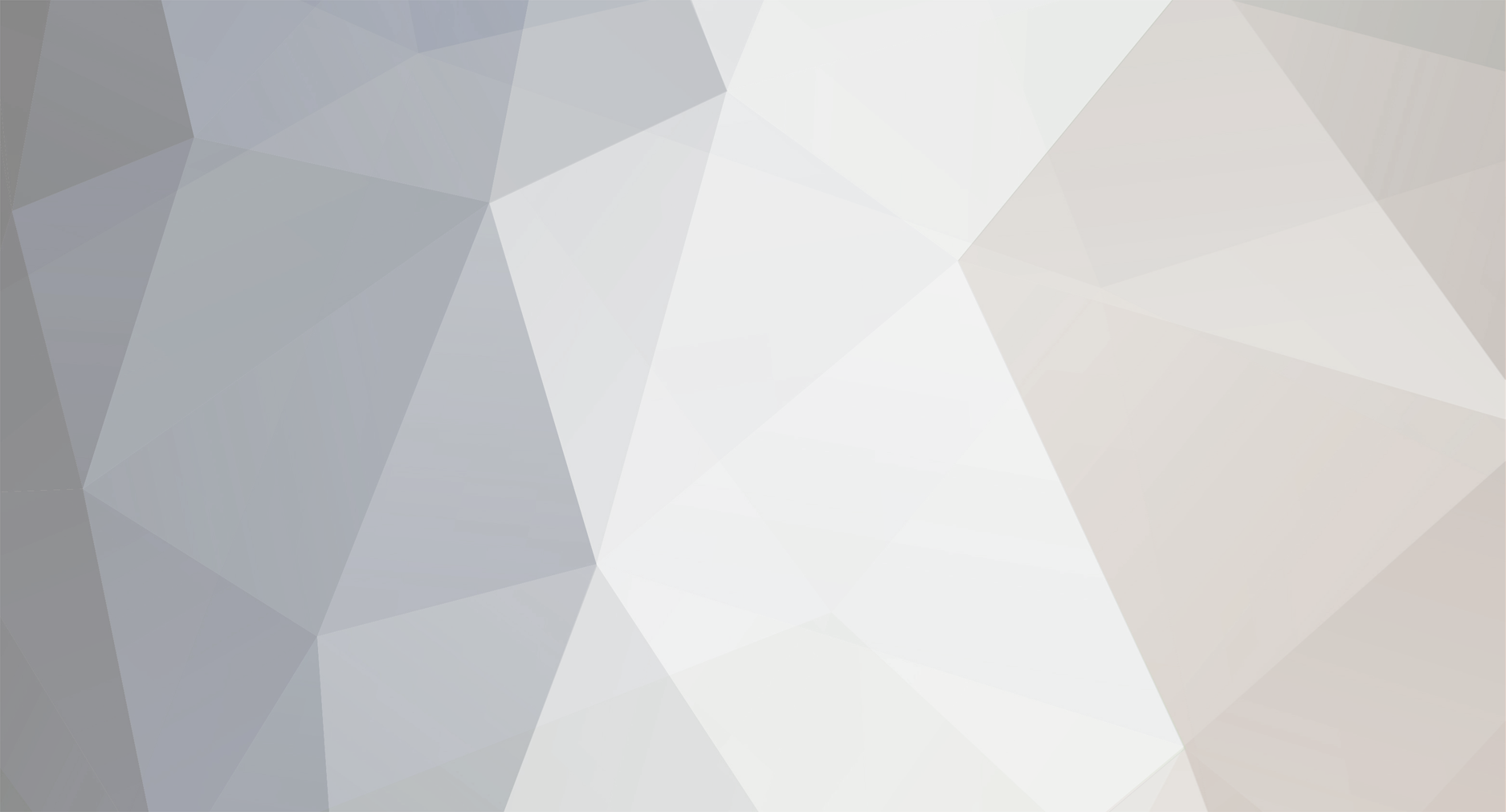
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
Those are called ID3 tags. I've never used it from php before. There is a PECL library here which you may be able to use: http://www.php.net/manual/en/book.id3.php
-
Also, when using a function like trim(), you need to assign the result into a variable. There are some exceptions to this rule, such as the sorting functions, but usually you need to assign the result. function checkStringText($str) { // checks proper syntax //if(preg_match("/^([a-zA-Z0-9])+([a-zA-Z0-9\._-])*@([a-zA-Z0-9_-])+([a-zA-Z0-9\._-]+)+$/" , $firstname)) { //Removes white space, null, tabs, new lines from both sides $str = trim($str); // Strips HTML Tags $str = strip_tags($str); if (preg_match("/^[0-9]+:[X-Z]+$/D",$str)) { return true; } return false; }
-
There's numerous javascript implementations of this. If you google for something like "javascript check multiple checkboxes" you'll see quite a few. If you want specific advice on how to implement it for your page, I think we would need to see the html you are using.
-
It looks like your file is using windows style end of line characters instead of unix style. Can your editor save the file with unix style end of line characters? Maq, php-cgi itself gave him the first error message, it definitely exists
-
Yes it is possible, and that query will do it. If you need the other data items as well then things can get tricky. It it possible for the same user to have two entries with the same score? The answer to that determines how the query will look. It's simpler if they cannot have two entries with the same score, as then (user_id, score) is a unique identifier for the row you want to display. Otherwise you'll need a way to choose between two equally high scoring rows.
-
If you want user and their highest score only, you can do this SELECt user,max(score) FROM table GROUP BY user ORDER BY max(score) DESC
-
It sounds like you want the MSG_DONTWAIT flag, aka "non-blocking". That will let your code continue even if there's nothing to read from the socket. Have you looked at socket_select() ? That's one way to deal with the problem of knowing when to read and when to write to a socket. I still don't know what you mean with "include". Can you show me that code?
-
At a wild guess, I would say either 1. Your form is using the get method, not the post method 2. Or, your input tags have different names to what you are looking for in the script 3. Or, the input tags are not inside the form you are submitting
-
The additional GET lines you see from LiveHTTPHeaders are additional requests made by the browser, based on the HTML. If you are using curl you won't be requesting those, because curl just does a single request (and follows 30x redirects if configured to do so). Curl doesn't process the HTML and request additional items like images and javascript. What you can try is parse the HTML and find where that captcha request is generated. As long as it's not generated by javscript .. and good luck trying to decode the captcha image
-
What file are you including to send the message? I don't understand how including a file would slow down sending data over a socket.
-
PHP can become unstable if you deal with large amounts of data, and with complex structures, especially those involving references. There's a hard limit on the number of references a single variable can have, and if you exceed it php will just die. The limit is around 65k, so you won't meet it unless you're doing something unusual. I've encountered instability when dealing with arrays with around 100k entries, where memory usage is getting into the gigabytes. As long as you stick to small operations though, it's rock solid. We use it in production systems and never have problems, except in those unusual circumstances listed above. gizmola seems to have covered the other things I would have mentioned.
-
Huh? What I provided was the actual implementation details you would need to do what was described in btherl's reply. Yours was considerably scarier Even though it is the same thing. It actually reminds me of this: http://www.ariel.com.au/jokes/The_Evolution_of_a_Programmer.html
-
I don't know how to do that in sql. But you could do this: 1. Read all the results into an array 2. Go through the array counting how many times each term appears on artist and title, and add up the results, then store that back into that array entry. You could do this by breaking artist and title into words and checking each word. 3. Sort the array by number of matches descending. This could be done using usort(), which is very powerful but can take some time to learn how to use..
-
Ah, in that case you could do something like this: if (preg_match('|^[a-z]$|i', $w) which means "One character from a-z which is both at the beginning (^) and the end ($) of the string", another way of saying "a single alphabetical character". or if (strlen($w) == 1 && ctype_alpha($w)) It's much easier if you compare a fixed regexp against the word, rather than using the word as a regexp against a fixed string. Then you don't need to check for special characters in the input. Plus, your original regexp would match things like "ab" or "nop"
-
I would try Ascii or ISO-8859-1 if those are options. "Plain text" is another good one if that's available.
-
Hang on, what exactly is your preg_match() supposed to do? I don't understand it. If you can tell me what it's supposed to do then I can give you some code that does it. Also this might not be doing what you expect: if (!$var=="") That's saying: If (not $var) is equal to "" Not If $var is not equal to "" which would be: if ($var != "") So it's taking the boolean inverse of $var, and comparing it to the empty string
-
Is sharepoint what you use to edit the files? You might want to try googling "sharepoint bom" and see if any of those help.
-
Do you want to be able to search for those characters? If not, it's probably easier to remove them and just allow letters and perhaps numbers through. preg_match() has a lot of special characters. The reason "_" gives you everything is that "_" means "any character" for the mysql like operator. You'll see the same if you searched for "%" which means "any number of any character". What i mean with brackets is changing this $query .= $sep . "artist like \"%$w%\" OR title like \"%$w%\""; $sep = ' AND '; to this $query .= $sep . "(artist like \"%$w%\" OR title like \"%$w%\")"; $sep = ' AND '; That makes it clear to mysql and to people reading your code that you want each word matched at least once in either artist or title, and not any other interpretation, such as 'artist like "foo" OR (title like "foo" AND artist like "mario") OR title like "mario"' I don't know if it actually will affect behaviour of the query, but it's generally a good idea.
-
You can store the poll structure with a table having user id, poll id, poll name, option number, answer. Then you can store one row for each possible answer in the poll. Answers to the poll would be stored in another table having user id (of the answerer, not the poll owner), poll id, option number. If you want to make the interface for designing the poll simple to code, you can give them a fixed number of text boxes, one per answer, say 10 or 20 boxes. You can name them "poll_answer[]" for example, and php will give you an array called $_POST['poll_answer']. It's the "[]" in the variable name that tells php to make it into an array.
-
That's a byte order marker: http://en.wikipedia.org/wiki/Byte_order_mark Your editor probably isn't showing it because it understands the marker and is letting you edit the file as UTF-8. I can't suggest a solution unfortunately as I'm not familiar with how BOM files work with PHP.
-
The "case" have to go directly inside the switch. You can't have code inbetween the switch and the case, and that especially applies to "while" loops. Also the syntax for case requires ":", not ";" I recommend you start with writing simple switches, which do something basic like printing out "This is case 4". Once you have mastered those, try adding more code and building up to something bigger.
-
Did you add brackets when using "AND"? If not it may not act how you expect. If you want the results you mentioned in the first post, ie all results with either "super" or "mario", then you should use "OR". If you want all results with "super" and "mario" then you should use AND but also add brackets around each word.
-
Right now you have this: $query = "select * from `sheets` where `active`='yes' AND (artist like \"%$trimmed%\" OR title like \"%$trimmed%\") ORDER BY `artist`"; Instead, try something like this: $query = "select * from `sheets` where `active`='yes' AND ("; $words = explode(' ', $trimmed); $words = array_map('trim', $words); $sep = ''; foreach ($words as $w) { $query .= $sep . "artist like \"%$w%\" OR title like \"%$w%\""; $sep = ' OR '; } $query .= ") ORDER BY `artist`"; The important part there is the loop to build the query by adding "OR" conditions for each word in the query string, instead of just one pair of conditions for the entire string.
-
What you would do is create a php script called "mark_user_as_timed_out.php". That script is php, so it can do anything you want, including updating the database, which you couldn't do directly from JS. If you have the user's information in a session then you can use that in mark_user_as_timed_out.php
-
You can send the data in a post. There's actually a standard for uploading files, the technical details are here: http://www.php.net/manual/en/features.file-upload.php