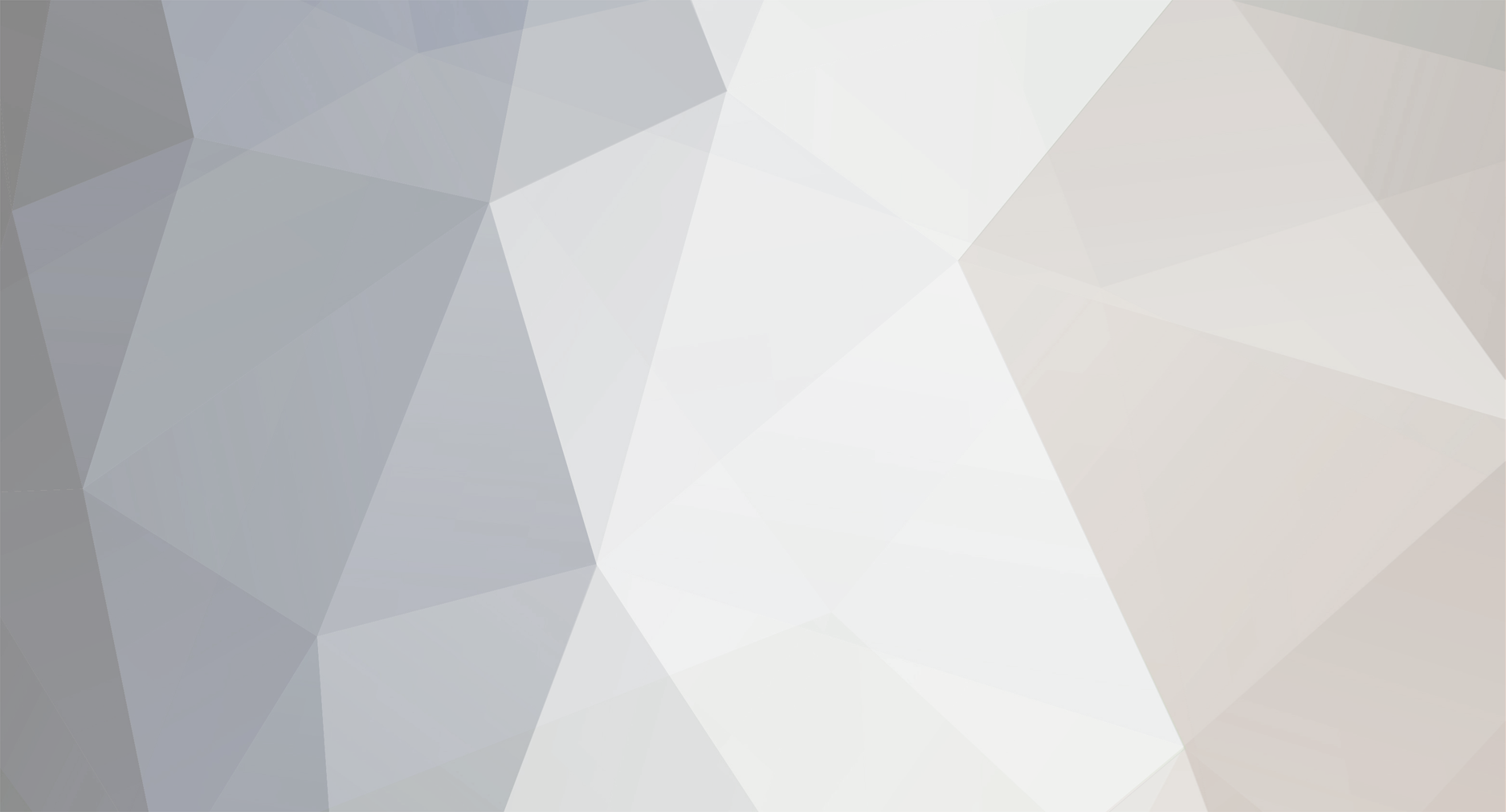
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
Try this: if (preg_match("|$file|i",$_SERVER["HTTP_ACCEPT_LANGUAGE"]) && !$use_lang) $use_lang = $file; The preg functions need a delimiter around the expression being searched for. That's because it came from perl, which expects delimiters. I've used "|" as the delimiter here. And the "i" at the end means "case insensitive".
-
PHP with HTML = Bad....How do I stop this habit?
btherl replied to 3raser's topic in PHP Coding Help
Using a templating system like Smarty makes it easy to keep the php and HTML seperate. It'll make both your php and HTML much easier to read. -
OK, I think the "displays folder only" issue may be here: foreach ($m_list as $m_object) { if (is_dir($m_object)) { // Check if current object is a directory. $dir_link = $m_object; $headers[] = array($m_object => "index.php?s=". $n); // Assign a unique link for each of the folders. $sublist[$n] = subdir($m_list, $dir_link); // Acquire the list of all objects inside a folder. } $n++; } Here you have a condition for is_dir(), but there is no "else" branch to handle the things which are not folders. I think you general algorithm should be: 1. Get path from user input, or default to "/" 2. Get list of all folders and files in that path 3. Display them (making sure folders are links which include the full path name to that folder). Unless you want those folder links to be expandable without a request back to the server. IE the expansion and collapsing is done in javascript, and the entire directory structure is provided to the web browser with each request. If that's the case then you do need to read them all.. If that is what you want to do, then your subdir() is going to need another call back to either subdir() or read(), so it can continue going down the levels until it reaches the end. By far the simplest option is to send the current path back to PHP and have it generate the list for just that path.
-
More or less. While I trust php's "isset" and it is useful for exactly what you describe, I don't trust php's "is true". The big problem with it is that "0" is false. But 0 is a perfectly good number, and it's most definitely "not empty", even though both !0 and empty(0) are TRUE!! This quirk results in so many bugs, usually forms that mysteriously fail when particular input results in a 0 being tested for true-ness. And the biggest gotcha with isset() is that false and 0 are "set" values, because "set" refers to the variable itself being set and not the value.
-
http://php.net/manual/en/types.comparisons.php isset() is typically used to see if a variable was passed through a form, even if it was passed as empty. But the second piece of code you've got checks if the value is "true" or not, which is defined according to some arcane rules listed in that table.
-
It's a good idea to terminate the script with die() or exit() if something doesn't work. "1. It only displays folders at the first level. (That's not a big issue. I think I know how to fix it)" - What is it supposed to do instead? "2. If you click on a folder, it will display its' contents under ALL of the folders, not only under the specific one. I'm not sure what condition I can use to fix that." - How does the link clicked on by the user tell your script which folder to use? Eg the link could be "/?path=foo/bar", and then the path is available as $_GET['path'] or $_REQUEST['path']. Then you can use that in your code. "3. It only scans one sublevel. For example it scans folder/subfolder. And I need it to scan any number of sub-levels, such as folder/subfolder/subfolder/subfolder/... " - Do you need to scan every level every time? If so, does that mean you are displaying links to every subfolder on a single page?
-
You can make the list of all the users, then find where the single user is in the list. Have you used arrays before?
-
First record is blank when I display xml on php page
btherl replied to cjackson111's topic in PHP Coding Help
I would do it like this. There is just one line added. "continue" means "jump to the next item in the loop", more or less. foreach( $xml as $event ) //foreach( $xml as $user ) { if (!$event->event_date) continue; # New line - skip event if it doesn't have a date echo '<tr valign="top" bgcolor="#000000">'; echo '<td width="300" background="image/bg_results2.gif"><font color="#FFFFFF" size="2" face="Verdana, Arial, Helvetica, sans-serif"><strong>'; echo ''.$event->artists->artist->artist_name.''; //artist_name echo '</strong><br> '.$event->event_date.'<br> Live at '.$event->venue->venue_name.'<br> '.$event->venue->venue_city.', '.$event->venue->venue_state.' '.$event->venue->venue_zip.''; echo '</font></td>'; echo '<td width="290" align="right" background="image/bg_results2.gif"> <a href="'.$event->event_url.'" target="_blank"><img src="image/detail_button.gif" alt="Event Details" vspace="5" width="71" height="25" border="0"></a>'; //event link //echo ' '; if (!$event->ticket_url){ } else{ echo ' <a href="'.$event->ticket_url.'" target="_blank"><img src="image/ticket_button.gif" alt="Get Tickets" vspace="5" width="71" height="25" border="0"></a>'; //ticket link } echo '</td>'; echo '</tr>'; } -
In the post above I added a variable $rank. This tells you what rank each user is.
-
Please post your current code
-
First record is blank when I display xml on php page
btherl replied to cjackson111's topic in PHP Coding Help
Maybe your parser is giving you a blank entry at the start? You could check it and ignore any blank entries. Or it may be parsing something at the start which is not an event. -
You could do: $result= mysql_query("SELECT * FROM `grpgusers` ORDER BY `grpgusers`.`strength` DESC"); $rank = 1; while($line3 = mysql_fetch_array($result, MYSQL_BOTH)) { print "Rank $rank: "; # Display data from $line3 $rank = $rank + 1; } $rank will increase by 1 each time through the loop.
-
If it's a number then you don't need the quotes (though it's a good idea to use them, as well as mysql_real_escape_string(), to avoid SQL injection). If it's a string and it works, then the quotes ARE getting added somehow. I couldn't tell you how without seeing the code.
-
First record is blank when I display xml on php page
btherl replied to cjackson111's topic in PHP Coding Help
Please show the specific code. -
Try this: WHERE page_name = '$page' The difference is quotes around $page.
-
Do you want to find the user with the 3rd highest strength? Eg User A: Strength 100 User B: Strength 90 User C: Strength 85 <-- this one User D: Strength 80 And is strength stored in the grpgusers table as "strength"?
-
No you're not worrying too much Each variable that comes from $_GET needs to have mysql_real_escape_string() applied to it once and only once. Then you can safely insert it into your query (inside quotes, as you are doing already). If you don't do that, then your users can gain full control of your database. htmlentities() is used when you want to display data back to the user. It's not needed before doing an SQL query. Usually it's used on data you have just fetched from the database. For example: function sanitizeString($string) { return mysql_real_escape_string($string); } $description = sanitizeString($_GET['description']); $query .= " WHERE description like '%$description%'"; This is safe. You also need to learn what magic_quotes_gpc is and whether or not it's enabled on your server.
-
This can be done in apache, using the referrer header. I don't think you can do it in PHP unless you tell apache to direct all your swf requests to a php script, which can then decide if it's going to serve the real file or serve an ad. A determined user can still download your swf files directly by faking the referrer, but it doesn't sound like that's the case you're interested in. People clicking through on hotlinks will still get intercepted and shown ads.
-
Try changing the limit to 2, 1. But that won't take into account if some users have the same strength.
-
How about this: mail($email, $subject, "", $headers); Do you want to send to one email address or two?
-
Let's say I'm writing a collection of RSS feed fetchers. Usually they use the same format, but some of them have quirks. Each has a different url, and may have different settings. I could implement them as a single parent class called RSSFeed, with a subclass for each site. The parent class defines a common interface and also has code for handling the most common format (or formats). In most cases I can implement a new feed by just subclassing the parent class and changing the url only. If a new feed has some kind of quirk, I can override whatever methods are affected. There are problems with this approach - overriding the parent method may lead to code duplication, and it may be better to alter the parent methods so they support the new format. But in general it allows me to implement a new feed like this: class TheAgeFeed extends RSSFeed { private $url = 'http://feeds.theage.com.au/rssheadlines/top.xml'; } And that's it. Now to use the new feed: $feedObj = new TheAgeFeed(); $headlines = $feeObj->fetch(); Assuming fetch() is defined in the parent, and fetches and parses a standard RSS feed. So that's an example where inheritance works well. If you're not using the fancy features like inheritance, then the main benefit of OOP is that it helps to organize things, and allows you to make visibility rules. It's especially important in PHP as PHP has no other mechanism (apart from resources which have to be created in C code) to declare that a variable is a specific type of thing, such as an RSS feed. Then if you try to use the wrong type of object in the wrong place it'll tell you. That means PHP is doing the debugging for you, by checking that your code follows the rules you set for it.
-
postfix might be overkill. You would probably do better with a mail library that sends over SMTP directly using whatever SMTP relay Gmail gave you for outgoing mail. Such as this: http://pear.php.net/manual/en/package.mail.mail.factory.php It has an "smtp" back end which won't require any mail software installed on your server.
-
Googling for "how to replace iframe content" gave this, which answers your second question: http://www.gotfusion.com/tutorials/tut.cfm?itemID=327 If you make an attempt at the navigation menu we might be able to help. Otherwise all I can suggest for that is to look for tutorials in google, eg "display table in php" or "display html list in php"
-
If you download a program like Filezilla, you can login to the ftp server using the same details you are using for ftp_connect(), and it will display the path for you. It's likely the path will be just "/", but it depends on how your host's ftp server is configured. Once you find out the correct path, you can use that with ftp_put()