".$_POST['firstname']."','".$_POST['surname']."','".$_POST['address1']."','".$_POST['address2']."','".$_POST['town']."','".$_POST['county']."','".$_POST['postcode']."','".$_POST['telno']."','".$_POST['mobile']."','".$_POST['email']."')";[/quote] Basically, you missed some dots and some single and double quotes. One tip though, you assigned each of these POST values to variables earlier, you could use those to avoid confusion: [quote]VALUES ('$username','$password','$firstname','$surname','$address1','$address2','$town','$county','$postcode','$telno','$mobile','$email')";[/quote] And, again, please don't use .inc as a include extension. It's dangerous :D
-
Class variables are all being set to the last variable
dcro2 replied to spelltwister's topic in PHP Coding Help
Variables in classes are accessed without the $, like: [code]$this->infantry = $g;[/code] not [code]$this->$infantry = $g;[/code] -
Try echoing mysql_error() to see what the error is. Also, never use .inc as an include extension, use .inc.php so the source doesn't show up in a browser when that file is accessed.
-
I'm not exactly sure what you mean, but you can't view the source code of just any .php file, they are pre-processed by PHP and you recieve only the HTML generated by the PHP script. ;D
-
Your problem is, first, you're creating an infinite loop by using [code]while($contents = file_get_contents("C2.txt"))[/code] This should always return true and therefore will keep repeating. I don't think you need this for counting anyways. Second, you're using substr_count() on the wrong variable ($put) which is defined as [code]$put = file_put_contents($filename, $selection);[/code] [code=php:0]<?php session_start(); $selection = $_POST['select']; // store location of file in variable $filename = "/home/eland/u1/kbccs/w1009048/public_html/Internet_Pg/C2/C2.txt"; function file_put_contents($filename,$selection) { $fp = fopen($filename,'a+'); if(!$fp) { return false; } else { $write = fwrite($fp, "$selection\r\n"); fclose($fp); return true; } } $put = file_put_contents($filename, $selection); $contents = file_get_contents("C2.txt"); $count = substr_count($contents, 'Mr.X'); ?> <?php echo $count ?> people chose Mr.X[/code] One last suggestion, if you have a MySQL database available and know how to use MySQL, it would be a better choice for poll counts than reading and writing to a file. This would also be better for getting a percent of the people who chose the same option. :) Good luck :D
-
Besides that, after the first condition and $sql, you are forgetting to put a ")" to the end of mysql_query()
-
I'm pretty sure it has something to do with how you get the value of $value1 and $value2: [code]$value1 = "$_GET[subType]"; $value2 = "$_GET[city]";[/code] To get a value from an array ($_GET) you need to put it inside curly brackets ({}) while inside quotes. You also don't need to put it inside quotes, if it's a string, then the $value variable is going to be a string also. [code]$value1 = $_GET[subType]; $value2 = $_GET[city];[/code]
-
Well, for one thing, the correct syntax for SQL INSERT is: INSERT INTO table_name VALUES (value1, value2,....) not INSERT INTO table_name SET column = value, column = value, .... I don't know much about WordPress, so I'll leave it at that.
-
You could use the referer variable in php, $_SERVER['HTTP_REFERER'], but just remember that the referer can be spoofed very easily.