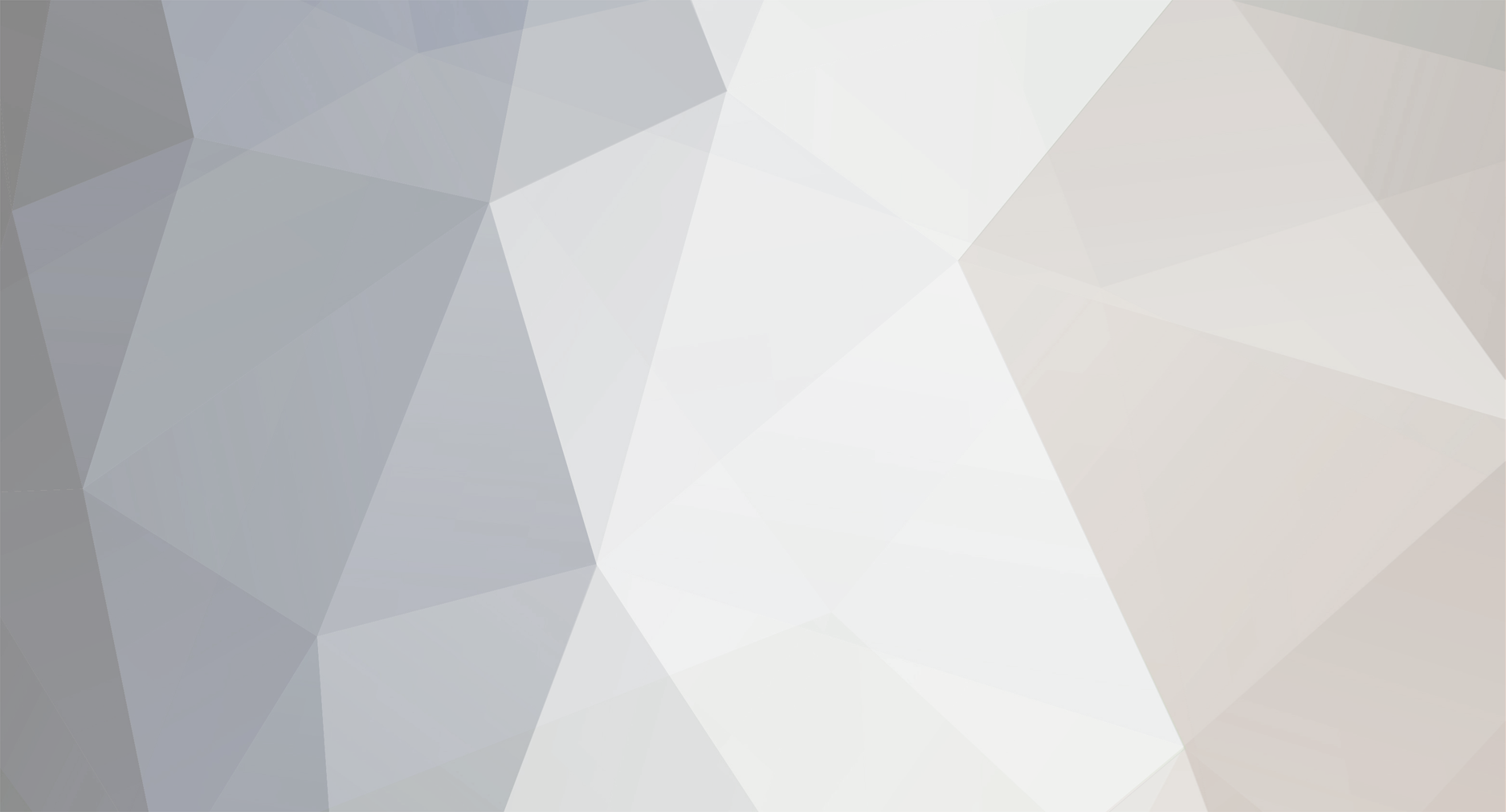
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
recommened method of embedding PHP with HTML
btherl replied to cbassett03's topic in PHP Coding Help
There's no difference in security, it's a matter of style. I'm assuming you meant this for the second example: <p><strong>Hello World!</strong></p> <?php echo "<p><strong>You are $age year(s) old!</p>"; ?> I use Smarty templates myself, which are a lot more flexible but they are more effort to set up. They allow a very clean separation of the display logic and the back end logic. -
pengu, the first query finds matching rows in both tables and counts those, whereas the second query (without the join) counts ALL rows fitting the "where" condition, even if they don't match a row in the other table. So it will work only if there's a 1 to 1 correspondence between ids in each table, which is unlikely when one table is a basket and the other is a product list. I think what the OP actually wanted was this: SELECT COUNT( b.id ), SUM(p.search_price) FROM basket b INNER JOIN products b ON b.id = p.id WHERE p.for_sale = "yes" AND b.ip_address = "'.$_SERVER["REMOTE_ADDR"].'" GROUP BY b.ip_address That gives the total product count and the total price. It doesn't get the unique item count, but I'm not clear on exactly what was wanted.
-
INNER JOIN means "find matching rows in each table" LEFT JOIN means "find matching rows in each table, AND also include any unmatched rows in the LEFT table" RIGHT JOIN means "find matching rows in each table, AND also include any unmatched rows in the RIGHT table"
-
Your "if" should be outside the "while". Because the "while" will never get to run if there are no results, it will just get skipped right away. Then inside the while you will have no if, you will just have the code that is supposed to run for each row (which is currently in the "else")
-
That looks right to me. As long as you only need a single level of decoding. You might want to check the result of that preg_replace() to make sure it is what you expect it to be. Russel, if you're replacing with X then you don't need to worry about character sets luckily Unless there are some html entities that appear as more than one character, that's a possibility .. but I can't think of any.
-
If you really have "®" in your db, you might need to html_entity_decode() twice to get the actual character. Keep in mind that ® and ™ are not going to map to ascii characters though .. Instead of decoding, you might want to just replace "&...;" sequences with a single dummy charater, so you can get the length count correct. Then use the original string for displaying.
-
What types of problems are appropriately solved by create_function() ?
btherl replied to jayarsee's topic in PHP Coding Help
usort($KeywordSuggestions, create_function('$a,$b', 'return strcmp($a["vertical_name"], $b["vertical_name"]);')); Here I could have defined a function called "compare_vertical_name()", but it seems like a waste when it's so simple. Creating it inline makes sense to me here. I don't use anonymous functions for anything other than sorting callbacks. If I used preg_replace_callback() I might also use them as callbacks there. -
You'll need to set the headers yourself if you are fetching a file with curl and then displaying it yourself. Rather than copying the original headers I would just set the ones you need using header().
-
Sounds like you need to change the HTTP headers. Here's what microsoft says about it: http://support.microsoft.com/kb/260519
-
Usually you don't need to urldecode a $_GET value. Try viewing the source of the page and see what you are getting. You should see "&" in the source. If you don't, post what you see here as well as the code that generates it, and we can probably tell you where the encoding is going wrong. Also it would be good if you print out the original value from $_GET as well.
-
Is that the entire script? I don't see where $core and $db are defined. Also is the script named "index.php"? The first line there will exit if that is not the script's name. You might want to add this at the top: ini_set('display_errors', 1); And see if you get something useful.
-
<?php $id = $_GET["id"]; foreach ($rulepages as $rulepage) { if ($rulepage['id'] == $id) { // $rulepage is the one matching $_GET['id'] } } ?>
-
mysql_fetch_array() gets only one product, not all products. You could use mysql_data_seek() to move to the row you want, but it makes more sense to use the SQL offset and limit commands to get only the rows you need.
-
If you have a list of characters to allow, just list those characters, eg preg_match("/^[0-9a-zA-Z_-]+$/", $getUser); That allows 0-9, a-z, A-Z, underscore and dash only. Make sure the dash appears last or first, so it doesn't get mistaken for a range of characters.
-
If the array DOES only contain numbers and it's only 1 dimension, I would use implode(). There's an advantage in debugging when you have human readable data, as well as having it take less space. But be aware that implode() will convert all of false, null and "" to an empty string, so you won't be able to distinguish between those.
-
Try this: while ($fav_products = mysql_fetch_array($fav_products_rs)) { var_dump($fav_products); } If it shows all your rows, then replace the var_dump() with the code you want to have in there. BTW your code as it is will replace $_SESSION['fav_products'] each time through the loop. Probably you want something like this: $_SESSION['fav_products'] = ''; while ($fav_products = mysql_fetch_array($fav_products_rs)) { $_SESSION['fav_products'] .= "<li>" . $fav_products['product_order'] . ": " . $fav_products['product_name'] . "</li>"; } The ".=" instead of the "=" means "Add on to the end". Also known as "append" or "concatenate"
-
If you find that it's not a bug at all and you just want to get rid of that message, you can do this: error_reporting(E_ALL ^ E_NOTICE); Make sure you are 100% sure it's not a bug first though, by doing what MatthewJ said. Otherwise you'll just be hiding the problem instead of fixing it. That message is a "notice" rather than an "error", meaning php is telling you about it, but the script can still continue to run anyway.
-
The error is that you missed the ";" on the end of the line before the "while". So it thinks you're trying to put the "while" as part of the same command where you set $result.
-
Fetching google.com is probably enough. A codeen proxy will return the codeen intro page. If you get google.com, then you might try doing a post. If the proxy lets you get and post, that's probably a good definition of a "good" proxy. If you're processing large numbers you might want to record the response time of each. and sort by that. Hypothetically speaking of course.. I am in no way advocating the unauthorized usage of open proxies
-
I don't understand this browser detection code.
btherl replied to silverglade's topic in PHP Coding Help
They used strtoupper() so they can match the string in any case. You can use strtolower() the same way. Eg strtoupper('Lynx') == 'LYNX'; strtoupper('LyNX') == 'LYNX'; strtoupper('konqueror') == 'KONQUEROR'; So regardless of whether the string is upper or lowercase at the start, the strtoupper() makes sure it matches. -
Hmm, on re-reading the original post, this might be a job for left joins .. eg SELECT A,B,C,D,E,F FROM tab1 LEFT JOIN tab2 ON (tab2.A = tab1.A) LEFT JOIN tab3 ON (tab3.B = tab2.B) ... WHERE A = subject1 That implements "If there's a result from tab1, then fetch matching data from tab2. If there's a result from tab2, fetch matching data from tab3"
-
One simple parsing structure is a loop like this: $results = array(); $current_result = null; foreach ($lineArr as $line) { if ($line == "}\n") { $results[] = $current_result; $current_result = null; } if (strpos($line, '"STEAM') === 0) { # Start of a new block $current_result['block_header'] = $line; } if (some condition to recognize a data line, like "admin" "(Console)") { # Parse the data line, and add a row like $current_result['admin'] = '(Console)' } } var_dump($results); That's a sketch of how it will work. It uses the "}" line as a marker to recognize when a block has finished, so it can add the results to the output array.
-
I don't understand this browser detection code.
btherl replied to silverglade's topic in PHP Coding Help
The issue with strpos() returning 0 will only affect lynx, as the other browsers start their user agent with Mozilla, and have the real identifying info later. silverglade, in php any number like 1, 2, 3 is considered as "true" for an "if". So if strpos returns the position of a string, the "if" will succeed. If strpos doesn't find the string then it returns false, which of course makes the "if" fail. Is that the bit you were unsure of? -
Does this do what you want? $result = mysqli_query($connection,"SELECT * FROM table") or die(mysqli_error($connection)); $index = 2; while($row = mysqli_fetch_array($result)) { //get all rows you want from the table $var1 = $row['var1']; $var2 = $row['var2']; $var3 = $row['var3']; $var4 = $row['var4']; $objPHPExcel->setActiveSheetIndex(0) ->setCellValue('A' . $index, $var1) ->setCellValue('B' . $index, $var2) ->setCellValue('C' . $index, $var3) ->setCellValue('D' . $index, $var4); $index++; }
-
OK, the next step I would do is to change this mysql_query("UPDATE countries SET country_id = '{$_POST['update_value']}' WHERE country_id = '{$_POST['original_html']}'") or die(mysql_error()); to this $sql = "UPDATE countries SET country_id = '{$_POST['update_value']}' WHERE country_id = '{$_POST['original_html']}'"; print "About to execute '$sql'<br>"; mysql_query($sql) or die(mysql_error()); So we can see exactly what query is getting executed.