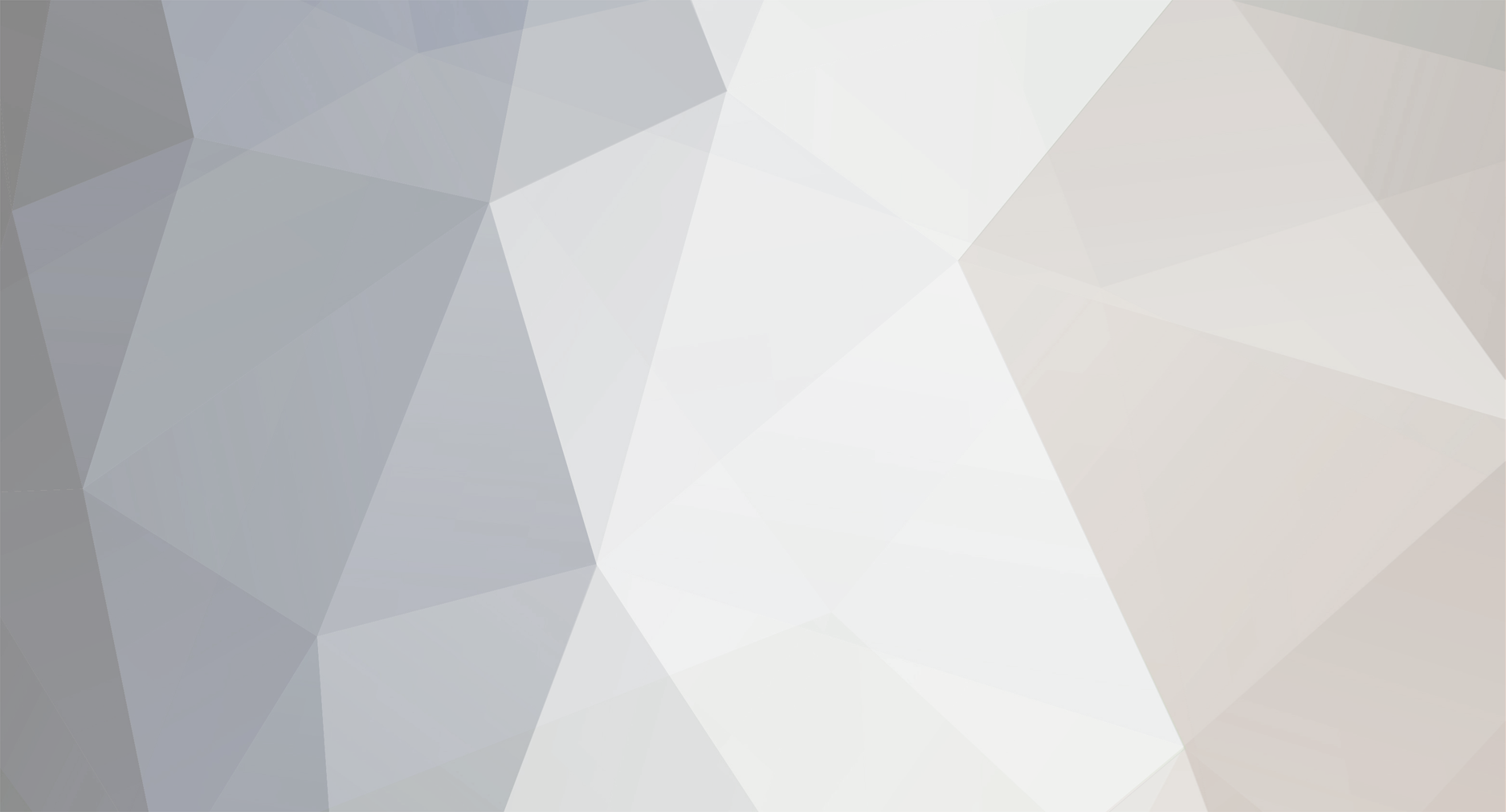
JasonLewis
Members-
Posts
3,347 -
Joined
-
Last visited
Everything posted by JasonLewis
-
Ah, yeah. Easy, instead of doing it like this: $id = 80; include('path/to/file.php'); Use: $_GET['id'] = 80; Then the code I showed you before in posts.php can be almost changed back, but we'll use something a bit different. if(isset($_GET['id']) && preg_match('/[0-9]+/', $_GET['id'])){ $id = $_GET['id']; }else{ $id = 2; } That checks to see if the ID has been set and that it is a number, you could also use is_numeric if you wanted to instead of the regular expression.
-
Parametrized Queries - Why are they not popular?
JasonLewis replied to bachx's topic in PHP Coding Help
You might need to write a few extra lines, but in the long run it could save you lines. It also removes any overhead from creating multiple queries, because it allows you to bind more params to the statement then execute it again. Most people tend to just use MySQL, however I would recommend switching to at least MySQLi, but I myself prefer to use PDO, as it gives you the added functionality of switching to a different database driver without having to go through and change up all your code. -
Yes, and then replace any occurrences of $_GET[id] with just $id.
-
On a linux box (not that I'm a pro or anything) you don't need to specify a location to run a PHP script from the command line. All you need to write is: php -f [file] Or whatever the command is. As for Windows, perhaps provide a prompt for the user to input the location of their php.exe?
-
There there is something wrong with your posts.php file. You're probably doing this: if(!isset($_GET['id'])){ $id = 2; }else{ $id = $_GET['id']; } You'd want to be doing this now, because you're not declaring a $_GET superglobal, your declaring a standard variable. if(!isset($id)){ $id = 2; } You won't need to set it to itself again because it'll already be set.
-
Or you could just go: if($userdata['user_level'] == 2){ die('Wrong user level.'); } Or if you end up with a few levels that are restricted. $restricted = array(2, 4, 5, 7); // levels 2, 4, 5 and 7 can't access the page if(in_array($userdata['user_level'], $restricted)){ die('Wrong user level.'); }
-
Try declaring the $id variable prior to including your script. $id = 80; include('../mb/getposts/posts.php');
-
Parametrized Queries - Why are they not popular?
JasonLewis replied to bachx's topic in PHP Coding Help
Having never heard of them, I can only say that most people these days either opt for MySQLi or using a database abstraction layer such as PDO. Both of these offer what is called prepared statements. Of course, that link is for PDO, but MySQLi offers the same thing. Most people these days tend to use these methods for preventing SQL Injection. -
Because it's in the format of: border-bottom: 1px solid #000;
-
You need to create a RegExp object out of your location, and use the i modifier on it. I took what you gave me and created an example, hopefully this is what you're after. <script type="text/javascript"> // Create an example user inputted string. var loc = 'Rose'; // Taken from your example. var rloc = []; rloc[55068] = 'Rosemount, MN'; rloc[55124] = 'Apple Valley, MN'; rloc[55401] = 'Minneapolis, MN'; // Convert the location to a string. loc = loc.toString(); // Create our regexp. regex = new RegExp(loc, 'i'); // Now run the for loop. for(var l in rloc){ // Now we can test them. if( rloc[l].match(regex) !== null || l.match(regex) !== null ){ // Write out the matches. document.write(rloc[l] + '<br />'); } } </script> Try copy and pasting that into a blank page and running it, change the loc to something else and test it out. I tried it and it seemed to be working. What I've done is created a RegExp object from the loc, firstly converting it to a string. Then you use the rloc variables as the test subject. Good luck man.
-
netbeans teething troubles
JasonLewis replied to freelance84's topic in Editor Help (PhpStorm, VS Code, etc)
I guess it depends on how your application is set up. As far as I know you can't change the color of the heredoc. Just going to have to make do with it. -
netbeans teething troubles
JasonLewis replied to freelance84's topic in Editor Help (PhpStorm, VS Code, etc)
If you go into Tools > Options and go to Fonts & Colors you can adjust the colors to your liking. Change the Language from All Languages to PHP and scroll down to find string. Of course, it'll also affect strings inside the heredoc, which you'll see in the example. -
I pasted your code and ran it, I received the syntax errors which you should have received.
-
You should be get errors when you run this script. The errors will tell you where the problem is. Look at this line: echo ", <a href="modcp.php">Mod CP</a>"; You are opening a string with quotes, then inside the string you are using the quotes again. This is a no-no, because PHP thinks you are ending the string, and so it throws an error. You need to either escape the quotes, or use single quotes on the inside or outside. echo ", <a href=\"modcp.php\">Mod CP</a>"; or echo ", <a href='modcp.php'>Mod CP</a>"; or echo ', <a href="modcp.php">Mod CP</a>'; You do the same thing a few lines down. You really need to look at your errors and check the lines out, then do a quick search on Google for the error. Generally these syntax errors are very easy to fix.
-
There's been a few topics about this and I once created a semi-working path finding script when someone was asking about it. http://www.policyalmanac.org/games/aStarTutorial.htm It's not for PHP, but it shows you the basics and it shouldn't be too hard to convert it to PHP.
-
You should be escaping data before it goes into a database, using mysql_real_escape_string, so that characters such as a single quote are escaped. If you have very long text then you should be using TEXT as your column type, not VARCHAR. Just ensure you escape any data before inserting it.
-
Are Attitude Posts Getting More Frequent?
JasonLewis replied to johnsmith153's topic in Miscellaneous
I personally find his reaction hilarious, and a great addition to some of the posts you get on the forums. These members come and go, and I'm surprised it wasn't thorpe (no disrespect to thorpe ) who caused the drama this time. I think they feel insecure because the answer is general so clear, so they go way overboard and portray themselves as a bit of an idiot. -
When you create a floating element you take it out of the document flow and position it either to the far left or far right of its containing element. What you need to do is create another element beneath both of these content divs and clear the floats. Since they're both floated left, you can just use a line break and set an inline style on it. <br style="clear: left" />
-
Well, the only thing I can suggest is making sure they are strings with .toString(), and instead of using toLowerCase make your expressions case insensitive by using the i modifier.
-
I need a new computer, and a new TV as well I think, once I have them I'm good. It seems as though you are demanding code to be written for you, not going to happen like that mate.
-
With NetBeans you can use the navigator which keeps you updated on your methods and properties etc etc. I find that handy, there are also many debugging tools.
-
Files created from Windows box on Linux box don't get correct permissions.
JasonLewis replied to JasonLewis's topic in Linux
Okay, well its working so I shall just go with that. Cheers. -
Files created from Windows box on Linux box don't get correct permissions.
JasonLewis replied to JasonLewis's topic in Linux
This is what I have, which I ended up with after following a tutorial. [www] comment = www directory path = /var/www public = yes writable = yes valid users = jason create mask = 0771 directory mask = 0771 force user = jason force group = www So create mask should be 022? I change create mask = 0771 to 022 and now when I create files I get the following permissions: -----w--w- 1 jason www 0 2010-09-03 20:11 test.php NetBeans also says this: Cannot lock read-only file \\jcl-webserver\www\sites\home\htdocs\test.php Edit: I changed the create mask to 644 and now the permissions are set to the same as when created through SSH. I take it this is the right way? -
Okay, I set up samba so that it shares my /var/www directory so that I can gain access from my Windows box. The reason I did this was so that I could run NetBeans from my Windows box and edit files inside my /var/www directory. I've set up multiple sites so they are all stored in /var/www/sites/<sitename>/htdocs The problem is when I create a file from NetBeans it gives it incorrect permissions. Running ls -l from inside htdocs outputs this: -rw-r--r-- 1 jason www 19 2010-09-03 18:42 index.php drwxrws--x 3 jason www 4096 2010-09-03 18:34 nbproject -rwxrw---- 1 jason www 511 2010-09-03 18:43 netbeans_file.php index.php was created from SSH, and my linux isn't great but from looking at it index.php has read permissions for every group, and the owner can write too it (does it need execute?). However the netbeans_file.php (which was created from inside NetBeans), doesn't have any permissions for other. I'm kind of lost here, I can manually assign permissions to the file from SSH (chmod 644), however I'd prefer if files gained permissions when created. I'm not even sure where to start...
-
And are they expressions, consider the following example: var patterns = [/^New York$/i, /^12345$/]; var test_one = 'new york'; var test_two = 'new york city'; document.write(test_one.toString().match(patterns[0])); // will write new york document.write(test_two.toString().match(patterns[0])); // will write null