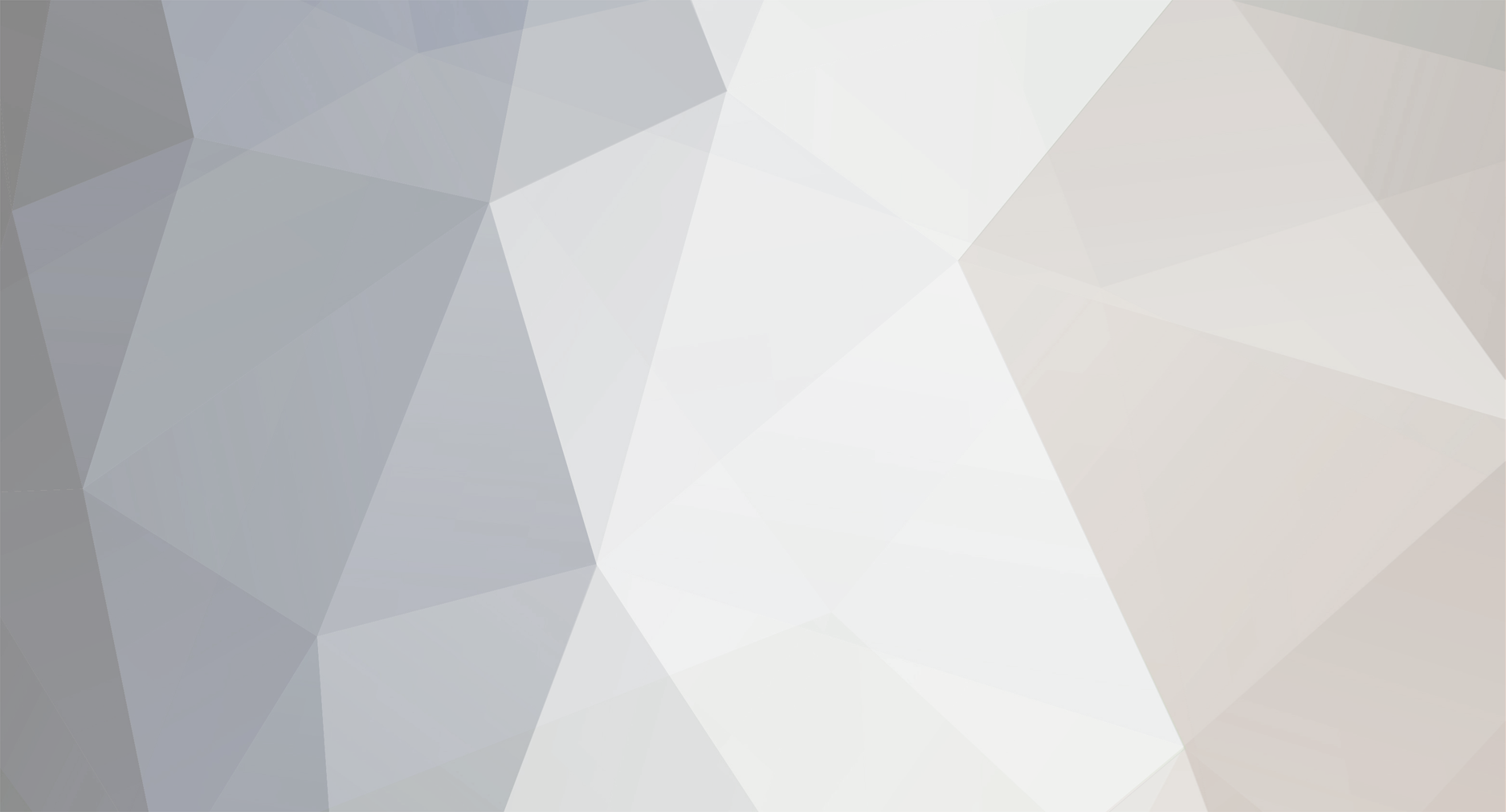
sKunKbad
Members-
Posts
1,832 -
Joined
-
Last visited
-
Days Won
3
Everything posted by sKunKbad
-
Please make sure to validate $id before using it in your query. If you don't, you could suffer from an sql injection attack. A person could craft the value of $id to anything, and they could do anything to your database that the mysql user has privileges to allow. In your case, if $id is always an integer, you should at least do something like: <?php $id = (int) $id; ?>
-
How does one avoid resubmitting search results?
sKunKbad replied to drayarms's topic in PHP Coding Help
If you do change the method, you will also need to change it in the form. Example: <form method='get' action='whatever.php'> <!-- form elements go here --> </form> -
<?php if( isset( $_GET['id'] ) ) { echo 'id is ' . (int) $_GET['id']; } else { echo 'no id in query string'; }
-
How does one avoid resubmitting search results?
sKunKbad replied to drayarms's topic in PHP Coding Help
If you use query strings ($_GET) instead of $_POST, you won't see the annoying message. -
The decoded array shows that o is a stdClass Object, and the same is true for user. <?php $array = json_decode( $the_json ); // You should be able to get the location with something like this: echo $array['o']->user->location;
-
Error handler doesn't work the same on dev / prod environments
sKunKbad replied to sKunKbad's topic in PHP Coding Help
Yes, the function is for sure being called. I'll need to check display_errors. What is odd is that most errors are being sent to me via email, which is a function of the custom error handler. There are just some instances where I'm getting a blank white screen, and removing the custom error handler seems to make that go away. -
I've made a custom error handler, but it doesn't seem to work the same way on the dev and prod environments. Dev is windows/xampp, and prod is a standard LAMP install. Sometimes on prod I get a blank white screen and dev shows no errors. If I remove the error handler, everything goes back to normal. Just wondering if anyone sees something wrong here: <?php function my_error_handler ($e_number, $e_message, $e_file, $e_line, $e_vars) { // email address to email errors to on the production environment $email_address = 'myemailaddress@gmail.com'; switch ($e_number) { case E_USER_ERROR: $error_type = 'E_USER_ERROR'; break; case E_USER_WARNING: $error_type = 'E_USER_WARNING'; break; case E_USER_NOTICE: $error_type = 'E_USER_NOTICE'; break; case E_WARNING: $error_type = 'E_WARNING'; break; case E_NOTICE: $error_type = 'E_NOTICE'; break; case E_STRICT: $error_type = 'E_STRICT'; break; default: $error_type = 'UNKNOWN ERROR TYPE'; break; } $message = '<hr />PHP ' . $error_type . ' #' . $e_number . ' - Date/Time: ' . date('n/j/Y H:i:s') . "\n" . '<br />File: <b>' . $e_file . "</b>\n" . '<br />Line: <b>' . $e_line . "</b>\n" . '<br /><b>' . $e_message . '</b><hr />'; // Output for development environment if (stristr($_SERVER['HTTP_HOST'], 'localhost' )) { echo $message; } // Email for production environment else { error_log($message, 1, $email_address ); if ( $e_number != E_NOTICE && $e_number < E_STRICT) { die('A system error occurred. We apologize for the inconvenience.'); } } // Don't execute PHP internal error handler return true; } function my_error_handling() { set_error_handler('my_error_handler', E_ALL); }
-
I'm working on an image management page, and everything seems to be working, except the confirmation that should come up after every event (changed order or deleted image) only comes up the first time. alert() on line 28 always shows the response, but for whatever reason, the message doesn't get applied to #content after the first time. <!DOCTYPE html> <html lang="en"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>jQuery Dynamic Drag'n Drop</title> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.5.1/jquery.min.js" type="text/javascript"></script> <script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jqueryui/1.8.11/jquery-ui.min.js"></script> <script type="text/javascript"> $(document).ready(function(){ $(function(){ // make the list of images sortable $("#image-ul").sortable({ // allow dragging into the trash can connectWith: '#trash_can', item: 'li', // revert causes bugginess in IE, so left out even though cool //revert: 200, opacity: 0.6, cursor: 'move', // apply this css class to available places to drop within list placeholder: 'placeholder-border', // drop available once mouse has touched droppable area tolerance: 'pointer', update: function(){ var order = $(this).sortable("serialize"); $.post("/drag_drop/update", order, function(theResponse){ // show the confirmation and fade it away after 2.5 seconds alert(theResponse); $("#content").html(theResponse).delay(2500).fadeOut('slow'); }); } }); // allow for deleting images by sending them to the trash can $("#trash_can").droppable({ accept: '#image-ul > li', // when the trash can is hovered on by a draggable, set the css class hoverClass: 'delete-border', drop: function(event, ui) { // setTimeout takes care of IE bug where deleted item remains setTimeout(function() { ui.draggable.remove(); }, 1); deleteImage(ui.draggable,ui.helper); } }); // what to do when an image is dropped into the trash can function deleteImage($draggable,$helper){ // strip out "image_data_" so that only the actual image ID is sent to delete query var id = $draggable.attr('id'); id = id.replace('image_data_',''); params = { 'id': id, 'path': $draggable.find("img").attr("src") } $.ajax({ url: '/drag_drop/delete', type: 'POST', data: params }); // provides a css class to an image that was just dropped // which I think looks crappy, so I'll just comment it out $helper.effect('transfer', { to: '#trash_can', className: 'purple' },500); } }); }); </script> <style> #image-list { float:left; width:500px; } ul#image-ul {margin:0;padding:0;} #image-ul li {float:left; list-style:none; margin-right:10px;margin-bottom:10px;} #outer-shell {float:left; width:100%; height:100px;} #content p {padding:10px;background-color:yellow;} .sortable-element{width:100px; height:100px;border:solid 2px #fff;} .placeholder-border{width:100px;height:100px;border:dashed 2px #999;-moz-border-radius:10px;border-radius:10px;} .purple{border:dashed 6px red;-moz-border-radius:10px;border-radius:10px;} #trash_can{float:left;width:100px;height:100px;} .unhovered {background:url(/img/trash-can.jpg)} .delete-border{background-image:url('/img/trash-can-hovered.jpg');} </style> </head> <body> <div id="outer-shell"> <div id="content"> </div> </div> <div id="image-list"> <ul id="image-ul"> <?php if($images !== FALSE) { foreach ($images as $k => $v ) { echo '<li class="sortable-element" id="image_data_' . $v['id'] . '"><img src="' . $v['path'] . '" width="100" height="100" /></li>' . "\n"; } } else { echo '<li>NO IMAGES TO LIST</li>'; echo '<li>Go <a href="/">Home</a></li>'; } ?> </ul> </div> <div id="trash_can" class="unhovered"> </div> </body> </html> Any advice?
-
I'm trying to get all of my rows to have the category IDs that belong to them, but I'm not doing it right. My query: SELECT l . * , c.id AS category_id FROM listings l LEFT JOIN categories c ON l.id = c.id LIMIT 0 , 30 There are only two categories, and four listings. The first two listings have the correct category id in the result row, but the last two listings have NULL. Is there a way to have the category ids in all of the rows?
-
Look at php.net's uasort() page. http://us.php.net/manual/en/function.uasort.php. You might have to modify some code to suit your needs, but that function will be useful. Consider this: function compare($x, $y) { if ( $x[0]['created'] == $y[0]['created'] ) { return 0; } return ($a < $b) ? -1 : 1; }
-
How can you go to a non-existant URL and still get a page there?
sKunKbad replied to millicent's topic in Apache HTTP Server
The blog may be accessible through some url rewriting in an .htaccess file. You might search for mod rewrite. Also, depending on if the site is built in a framework, there may be a custom route. If you have all of the files on your computer, try searching the files for /blog/ and see what comes up. -
How to force 404 or 410 if query string contains 'attachment_id'
sKunKbad replied to sKunKbad's topic in Apache HTTP Server
Well, I figured out a solution based on stuff I saw at corz.org: RewriteCond %{QUERY_STRING} attachment_id=(.*) RewriteRule ^(.*) /404 [L] This is for Wordpress, and while I'd rather have Wordpress display it's own 404 page, I just had to get something working. -
I'm trying to force an error (either 404 or 410) if 'attachment_id' is in the query string. I had tried something with mod_rewrite, and then thought that this is probably not even something that needs to be done with mod_rewrite, but I don't know how to handle this. Any suggestions, links to something similar, or help with actual code is appreciated.
-
The current contrast is hard on the eyes, and I have good eyes, so that means do something about it. A HTML table is only really meant to hold data, like a spreadsheet. You have used it for page structure, which is bad. Is that what they teach you in this class? I see you said that the professor said this is beyond the scope of his class. I'd say you should start over, and look at some CSS templates that validate. Learn how to use relative vs. absolute positioning. You really just have a simple two column layout with a header and a footer, so do it again.... but better.
-
Since it's for a bar, you probably need more pictures of girls, beer, and sports stuff.
-
It's just rather plain, but my real complaint would be that the main menu and the slideshow are placed higher in the code than the actual content. Also, you really shouldn't need that IE emulate IE7 meta tag. A proper template would work in IE without this meta tag.
-
I think the key is that strpos can return a boolean FALSE or TRUE, so you would normally want to test for FALSE or TRUE: if(strpos($haystack, $needle) !== FALSE) { } // or if(strpos($haystack, $needle) === FALSE) { }
-
You can try caching some views. Depending on the type of traffic you are getting, this can speed things up.
-
Actually, CodeIgniter does not have a built in authentication class, so not just any mainstream PHP framework will meet the OPs needs. I've tried Kohana, and it has an authentication class. I don't use Kohana anymore, but the OP might check it out.
-
You might think of the ! as making things backwards. So: if( 3>2) means if 3 is greater than 2 if( ! 3>2 ) means if 3 is not greater than 2 if (3<2) is the same as if(!3>2) because ! makes it backwards This is a really simple, and perhaps lame way of explaining it, but since you asked, then you probably need to read a book on php!
-
If you're using 1.7.2, there really aren't any reasons to upgrade. 2.0 doesn't do a whole lot more for you. They say it's ready, but they why haven't they released it?
-
INSERT INTO `myDB.titles` (`Title`, `Year`, `cID`) VALUES ('Test', '2000', '2'); You could also try running the insert straight from phpMyAdmin and see what errors it spits out at ya.
-
I'm trying to pass some options over to the jquery superfish plugin, based on if the browser is IE7 or 8. I've asked elsewhere with no answer, so hoping somebody here can help. In the code below, I want to set animation and speed, but different values if IE7 or 8. Currently it breaks superfish: $(document).ready(function() { $('ul.sf-menu').supersubs({ minWidth: 12, maxWidth: 27, extraWidth: 1 }).superfish({ animation: (function(){ if ($.browser.msie && $.browser.version > 6){ return "{height:'show'}"; } else { return "{animation:'show'}"; } }), speed: (function(){ if ($.browser.msie && $.browser.version > 6){ return 'fast'; } else { return 'normal'; } }) }); $('.sf-menu > li a:not(li li a)').css({ '-moz-border-radius': '3px', '-webkit-border-radius': '3px', 'border-radius': '3px' }); }); I've been going around in circles with this for an hour or two. Any help is appreciated.
-
Site does look good, but why a table based layout? For the purpose of SEO alone, you would be better off using modern CSS styling.