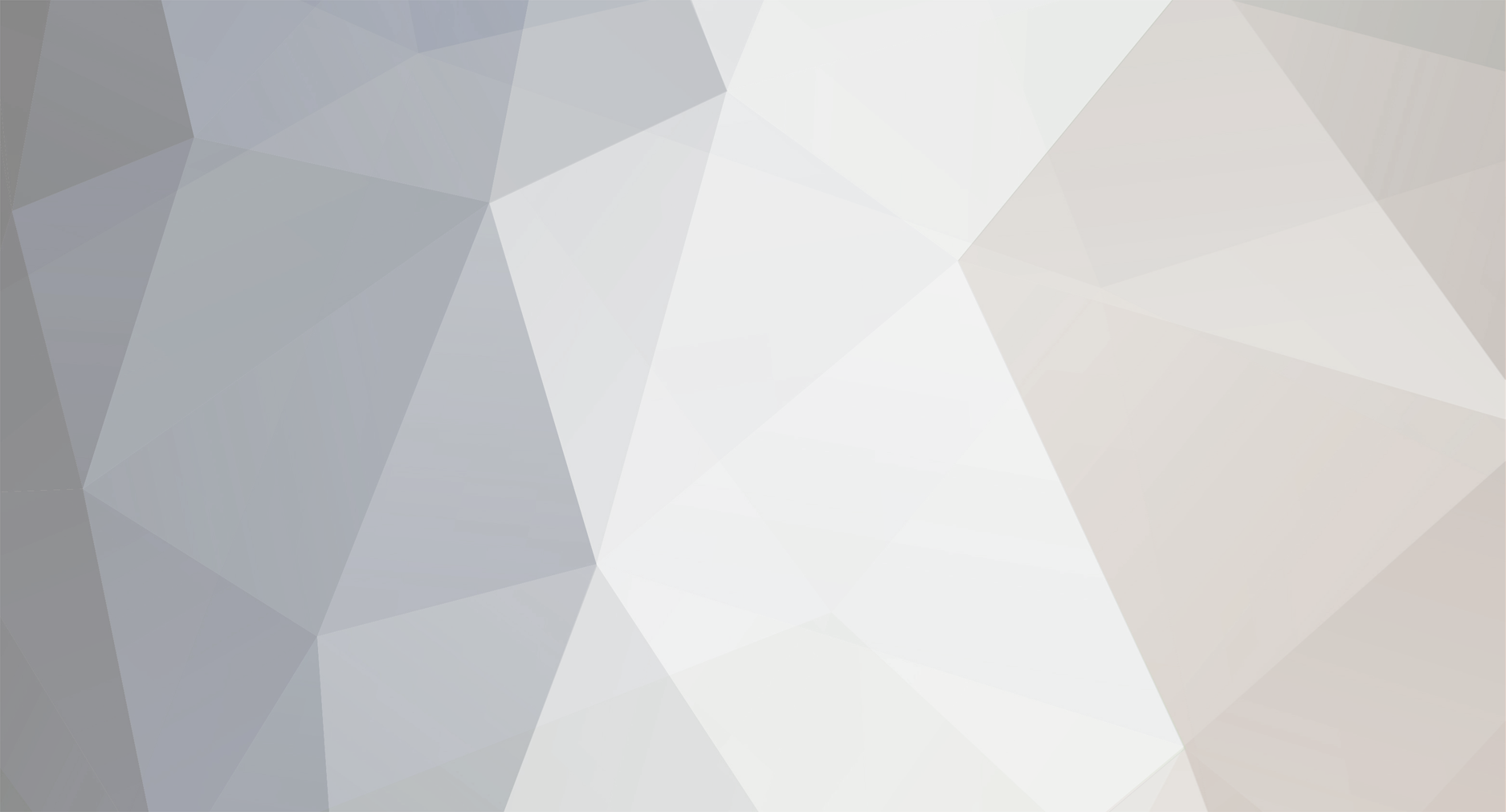
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
Call to a member function escapeValue() on a non-object
btherl replied to erikgoranzon's topic in PHP Coding Help
I strongly suspect your "create view" didn't do anything. Firstly you can't create a view as an "INSERT", secondly you didn't give it a name, so I would expect it to fail without changing your database at all. Was there anything else you did around the same time? Also the general meaning of your error is "I am not connected to the database". Because there is no db connection object, you get the error "Call to a member function escapeValue() on a non-object". That's the issue you need to debug. -
The kind of google search term you need is "php dom html parser tutorial". One result is this, showing xpath queries: http://stackoverflow.com/questions/2571232/parse-html-with-phps-html-domdocument Here's another style: http://www.nicolaskuttler.com/post/php-innerhtml/
-
The first step is to add some debugging statements to tell you what part is not working. There are many places it could fail and you need to narrow it down.
-
This should work: <?php print"<script> $(document).ready(function() { $.sticky('The page has loaded!'); }); </script>"; ?> Or you can just put the code outside php tags altogether. Also be careful of using "$" inside a print statemente - if you have $ followed by a letter it will be interpreted as a variable name and interpolated.
-
Some people use preg_match() for this kind of task. The exact expression to use depends on which text you need and what format you need it in. You can also use a parser like http://simplehtmldom.sourceforge.net/ which will give you a data structure.
-
Some people use preg_match() to extract data from HTML with regular expressions. You can also use a parser like this one: http://simplehtmldom.sourceforge.net/
-
Ok that makes it clear. Then you should use this to get the current number: $currentSRNum = (int)(substr($row['sr_num'],6,4); And to know when to increment it is very simple - you should compare the year, month and day together: $currentSRYMD = substr($row['sr_num'], 0, 6); $currentYMD = date("ymd"); if ($currentYMD > $currentSRYMD) { $currentSRNum = 1; } else { $currentSRNum += 1; } This is much simpler than comparing year, month and day individually.
-
$currentSRNum = (int)(substr($row['sr_num'],0,3)); $currentSRYear = (int)(substr($row['sr_num'],2,2)); $currentSRMonth = (int)(substr($row['sr_num'],0,2)); I would try printing out these values and see if they are really what you expect them to be. It doesn't look right to me. I think you want: $currentSRYear = (int)substr($row['sr_num'],0,2); $currentSRMonth = (int)substr($row['sr_num'],2,2); $currentSRDay = (int)substr($row['sr_num'],4,2); $currentSRNum = (int)(substr($row['sr_num'],6,3); Unless I misunderstand the structure of the SR number. I'm also suspicious of the rules used to increment the number - can you describe what the rules are supposed to be? Do they start from 1 each day?
-
Help with returning multidimensional array
btherl replied to emilysnothere's topic in PHP Coding Help
Maybe you want this: $out[$alt_css_template_file] = WSME_THEME_CSS.'/'.$alt_css_template_file; //create the array(filename => file path) for each file in the directory -
Will that trigger work being run "before insert"?
-
I can't help you with that unfortunately. I'm not familiar with the facebook API.
-
Please post your PHP code.
-
Thanks salathe, that looks like a better way to do it randall, the first thing to do is check for errors every time you do something which could fail. mysql_query() can fail, so you should write: $SQ_query = mysql_query($get) or die("Query failed: $get\n" . mysql_error()); Secondly, I don't know what you are trying to do with $str = ($fetch), but I would use var_dump() to display what those values are. First var_dump($fetch), then var_dump($str) after you assign it, and check if it did what you expected it to.
-
That was it in my post above - the file words.txt will look like this: the a and And the code to read the words into an array is: $words = explode("\n", file_get_contents("words.txt")); This code has one problem - the file words.txt is often stored like this: the\n a\n and\n That is, there is a newline after every line. When you explode to get the words, the array will look like this: $words = array( "the", "a", "and", "" ); The extra entry at the end is because explode() sees three "\n", and assumes they are seperating 4 words. So you need to get rid of that extra entry, for example like this: for ($words as $k => $v) { if ($v == '') unset($words[$k]); } If that's all very confusing, try putting it in your code and running var_dump($words) between each part, so you can see what's going on.
-
include() would work. Normally I would do something like this though (with error checking) $words = explode("\n", file_get_contents("words.txt")); Then the word list is just a plain text file. You can make it fancier by trimming spaces and comments out of the file as you read it, making the format more flexible and allowing documentation.
-
It's a messy problem in general. If I was doing it I would start by deciding the number of columns, then developing a way to measure the vertical size of each category. That would be something like "title + 1 (the gap) + number of subcats". Then it's a matter of adding categories to each column (which could be represented by adding them into an array) until they reach approximately 1/5 of the total size of all categories. Then start adding to the next column, and so on.
-
require_once() is almost universally better. The only situation you would consider using something else is if you wanted to include a file multiple times (which I have never had to in 7 years of professional PHP programming), or if you want your script to continue even if the file couldn't be included (again, I have never wanted this). In those cases you would use require() or include_once() respectively. include() is for if you want to include something multiple times AND you want to continue your script even if it fails. For connection.php, require_once() is the right option.
-
I've never had to. Using var_dump() on the result should show you the structure returned. In fact I've never used SoapVar at all when using PHP's SoapClient, it can understand arrays. It may be worthwhile if you need to specify the types though when sending data to the server.
-
You might want to try \b instead of \s around the words in preg_replace().
-
Are you talking about mysql_select_db()? That can go into your connection.php. So can the check for the connection. Your common code in each file can look like this only: require_once('connection.php'); As long as connection.php dies if anything goes wrong, there is no need to check the return value from require_once().
-
The first time you posted line 80 it said intval($value * $ratio), but the second time you posted it it said intval($values * $ratio). It needs to be $value, not $values. Whenever you have the error about unsupported operand types, use var_dump() to print out the values you are using in that line. You should find that the values aren't what you expect them to be.
-
That line looks sensible to me. Can you insert this line just before it please: print "Margins: " ; var_dump($margins); print "graph_height: " ; var_dump($graph_height); print "value: " ; var_dump($value) ; print "ratio: " ; var_dump($ratio); With any luck that will show you that one of those variables is not what it should be.
-
Ok that's an improvement on the previous version. Are you familiar with arrays? What you need to do is add one item into the $values array each time around the while loop. For example, it might look like this: while($row = mysql_fetch_array( $result )){ $months = date("M", strtotime($row['RptDate'])); $ip = $row['referrer']; $values[] = array("$months" => $ip); } #-- end while loop At the end of this you can insert the following line to debug: print "<pre>"; var_dump($values); exit; If it all looks correct, remove the line and continue coding.
-
Ok, the first thing to do is use consistent indentation. The "}" which ends the while loop needs to be directly under the "w" from the start of the word "while". That will make it clear where the end of the loop is. If the end of the loop is a long way away, add a comment after the "}" to tell you which loop it's from. I can't actually tell from your code where the while loop ends, but I suspect it includes all your graph drawing code.
-
It looks like you are running all the graph drawing code for every row returned from the database. Is that the way it is supposed to be working? Or do you want to read all the data from the database first and then draw the graph in one go?