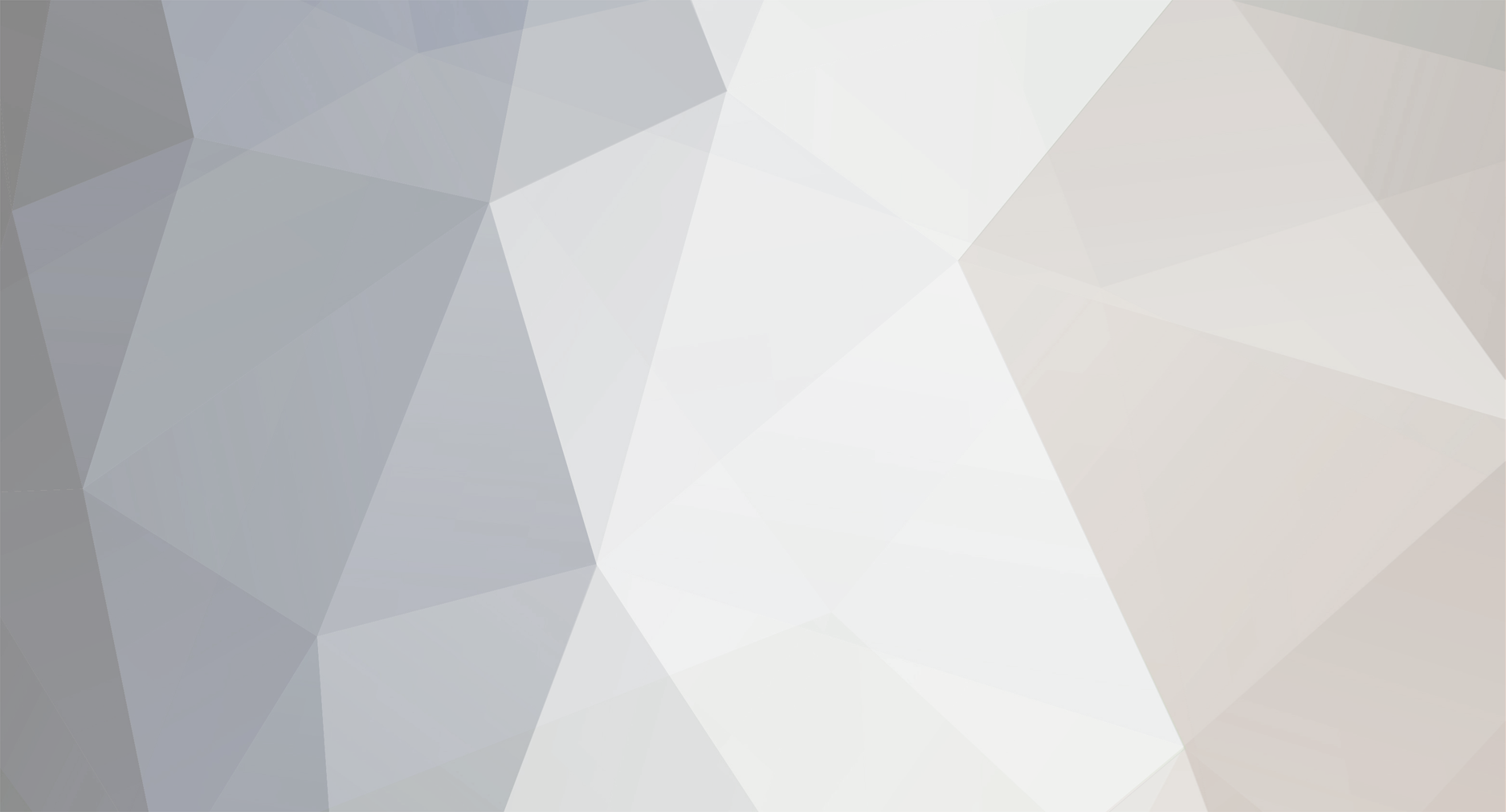
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
Does that script really prompt you to enter your name (in javascript), and then display "Hello there <name>!", where <name> is the name you typed in? I don't understand how it could do that. It definitely doesn't look like the proper way to do it.
-
Googling for "javascript confirmation box" will give you the standard javascript method. There are fancier ways, but I can't help you with them as I don't use them myself.
-
Keeps adding two months to March 31st instead of one
btherl replied to Simmo's topic in PHP Coding Help
Is date("Y-m-d") producing what you expect? Have you printed it out? -
Try making 5 different variables, each one a new Horse(), and create() each one. If you're comfortable with arrays you can put them all in an array, letting you extend it to as many horses as you want. Once you have those 5 object instances you can access the properties of each one.
-
I think you will find the value in $_POST['expMonth']. I'm not 100% sure because I don't use HTML much, although I use a lot of PHP.
-
What are the contents of $_SERVER['PHP_SELF'] and $_SERVER['QUERY_STRING'] ?
-
Yes, the key is the input name and the value is what the user entered or selected. There's also special rules for multi-valued inputs, with an example on the page I linked for "beer[]".
-
That depends on the input type used, but this page covers some common cases: http://www.php.net/manual/en/language.variables.external.php
-
The $_SERVER array is case sensitive, and you need to use uppercase.
-
Ok, so we've confirmed that your query and count code is correct. My gut feeling is that you are actually seeing output from different code, not the code you are editing. There are 2 approaches I use in this kind of situation, and you can use either or both: 1. Keep adding print/var_dump statements until it becomes obvious where things are going wrong. A good idea is to include some text to identify what part of the code is producing the output, so you don't spend hours debugging the wrong code (yes I've made that mistake many times!) 2. If your script is not terminating, add "exit" at various points so you can confirm how far it gets before it hangs. Then you can narrow down the problem area.
-
Can you please copy this code to the top of your script, directly after the database connection is made. Then post the output. $result1 = mysql_query("SELECT * FROM music") or die ("Could not get result1"); print "<pre>"; var_dump($result1); $count = mysql_num_rows($result1) or die ("Could not count rows"); //Changed this to an echo without $count and prints a 1. - Should be greater than 8 echo "Count: $count\n"; exit;
-
Is the code you posted not working? What do you expect it to do, and what is it doing instead of that?
-
What exactly is the problem you are having? What do you expect to see and what are you seeing?
-
The syntax of the first one is a little messed up. But when used correctly, you can use "or die" in the same way as you would use an "if". I would expect the same results when using either approach, but you need to invert the condition, because "or die" triggers on false, but "if" triggers on true.
-
Moving file from current server another server
btherl replied to imanewbe's topic in PHP Coding Help
You could have your script use readdir() or glob() to look at each file in turn, and then connect to each ftp server in turn, uploading all the files. That seems like a logical approach to me. -
If you're doing what I think you're doing, you should set the expiry date to be today's date + 30 days. Then the account is expired when the current date is after the expiry date.
-
Why do you need to minus the expiry dates by 1 every day?
-
All I can suggest is- add print statements everywhere: print "About to check GET[comment]<br>"; if ($_GET['comment'] == 'edit') { print "GET[comment] = edit<br>"; $comment_id_edit = (INT)$_GET['id_edit']; $comment_text = $row_news_comment['hosting_comment_text']; $edit_comment_text = $_POST['hosting_comment_text']; print "About to check POST[hosting_comment_text]<br>"; if(isset($_POST['hosting_comment_text'])) { print "POST[hosting_comment_text] is set<br>"; mysql_query("UPDATE hosting_comment SET hosting_comment_text = '".$edit_comment_text."' WHERE hosting_comment_id = '".$comment_id_edit."' ") or die (mysql_error()); } if (isset($_POST['Submit'])) { echo "<meta http-equiv='REFRESH' content='0;url=/?section=nwcomment&id=".$id."'>"; } echo ("<form action='/?section=nwcomment&id=".$id."&comment=edit&id_edit=".$comment_id_edit."'' id='edit_comment' name='edit_comment' method='post'> <p> <textarea name='hosting_comment_text' cols='50' rows='10' id='textarea' value='$edit_comment_text' ></textarea> <p> <input type='submit' name='Submit' id='button' value='{$lang['BODY_NEWS_COMMENT_SUBMIT']}' /> <input type='reset' name='Reset' id='button' value='{$lang['BODY_NEWS_COMMENT_RESET']}' /> </p> </form>"); } I have added 4 prints above. Keep adding more until you see where the problem is.
-
left join is hanging but join works fine - i need null rows
btherl replied to optikalefx's topic in MySQL Help
Typically a left join will fetch matching rows from the left table (users) and then look up matches in the right table (userpermissions). The index you created allows it to look up those matches in userpermissions very fast. Previously it had to examine all 27,000 rows in userpermissions to see which ones match. A good analogy is a book index - it'll take a long time to read 300 pages looking for a mention of "index optimization", but it's very fast to look at the index at the back of the book and get a list of which pages mention it. -
I don't recommend leaving out brackets. I very rarely do it myself, even if it's just a single line. It's more a matter of taste. I often leave the brackets out if I put debugging code on a single line (and I often indent debugging code to the left of real code, so I know it's to be ignored). Back to error checking - yes you are right. That's what I was trying to say, though I might not have been clear As long as you use mysql_real_escape_string() and put the result in quotes in the query, you are safe from injection, even if you don't check for errors from mysql_query(). But if you do check for errors from mysql_query() and have it email you both the original query and mysql_error(), then you will be able to identify and fix the problem quickly.
-
Any slow-down due to echoing a variable instead of having code in the HTML itself is negligible. Most of the time will be spent in fetching database data, processing the data and parsing the script. Memory usage due to having to store all data until HTML is displayed is usually not an issue as HTML pages tend to be small, and use paging for large data sets. To speed up fetching of database data, the first step is to optimize your queries, including adding (and removing) indexes. If that fails, you can use a caching layer like memcache (Facebook uses this). Processing of data can be sped up by choosing better algorithms and data structures, if some are available. Parsing time can be eliminated by using a cache such as Xcache. All of these don't really matter unless you are experiencing slow response time though. Optimizing before there is a problem often causes more trouble than it fixes, because you don't know where the optimizing is needed when there's no problems. In answer to your error checking question - no, you still need error checking. Queries can fail due to the database crashing, or someone altering the database, or the database getting corrupted, or someone tripping over a network cable, or a million other reasons beyond your control. Your script's job is to take appropriate action, which is usually to send an email to you reporting the error message, and to tell the user that something went wrong and the administrator has been notified. If you want you can make a "my_mysql_query()" wrapper which does all this checking for you. Then you just replace every mysql_query() with your wrapper, and save yourself some time. Regarding santizing of user input destined for mysql queries, your first db query does not escape $id. If you know it should be a number, then verify that first - check for any non-numeric characters and give an error if you find one. Then you can use the sanitized number directly in your query. For strings it is the same, but you have a choice - either restrict the character set so that no dangerous character can be present, or use mysql_real_escape_string(). In both case, it's better practice to validate the character set and give an error first instead of passing the invalid data through to the mysql query and getting the error there. BTW this ties in with your already-tested mysql queries - bad user provided data might make these fail, or even worse, do something else. Eg if I give your script "id=';DROP TABLE users;--" then you query will be this: SELECT title,question,status FROM tickets WHERE tracking = '';DROP TABLE users;--'
-
What does this show: $result = mysql_query("SELECT * FROM signin WHERE email = '" . mysql_real_escape_string("Here's a test.") . "'") or die (mysql_error()); $row = mysql_fetch_row($result); var_dump($row);
-
Hmm, try this code instead of the plain echo: echo urlencode($test);
-
Sorry, I usually don't use PDO .. if you have used a select query elsewhere in the script, you can copy the way that one works.