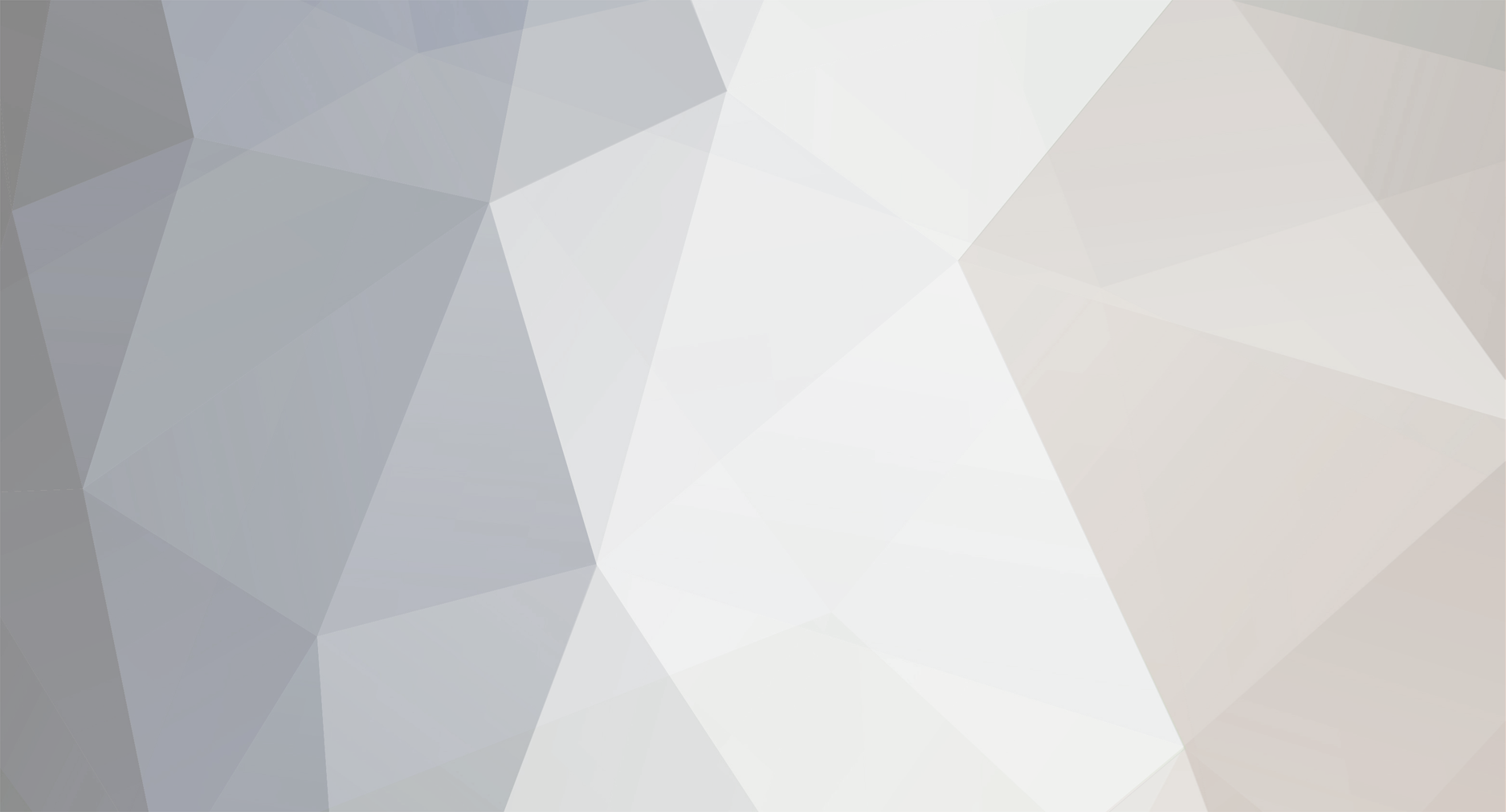
sKunKbad
Members-
Posts
1,832 -
Joined
-
Last visited
-
Days Won
3
Everything posted by sKunKbad
-
You could apply a height to the outer columns, but that really isn't the best way to handle a 3 column layout. Normally, you would just use a background image that makes the columns look like they are going all the way down. Apply this background image to the div that contains your columns, and your good to go. My website uses something similar, so you might take a look.
-
Followlocation allows curl to follow redirects. I don't think there is much that you can do to duplicate this behavior. I'm curious about the security exploit. I've never heard of this, and not being able to use Followlocation really hurts the way I use cURL. I'd change hosts.
-
Yes, you still have to log in, this is just how to get there
-
Dont know if this is a confirmation, but these are my notes for tunneling in to my phpmyadmin via Putty: So, I'm pretty sure in this case, your script/apache would recognize the IP as 127.0.0.1. I'd have to check my server log to be sure.
-
something like this: RewriteRule ^(myword)/([0-9]*)$ /index.html?sid=10&cid=$2 the backslash before index might need to be removed???
-
There's a lot to think about when authenticating a user. I'm certainly no expert, but I've been working for a long while (in my spare time) on a login system. Searching the internet for topics such as "session hijacking" and "session fixation", as well as "sql injection", "XSS attacks", etc. will give you a good preview of what you will be planning for when making your login script. I'm going to post some code below for you to check out. This is only part of the login script I've been working on, but it might help steer you in a direction where you understand more about what you are doing: <?php /* This script is used to check if a person is a user or admin. Cookies, must be enabled on the client's browser for this script to work. Another option would be to have the session ID appended to the URL by setting session.use_trans_sid to true in the server's php.ini file. Author: Robert Brian Gottier Date: January 15, 2009 Example usage for a user page: if (!isset($user_is_logged_in) || $user_is_logged_in == 'false') { //show login form, or redirect to the login form }else{ // display user's content // the specific user is determined by the $clean_user_id variable } Example usage for an admin page: if (!isset($admin_is_logged_in) || $admin_is_logged_in == 'false') { //show login form, or redirect to the login form }else{ // display admin's content } SQL to get started: CREATE TABLE `admins` ( `admin_id` tinyint(3) NOT NULL auto_increment, `username` varchar(12) NOT NULL, `password` varchar(40) NOT NULL, `timestamp` int(10) NOT NULL, `login_hold` int(10) NOT NULL default '0', PRIMARY KEY (`admin_id`), UNIQUE KEY `username` (`username`) ) ENGINE=InnoDB; CREATE TABLE `admin_login_attempts` ( `admin_id` tinyint(3) NOT NULL, `attempt_count` tinyint(2) NOT NULL default '0', `last_attempt` int(10) NOT NULL ) ENGINE=InnoDB; CREATE TABLE `ip_login_attempts` ( `IPaddr` varchar(15) NOT NULL, `attempt_count` tinyint(2) NOT NULL, `login_hold` int(10) default '0', `last_attempt` int(10) NOT NULL ) ENGINE=InnoDB; CREATE TABLE `users` ( `user_id` smallint(5) NOT NULL auto_increment, `username` varchar(12) NOT NULL, `password` varchar(40) NOT NULL, `timestamp` int(10) NOT NULL, `login_hold` int(10) NOT NULL default '0', PRIMARY KEY (`user_id`), UNIQUE KEY `username` (`username`) ) ENGINE=InnoDB; CREATE TABLE `user_login_attempts` ( `user_id` smallint(5) NOT NULL, `attempt_count` tinyint(2) NOT NULL default '0', `last_attempt` int(10) NOT NULL ) ENGINE=InnoDB; Users and admins will still need to be able to log out. Logging out usually requires that the session is unset, and that the user or admin is redirected to somewhere. */ // initialize some variables $user_is_logged_in = 'false'; $login_error = 0; $admin_is_logged_in = 'false'; $admin_login_error = 0; // check if this is a login form submission if(isset($post_submit_login) && $post_submit_login == 'submit'){ // If the form post did not come from the same domain on the development or production server, according to the HTTP_REFERER value, then the form post is an attack because of the way I have implemented the login form. if (isset($_SERVER['HTTP_REFERER']) && !stristr($_SERVER['HTTP_REFERER'], DOMAIN ) && !stristr($_SERVER['HTTP_REFERER'], SHARED_SSL_DOMAIN ) && !stristr($_SERVER['HTTP_REFERER'], LOCAL_DOMAIN )){ die('die!'); } // If the POST vars did not pass filter_input() if ($post_login_username == FALSE || $post_login_username == NULL || $post_login_password == FALSE || $post_login_password == NULL){ $login_error = 1; $user_is_logged_in = 'false'; $admin_login_error = 1; $admin_is_logged_in = 'false'; } // A username or password can not be more than MAX_USERNAME_LENGTH or MAX_PASSWORD_LENGTH characters, and a password cannot be less than MIN_PASSWORD_LENGTH chracters. If either posted values are not the appropriate character length, login fails if( strlen( $post_login_username ) > MAX_USERNAME_LENGTH || strlen( $post_login_password ) > MAX_PASSWORD_LENGTH || strlen( $post_login_password ) < MIN_PASSWORD_LENGTH ){ $login_error = 1; $user_is_logged_in = 'false'; $admin_login_error = 1; $admin_is_logged_in = 'false'; } // Check if the remote IP address is on hold // This might hinder a brute force attack if the attacker isn't spoofing the IP address $ip_on_hold = 0; if( isset($_SERVER['REMOTE_ADDR']) && $_SERVER['REMOTE_ADDR'] != '' && $_SERVER['REMOTE_ADDR'] != NULL ){ $clean_remote_ip = clean_for_query($_SERVER['REMOTE_ADDR']); $k = mysqli_query($db , "SELECT login_hold FROM `ip_login_attempts` WHERE IPaddr = '$clean_remote_ip' LIMIT 1"); $l = mysqli_num_rows($k); if($l == 1){ $row = mysqli_fetch_assoc($k); if ($row['login_hold'] > time() ){ $user_is_logged_in = 'false'; $admin_is_logged_in = 'false'; $login_error = 1; $admin_login_error = 1; $ip_on_hold = 1; } } mysqli_free_result($k); } // check if the user is on hold $user_on_hold = 0; $clean_login_username = clean_for_query($post_login_username); $m = mysqli_query($db , "SELECT login_hold FROM `users` WHERE username = '$clean_login_username' LIMIT 1"); $n = mysqli_num_rows($m); if($n == 1){ $row = mysqli_fetch_assoc($m); if ($row['login_hold'] > time() ){ $user_is_logged_in = 'false'; $login_error = 1; $user_on_hold = 1; } } mysqli_free_result($m); // check if the admin is on hold $admin_on_hold = 0; $clean_login_username = clean_for_query($post_login_username); $m = mysqli_query($db , "SELECT login_hold FROM `admins` WHERE username = '$clean_login_username' LIMIT 1"); $n = mysqli_num_rows($m); if($n == 1){ $row = mysqli_fetch_assoc($m); if ($row['login_hold'] > time() ){ $admin_is_logged_in = 'false'; $admin_login_error = 1; $admin_on_hold = 1; } } mysqli_free_result($m); // If the login attempt seems normal so far, and the login attempt has not produced an error thus far, do potential clean-up of the database. // This could be put into a cron job, but in case a person has no access to cron, then it ought to be performed here. if( $user_on_hold == 0 && $admin_on_hold == 0 && $ip_on_hold == 0 && $login_error == 0 && $admin_login_error == 0 ){ $old_hold = time() - ( LOGIN_RESTRICTION_MINS * 60); mysqli_query($db , "DELETE FROM `user_login_attempts` WHERE last_attempt < '$old_hold'; DELETE FROM `admin_login_attempts` WHERE last_attempt < '$old_hold'; DELETE FROM `ip_login_attempts` WHERE last_attempt < '$old_hold'" ); } // if there was no error with login form submission validation, check if the user's username and password match a database row if($login_error != 1 && $user_on_hold != 1 && $ip_on_hold != 1){ $clean_login_username = clean_for_query($post_login_username); $clean_login_password = clean_for_query($post_login_password); $super_password = sha1(PASSWORD_SALT . $clean_login_password); $a = mysqli_query($db , "SELECT * FROM `users` WHERE username = '$clean_login_username' AND password = '$super_password' LIMIT 1"); $b = mysqli_num_rows($a); if($b == 1){ // if they match, then add some session keys, and $user_is_logged_in = 'true' $row = mysqli_fetch_assoc($a); $fingerprint = $row['timestamp']; session_regenerate_id(); $_SESSION['user_id'] = $row['user_id']; $_SESSION['user_token'] = sha1($fingerprint . session_id() . $_SERVER['HTTP_USER_AGENT']); $user_is_logged_in = 'true'; mysqli_query($db , "UPDATE `users` SET login_hold = 0 WHERE user_id = '{$row['user_id']}' LIMIT 1"); mysqli_query($db , "DELETE FROM `user_login_attempts` WHERE user_id = '{$row['user_id']}' LIMIT 1"); if( isset($_SERVER['REMOTE_ADDR']) && $_SERVER['REMOTE_ADDR'] != '' && $_SERVER['REMOTE_ADDR'] != NULL ){ // clean up the ip_login_attempts log $clean_remote_ip = clean_for_query($_SERVER['REMOTE_ADDR']); mysqli_query($db , "DELETE FROM `ip_login_attempts` WHERE IPaddr = '$clean_remote_ip' LIMIT 1"); } }else{ // if they don't match, then the form submission matched no user $login_error = 1; $user_is_logged_in = 'false'; } mysqli_free_result($a); } // if there was no error with login form submission validation, check if the admin's username and password match a database row if($admin_login_error != 1 && $admin_on_hold != 1 && $ip_on_hold != 1){ $clean_login_username = clean_for_query($post_login_username); $clean_login_password = clean_for_query($post_login_password); $super_password = sha1(PASSWORD_SALT . $clean_login_password); $a = mysqli_query($db , "SELECT * FROM `admins` WHERE username = '$clean_login_username' AND password = '$super_password' LIMIT 1"); $b = mysqli_num_rows($a); if($b == 1){ // if they match, then add some session keys, and $admin_is_logged_in = 'true' $row = mysqli_fetch_assoc($a); $fingerprint = $row['timestamp']; session_regenerate_id(); $_SESSION['admin_id'] = $row['admin_id']; $_SESSION['admin_token'] = sha1($fingerprint . session_id() . $_SERVER['HTTP_USER_AGENT']); $admin_is_logged_in = 'true'; mysqli_query($db , "UPDATE `admins` SET login_hold = 0 WHERE admin_id = '{$row['admin_id']}' LIMIT 1"); mysqli_query($db , "DELETE FROM `admin_login_attempts` WHERE admin_id = '{$row['admin_id']}' LIMIT 1"); if( isset($_SERVER['REMOTE_ADDR']) && $_SERVER['REMOTE_ADDR'] != '' && $_SERVER['REMOTE_ADDR'] != NULL ){ // clean up the ip_login_attempts log $clean_remote_ip = clean_for_query($_SERVER['REMOTE_ADDR']); mysqli_query($db , "DELETE FROM `ip_login_attempts` WHERE IPaddr = '$clean_remote_ip' LIMIT 1"); } }else{ // if they don't match, then the form submission matched no admin $admin_login_error = 1; $admin_is_logged_in = 'false'; } mysqli_free_result($a); } $try_ip = 0; // If a login attempt was made with an actual user's username, but the password was wrong, log the attempt if( $login_error == 1 ){ $clean_login_username = clean_for_query($post_login_username); $e = mysqli_query($db , "SELECT user_id FROM users WHERE username ='$clean_login_username' LIMIT 1"); $f = mysqli_num_rows($e); if ( $f == 1 ){ // If the post contains a real username $row = mysqli_fetch_assoc($e); $failed_user_id = $row['user_id']; mysqli_free_result($e); $i = mysqli_query($db , "SELECT attempt_count FROM user_login_attempts WHERE user_id ='$failed_user_id' LIMIT 1"); $j = mysqli_num_rows($i); if ( $j == 1 ){ // If the user already has a failed login attempt $row = mysqli_fetch_assoc($i); $previous_attempts = $row['attempt_count']; $current_attempts = $previous_attempts + 1; $hold_time = time() + ( LOGIN_RESTRICTION_MINS * 60); if ($current_attempts >= LOGIN_ATTEMPT_LIMIT) { // set or reset the login hold if the user has LOGIN_ATTEMPT_LIMIT failed login attempts or greater mysqli_query($db, "UPDATE `users` SET login_hold = '$hold_time' WHERE user_id = '$failed_user_id'"); } mysqli_query($db, "UPDATE `user_login_attempts` SET attempt_count = '$current_attempts' , last_attempt = " . time() . " WHERE user_id = '$failed_user_id'"); }else{ // Else create a database row to start loggin failed login attempts mysqli_query($db, "INSERT INTO `user_login_attempts` (user_id,attempt_count,last_attempt) VALUES ('$failed_user_id',1," . time() . ")"); } mysqli_free_result($i); }else{ $try_ip = 1; } } // If a login attempt was made with an actual admin's username, but the password was wrong, log the attempt if( $admin_login_error == 1 ){ $clean_login_username = clean_for_query($post_login_username); $e = mysqli_query($db , "SELECT admin_id FROM admins WHERE username ='$clean_login_username' LIMIT 1"); $f = mysqli_num_rows($e); if ( $f == 1 ){ // If the post contains a real admin's username $row = mysqli_fetch_assoc($e); $failed_admin_id = $row['admin_id']; mysqli_free_result($e); $i = mysqli_query($db , "SELECT attempt_count FROM admin_login_attempts WHERE admin_id ='$failed_admin_id' LIMIT 1"); $j = mysqli_num_rows($i); if ( $j == 1 ){ // If the admin already has a failed login attempt $row = mysqli_fetch_assoc($i); $previous_attempts = $row['attempt_count']; $current_attempts = $previous_attempts + 1; $hold_time = time() + ( LOGIN_RESTRICTION_MINS * 60); if ($current_attempts >= LOGIN_ATTEMPT_LIMIT) { // set or reset the login hold if the admin has LOGIN_ATTEMPT_LIMIT failed login attempts or greater mysqli_query($db, "UPDATE `admins` SET login_hold = '$hold_time' WHERE admin_id = '$failed_admin_id'"); } mysqli_query($db, "UPDATE `admin_login_attempts` SET attempt_count = '$current_attempts' , last_attempt = " . time() . " WHERE admin_id = '$failed_admin_id'"); }else{ // Else create a database row to start loggin failed login attempts mysqli_query($db, "INSERT INTO `admin_login_attempts` (admin_id,attempt_count,last_attempt) VALUES ('$failed_admin_id',1," . time() . ")"); } mysqli_free_result($i); }else{ $try_ip += $try_ip; } } // If a login attempt was made with no match for user's username or admin's username, log the attempt by IP address (if possible) if($try_ip == 2){ if(isset($_SERVER['REMOTE_ADDR']) && $_SERVER['REMOTE_ADDR'] != '' && $_SERVER['REMOTE_ADDR'] != NULL){ $clean_remote_ip = clean_for_query($_SERVER['REMOTE_ADDR']); $g = mysqli_query($db , "SELECT attempt_count FROM ip_login_attempts WHERE IPaddr = '$clean_remote_ip'"); $h = mysqli_num_rows($g); if ( $h == 1 ){ $row = mysqli_fetch_assoc($g); $previous_attempts = $row['attempt_count']; $current_attempts = $previous_attempts + 1; $hold_time = time() + ( LOGIN_RESTRICTION_MINS * 60); if ($current_attempts >= LOGIN_ATTEMPT_LIMIT) { mysqli_query($db, "UPDATE `ip_login_attempts` SET attempt_count = '$current_attempts', last_attempt = '" . time() . "', login_hold = '$hold_time' WHERE IPaddr = '$clean_remote_ip'"); }else{ mysqli_query($db, "UPDATE `ip_login_attempts` SET attempt_count = '$current_attempts', last_attempt = '" . time() . "' WHERE IPaddr = '$clean_remote_ip'"); } }else{ mysqli_query($db, "INSERT INTO `ip_login_attempts` (IPaddr,attempt_count,last_attempt) VALUES ( '$clean_remote_ip' , 1 , " . time() . ")"); } mysqli_free_result($g); } } // If max logins are preventing a user / admin / attacker from logging in, show this message if ( $user_on_hold == 1 || $admin_on_hold == 1 || $ip_on_hold == 1 ){ echo "You have exceeded a normal number of login attempts, and must now wait " . LOGIN_RESTRICTION_MINS . " minutes before trying again. Please come back later."; } } // if the user or admin is not logging in through the form, we need to check who they are // the session variables are used to see if the person is a user if(isset($_SESSION['user_id']) && isset($_SESSION['user_token'])){ $clean_user_id = clean_for_query($_SESSION['user_id']); $c = mysqli_query($db , "SELECT * FROM `users` WHERE user_id = '$clean_user_id' LIMIT 1"); $d = mysqli_num_rows($c); if($d == 1){ // if there was a match for the user's ID, we proceed to check the token $row = mysqli_fetch_assoc($c); $fingerprint = $row['timestamp']; if($_SESSION['user_token'] = sha1($fingerprint . session_id() . $_SERVER['HTTP_USER_AGENT'])){ // user's timestamp, session ID, and user agent must stay the same session_regenerate_id(); $user_is_logged_in = 'true'; }else{ // if user's timestamp, session ID, and user agent are not the same, they will have to log in again $login_error = 1; $user_is_logged_in = 'false'; } } mysqli_free_result($c); } // the session variables are used to see if the person is an admin if(isset($_SESSION['admin_id']) && isset($_SESSION['admin_token'])){ $clean_admin_id = clean_for_query($_SESSION['admin_id']); $c = mysqli_query($db , "SELECT * FROM `admins` WHERE admin_id = '$clean_admin_id' LIMIT 1"); $d = mysqli_num_rows($c); if($d == 1){ // if there was a match for the admin's ID, we proceed to check the token $row = mysqli_fetch_assoc($c); $fingerprint = $row['timestamp']; if($_SESSION['admin_token'] = sha1($fingerprint . session_id() . $_SERVER['HTTP_USER_AGENT'])){ // admin's timestamp, session ID, and user agent must stay the same session_regenerate_id(); $admin_is_logged_in = 'true'; }else{ // if admin's timestamp, session ID, and user agent are not the same, they will have to log in again $admin_login_error = 1; $admin_is_logged_in = 'false'; } } mysqli_free_result($c); } ?>
-
I actually tried for at least a half hour to figure out why the menu doesn't work in IE6, and I couldn't get it working. I'm sorry that your stuck with this menu, because I'd be pulling my hair out if it was my responsibility. Because I have had similar problems with other menus working in IE6, I'm going to take a guess that IE6 isn't recognizing the way :hover is being implemented, or that IE6 is evaluating the CSS specificity in a way that is unique. Sorry, but I just can't spend more time on this. Good luck.
-
You always need to start with the basics when trying to debug CSS. The CSS for your test/nav generates 18 warnings. Fix those first, and see what happens: line 119 column 7 - Warning: <a> attribute "href" lacks value line 135 column 7 - Warning: <a> attribute "href" lacks value line 22 column 8 - Warning: trimming empty <span> line 24 column 8 - Warning: trimming empty <span> line 40 column 8 - Warning: trimming empty <span> line 42 column 8 - Warning: trimming empty <span> line 61 column 8 - Warning: trimming empty <span> line 63 column 8 - Warning: trimming empty <span> line 78 column 8 - Warning: trimming empty <span> line 80 column 8 - Warning: trimming empty <span> line 91 column 8 - Warning: trimming empty <span> line 93 column 8 - Warning: trimming empty <span> line 104 column 8 - Warning: trimming empty <span> line 106 column 8 - Warning: trimming empty <span> line 120 column 8 - Warning: trimming empty <span> line 122 column 8 - Warning: trimming empty <span> line 136 column 8 - Warning: trimming empty <span> line 138 column 8 - Warning: trimming empty <span>
-
So, you must be using some sort of form creation class eh? You should supply more code, and then you have a better chance of having somebody help you.
-
Hey, that's cool! I've read a lot of books on php, and not one of them showed the use of AND and OR. I always thought that && and || were the only choices for AND and OR.
-
I found a solution that worked for me. I just put the following in my root .htaccess file: SETENV PHPRC /HOME/PUBLIC_HTML/php.ini I have a subdomain that is in a directory off of public_html, and it was showing errors after I did this, so I just put the same code in its directory, but changed the path so that it had its own php.ini, made the specific changes necessary, and all is well. I guess this only works if you or your host is running php in CGI mode, which mine is. I did however find solutions that would have worked regardless of what way php is running.
-
I've got a shared hosting account, and it turns out that each directory needs its own php.ini. If I was in full control, then I could make a symbolic link in each sub-dir, and that way when a main php.ini in the www root was changed the sub-dir php.inis would also. So, am I stuck putting a php.ini in each sub-dir? Is there a way to have a symbolic link in php that mimics the behavior of the normal linux symbolic link? What are my options, if any?
-
AND is &&, and OR is ||
-
You just use 2 seperate INSERT queries.
-
I'm sort of guessing about what you want, because your question is not very clear. If you want the phrase to change when somebody selects an option, then you're going to use javascript to replace the innerHTML of an elements ID (which you access by getElementByID()). You will need to use an event handler to have javascript do this. This is simple stuff. Good luck.
-
Just use some simple regex to scan posts for the links, and then replace them with the linkified version. preg_match() or preg_replace are probably good functions to look at.
-
need ip locator script... anyone can suggest something
sKunKbad replied to Looktrne's topic in PHP Coding Help
it's been a long time, but a long time ago there was a script called ip2country. If you search for it, I'm sure you will find it. -
how to submit similar to onclick:submit() but should work w/o javascript
sKunKbad replied to syntaxerror's topic in HTML Help
It sounds like you are asking for a simple HTML form, which doesn't require javascript, and can post to whatever script you specify in the action. Take a look here for more information about basic forms: http://w3schools.com/html/html_forms.asp -
Yes, you are obviously right. Your olsenportfolio.com site looks pretty nice.
-
I used to swear that the Google sandbox conspiracy theory was true, and I still believe new or recently new sites are penalized. You could be feeling the effects of this. I'm not trying to start an argument, this is just my opinion. One thing I know is for sure, Ranking/placement doesn't change quickly. You are likely stuck in your current position for 1 to 2 months.
-
I don't think image replacement of headers will actually penalize your site by itself. I've done it for sites that ranked well, even #1, so you obviously aren't going to be penalized enough to make a difference (or at all). I think Google is smart enough to realize what you are trying to do isn't bad. While the text-indent isn't my first choice for image replacement, I think all of the popular image replacement techniques are similar. I personally prefer to absolutely position the text and image in seperate divs, stacked one on top of the other, and use z-index for displaying the image. The benefit to this is that when images are turned off, your page will still make sense. And yes, people do use the web with images turned off.
-
The following is not real code (obviously), but is how I'd try to make it work: SELECT customer_id from orders // php that puts all query results in an array SELECT id from customers WHERE id NOT (in the array of customer who have orders)
-
[SOLVED] PHP Sessions not working on Linux Host
sKunKbad replied to sunwukung's topic in PHP Coding Help
Is there any chance that you are overlooking a character out of place in your real code? If we can look at it, we might be able to point out a mistake. I guess if it works on your home server, then there's really no reason it shouldn't work on the hosted server. I would do a little more testing, and then switch hosts. You shouldn't have to deal with irritating stuff like this. -
http://www.php.net/manual/en/function.unlink.php
-
First, let me say that I hate Flash. Not that it is important to this question, but I hate using Flash, seeing Flash content, and I wish it would just go away. Now here's my problem. I made a simple .swf for a lady, and she doesn't want an http request / download for it when the page loads. This .swf is about 2MB, and she wants it downloaded and played only when the user clicks on "play". "play" could be a video player of some sort, or even another light-weight .swf. I just don't have enough experience to do this without help. I promise if you help, I will never use Flash again, and then you won't have to help me again! Thanks, Brian