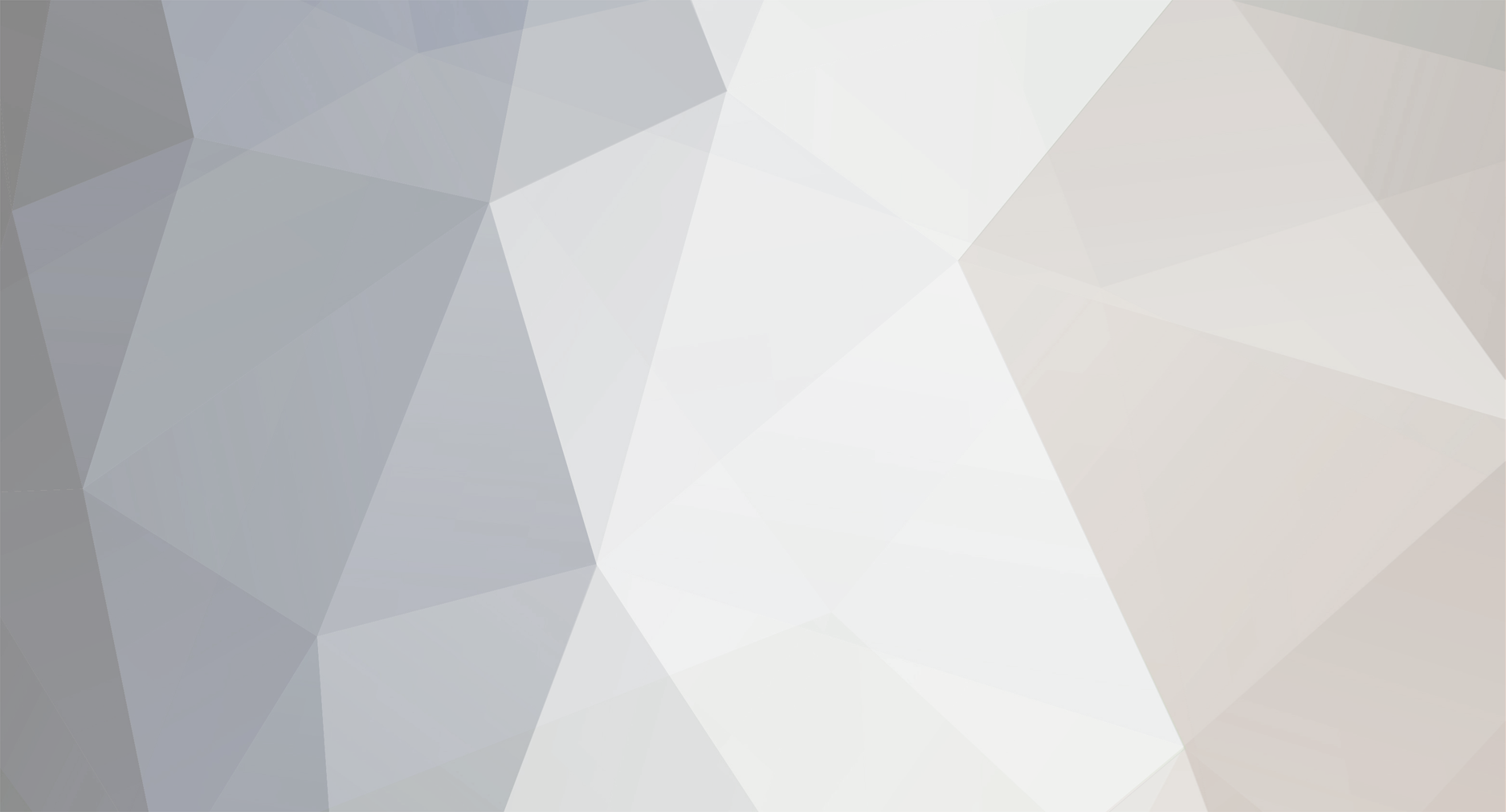
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
Why DB connection fails with config.php but not with direct DB call ?
btherl replied to vincej's topic in PHP Coding Help
Is that require_once() really outside the php tags, or was that because of the copy and paste? -
Period A: 09 - 17 Period B: 13 - 14 End of booking A (17) <= Start of booking B (13) is false End of booking B (14) <= Start of booking A (09) is false Therefore, the bookings overlap. So the algorithm is fine, but the implementation is not working. The code you have looks good here: $sql =" Select * from tModule where Semester_ = '$Semester' AND Day_ ='$Day' AND Room_ ='$Room' AND (End_Time_ <= '$Start_Time' OR '$End_Time' <= Start_Time_)"; The database is booking A and the new booking is booking B. So that condition is EndA <= StartB OR EndB <= StartA, which is the right condition. Can you post here the query which gives the incorrect results, as well as the row from the database which it is supposed to conflict with?
-
Array or session problem in php version 5.2.17
btherl replied to zeekonia2002's topic in PHP Coding Help
Is the old cart data lost even if you don't add another item? If that's the case, the likely cause is that session variables are not being stored. But if the old data is only lost when you add a new item, it's possible there's code somewhere overwriting the cart. It may not be the code you have pasted. If this is the case, you can debug the issue by printing the contents of the cart throughout the script, and see where it changes from non-empty to empty. -
Help with comparing two arrays to create a third array.
btherl replied to tnhillbilly's topic in PHP Coding Help
Congratulations, that's a pretty tricky task to do in SQL! So you're taking advantage of that count(PeopleID) will always be the total skill count, but count(e.SkillID) will skip null values, so it'll be the total count of skills matched by that person. If the counts match, then that person has all required skills. -
If you haven't got something equivalent, I would recommend using LiveHTTPHeaders in Firefox. It will show you exactly what is sent, so you can replicate it with curl.
-
That is pretty cryptic code there. I'm not sure why you had trouble, as any variable not declared global is local to a function. If you call the same function recursively you will get new copies of those variables for each call. The common situations where variables are not function scoped are: 1. $this->var is object instance scope 2. class::var is class scope 3. "global $var" makes $var global scope for that function only (You can still use $var locally within other functions) 4. When dealing with external resources like databases, some sharing may occur. But typically, a resource named with a local variable (such as $result = mysql_query(...)) will be local to the function only.
-
Hmm, so is the problem that you have some threads (aka mains), and a bunch of replies, and you're not sure how to put those replies into the tree-like structure in your first post? The first way I think of is to do it like this: For each thread t For each reply r Is r a reply to t? If yes, put it in a replies array under t For each reply rr Is rr a reply to r? If yes, put it in a replies array under r .. and recursively call a function that looks for replies to rr, and so on until you get to a reply with no replies End for each (rr) End for each ® End for each (t) Then you have everything in a tree structure. This algorithm is not the most efficient, and it's probably better to index the replies by what they are replying to first, but it'll work. Then you can sort your threads by stickiness and "last reply", and the replies by "time started". Have you already worked out how to calculate "last reply" time for the threads? If not you can calculate it while creating the tree structure above - each time you add a reply under the thread (or a reply under a reply under a thread, and so on) then you can remember which reply has the most recent time. Then once you've found all replies, assign that time to the entire thread as the "last reply" time. The basic strategy I'm using is to put everything into the right structure first, and calculate any additional metadata (like the most recent reply for a thread) first. And then the sorting should be easy (in theory).
-
I hope you are sorting threads rather than posts.. otherwise it could get very complicated. Regardless of which you are sorting, if you can express your sorting by comparing two objects and saying which should go first, then the way to go is usort() with a custom sort function. But if your sorting is not that simple (such as sorting posts but expecting them to end up with a thread structure), then I wouldn't even attempt that - I would put them into threads first and then sort.
-
Help with comparing two arrays to create a third array.
btherl replied to tnhillbilly's topic in PHP Coding Help
So you want to know which people have all skills required for an equipment item? You could start with a left join from equipment to people: SELECT EquipID, PeopleID FROM Equipskills e LEFT JOIN Peopleskills p ON (p.SkillID = e.SkillID) If a person is missing a skill for an equipment, there will be a row for that equipment/person combination with "p.SkillID is null". If the person has all skills, there will be no row with "p.SkillID is null" for that equipment/person combination. So to further reduce this to just "people with the required skills": SELECT EquipID, PeopleID FROM (the query above) AS subq GROUP BY EquipID, PeopleID HAVING MIN(CASE WHEN PeopleID IS NULL THEN 0 ELSE 1 END) = 1 There's no aggregator which will directly tell you if there's a null present, so i'm converting the nulls into 0s and the non-nulls into 1s, then finding the minimum. If any nulls are present the minimum is 0 (and the person is not skilled for that equipment), otherwise it's 1 and the person is skilled. If you try this query and it doesn't work please show me the error message (or the result set if the result set is incorrect). -
Ok. In PHP that will be: if ($user_class->strength > $attack_person->strength) { # Attacker wins $damage = $yourhp * 0.2; # Damage is 20% of current health? Or of total health? $yourhp *= 0.8; # Set hp to 80% of old total $theirhp = 0; } else { # Defender wins $damage = $theirhp * 0.2; $theirhp *= 0.8; $yourhp = 0; } And then you would use the other code you already have. There is no need to use while() if the battle only has one round.
-
What happens when you try that code?
-
Ok, so a battle is like this: If attack strength > defence strength then attacker hp = attacker hp * 0.8, defender hp = 0 else attacker hp = 0, defender hp = defender hp * 0.8 Does that look right?
-
Maybe you can post a var_dump() of your array structure, as I'm not clear on what it is. "Variable properties" do work, such as $propertyname = 'bar'; $instance->$propertyname being resolved to $instance->bar
-
According to the comments on that site it's \p{Cyrillic}, and it will only work if PHP was compiled with a regexp library which supports that. But you can still do it like this: $pattern = "/[абвгдАБВГД]/"; preg_match($pattern, $str); And if you list every possible cyrillic character in there, it'll work..
-
Maybe you need a clearer statement of how a battle works. When does a player "win"? When is a player "hit"? How do you decide who gets hit, and how do you decide who wins? Does a battle have only 1 hit?
-
Does $this->$objArrayName work?
-
The comments here look relevant (see below in the responses where someone discusses PHP and Cyrillic): http://stackoverflow.com/questions/4907342/javascript-regexp-cyrillic-pattern Also are you sure your cyrillic string is encoded in UTF8 when it goes into the preg_match()?
-
Looks like you have a lot to learn! Especially as most tutorials deal with mysql rather than sqlite. I would start out with a simple task like displaying a static html table from php. Then display data from the table using fixed conditions (for example, display week 1 only). Then extend it to display multiples weeks, and then to take the page number from a form. You can probably find some useful information in the comments on this page: http://php.net/manual/en/book.sqlite.php
-
Is it the querying from the database or the paging (ie displaying page 1, page 2 etc) that you are unclear on? Or both? Have you got any code so far?
-
Most likely simple fix, script doesn't update MySQL query.
btherl replied to Clandestinex337's topic in PHP Coding Help
You can do it like this: header("Location: /index.php"); exit(0); The biggest issue here is if you want to display a notification that the post was deleted. For this reason you may want to use meta refresh instead: http://en.wikipedia.org/wiki/Meta_refresh This lets you set a delay of a few seconds before the redirection, which can be better than the header redirect, which redirects without displaying anything. -
nicholasolsen, please see the following on the manual page for session_unset(): As the OP was using $_SESSION (and most PHP installations these days do), session_unset() is not the correct function to use. Also, the session_destroy() manual page says that session_unset() is deprecated, and specifically instructs to use it only for code that does not use $_SESSION. Edit: I would also like to point out that these are the things you won't find easily by using google.
-
Most likely simple fix, script doesn't update MySQL query.
btherl replied to Clandestinex337's topic in PHP Coding Help
Ok, I had assumed you had the old setting to make $_POST values turn magically into local variables on. But if you don't, you will need to use $_POST['name'] and $_POST['password'] when you want to refer to the data from the form. An easy way to do this is to add this code inside the second "if": $name = $_POST['name']; $password = $_POST['password']; Once you've got it all working, you might want to consider using mysql_real_escape_string() on your variables that go into the database. This is essential if this script is going to be accessible on the internet, as otherwise people will be able to take control of your database. But I would get it working properly first. -
No worries, it's a bit of a confusing concept. Just think about the possibilities: 1. Booking A is completely before Booking B (End of booking A <= Start of booking B) 2. Booking B is completely before booking A (End of booking B <= Start of booking A) 3. ??? What is possiblity 3? Well if one booking is not entirely before or after the other, then they must overlap. It doesn't matter how they overlap - you already know there is a conflict, and you can report it back to the user. So you just need to check those two conditions: If End of booking A <= Start of booking B OR End of booking B <= Start of booking A, then the bookings do not overlap. Note that that is "OR", not "AND".
-
I'm not aware of any rule that only moderators can correct members. There's also no rule that members can tell other members what to do. So I would say common sense and politeness should take priority here. Especially since he gave you the wrong answer, it would have been better if he said "I found this on google" rather than "google next time".
-
Most likely simple fix, script doesn't update MySQL query.
btherl replied to Clandestinex337's topic in PHP Coding Help
The variables can have different names for different purposes. One is "name from the db" and "password from the db". The other is "name from the form" and "password from the form". So: <?php @mysql_connect('localhost', 'root', '') or die("Could not connect to Mysql Server. " . mysql_error()); @mysql_select_db('tutorials') or die("Could not connect to Database. " . mysql_error()); if(isset($_GET['edit'])) { $id = $_GET['edit']; $query = "SELECT `username`, `password` FROM `users` WHERE `id` = '$id'"; $result = mysql_query($query); $row = mysql_fetch_array($result); $db_name = $row['username']; $db_password = $row['password']; } if(isset($_POST['edit'])) { $id = $_GET['edit']; $query = "UPDATE `users` SET `username` = '$name', `password` = '$password' WHERE `id` = '$id'"; $result = mysql_query($query); echo $query; if(!$result) { echo mysql_error(); }else{ echo 'updated post'; $db_name = $name; $db_password = $password; } } ?> <form method="POST" action="" > <input type="text" name="name" value="<?php echo $db_name; ?>" /> First name <br /> <input type="text" name="password" value="<?php echo $db_password; ?>" /> Last name <br /> <input type="submit" name="edit" value="edit" /> </form> The two changes are to use $db_name and $db_password for the data fetched from the database, and also to overwrite the db variables when an updated name and password is written to the database.