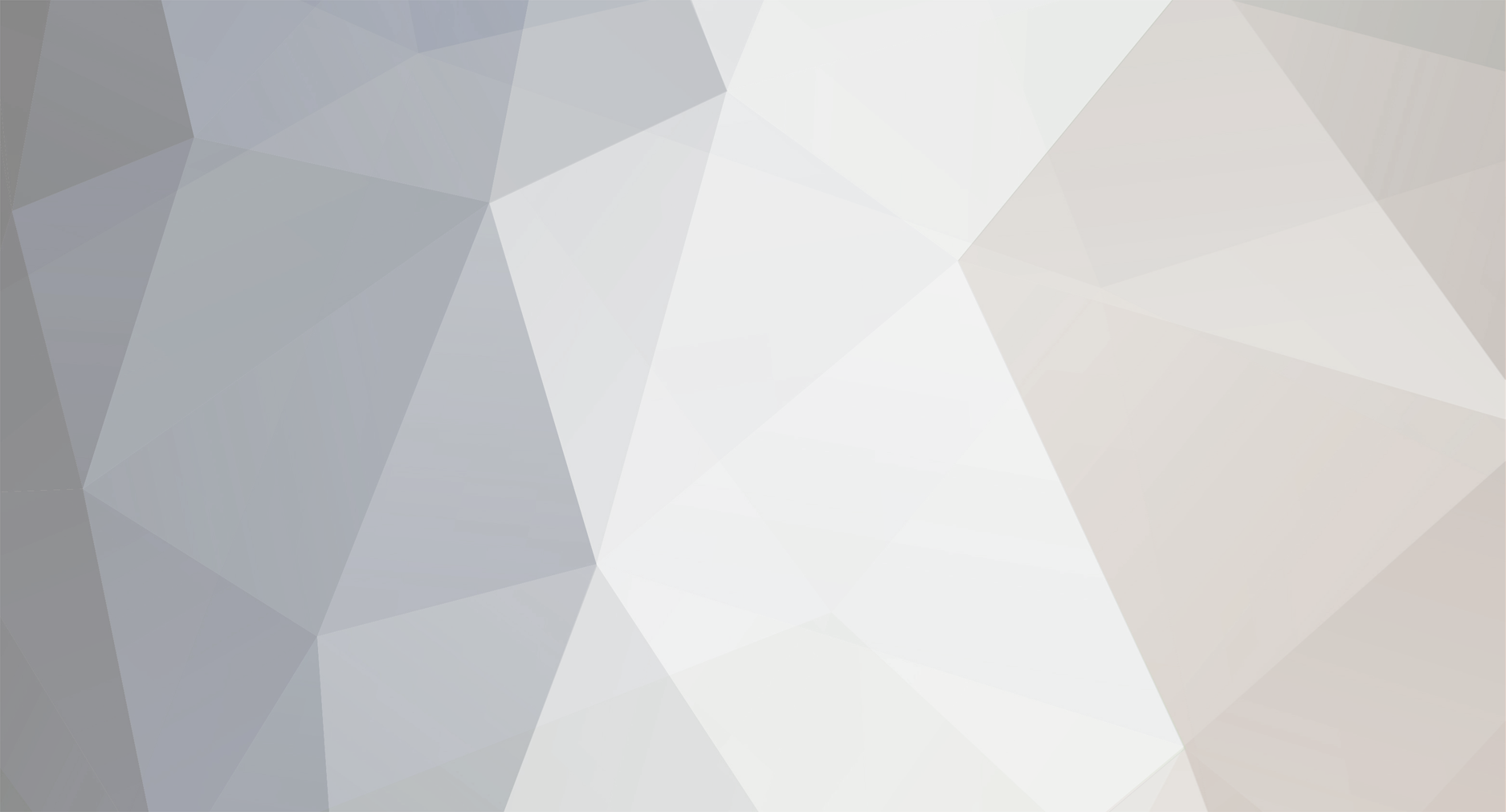
sKunKbad
Members-
Posts
1,832 -
Joined
-
Last visited
-
Days Won
3
Everything posted by sKunKbad
-
Without knowing more about the application, I can only show you code that is similar to what you want to do. $sellers_count = array(); foreach( $sellers_array as $seller ) { if( ! in_array( $seller, $sellers_count ) ) { $sellers_count[] = $seller; } } $total_unique_sellers = count( $sellers_count ); If you simply replace $sellers_array with the actual array of sellers, you should be able to use $total_unique_sellers as your count of unique sellers.
-
stream_context_create() and disappearing cookies
sKunKbad replied to sKunKbad's topic in PHP Coding Help
Turns out that since the requesting script and the requested script were both using sessions, I had to use session_write_close() before file_get_contents(). -
I've been trying to pass a cookie along to one of my sub domains, and the I'm getting an error: An error #2 occurred in script 'C:\xampp\htdocs\optifit-hcg\application\controllers\test.php' on line 66: file_get_contents(http://localhost.optifit-hcg/horz_text_menu) [function.file-get-contents]: failed to open stream: HTTP request failed! The idea is that the script on the sub domain would return some data based on the cookie contents, but when I print_r() both $_SESSION and $_COOKIE the arrays are blank. Please let me know if you see anything wrong: $opts['http']['method'] = 'GET'; $opts['http']['user_agent'] = $_SERVER['HTTP_USER_AGENT']; $cookie_string = 'Cookie: '; // If there are cookies, send them with the request if( count( $_COOKIE ) > 0 ) { $i = 1; foreach( $_COOKIE as $k => $v ) { if( $i !== 1 ) { $cookie_string .= '; '; } $cookie_string .= $k . '=' . urlencode( $v ); $i++; } // Development environment not compiled with curl wrappers if( ENVIRONMENT != 'development' ) { $opts['http']['header'][] = $cookie_string; } else { $opts['http']['header'] = $cookie_string; } } $context = stream_context_create( $opts ); $contents = file_get_contents( 'http://localhost.optifit-hcg/horz_text_menu', FALSE, $context ); echo $contents;
-
Any documentation on .tmp files related to $_FILES ?
sKunKbad replied to sKunKbad's topic in Application Design
Found my answer here: http://php.net/manual/en/features.file-upload.post-method.php -
I've been uploading files, but instead of moving the files from /tmp/ to somewhere on the file system, I've been either storing them in the database, or using FTP functions to send them to another server. I'm not specifically deleting the file that is in /tmp/. I tried to find more about garbage collection, or what happens if I don't move or copy the file out of /tmp/, but have been searching for a while with no luck. If I watch my /tmp/ directory, as I do my uploads I don't even see the file in there for a second. So... Is the file only there until the script finishes, and then it gets deleted, or what? Am I making sense? My concern would be having a bunch of .tmp files building up over time.
-
I'm using a upload class that works with $_FILES, but have a couple of reasons to need the content of php://input: 1) When submitting PDF forms as a complete form, the form is available in php://input. 2) An ajax uploader I'd like to use submits the file making it only available in php://input. I know I could use an if statement to check $_FILES else php://input, but I'm concerned about security, and being able to identify the filetype, get the size, etc. I'm skipping over some other details, because I need to leave in 5 minutes. I've not seen any classes that merge the two ( $_FILES and php://input ), and really haven't seen any that are specifically for php://input. Can anyone offer advice or point me in the right direction?
-
I tested similar code in my local CI playground, and it works fine, so as thorpe said, check mod_rewrite, and also check your .htaccess file. For reference, here is mine: RewriteEngine On RewriteBase / RewriteRule ^(system|application|cgi-bin) - [F,L] RewriteCond %{REQUEST_FILENAME} !-f RewriteCond %{REQUEST_FILENAME} !-d RewriteRule .* index.php/$0 [PT,L]
-
Calling function via a switch does not seem efficient
sKunKbad replied to sKunKbad's topic in Javascript Help
Wow, that's a lot better! I was actually trying to do something like that initially, but didn't know I needed the square brackets. Thanks. -
I'm wondering if there is a more efficient way to call one of the functions in the switch statement below (but without using eval(), or is eval() the best way to go? I just basically need to do a little client side validation in a multi page form, and if the processing function returns true or false, I'll either send the user to the next page or output error messages. switch(page){ case '1': var processing_function = part_1(); break; case '2': var processing_function = part_2(); break; case '3': var processing_function = part_3(); break; case '4': var processing_function = part_4(); break; case '5': var processing_function = part_5(); break; case '6': var processing_function = part_6(); break; case '7': var processing_function = part_7(); break; case '8': var processing_function = part_8(); break; case '9': var processing_function = part_9(); break; case '10': var processing_function = part_10(); break; } if( processing_function ){ // Do something });
-
I know you can set up your curl options to follow redirects, but I'm not aware of any setting that allows you to retrieve the actual URL that you are being redirected to.
-
If you are asking how to get the JSON, you could use an ajax/javascript approach, or use php's file_get_contents function. You could also use cURL. Once the json is returned, you would either deal with it though javascript if you choose that approach, or use php's json_decode function, which turns the json back into an array.
-
How do I pass radio button values to a php email.
sKunKbad replied to frank_solo's topic in PHP Coding Help
The first radio button value is available as $_POST['reservations_01'] -
You're not really echoing it twice. If you look closely, the content of the list items is slightly different.
-
I think a lot of people want to believe their framework is better than CI, so CI takes a lot of beatings. I've only used Kohana and CI, and I only use CI now. If you don't need a well documented framework, and if being part of an "elite" community that believes the best way to learn a framework is to look at the code, and if you want to wait for days to get an answer when you ask a question on a framework user forum, then you might try Kohana. I also have other issues, like performance, that keep me using CI. I've never used Zend, but it has a bad reputation for being really slow. I have about 5 years of php experience, and 2 of those using CI. I feel that I can do anything I want to with CI, and I can do it fast because the documentation made it easy to learn. You could say that CI usage in general is easy, and that's why some might think it is for beginners. Take away the great documentation and the community, and you'd have a framework that is similar to others.
-
When parsing an element's children with simpleXML, the children are returned as an array of objects, and I'd like to be able to get the actual value for validation, but haven't successfully done so. For instance: foreach( $xml->sub_key->children() as $element_name => $value ) { var_dump( $value ); } Output here shows that $value is something like: I've tried to access [0] by $value->0 and $value->{'0'} but that doesn't work. What I'm trying to do, for instance, is use is_numeric() to test the $value, but $value is always an object, so is_numeric won't work on it directly. How can I test with is_numeric()? Actually, even when I try to test the element directly it is an object: if( ! is_numeric( $xml->sub_key->child ) ) { echo gettype( $xml->sub_key->child ); } //returns object
-
The problem with that is that it's not an independent script. It's actually a controller/method in a CodeIgniter installation, and can't be accessed directly.
-
I use Win7 64bit on two computers with no problems. I have little gripes about it, but I like it better than anything else, and I own a mac and two ubuntu computers.
-
But I don't want to write to the file, only read, which it seems to be doing, because the script on it does run. I tried looking at wget config options to see if I could figure it out, but haven't done any testing yet because I haven't had time.
-
Didn't know where to ask this, so thought I ask here. My cron works: 0 * * * * wget "http://mysite.com/test-cron.php" but I get an email from the server that makes it sound like I'm not doing something right: I know I can turn off the email by using >/dev/null 2>&1, but I'm just wondering if I did something wrong, and that's why it says it can't write to the file, and permission denied. I don't care about writing to the file, I just want to run the php so it sends me an email. Also, I'm using wget because the file must be accessed via http for it to work.
-
Actually, what I needed was if A = 0, then update A to 1. If A was already 1, then update B to 1. This SQL statement ended up working: UPDATE `the_table` SET `B` = CASE WHEN `A` = 1 THEN 1 ELSE `B` END, `A` = CASE WHEN `A` = 0 THEN '1' ELSE `A` END WHERE `ID` = 1 I guess I didn't really say why it wasn't working, so nobody would have known what I was after. Thanks for your time.
-
I'm trying to evaluation field A and update field A and B accordingly. If A = 0, then it should become 1. If A = 1, then B should become 1. It seems like it should be so simple, but it's not working: UPDATE `the_table` SET `A` = CASE WHEN `A` = 0 THEN `A` = 1 ELSE `A` END, `B` = CASE WHEN `A` = 1 THEN `B` = 1 ELSE `B` END WHERE `ID` = 1
-
I started with Frontpage for our family business, about 8 years ago. (Early 2003) I switched to Adobe GoLive after about a year of Frontpage usage. I realized that WYSIWYG wasn't the way to go, and started hand coding HTML/CSS about 6 years ago. Started in with php a little over 5 years ago. (About the time I started coming to this forum) I've stumbled through many other languages/technologies, and am pretty comfortable with javascript. About two years ago was introduced to the Kohana and CodeIgniter php frameworks, and now exclusively build websites with CodeIgniter.
-
I had a weird dream and woke up with a stomach ache. I hope this day gets better. Happy Father's Day!
-
The internet is a big scary place, and if you could get a random picture, you'd probably find that most of them would be worthless. That said, if it were me I'd probably use a dictionary and have my script randomly search google for combinations of dictionary words. Then randomly choose one.
-
My point was simply that SF23103 needs to have at least something in the way of validation. Type casting as an integer, or using is_numeric would be a better choice than nothing at all. I had never seen ctype_digit before, but checked it out at php.net. Again, the point is, that left as coded above, there'd be nothing to stop a person from altering the query. SF23103, you need to search Google for "sql injection". There are some good videos out there that teach all about php security. Take the time to learn before creating an application that will get hacked.