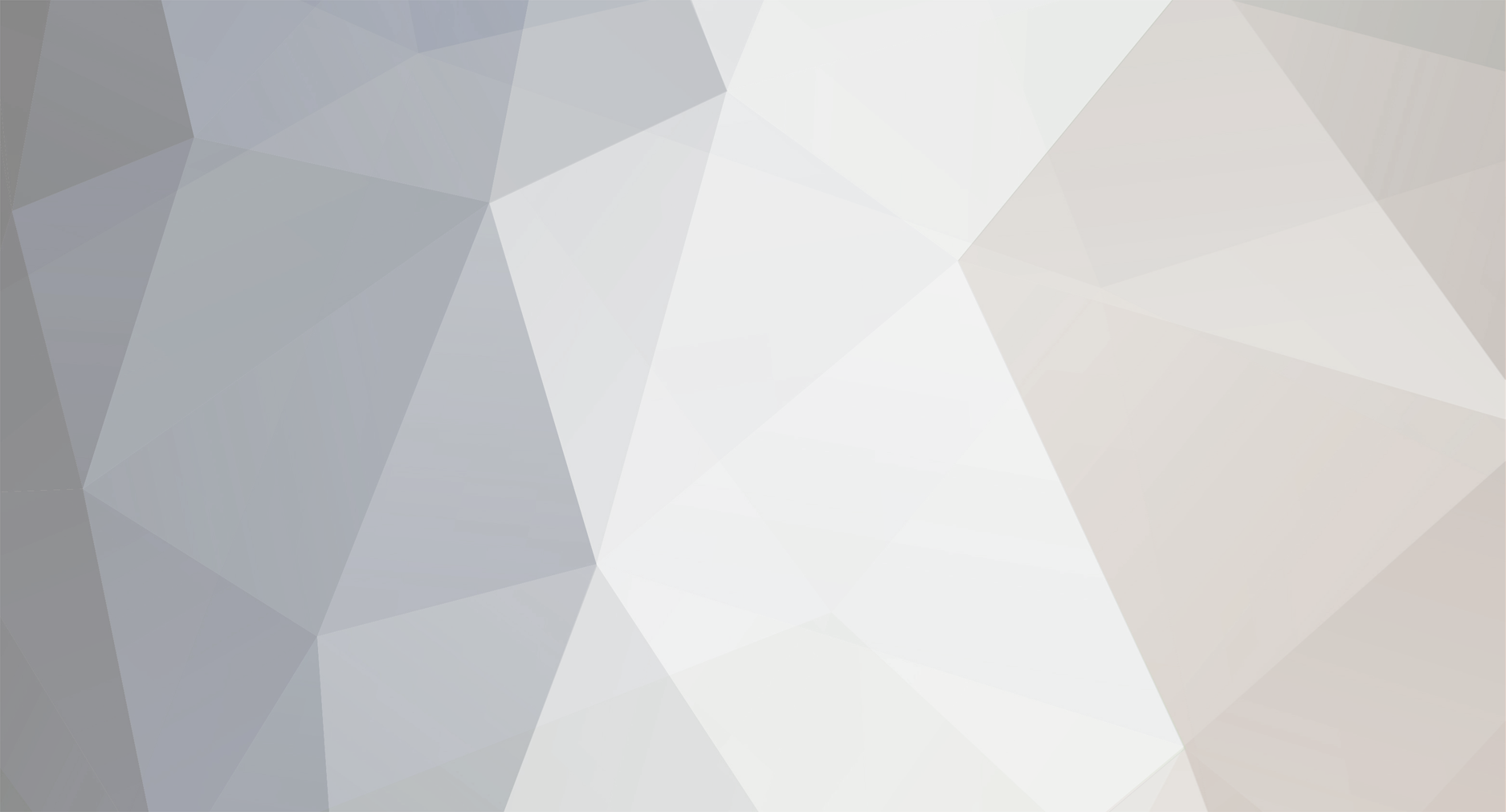
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
I just cant understand the concept of referencing
btherl replied to farban6's topic in PHP Coding Help
Another use for references is like this: $big_array = <some huge array of data>; add_foos($big_array); # add_foos(): Adds a foo to each array element of $big_array, without generating a second copy of the array function add_foos(&$big_array) { foreach ($big_array as $key => $val) { $val['foo'] = 'bar'; $big_array[$key] = $val; } # No return value - parameter $big_array is passed by reference and modified return; } If instead you passed $big_array by value and added the foo elements and returned the modified array, PHP will create a second copy of the entire array. If your array is huge this can double your memory usage. In this case it makes sense to use a single well documented reference. If you're not modifying the array then you don't need to do this, as php won't copy the array unless you modify it. Another example is php's built in sort() functions - these all use references so they can do the sorting without making a copy of the array. -
I just cant understand the concept of referencing
btherl replied to farban6's topic in PHP Coding Help
Ok I think I'm seeing now why he uses references. References are "transitive", in this sense: $a = &$b; $b = &$c; Now $a, $b and $c are all bound together in the same way. They reference each other. If you change any of them, you change all of them. bind_result() "binds" its arguments by making them references. And because the arguments passed to bind_result with call_user_func_array() are already bound to the $row[$field->name] variables as well, the result is a 3 way reference binding. $row[$field->name] is bound to $parameters[$i] which is bound to whatever bind_result() binds it to. I could be totally wrong here - still can't watch that video until I get home. But that seems like a situation where references are the only solution, and where they are appropriate, in that data isn't going to leak in and out of functions (as long as you are careful). -
Is there any way too pass variables through include files.
btherl replied to Orionsbelter's topic in PHP Coding Help
Oops, and I made a typo: $var1 = 'one'; include("file.php"); I had forgotten the quotes around one. -
From the PHP documentation at http://www.php.net/manual/en/reserved.variables.server.php What that means is it's due to the web server configuration by your host. And it also means that PHP makes no guarantees about the contents of PHP_SELF, so it's up to you to deal with it. As for the specific problem, try printing out the value of $_SERVER['PHP_SELF'] before applying dirname(). It's likely that it already contains the value you want, and that dirname() is actually removing the index.php from the end. It would have worked before because it had a "/" character at the end. That's my guess. As for how many places you need to change this in, consider it a lesson in why you shouldn't copy and paste code - if you had this variable defined in one central location and then referenced in other scripts, you would only need to change it once.
-
I just cant understand the concept of referencing
btherl replied to farban6's topic in PHP Coding Help
IMHO, references should be avoided unless there's no other choice, or you have a very good reason to use them. They are as bad as global variables, in that they allow you to modify variables elsewhere in the program without it being obvious that the modification is happening. Unfortunately I can't see that video as I have no speakers here, so I don't know why he is suggesting references. Based on that code I don't see why you would need them. -
If what requinix suggested doesn't fix it, I would check that you are using binary (aka image) mode when uploading the excel files, and not text mode. That's a common problem with FTP.
-
Is there any way too pass variables through include files.
btherl replied to Orionsbelter's topic in PHP Coding Help
You can pass variable like this: $var1 = one; include("file.php"); $var1 will be available inside file.php. If you are using include() with a url instead of a filename, don't do that - you should be using file_get_contents(). In that situation you do need to pass variable a different way, so let me know if you are using urls. -
That error is given by your webserver, and occurs before php execution, in that particular request anyway. It may be the result of this redirect though: header("Location: ?p=map&view=local"); The specification for Location headers says you must provide a full url, although most browsers will try to make a relative url like this work. You could try including the script name in the url, to make sure it goes to your script and not main.php
-
date is fine for storing dates without the time of day. timestamp stores date and time, something like datetime in mysql I believe. Timestamp can be with timezone or without timezone.
-
Fair enough.. if your boss asks you to re-invent the wheel then you have to re-invent the wheel. And the best way is to look at an existing wheel and more or less copy it. Googling "PHP MVC tutorial" turns up quite a number of tutorials, so that's another possible angle to start with. I'm a bit reluctant to give advice at this stage - I would find it much easier if you started writing the framework and posted back here when you got stuck. One tip - if you're going to be integrating with 3rd party spaghetti code, I would seriously consider using namespaces to insulate your code from the spaghetti. Unless the spaghetti happens to have clearly defined boundaries in how it deals with the global namespace.
-
Why were you asked to re-write it without a framework? I would just go ahead and copy the framework's structure. Smarty is just a templating engine, not a framework or a structure, although it can form part of one. Honestly the best approach is to find a framework you like and use that, but if you were specifically asked not to use an existing framework, then I would instead find a framework you like and copy how it works. The structure we use is more or less MVC, with a small controller sending requests off to various files that implement a particular page, and they send requests off to classes that do most of the work. Those pages then return an array to smarty which renders the template.
-
"text mining" data from LUA files into MySQL database
btherl replied to knar's topic in PHP Coding Help
A reliable way to parse something like that would be a loop with a few flags to remember where it is. Something like this: $in_item = false; $in_resources = false; while ($line = fgets($input_fp)) { $line = trim($line); if ($line == '') continue; if ($in_item) { if (strpos($line, 'pretty_name') === 0) { $pretty_name = str_replace("pretty_name ", "", $line); # Note - doesn't remove quotes } if ( ... ) } if ($in_resources) { if ($strpos($line, "object ") === 0) { $resources[] = ... } } if (we're at the end of the resources list) { # Store the resources } if (we're at the end of an item) { # Store item } } I've been a bit general there as I'm not sure of the exact file structure. But the basic idea is to remember what you're doing, and then handle each line as it comes according to what you are currently doing. The other key part is to trigger storing of each item/resource/whatever at the appropriate times. Googling for "php lua parser" also showed up some results, but I don't know what they are capable of parsing and if they apply to your situation. -
I don't see any error on that page either. Which browser are you using, and do you have any add-ons installed?
-
I don't see any error in the link you posted
-
It depends on what exactly you want, but you could try using base64_encode() on the result of your encryption. Also I might rethink your calculation for the value to add - for many values of $i it has little or no effect, and it is very predictable.
-
Can you post the code you want to modify?
-
<?php $conn = mysql_connect("localhost","XXX","XXX"); $db = mysql_select_db("XXX"); class Map { function ImageWork($ImageNumber) { echo("<a style='cursor:pointer' id='opener2'><img src=\"Pictures/$ImageNumber.jpg\"/>");} function ImageFail() { echo("<a style='cursor:pointer' id='opener2'><img src='Pictures/base/3.jpg'/>");} } ?> <table width="75%" border="0"> <tr> <td><?php @$worked = mysql_query("SELECT * FROM `map` WHERE `x`=('".$_GET['x']."' + 0) AND `y`=('".$_GET['y']."' + 0)"); @$result = mysql_fetch_object($worked); if($result->x != ""){Map::ImageFail();} else {Map::ImageWork($result->Number);}?></td> <td><?php @$worked2 = mysql_query("SELECT * FROM `map` WHERE `x`=('".$_GET['x']."' + 1) AND `y`=('".$_GET['y']."' + 0)"); @$result2 = mysql_fetch_object($worked2); if($result2->x != ""){Map::ImageFail();} else {Map::ImageWork($result2->Number);}?></td> </tr> </table> So what's happening is that Map::ImageWork() now takes a number as an argument, and it substitutes that number into the HTML. You can then choose which number to give it when you call it.
-
You're welcome! Either of those file delivery methods can work, each requires a slightly different approach. If you point them to the created file then you need Apache to set the mime type. The big limitation here is access control is trickier if you do your authentication in PHP. If you serve the file from PHP then you need to set the mime type and headers from PHP, but you can also do access control in PHP, which can be simpler. I can provide you some code for this if you're interested, as we typically use this method.
-
I'm pretty sure you'll need to call latex from php, using exec or similar. The exact implementation depends a lot on the situation you need this conversion for. Is it running on a server or a desktop, and is the system running windows or linux? I don't think FPDF is going to help, as it does not appear to have any integration with latex.
-
You should be passing $result as an argument to ImageWork() and ImageFail(). Or you can pass $result->Number if you prefer.
-
What error is reported?
-
Instead of the foreach loop you can use this: if (in_array($row['id'], $explode_amen)) { # Yes, it's checked } else { # No it's not checked } That should fix the problem. The problem is that your checkbox display code is inside the foreach loop.
-
Please post your current query code and the foreach loop. What goes wrong when there is more than one division and one school in the zone?
-
Ok. Using explicit join syntax, you could write that as: SELECT * FROM teachers t JOIN teacher_appointements ta ON (ta.nic = t.nic) JOIN school_locations sl ON (sl.census_id = ta.census_id) JOIN divisions d ON (d.division_id = sl.division_id) WHERE d.division_name = '{$_POST['division_name']}' I have given each table a short alias. Each "JOIN" is followed by an "ON", which tells Postgres how that table relates to the other tables. Can you try this query and see if it does what you want?