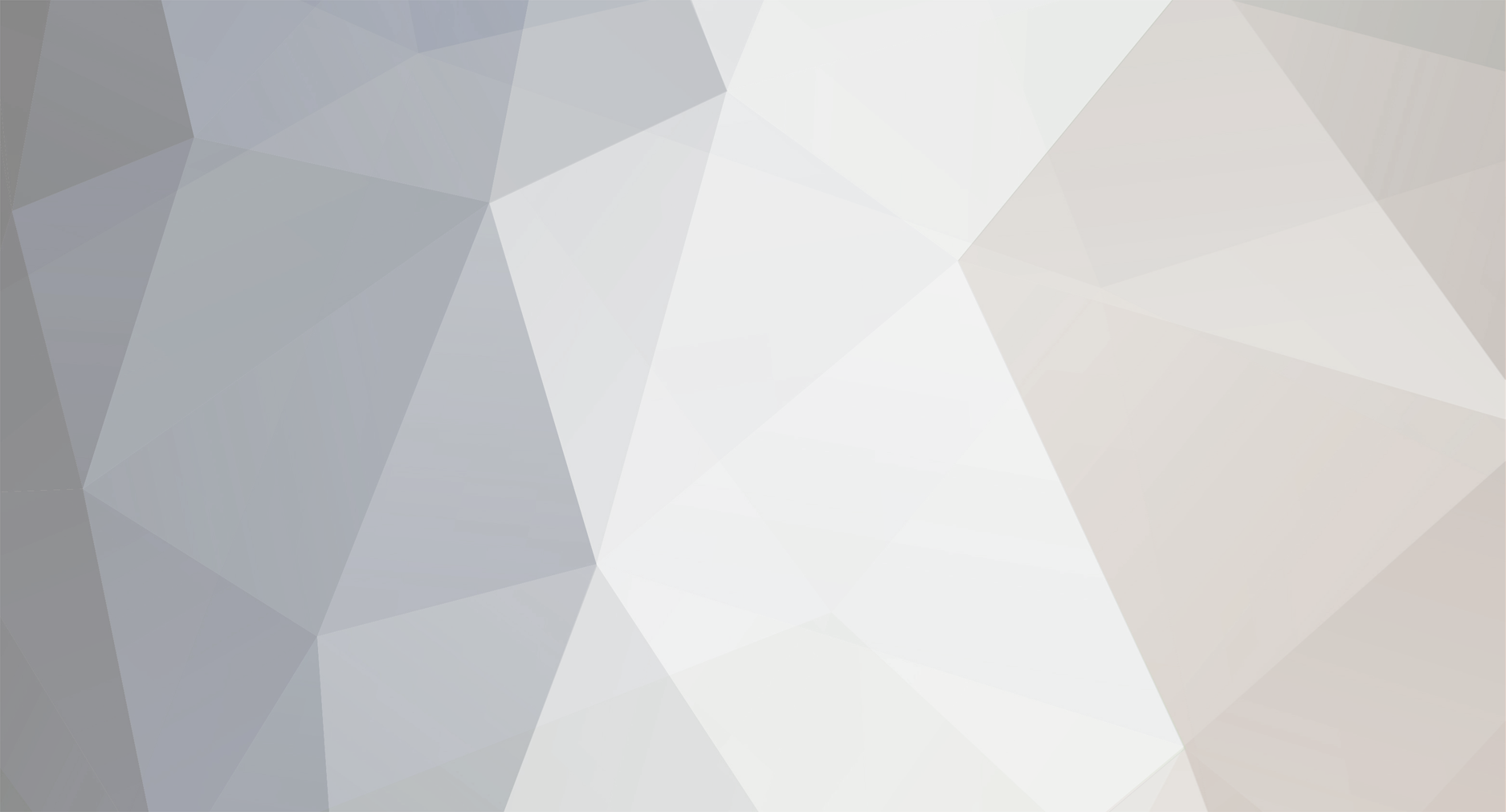
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
Try printing out the values of variables throughout your code, especially any code that may affect the potions data. You will find the bug. IF you don't find the bug, start printing out values of variables that you know could never ever ever ever ever ever EVER possibly be wrong I don't think it's your database query.
-
[SOLVED] extra comma in update sql with foreach
btherl replied to cedtech31's topic in PHP Coding Help
You can make an array and then implode it, like this: $sql = "UPDATE facform SET "; $assignments = array(); foreach ($_POST as $key => $value) { if($key != 'submit' && $value != ''){ $assignments[] = "$key = $value"; } } $sql .= implode(', ', $assignments); $sql .= "WHERE id = $id"; -
I'm a print man myself.. and I think it's time we answered this question once and for all.. .. with a FIGHT TO THE DEATH! And while we're on the topic of miniscule gains in efficiency, single quotes are slightly faster than double quotes.
-
Need some help with a guestbook script urgently
btherl replied to daveclissold's topic in PHP Coding Help
It is indeed a lot to look through. Too much even Did you install all other files along with the php script, according to whatever instructions came with the package? -
Please try the following code (standalone), then try incorporating it (without all the debugging statements) <?php function display($s) { print $s; } $userrow['potionpack'] = 1; $userrow['potions'] = '0,0,0,0,0'; $itemsrow['id'] = 1; if ($userrow["potionpack"] == 0) { display("You do not have a potion pack. You must have that item in order to carry potions without spilling them.<br /><br />You may return to <a href=\"index.php?do=religiousdistrict\">town</a>, <a href=\"index.php?do=apothacary\">apothacary</a> to buy a potion pack, or use the navigation buttons on the left to start exploring.", "Buy Potions"); die(); } elseif ($userrow["potionpack"] == 1) { $potions1 = $userrow["potions"]; $potions = explode(",",$potions1); $add = array(); for($i=0;$i<count($potions);$i++) $add[$i] = ($potions[$i] != 0)?1:0; $potioncount = array_sum($add); print "potioncount: $potioncount\n"; $okaytobuy = ($potioncount < 5)?true:false; if ($okaytobuy) { $id = $itemsrow["id"]; $bought = false; for ($i=0;$i<count($potions);$i++) { print "Checking slot $i.. "; if ($potions[$i] == 0 && !$bought) { $potions[$i] = $id; $bought = true; print "Bingo! Allocating potion\n"; } else { print "nope\n"; } } $potions = implode(",",$potions); } else { display("You do not have room in your potion pack. You must clean out some space to carry more potions or upgrade your pack.<br /><br />You may return to <a href=\"index.php?do=religiousdistrict\">town</a>, <a href=\"index.php?do=apothacary\">apothacary</a> to upgrade your potion pack, or use the navigation buttons on the left to start exploring.", "Buy Potions"); die(); } } var_dump($userrow); var_dump($potions); ?>
-
[SOLVED] PHP-based timer system...even possible?
btherl replied to mem0ri's topic in PHP Coding Help
I don't know what you mean by the "CONSTANTS" page. I'm also not sure what you mean by "call a PHP page" Can you show your code (if you have some). If you are using require() or include() to use a php file which defines some constants, then those constants will only change when the file is edited. -
[SOLVED] PHP-based timer system...even possible?
btherl replied to mem0ri's topic in PHP Coding Help
Yes there is, but probably you should look into "cron" instead. cron can call a script at specific times throughout the day. What you described is commonly called a "daemon" .. it's not common to write one in php but it's certainly possible. -
Does the admin has a user id?
-
It depends on the setting of "register_globals". It used to be usually on on php sites, but now it is usually off by default, as it increases security risks.
-
Password Must have X letters and Y numbers, Best method?
btherl replied to corbin's topic in PHP Coding Help
You can use this, along with a second pass to count up the categories. http://sg2.php.net/manual/en/function.count-chars.php Or a suitable regexp could be $digits = preg_replace('|[^0-9]|', '', $str); $digits_count = strlen($digits); $alphas = preg_replace('|[^a-zA-Z]|', '', $str); $alphas_count = strlen($alphas); -
[SOLVED] Parse error: parse error, unexpected T_STRING
btherl replied to drastixnz's topic in PHP Coding Help
You have two double quotes around "egroupware". Try: if (!($link=mysql_connect("10.1.1.4","egroupware","egroupware"))) -
The short answer is that there is no easy way. Browsers are very defensive about downloading (due to trojans and viruses), and will expect user confirmation on every file downloaded. Some sites get around this by providing a control or a program which does the downloading.
-
It depends on which database version you are using, but try this: SELECT count(*) FROM (select id from Table1 where age = 10 UNION select id from Table2 where age = 10) It might ask you to give an alias for the subquery.. if so, add "AS foo" at the end of the query. Or it may give an error if you are using mysql version 4. There is no need to use distinct, as UNION already does that. If you don't want distinct values you should use UNION ALL instead.
-
That sounds like a database error. Can you post your deleteid.php script? Edit: Oops, I mangled my my first message. What I meant was that you had "a href" at the start, but "url" at the end. "a href" is from HTML, but "url" is the code used on forums.
-
I have a few more questions. 1. Is what you posted your entire script? 2. How do you test to see if the database has been altered? 3. What happens if you add an echo inside the while loop? Do you get any output? If not, it's possible your select is not returning any rows.
-
Can you give more details about how you tested the script? The exact inputs as well as the exact output would be ideal.
-
If I understand you correctly, you can do something like <a href="deleteid.php?id=$id">Delete</a> Then in your deleteid.php $id = $_GET['id']; And then just use $id in your query. PS You mixed HTML and style code in your example. If you start with "<a href=''>" you should end with "</a>"
-
The second query will look for other users with the same mod? $mod = $row['mod']; # From the first query $sql = "SELECT date, mod FROM win WHERE mod = '$mod'"; It wouldn't be necessary to select mod again since you already know what it is..
-
Can you fetch id from the database query? $id = $row['id'] Then in the HTML below you just use $id instead of $_GET[id] I recommend you check your mysql query for errors also.
-
Photos aren't disappearing??? MUST BE SOLVED SOON!
btherl replied to cturner's topic in PHP Coding Help
You should order your results when doing paging, otherwise mysql is free to return them in any order. Edit: Do you want the photos only on the first page, and on subsequent pages display no photos at all? -
The biggest differences are the new functions, and a few incompatible changes. The biggest problem is that some OO code from PHP4 (notably squirrelmail) will not work in PHP5. Also the DOM XML interface changed.
-
Try this instead.. I added {} around the variable and added a missing closing bracket on die() $sql = "INSERT INTO cmscontent where PRIMARY ID = 1(links) VALUES ({$_POST['imagelist']})"; $queryResult = mysql_query($sql) or die("An error has happened: " . mysql_error());
-
Try doing the following to all your input: $var_stripped = stripslashes($_REQUEST['var']); $var_esc = mysql_real_escape_string($var_stripped); Then use $var_esc in your mysql query. With any luck, it'll pop out the other end in the way you want it to If it doesn't, try printing out the variable at every stage of processing, to see where the processing goes wrong.
-
Is this what you want? if(!$ld['email']) { $ld['error'].="Please fill in the 'Email Address' field."."<br>"; $is_ok=false; } elseif(!secure_email($ld['email'])) { $ld['error'].="Please provide a valid email address."."<br>"; $is_ok=false; } elseif(!$allowed_email[$ld['email']]) { $this->dbu->query("select members_id from members where email='".$ld['email']."'"); if($this->dbu->move_next()) { $ld['error'].="This email address is already registered."."<br>"; $is_ok=false; } $this->dbu->query("select banned_email from banned_emails where email='".$ld['email']."'"); if($this->dbu->move_next()) { $ld['error'].="This email address is banned. Please contact the administrator."."<br>"; $is_ok=false; } }
-
Are you using $ss (with register_globals on) or $_REQUEST['ss'] (will work even with register_globals off) ? About the &, it's fine to have & in the html. & is the html entity for the ampersand, "&". It all depends on where that url is.