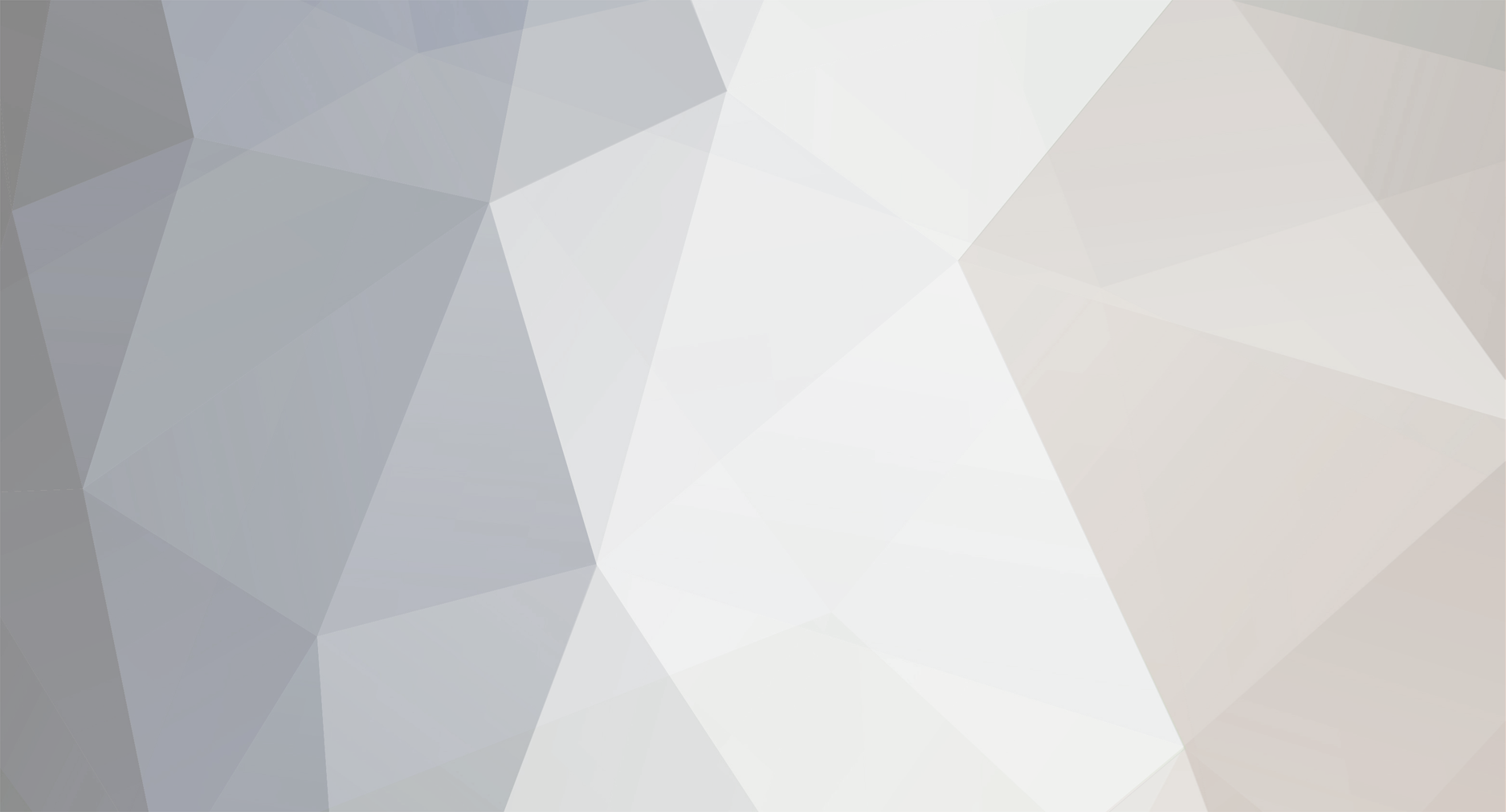
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
This might be the time to learn ajax It's actually very simple if you use jquery. It can be as simple as this: $.get('mark_user_as_timed_out.php'); You would want to have that executed after a 30 second delay, using javascript setTimeout(), such as at http://www.htmlite.com/JS018.php . What will happen is the user's browser will run that php script, but their page won't be updated (unless you want it to be).
-
Do you want the update to occur if the user is still viewing the page after 30 seconds? In that case I would use ajax with a timer. If you want it to act more like a timeout, where something occurs unless the user does something within a time limit, then you could store a timestamp in the database. When they take the required action you check the timestamp and see if it's more than 30 seconds old. In this case the action won't occur exactly after 30 seconds, but maybe that doesn't matter. It depends on the reason for the 30 seconds.
-
If you break the search term into words and search for each one, that should give you what you want. You can still do it all in one query, with lots of "OR"s.
-
You can fetch the page using curl (or a simpler method like file_get_contents()) and use strpos() to look for a string that only appears on the page when registration is open.
-
Here's another way to look at it: Let p(n,a) be the length n permutations of the array "a" p(3, array(1,2,3,4,5)) (0) = 1, p(2, array(2,3,4,5)) (1) = 1, 2, p(1, array(3,4,5)) (2) = (1, 2, 3) , (1, 2, 4) , (1, 2, 5) (3) Now (2) is finished, but there's still more possibilities from (1): = 1, 3, p(1, array(2, 4, 5)) = (1, 3, 2) , (1, 3, 4) , (1, 3, 5) = 1, 4, p(1, array(2, 3, 5)) = (1, 4, 2) , (1, 4, 3) , (1, 4, 5) = 1, 5, p(1, array(2, 3, 4)) = (1, 5, 2) , (1, 5, 3) , (1, 5, 4) Then that's all the permutations starting with 1. To implement all of this you would need to write the function p(n,a), which returns an array of permutations. When p(n,a) calls itself recursively it adds a number on to the front of each result from the recursive call.
-
You can approach it recursively. The length 3 permutations of (1,2,3,4,5) are: "1" combined with the length 2 permutations of (2,3,4,5) "2" combined with the length 2 permutations of (1,3,4,5) etc etc And of course the length 1 permutations of a single item are just that item itself. As for filtering out duplicates, you could compare the sorted permutations to see if they contain the same numbers.
-
Oops, that one is a method, not a function. If you replace "$this->_SOCK" with "$sock" and "$this->errstr" with "$errstr" then that should fix the errors. You would also need to make it return $sock instead of 1.
-
If you want the old list of urls to be replaced with the new list, you can delete all rows matching the user id and then insert all the new rows. That's the most conceptually simple way to do it, rather than thinking about what got changed.
-
How did you implement file caching? And how did you measure the times, and determine that the time difference was due to fopen() ?
-
Can you post your code, along with the expected results and actual results?
-
There's a curl multi interface for php: http://www.php.net/manual/en/function.curl-multi-add-handle.php It's a bit experimental and light on the documentation however. I had to refer back to the libcurl documentation to work out how the php interface was supposed to be used.
-
PHP will put arrays in $_POST as well, such as in this thread: http://www.phpfreaks.com/forums/index.php/topic,310848.0.html But if it's not an array then yes, i'ts a string. That's the same for $_GET and $_POST. PHP will urldecode() $_GET data automatically for you, so it arrives ready to use. Did you mean urldecode() or urlencode()?
-
Need help with multiple html form arrays going to one SQL table
btherl replied to meisenheimer's topic in PHP Coding Help
Here is one option: foreach($_POST['conference'] as $index => $conference) { // Update Conference // Access $_POST['confDate'][$index], $_POST['confDesc'][$index], etc etc. You can use either $conference or $_POST['conference'][$index] $degreeQuery = "UPDATE CONFERENCES SET Conference = '".$conference."', StaffPK1 = '".$getPK1."' WHERE ConferencePK1 ='".$confID."'"; $degreeQuery = mysql_query($degreeQuery); } -
array and Array are the same, it's case insensitive. So 1 and 3 are the same. function a(array $var) { } This says that a() MUST take an array, and it's an error if you give it something else. "Catchable fatal error: Argument 1 passed to a() must be an array, string given"
-
What you've said so far sounds like a good start. Another idea might be to store the user's current page in a single location, along with the timestamp. Each time they access a page you can check to see if it's a valid choice for the last page they accessed. If it's not, then you can set a flag on their account saying they need to wait. As for storing all those pages and linking them, I would be thinking of a structure like this: CREATE TABLE page ( page_name varchar not null, page_id integer not null primary key, -- This should be auto increment, i don't remember how to do that in mysql content varchar ); CREATE TABLE choice ( page_id integer not null, choice_id integer not null, choice_description varchar not null -- Optional - you can store text here like "Tell me about Japan" ); If (page_id, choice_id) is in the choice table, then choice_id is a valid choice from page_id. This lets you store the structure.
-
A good way to find missing "}" is to indent your code like this: if ($a == 1) { while ($row = mysql_fetch_row($res)) { print $row['val']; } } By indenting whenever you have a "{" and reducing indent when you have a "}", it is obvious when one is missing. If you want someone else to do the code for you then you should try the freelancing section of the forum. In this section we will help you to learn to do the code yourself.
-
If you don't use frames or iframes (they are different btw), what do you use?
-
Most browsers will send the original referrer when you do a 302 redirect, as you are doing there. Are you expecting the referer to be page A? Because that is not the normal behaviour. If you really do want page A to be the referrer, you can achieve that by doing the redirect in javascript, setting window.location.
-
What error do you get? The first step is to check for errors in EVERY call to mysql_query(). If any mysql_query() returns false your script needs to stop right there and report the problem.
-
Try this: preg_match('~^[0-9a-f]{32}$~', $string) I'm assuming you want 32 hexadecimal digits
-
Please post your code. I'll need to see everything related to getting the data from the form and storing it in the session, and everything from where the data is fetched from the session to where it is put into the HTML again. BTW "\n" is a linefeed, '\n' is a backslash followed by the letter n.
-
Are they in frames? You would do that with javascript, though I'm not sure on the details.
-
How are salt values typically implemented in a project?
btherl replied to DWilliams's topic in PHP Coding Help
I would generate it if it's not there, and store it somewhere. I'm being vague about where to store it because it really depends how your script will be used and how the salt will be used. Asking the user to make it seems like asking them to do something they don't really need to. Though you can allow them to manually override it if needed. -
Building multidimensional array from url params
btherl replied to dangermark's topic in PHP Coding Help
Here is how I would do it: <?php $searchArray = array(); if (!empty($_GET['ind'])) { array_push($searchArray, $_GET['ind']); } if (!empty($_GET['loc'])) { array_push($searchArray, $_GET['loc']); } if (!empty($_GET['wt'])) { array_push($searchArray, $_GET['wt']); } echo '<pre>'; print_r($searchArray); echo '</pre>'; ?> The reason for using "not empty" instead of "isset" is that an empty variable is set, and you don't want an empty variable included in the array. -
What happens if you use 24 hours instead of 1 day?