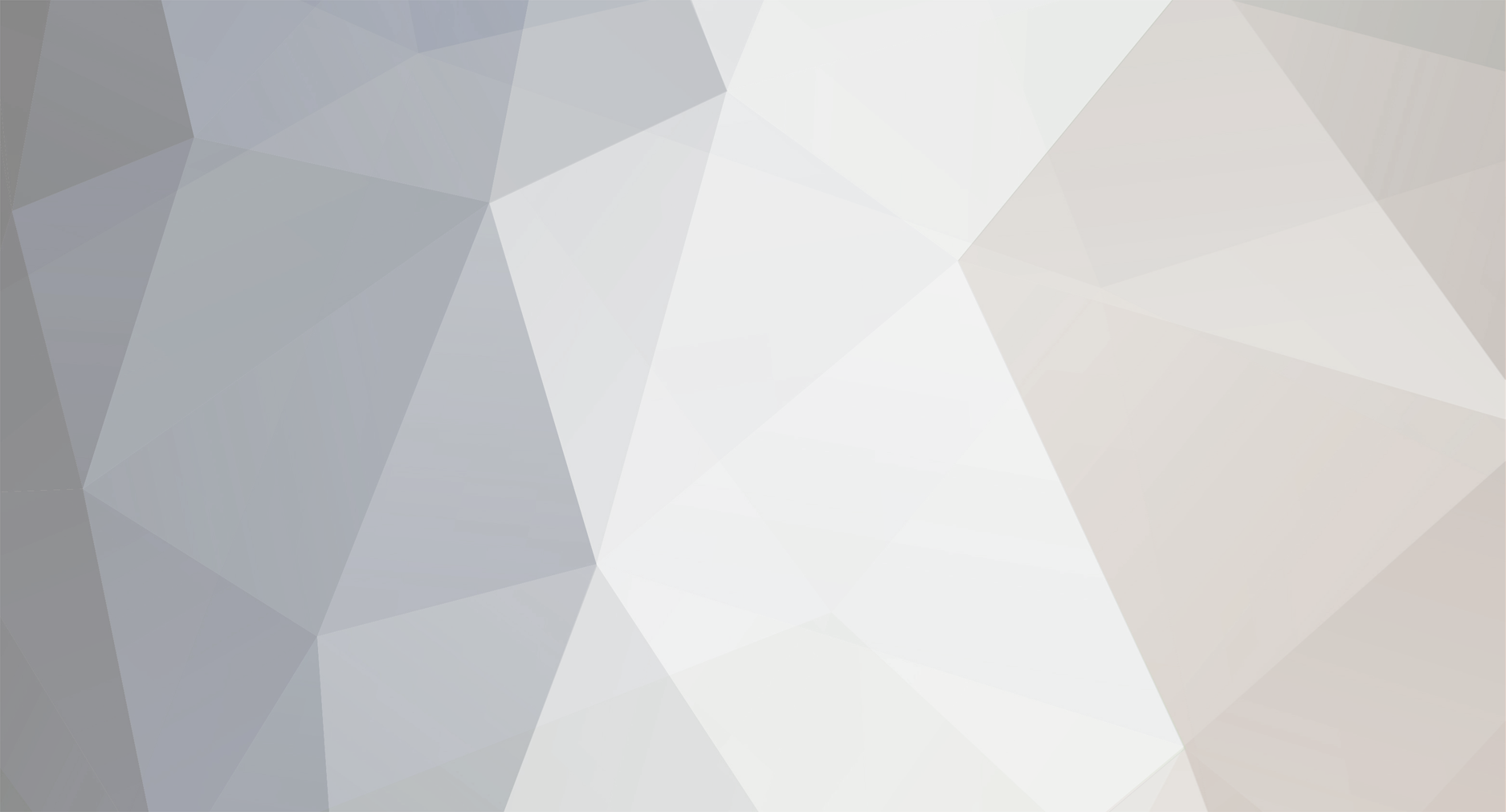
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
You can select them all and then order them so that the result you want appears first .. the exact sql depends on your database structure. But it won't be pretty. I suggest you do it in php unless you have a good reason not to.
-
I also want to check if the query is being executed. That's the first step to debugging why it's not updating. If it's being executed and it's still not updating, then it's time to look at the query itself. Yes the whole script will help And also a brief description of the circumstances in which the update should be triggered.
-
The next things I would try are: 1. Can I create a file with that name manually? 2. Can I create a file called "1281999572_download.zip" without generating it automatically with time(), instead using a static string? 3. If it still doesn't work, try filenames with different lengths and with different character sets in the filenames.
-
Make sure you use htmlentities() only once. If you use it more than once on the same data, it will get corrupted. I'm not sure from the post if you are clear on this, but htmlentities() is to encode data for inserting into the html output from your script. It shouldn't be applied to input coming from a form, in usual circumstances.
-
Parse error: syntax error, unexpected T_STRING
btherl replied to Mavrik347's topic in PHP Coding Help
clientdbdelete may be a teamspeak 3 command, but it's not a php method name. You'll need to find which php method corresponds to it. For example, it might be something like $virt->ClientDbDelete($cid). Alternatively, if the teamspeak3 object lets you send raw commands you could try it like that too. -
And, it is never necessary to declare "global $_POST;" - $_POST is automatically global. To fix your error message, the first thing to do is to print out $sql whenever there is an error. For example: mysql_query($sql, $conn) or die("Error in $sql\n" . mysql_error()); Then you can see exactly what query is getting sent to mysql and having the error. And use the code wildteen88 posted under "It is generally bad practice to ...", that has fixed the syntax problems, assuming sqlvalue() does what we're expecting it to.
-
Elegant way to initiate database & other classes in PHP page
btherl replied to Azsen's topic in PHP Coding Help
Yep looks like you're on the right track. As for templating, that's entirely up to you. If you do decide to learn about smarty you won't regret it. Having a clean separation of the HTML and the back end allows you to do a lot more. If you go further and make sure your core functionality is all in the classes and that those classes never access HTTP specific stuff like $_GET directly, then you your code is ready to plug into an XML or SOAP api. Which you may never want to do, but anyway.. I always end up having to do that, so that's why I try hard to keep the presentation code (Smarty and HTML) separate from the back end code, and the interface code (HTTP, $_GET, $_REQUEST, $_SESSION) separate from the back end also. -
I wouldn't worry about efficiency here. More important is how clear the code is. If you want to calculate the probability of any of a number of random events happening, the formula is: Let P1 = 0.1, P2 = 0.01, P3 = 0.001 (probabilities of winning x2, x10, x100). The chance of NOT getting ALL of those is (1-P1) * (1-P2) * (1-P3). The inverse of that is the chance of getting AT LEAST ONE of those, 1 - (1-P1) * (1-P2) * (1-P3) = 1 - (0.890109) = 0.109891 That's just under 11% That doesn't take into account how much they win each time though. Calculating their likely winnings is another matter altogether, complicated by the fact that their winnings on the next round are affected by how much they won/lost the previous round.
-
Elegant way to initiate database & other classes in PHP page
btherl replied to Azsen's topic in PHP Coding Help
Azsen, what I was talking about is basically what's in that bootstrapping article. The details of our system are like this, let's say someone requests http://domain.com/admin.php. Apache sends the request to index.php, which includes all the classes and libraries, meaning these are included only in one file. index.php sees that the url is requesting the "admin" page, and looks for the script "admin.php" and the template "admin.html". If it finds them, it includes admin.php and calls the function admin(). Any return values from admin() are passed to smarty for rendering the admin.html template. If it doesn't find them then it will generate either a 404 error or redirect to another page, depending on the needs of that particular site. Creating a new page is then just a matter of creating newpage.php and newpage.html, and the newpage() function. -
That looks like 1 in 15 to me. This is also 1 in 15: $selected_number = mt_rand(1,15); if ($selected_number == 1) { you won } 1 in 15 is not very good odds if they lose their entire wager when they lose. You could do something like 45 out of 100: $selected_number = mt_rand(1,100); if ($selected_number <= 45) { you won } Then they will generally lose in the long run, but it's also likely for them to have lucky streaks.
-
Elegant way to initiate database & other classes in PHP page
btherl replied to Azsen's topic in PHP Coding Help
We use a system where index.php uses the url to determine which php file to include and which function to execute as the entry point from that php file (the function name is derived from the file name). index.php includes everything that is required. It's more or less back to front - the libraries include the code, rather than the code including the libraries, which means there is no code duplicated on every single page. -
I would try using an anonymous function, as in the example on the manual page for preg_replace_callback() Alternatively you can declare name() once outside the loop and have it reference a global variable, which you can set to control its behaviour. But I don't like global variables
-
It's a zombie thread! Run for your lives! Seriously though, that looks like the right solution. If only integration was so simple..
-
Here's a few ideas for troubleshooting. Generic ideas unfortunately, I don't know what your actual problem might be. 1. Login as whatever user the web server runs as and run the command 2. Deliberately give the command files it doesn't have access to and see what happens 3. Execute "dir" on the paths you are giving to the convert command, see if it can access them 4. Execute "move" on files in the paths you are giving to convert 5. Execute your command with the "display help" option, and see if you do indeed get the help displayed as output. If not then it may not be able to access that command at all.
-
help preventing sql injection with pg_escape_string
btherl replied to jake2891's topic in PostgreSQL
Here's the modern way: $check_method_id = $test_db->queryOne("select method_id from tbl_methods where method_id = E'$method_id'"); And the deprecated way: $check_method_id = $test_db->queryOne("select method_id from tbl_methods where method_id = '$method_id'"); The only difference is the "E". It tells postgres that an escaped string follows. In practice it usually doesn't matter, but you might get a warning if you leave the "E" out. Or as the other poster said, if you're dealing with a data type with limited values like an integer, then checking that it only contains digits is enough. Then you don't need to use pg_escape_string for that value. -
Sorry, I didn't see your reply until now. I'm not familiar with the money type myself so I can't comment. We use numeric(9,2) at my workplace where exact money types are required. Sometimes we need more precision (eg we may need to account for partial cents), so we use a double precision there. That can give unexpected results occasionally, but it's ok for the situations we use it in.
-
If you want a list of patients for one specific GP: Select * FROM "Patient" WHERE GP_Name = 'Dr Btherl' ORDER BY Name If you want to do the same but selecting by the gp's id then you will need the join: Select * FROM "Patient" INNER JOIN "GP" ON Patient.GP_Name = GP.GP_Name WHERE GP.ID = 5 ORDER BY Patient.Name I should mention it's a bit odd to be using GP_Name for joining when GP has an ID column. Usually you would put the id column in the patient table and join on id. The reason is that if a GP's name changes, you just need to update the GP table only.
-
Aha .. if they're asking you to use postgresql, then you can't be using phpmyadmin. That's for the more common database package called Mysql. Judging by the wording, this is a report you are doing for a class? In which case your teacher should be able to tell you how you're expected to get access to Postgresql to complete it. It's difficult for me to comment more as I'm not sure how you're supposed to be accessing postgresql, and I'm not sure what features are considered "advanced" Possibly a join is considered advanced if it's a basic database class. Join basically means "Match table 1 and table 2 up wherever column a from table 1 matches column b from table 2". It can get more complex but that's the basic meaning. In your case you want to match the GP column from Patient table with the ID column from GP table, probably. You can write it as F1Fan wrote it above, or here's an alternative: SELECT * FROM Patient JOIN GP ON (Patient.GP = GP.ID) Here I'm using "*" instead of listing columns, and I'm using another syntax for joins, which I find easier to understand. And I'm not aliasing the tables.
-
Typically you'll use a join for that, though you can use a subquery. Most SQL tutorials will cover that. Are you definitely using postgres and not mysql?
-
Try putting single quotes around the money value (I'm taking a wild guess here)
-
Can you post your current code please.
-
What you've posted here is using $_session, not $_SESSION. That was the problem in the original topic you linked to. Only $_SESSION in uppercase will work.
-
Well that is very strange. those functions have been around since php 4.2.0 apparently, so it's not a php version issue. I wonder though if there's a mismatch somewhere between the versions you've got installed, or perhaps they weren't integrated properly. Building from source is not simple, especially for building php so that it can work properly with apache. If you just need things to work, I would go with the pre-packaged versions of everything. If your objective is to learn by building from source and have plenty of free time, then you're in for a ride
-
Can you post the code?