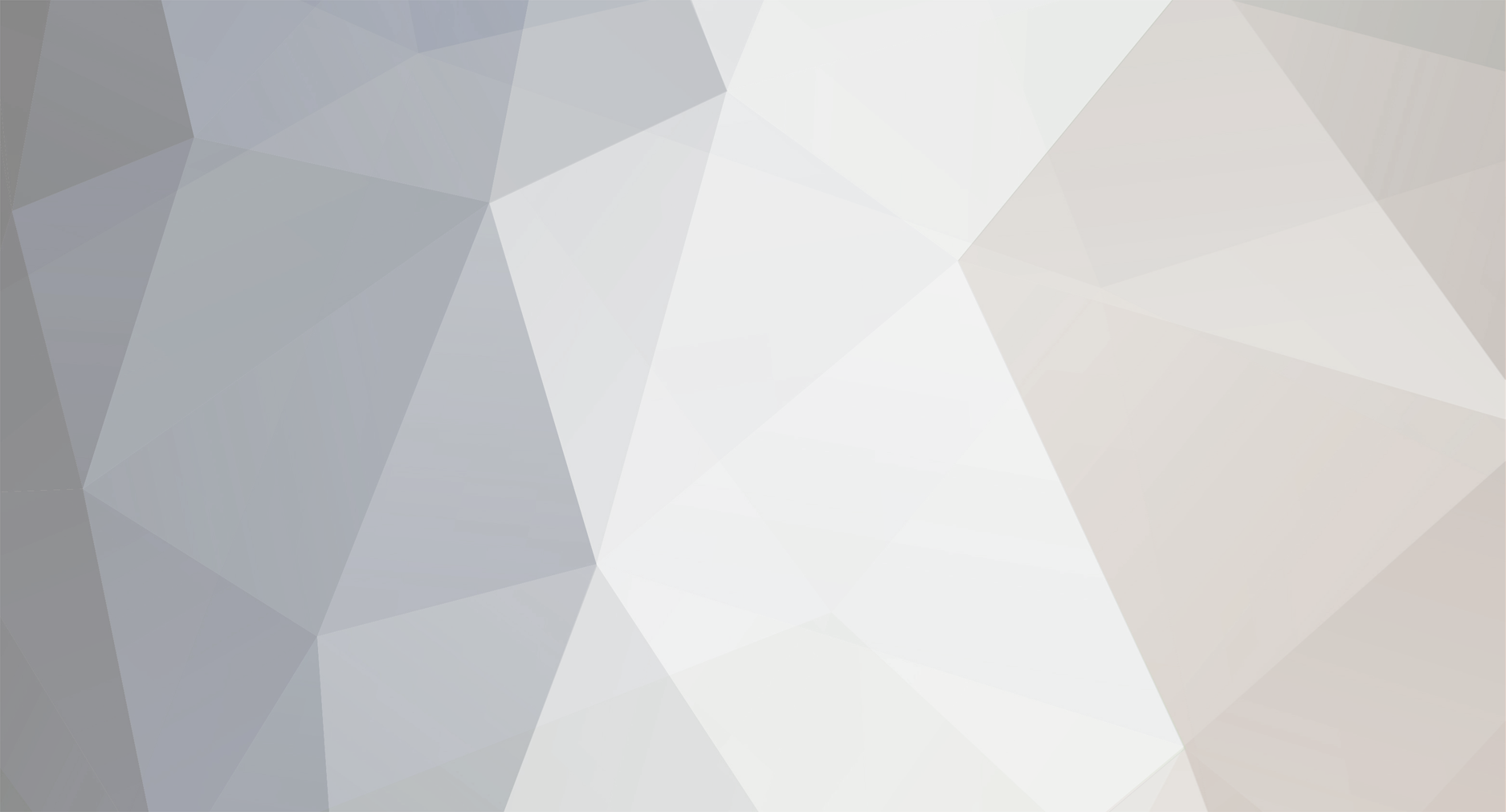
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
PHP Fatal error: Allowed memory size of 67108864 bytes exhausted
btherl replied to ballhogjoni's topic in PHP Coding Help
It says it's running out of memory. How many items do you have in $meta_list? And how many meta lists would you process in one run of the script? The memory usage may also be elsewhere - it could fail here because memory was filled up elsewhere in the script. It looks like your memory limit is 64 megabytes. -
Need help tracking pixel location with GD imagerotate
btherl replied to Andy-H's topic in PHP Coding Help
Which parts of that do you understand? Eg do you know how cos and sin work? Also are you rotating around (0,0) or are you rotating around an arbitrary point such as (50,50)? Such as when an image is indexed from 0-100 on each axis and you want to rotate around the center of the image? -
Thanks. I think what you want is this: $totalweight = $info['count'] * $info['weight']; Previously you were getting "0" because you were multiplying by $info['itemtype'], which is a string like "bananas". PHP treats non-numeric strings as "0" in calculations. Also to get a nicer output from print_r() you can do this: print "<pre>"; print_r($info) ; print "</pre>"; The pre tags tell the browser to use text formatting instead of html formatting.
-
Can you add this code please: print_r($info); and paste the output.
-
Call to a member function fetch_assoc() on a non-object
btherl replied to Mod-Jay's topic in PHP Coding Help
If it's not an object, what is it? Can you use var_dump() to find out? Then trace it back through the code until you get back to where it's created (which isn't far), and see if it's a non-object the whole way. -
To explain why it's not working, this is what php thinks: $info['count' * 'itemtype']; # Hmm, multiplying two strings? I'd better convert them to numbers first = $info[0 * 0]; # Ok, that makes more sense. Now simplify = $info[0]; # Ok now we can look up item 0 in the array (which is indexed by name AND number) = the count column, which happens to be index 0
-
Well the notices are useful.. if you are serious about writing solid code then you should have them on. Or use a custom error handler that switches off only the ones you know you don't need. But if you are taking someone else's code and plan to use it as-is, I would usually just switch them off. Then again, if I wanted to take that code and check it thoroughly for any security issues and THEN use it, I would switch them on. So it all depends on where the code is from and what you want to use it for, and whether you want to audit and/or modify it. Just today I found a months old bug in some mature code, and I found it because notices were recently switched on on that particular development machine.
-
Try like this: $pattern = "/^$cc([$ac]{1})([0-9]{{$ph}})$/";
-
Those are "notices", rather then errors. Everyone else probably has them disabled. And unless you want to go through and modify the entire script, I suggest you switch them off too. The relevant setting is this: http://php.net/manual/en/function.error-reporting.php You probably want this setting: // Report all errors except E_NOTICE // This is the default value set in php.ini error_reporting(E_ALL ^ E_NOTICE);
-
One more thing - you should start with $i = 1 and have the termination condition as ($i <= 14). Otherwise you will run through 0-13 instead of 1-14. Alternatively you can put the $i++ before the $message line, and leave the start and termination conditions unchanged.
-
You need double quotes around those first$i : $message .= "<tr><td>".$_POST["first$i"]." ".$_POST["last$i"].", ".$_POST["instrument$i"].", ".$_POST["grade$i"]."</td></tr>"; Double quotes allow variables like $i to be substituted, and also allow interpreting of escape sequences like "\n" for newline. Single quotes allow no processing inside, so if you write '$i' then you get exactly '$i', not the contents of $i. Regarding your mysql query, what happens when you try it? Does the query fail (mysql_query() returns false), and if so, what does mysql_error() show?
-
Working out separate numbers from combination and total
btherl replied to Kriptonic's topic in PHP Coding Help
If you store the previous attempts in a large enough hash table then it's almost always constant time to check if a possibility has been checked, with collisions taking slightly longer. Or you can use a large array and an addressing scheme that puts each possibility in one array slot. I've done a lot of research in reducing recursive boolean equations to non-recursive boolean equations for program analysis, and it turns out random approaches do quite well. Once you limit yourself to a particular input set that you know the characteristics of and can develop a systematic approach that works well for those kinds of input, then you can do better than random. But here I still don't know of any patterns in Kriptonic's input, so he might as well use a random approach. I would agree that the odds are in favour of a systematic approach being faster, even without knowledge of patterns in the input. -
Working out separate numbers from combination and total
btherl replied to Kriptonic's topic in PHP Coding Help
In this case I think a systematic approach is better, and that's because it's a very small search space, and the worst case of having to check every single possibility before finding the solution is not particularly bad. So I'm talking more about larger search spaces, where a random approach can have a generally a better average case, depending on the distribution of inputs. In that sense it's theoretical. The non-theoretical part is that I'm sure these inputs are skewed, and so a systematic approach has a chance of usually testing many wrong solutions before it finds the right one. But it becomes theoretical again because the potential speed up is too small to be worth the effort. The reason I didn't immediately discount Kriptonic's random approach is that it really isn't that bad with such a small search space. I didn't think it was worth trying to switch him to a systematic method when the efficiency gain wasn't going to be that much. I agree with what you're saying about $lengthMax. I think Kriptonic would just derive $lengthMax from the length of the total anyway, so I thought it would save a step if splitNumbers() did that itself. -
Working out separate numbers from combination and total
btherl replied to Kriptonic's topic in PHP Coding Help
"probably" is the word here If the inputs are skewed but the bias is unknown, a random approach which tracks which possiblities have been tried before is usually going to give you average performance, regardless of what the bias is. A systematic approach will either give you consistently better or consistently worse than the random approach, depending on what order the possibilities are tried in and how the data is skewed. So a systematic method which matches the data is better then random, but a systematic method which doesn't suit the data is worse than random. If it's truly random data then it doesn't matter which approach is used. In splitNumbers(), $lengthMax = strlen($total) is an upper bound on the number of digits in each number being searched for. -
You can try http://php.net/manual/en/function.mb-strlen.php Make sure you specify the encoding.
-
Have you used arrays before?
-
Can you try a simpler version of your login.php, which simply includes header.php, sets $_SESSION='message' = 'test' and then exits immediately. That will either conrfirm that sessions are working, or determine that they are not working.
-
What code do you have so far?
-
Working out separate numbers from combination and total
btherl replied to Kriptonic's topic in PHP Coding Help
So your approach is to split randomly until you find a match? That sounds reasonable. In your example you can make some extra assumptions: 1. The sum has 5 digits, therefore each individual number has between 1 and 5 digits (assuming these numbers are all positive and don't have leading 0s) 2. The sum is < 20,000 therefore no more than 1 individual number has 5 digits, the rest have 4 or fewer digits. Similar rules apply for other sums where the first digit is 1, 2 or 3. This may be too complex to be worth implementing. 3. If numbers don't have leading 0s then any split beginning with a 0 digit must be of length 1, so that limits your possibilities a bit. Rule #1 seems like the most useful, rules 2 and 3 might help a little. -
Working out separate numbers from combination and total
btherl replied to Kriptonic's topic in PHP Coding Help
Any sequence of numbers consisting of identical digits (except where there is a single digit in each number) is impossible to reverse unambiguously, so there will be some cases where you can't find the original numbers. For example: 1, 1, 1, 11 -> Total 14, concatenates to 11111, the 11 could be in any position 1, 11, 11, 1 -> Total 24, concatenates to 111111, the 11s could be in any position. Breaking the concatenated digits into every possible combination might work. If you do check every combination then you can also detect when there is an ambiguous case. -
I think you need to refer to the documentation for the geonames API. If you link to the docs I may be able to help you.
-
There are 4 things I would check there: 1. How is the form submitted, and does it really send fan=1? 2. Is the "if" actually executed, or is it skipped because of other code outside? 3. Is company_add_fan() being executed but not having the expected result? 4. Is your web server dropping the query string when it redirects that url to your script?
-
Can you try executing this (in place of the original corresponding code): $query_rsFigures = "SELECT Figure_Name, Figure_Thumbnail, series.Series_Id FROM figures INNER JOIN series ON figures.Series_Id = series.Series_Id WHERE series.Series_Id = 13 ORDER BY Figure_Name ASC"; $rsFigures = mysql_query($query_rsFigures, $FIGURES) or die(mysql_error()); print "<pre>"; print "Query: $query_rsFigures\n"; while ($row_rsFigures = mysql_fetch_assoc($rsFigures)) { var_dump($row_rsFigures); } exit; The idea is you should work with this code, which tests only the query and nothing else, until the query is working 100%. Then continue with the rest of the code. It also displays the query so you know exactly what you are executing (this is more relevant when the query has variables in it, but it's a good idea anyway). For your second code attempt you should definitely be printing out the query to see what's in it.
-
$_SESSION initializing with values before assignment
btherl replied to doubledee's topic in PHP Coding Help
Yes, destroying the session is a good idea when someone logs out. And probably a bad idea in any other situation. The reason is it's difficult to keep track of all the data you might have put in $_SESSION while someone was logged on. And you want to make sure no-one else who logs in later can access that same data. So it's safest just to get rid of it all. Was the problem that you hadn't called session_start() on the members only page, and then nothing set on that page was accessible from other pages? -
$_SESSION initializing with values before assignment
btherl replied to doubledee's topic in PHP Coding Help
The whole purpose of $_SESSION is that the values are populated when you call session_start(). $_SESSION is for you to store data that will be available every time the script runs. If you want to clear that data, you can use the sample code here: http://php.net/manual/en/function.session-destroy.php Usually you would run code like that when someone logs out.